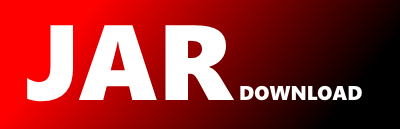
edu.isi.nlp.parsing.PatternMatchHeadRule Maven / Gradle / Ivy
package edu.isi.nlp.parsing;
import com.google.common.annotations.Beta;
import com.google.common.base.Function;
import com.google.common.base.Optional;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Iterables;
import edu.isi.nlp.ConstituentNode;
import edu.isi.nlp.symbols.Symbol;
import java.util.List;
import java.util.Map;
@Beta
final class PatternMatchHeadRule>
implements HeadRule {
private final boolean leftToRight;
private final ImmutableList candidateHeadSymbols;
private static final String LEFTTORIGHT = "1";
private static final String RIGHTTOLEFT = "0";
private PatternMatchHeadRule(final boolean leftToRight, final List candidateHeadSymbols) {
this.leftToRight = leftToRight;
this.candidateHeadSymbols = ImmutableList.copyOf(candidateHeadSymbols);
}
public static > PatternMatchHeadRule create(
final boolean leftToRight, List candidateHeadSymbols) {
return new PatternMatchHeadRule(leftToRight, candidateHeadSymbols);
}
public boolean leftToRight() {
return leftToRight;
}
@Override
public Optional matchForChildren(final Iterable childNodes) {
// get the children in the right order for this head rule
final ImmutableList children;
if (leftToRight()) {
children = ImmutableList.copyOf(childNodes);
} else {
children = ImmutableList.copyOf(childNodes).reverse();
}
for (final NodeT child : children) {
for (final String tag : candidateHeadSymbols) {
if (child.tag().asString().matches(tag)) {
return Optional.of(child);
}
}
}
if (candidateHeadSymbols.size() == 0) {
return Optional.fromNullable(Iterables.getFirst(children, null));
}
return Optional.absent();
}
public static >
Function>> fromHeadRuleFileLine() {
return new Function>>() {
@Override
public Map.Entry> apply(final String input) {
final ImmutableList lineParts = ImmutableList.copyOf(input.trim().split("\\s+"));
final Symbol parent = Symbol.from(lineParts.get(0));
final boolean leftToRight;
if (lineParts.get(1).equals(LEFTTORIGHT)) {
leftToRight = true;
} else if (lineParts.get(1).equals(RIGHTTOLEFT)) {
leftToRight = false;
} else {
throw new RuntimeException(
"Unexpected direction to move for head rule; options are 1 and 0 for left to right and visa versa, got "
+ lineParts.get(1));
}
final HeadRule rule = create(leftToRight, lineParts.subList(2, lineParts.size()));
return new Map.Entry>() {
@Override
public Symbol getKey() {
return parent;
}
@Override
public HeadRule getValue() {
return rule;
}
@Override
public HeadRule setValue(final HeadRule value) {
throw new UnsupportedOperationException("Cannot set the value here");
}
};
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy