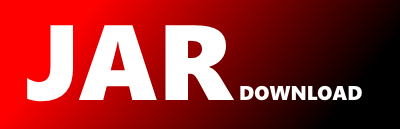
edu.isi.nlp.io.CachingOriginalTextSource Maven / Gradle / Ivy
package edu.isi.nlp.io;
import static com.google.common.base.Preconditions.checkNotNull;
import com.google.common.base.Optional;
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import edu.isi.nlp.files.KeyValueSource;
import edu.isi.nlp.symbols.Symbol;
import java.io.IOException;
import java.util.concurrent.ExecutionException;
/**
* This should be merged into the newer {@link KeyValueSource} code.
*
* @author Ryan Gabbard
*/
public final class CachingOriginalTextSource implements OriginalTextSource {
private final LoadingCache cache;
private CachingOriginalTextSource(final LoadingCache cache) {
this.cache = checkNotNull(cache);
}
public static OriginalTextSource from(final OriginalTextSource baseSource, int maxToCache) {
return new CachingOriginalTextSource(
CacheBuilder.newBuilder()
.maximumSize(maxToCache)
.build(
new CacheLoader() {
@Override
public String load(final Symbol key) throws Exception {
final Optional baseRet = baseSource.getOriginalText(key);
if (baseRet.isPresent()) {
return baseRet.get();
} else {
return null;
}
}
}));
}
@Override
public Optional getOriginalText(final Symbol docID) throws IOException {
try {
return Optional.fromNullable(cache.get(docID));
} catch (final ExecutionException e) {
if (e.getCause() instanceof IOException) {
throw (IOException) e.getCause();
} else {
throw new RuntimeException(e);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy