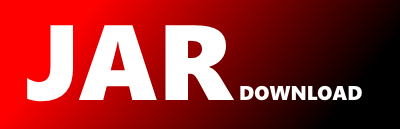
edu.isi.nlp.edl.ImmutableEDLMention Maven / Gradle / Ivy
package edu.isi.nlp.edl;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.common.base.Optional;
import com.google.common.base.Preconditions;
import com.google.common.collect.Lists;
import com.google.common.primitives.Doubles;
import edu.isi.nlp.strings.offsets.CharOffset;
import edu.isi.nlp.strings.offsets.OffsetRange;
import edu.isi.nlp.symbols.Symbol;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link EDLMention}.
*
* Use the builder to create immutable instances:
* {@code new EDLMention.Builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "EDLMention"})
@Immutable
final class ImmutableEDLMention extends EDLMention {
private final Symbol runId;
private final String mentionId;
private final Symbol documentID;
private final Optional kbId;
private final String headString;
private final OffsetRange headOffsets;
private final Symbol mentionType;
private final Symbol entityType;
private final double confidence;
private ImmutableEDLMention(
Symbol runId,
String mentionId,
Symbol documentID,
Optional kbId,
String headString,
OffsetRange headOffsets,
Symbol mentionType,
Symbol entityType,
double confidence) {
this.runId = runId;
this.mentionId = mentionId;
this.documentID = documentID;
this.kbId = kbId;
this.headString = headString;
this.headOffsets = headOffsets;
this.mentionType = mentionType;
this.entityType = entityType;
this.confidence = confidence;
}
/**
* @return The value of the {@code runId} attribute
*/
@JsonProperty("runId")
@Override
public Symbol runId() {
return runId;
}
/**
* @return The value of the {@code mentionId} attribute
*/
@JsonProperty("mentionId")
@Override
public String mentionId() {
return mentionId;
}
/**
* @return The value of the {@code documentID} attribute
*/
@JsonProperty("documentID")
@Override
public Symbol documentID() {
return documentID;
}
/**
* @return The value of the {@code kbId} attribute
*/
@JsonProperty("kbId")
@Override
public Optional kbId() {
return kbId;
}
/**
* @return The value of the {@code headString} attribute
*/
@JsonProperty("headString")
@Override
public String headString() {
return headString;
}
/**
* @return The value of the {@code headOffsets} attribute
*/
@JsonProperty("headOffsets")
@Override
public OffsetRange headOffsets() {
return headOffsets;
}
/**
* @return The value of the {@code mentionType} attribute
*/
@JsonProperty("mentionType")
@Override
public Symbol mentionType() {
return mentionType;
}
/**
* @return The value of the {@code entityType} attribute
*/
@JsonProperty("entityType")
@Override
public Symbol entityType() {
return entityType;
}
/**
* @return The value of the {@code confidence} attribute
*/
@JsonProperty("confidence")
@Override
public double confidence() {
return confidence;
}
/**
* Copy the current immutable object by setting a value for the {@link EDLMention#runId() runId} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for runId
* @return A modified copy of the {@code this} object
*/
public final ImmutableEDLMention withRunId(Symbol value) {
if (this.runId == value) return this;
Symbol newValue = Preconditions.checkNotNull(value, "runId");
return validate(new ImmutableEDLMention(
newValue,
this.mentionId,
this.documentID,
this.kbId,
this.headString,
this.headOffsets,
this.mentionType,
this.entityType,
this.confidence));
}
/**
* Copy the current immutable object by setting a value for the {@link EDLMention#mentionId() mentionId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for mentionId
* @return A modified copy of the {@code this} object
*/
public final ImmutableEDLMention withMentionId(String value) {
if (this.mentionId.equals(value)) return this;
String newValue = Preconditions.checkNotNull(value, "mentionId");
return validate(new ImmutableEDLMention(
this.runId,
newValue,
this.documentID,
this.kbId,
this.headString,
this.headOffsets,
this.mentionType,
this.entityType,
this.confidence));
}
/**
* Copy the current immutable object by setting a value for the {@link EDLMention#documentID() documentID} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for documentID
* @return A modified copy of the {@code this} object
*/
public final ImmutableEDLMention withDocumentID(Symbol value) {
if (this.documentID == value) return this;
Symbol newValue = Preconditions.checkNotNull(value, "documentID");
return validate(new ImmutableEDLMention(
this.runId,
this.mentionId,
newValue,
this.kbId,
this.headString,
this.headOffsets,
this.mentionType,
this.entityType,
this.confidence));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link EDLMention#kbId() kbId} attribute.
* @param value The value for kbId
* @return A modified copy of {@code this} object
*/
public final ImmutableEDLMention withKbId(String value) {
Optional newValue = Optional.of(value);
if (this.kbId.equals(newValue)) return this;
return validate(new ImmutableEDLMention(
this.runId,
this.mentionId,
this.documentID,
newValue,
this.headString,
this.headOffsets,
this.mentionType,
this.entityType,
this.confidence));
}
/**
* Copy the current immutable object by setting an optional value for the {@link EDLMention#kbId() kbId} attribute.
* An equality check is used to prevent copying of the same value by returning {@code this}.
* @param optional A value for kbId
* @return A modified copy of {@code this} object
*/
public final ImmutableEDLMention withKbId(Optional optional) {
Optional value = Preconditions.checkNotNull(optional, "kbId");
if (this.kbId.equals(value)) return this;
return validate(new ImmutableEDLMention(
this.runId,
this.mentionId,
this.documentID,
value,
this.headString,
this.headOffsets,
this.mentionType,
this.entityType,
this.confidence));
}
/**
* Copy the current immutable object by setting a value for the {@link EDLMention#headString() headString} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for headString
* @return A modified copy of the {@code this} object
*/
public final ImmutableEDLMention withHeadString(String value) {
if (this.headString.equals(value)) return this;
String newValue = Preconditions.checkNotNull(value, "headString");
return validate(new ImmutableEDLMention(
this.runId,
this.mentionId,
this.documentID,
this.kbId,
newValue,
this.headOffsets,
this.mentionType,
this.entityType,
this.confidence));
}
/**
* Copy the current immutable object by setting a value for the {@link EDLMention#headOffsets() headOffsets} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for headOffsets
* @return A modified copy of the {@code this} object
*/
public final ImmutableEDLMention withHeadOffsets(OffsetRange value) {
if (this.headOffsets == value) return this;
OffsetRange newValue = Preconditions.checkNotNull(value, "headOffsets");
return validate(new ImmutableEDLMention(
this.runId,
this.mentionId,
this.documentID,
this.kbId,
this.headString,
newValue,
this.mentionType,
this.entityType,
this.confidence));
}
/**
* Copy the current immutable object by setting a value for the {@link EDLMention#mentionType() mentionType} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for mentionType
* @return A modified copy of the {@code this} object
*/
public final ImmutableEDLMention withMentionType(Symbol value) {
if (this.mentionType == value) return this;
Symbol newValue = Preconditions.checkNotNull(value, "mentionType");
return validate(new ImmutableEDLMention(
this.runId,
this.mentionId,
this.documentID,
this.kbId,
this.headString,
this.headOffsets,
newValue,
this.entityType,
this.confidence));
}
/**
* Copy the current immutable object by setting a value for the {@link EDLMention#entityType() entityType} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for entityType
* @return A modified copy of the {@code this} object
*/
public final ImmutableEDLMention withEntityType(Symbol value) {
if (this.entityType == value) return this;
Symbol newValue = Preconditions.checkNotNull(value, "entityType");
return validate(new ImmutableEDLMention(
this.runId,
this.mentionId,
this.documentID,
this.kbId,
this.headString,
this.headOffsets,
this.mentionType,
newValue,
this.confidence));
}
/**
* Copy the current immutable object by setting a value for the {@link EDLMention#confidence() confidence} attribute.
* A value strict bits equality used to prevent copying of the same value by returning {@code this}.
* @param value A new value for confidence
* @return A modified copy of the {@code this} object
*/
public final ImmutableEDLMention withConfidence(double value) {
if (Double.doubleToLongBits(this.confidence) == Double.doubleToLongBits(value)) return this;
return validate(new ImmutableEDLMention(
this.runId,
this.mentionId,
this.documentID,
this.kbId,
this.headString,
this.headOffsets,
this.mentionType,
this.entityType,
value));
}
/**
* This instance is equal to all instances of {@code ImmutableEDLMention} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableEDLMention
&& equalTo((ImmutableEDLMention) another);
}
private boolean equalTo(ImmutableEDLMention another) {
return runId.equals(another.runId)
&& mentionId.equals(another.mentionId)
&& documentID.equals(another.documentID)
&& kbId.equals(another.kbId)
&& headString.equals(another.headString)
&& headOffsets.equals(another.headOffsets)
&& mentionType.equals(another.mentionType)
&& entityType.equals(another.entityType)
&& Double.doubleToLongBits(confidence) == Double.doubleToLongBits(another.confidence);
}
/**
* Computes a hash code from attributes: {@code runId}, {@code mentionId}, {@code documentID}, {@code kbId}, {@code headString}, {@code headOffsets}, {@code mentionType}, {@code entityType}, {@code confidence}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + runId.hashCode();
h = h * 17 + mentionId.hashCode();
h = h * 17 + documentID.hashCode();
h = h * 17 + kbId.hashCode();
h = h * 17 + headString.hashCode();
h = h * 17 + headOffsets.hashCode();
h = h * 17 + mentionType.hashCode();
h = h * 17 + entityType.hashCode();
h = h * 17 + Doubles.hashCode(confidence);
return h;
}
/**
* Prints the immutable value {@code EDLMention} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("EDLMention")
.omitNullValues()
.add("runId", runId)
.add("mentionId", mentionId)
.add("documentID", documentID)
.add("kbId", kbId.orNull())
.add("headString", headString)
.add("headOffsets", headOffsets)
.add("mentionType", mentionType)
.add("entityType", entityType)
.add("confidence", confidence)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends EDLMention {
@Nullable Symbol runId;
@Nullable String mentionId;
@Nullable Symbol documentID;
Optional kbId = Optional.absent();
@Nullable String headString;
@Nullable OffsetRange headOffsets;
@Nullable Symbol mentionType;
@Nullable Symbol entityType;
double confidence;
boolean confidenceIsSet;
@JsonProperty("runId")
public void setRunId(Symbol runId) {
this.runId = runId;
}
@JsonProperty("mentionId")
public void setMentionId(String mentionId) {
this.mentionId = mentionId;
}
@JsonProperty("documentID")
public void setDocumentID(Symbol documentID) {
this.documentID = documentID;
}
@JsonProperty("kbId")
public void setKbId(Optional kbId) {
this.kbId = kbId;
}
@JsonProperty("headString")
public void setHeadString(String headString) {
this.headString = headString;
}
@JsonProperty("headOffsets")
public void setHeadOffsets(OffsetRange headOffsets) {
this.headOffsets = headOffsets;
}
@JsonProperty("mentionType")
public void setMentionType(Symbol mentionType) {
this.mentionType = mentionType;
}
@JsonProperty("entityType")
public void setEntityType(Symbol entityType) {
this.entityType = entityType;
}
@JsonProperty("confidence")
public void setConfidence(double confidence) {
this.confidence = confidence;
this.confidenceIsSet = true;
}
@Override
public Symbol runId() { throw new UnsupportedOperationException(); }
@Override
public String mentionId() { throw new UnsupportedOperationException(); }
@Override
public Symbol documentID() { throw new UnsupportedOperationException(); }
@Override
public Optional kbId() { throw new UnsupportedOperationException(); }
@Override
public String headString() { throw new UnsupportedOperationException(); }
@Override
public OffsetRange headOffsets() { throw new UnsupportedOperationException(); }
@Override
public Symbol mentionType() { throw new UnsupportedOperationException(); }
@Override
public Symbol entityType() { throw new UnsupportedOperationException(); }
@Override
public double confidence() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator
static ImmutableEDLMention fromJson(Json json) {
EDLMention.Builder builder = new EDLMention.Builder();
if (json.runId != null) {
builder.runId(json.runId);
}
if (json.mentionId != null) {
builder.mentionId(json.mentionId);
}
if (json.documentID != null) {
builder.documentID(json.documentID);
}
if (json.kbId != null) {
builder.kbId(json.kbId);
}
if (json.headString != null) {
builder.headString(json.headString);
}
if (json.headOffsets != null) {
builder.headOffsets(json.headOffsets);
}
if (json.mentionType != null) {
builder.mentionType(json.mentionType);
}
if (json.entityType != null) {
builder.entityType(json.entityType);
}
if (json.confidenceIsSet) {
builder.confidence(json.confidence);
}
return (ImmutableEDLMention) builder.build();
}
private static ImmutableEDLMention validate(ImmutableEDLMention instance) {
instance.check();
return instance;
}
/**
* Creates an immutable copy of a {@link EDLMention} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable EDLMention instance
*/
public static EDLMention copyOf(EDLMention instance) {
if (instance instanceof ImmutableEDLMention) {
return (ImmutableEDLMention) instance;
}
return new EDLMention.Builder()
.from(instance)
.build();
}
/**
* Builds instances of type {@link EDLMention EDLMention}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
static class Builder {
private static final long INIT_BIT_RUN_ID = 0x1L;
private static final long INIT_BIT_MENTION_ID = 0x2L;
private static final long INIT_BIT_DOCUMENT_I_D = 0x4L;
private static final long INIT_BIT_HEAD_STRING = 0x8L;
private static final long INIT_BIT_HEAD_OFFSETS = 0x10L;
private static final long INIT_BIT_MENTION_TYPE = 0x20L;
private static final long INIT_BIT_ENTITY_TYPE = 0x40L;
private static final long INIT_BIT_CONFIDENCE = 0x80L;
private long initBits = 0xffL;
private @Nullable Symbol runId;
private @Nullable String mentionId;
private @Nullable Symbol documentID;
private Optional kbId = Optional.absent();
private @Nullable String headString;
private @Nullable OffsetRange headOffsets;
private @Nullable Symbol mentionType;
private @Nullable Symbol entityType;
private double confidence;
/**
* Creates a builder for {@link EDLMention EDLMention} instances.
*/
Builder() {
if (!(this instanceof EDLMention.Builder)) {
throw new UnsupportedOperationException("Use: new EDLMention.Builder()");
}
}
/**
* Fill a builder with attribute values from the provided {@code EDLMention} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final EDLMention.Builder from(EDLMention instance) {
Preconditions.checkNotNull(instance, "instance");
runId(instance.runId());
mentionId(instance.mentionId());
documentID(instance.documentID());
Optional kbIdOptional = instance.kbId();
if (kbIdOptional.isPresent()) {
kbId(kbIdOptional);
}
headString(instance.headString());
headOffsets(instance.headOffsets());
mentionType(instance.mentionType());
entityType(instance.entityType());
confidence(instance.confidence());
return (EDLMention.Builder) this;
}
/**
* Initializes the value for the {@link EDLMention#runId() runId} attribute.
* @param runId The value for runId
* @return {@code this} builder for use in a chained invocation
*/
public final EDLMention.Builder runId(Symbol runId) {
this.runId = Preconditions.checkNotNull(runId, "runId");
initBits &= ~INIT_BIT_RUN_ID;
return (EDLMention.Builder) this;
}
/**
* Initializes the value for the {@link EDLMention#mentionId() mentionId} attribute.
* @param mentionId The value for mentionId
* @return {@code this} builder for use in a chained invocation
*/
public final EDLMention.Builder mentionId(String mentionId) {
this.mentionId = Preconditions.checkNotNull(mentionId, "mentionId");
initBits &= ~INIT_BIT_MENTION_ID;
return (EDLMention.Builder) this;
}
/**
* Initializes the value for the {@link EDLMention#documentID() documentID} attribute.
* @param documentID The value for documentID
* @return {@code this} builder for use in a chained invocation
*/
public final EDLMention.Builder documentID(Symbol documentID) {
this.documentID = Preconditions.checkNotNull(documentID, "documentID");
initBits &= ~INIT_BIT_DOCUMENT_I_D;
return (EDLMention.Builder) this;
}
/**
* Initializes the optional value {@link EDLMention#kbId() kbId} to kbId.
* @param kbId The value for kbId
* @return {@code this} builder for chained invocation
*/
public final EDLMention.Builder kbId(String kbId) {
this.kbId = Optional.of(kbId);
return (EDLMention.Builder) this;
}
/**
* Initializes the optional value {@link EDLMention#kbId() kbId} to kbId.
* @param kbId The value for kbId
* @return {@code this} builder for use in a chained invocation
*/
public final EDLMention.Builder kbId(Optional kbId) {
this.kbId = Preconditions.checkNotNull(kbId, "kbId");
return (EDLMention.Builder) this;
}
/**
* Initializes the value for the {@link EDLMention#headString() headString} attribute.
* @param headString The value for headString
* @return {@code this} builder for use in a chained invocation
*/
public final EDLMention.Builder headString(String headString) {
this.headString = Preconditions.checkNotNull(headString, "headString");
initBits &= ~INIT_BIT_HEAD_STRING;
return (EDLMention.Builder) this;
}
/**
* Initializes the value for the {@link EDLMention#headOffsets() headOffsets} attribute.
* @param headOffsets The value for headOffsets
* @return {@code this} builder for use in a chained invocation
*/
public final EDLMention.Builder headOffsets(OffsetRange headOffsets) {
this.headOffsets = Preconditions.checkNotNull(headOffsets, "headOffsets");
initBits &= ~INIT_BIT_HEAD_OFFSETS;
return (EDLMention.Builder) this;
}
/**
* Initializes the value for the {@link EDLMention#mentionType() mentionType} attribute.
* @param mentionType The value for mentionType
* @return {@code this} builder for use in a chained invocation
*/
public final EDLMention.Builder mentionType(Symbol mentionType) {
this.mentionType = Preconditions.checkNotNull(mentionType, "mentionType");
initBits &= ~INIT_BIT_MENTION_TYPE;
return (EDLMention.Builder) this;
}
/**
* Initializes the value for the {@link EDLMention#entityType() entityType} attribute.
* @param entityType The value for entityType
* @return {@code this} builder for use in a chained invocation
*/
public final EDLMention.Builder entityType(Symbol entityType) {
this.entityType = Preconditions.checkNotNull(entityType, "entityType");
initBits &= ~INIT_BIT_ENTITY_TYPE;
return (EDLMention.Builder) this;
}
/**
* Initializes the value for the {@link EDLMention#confidence() confidence} attribute.
* @param confidence The value for confidence
* @return {@code this} builder for use in a chained invocation
*/
public final EDLMention.Builder confidence(double confidence) {
this.confidence = confidence;
initBits &= ~INIT_BIT_CONFIDENCE;
return (EDLMention.Builder) this;
}
/**
* Builds a new {@link EDLMention EDLMention}.
* @return An immutable instance of EDLMention
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public EDLMention build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return ImmutableEDLMention.validate(new ImmutableEDLMention(
runId,
mentionId,
documentID,
kbId,
headString,
headOffsets,
mentionType,
entityType,
confidence));
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if ((initBits & INIT_BIT_RUN_ID) != 0) attributes.add("runId");
if ((initBits & INIT_BIT_MENTION_ID) != 0) attributes.add("mentionId");
if ((initBits & INIT_BIT_DOCUMENT_I_D) != 0) attributes.add("documentID");
if ((initBits & INIT_BIT_HEAD_STRING) != 0) attributes.add("headString");
if ((initBits & INIT_BIT_HEAD_OFFSETS) != 0) attributes.add("headOffsets");
if ((initBits & INIT_BIT_MENTION_TYPE) != 0) attributes.add("mentionType");
if ((initBits & INIT_BIT_ENTITY_TYPE) != 0) attributes.add("entityType");
if ((initBits & INIT_BIT_CONFIDENCE) != 0) attributes.add("confidence");
return "Cannot build EDLMention, some of required attributes are not set " + attributes;
}
}
}