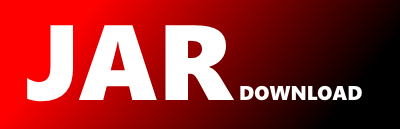
edu.jhu.hlt.util.Utilities Maven / Gradle / Ivy
package edu.jhu.hlt.util;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.regex.Pattern;
import edu.jhu.prim.util.random.Prng;
public class Utilities {
private static final double DEFAULT_DELTA = 1e-13;
private Utilities() {
// private constructor
}
private static final Integer INTEGER_ZERO = Integer.valueOf(0);
private static final Double DOUBLE_ZERO = Double.valueOf(0.0);
public static final double LOG2 = log(2);
public static Integer safeGetInt(Map map, X key) {
Integer value = map.get(key);
if (value == null) {
return INTEGER_ZERO;
}
return value;
}
public static Double safeGetDouble(Map map, X key) {
Double value = map.get(key);
if (value == null) {
return DOUBLE_ZERO;
}
return value;
}
public static boolean safeEquals(Object o1, Object o2) {
if (o1 == null || o2 == null) {
return o1 == o2;
} else {
return o1.equals(o2);
}
}
public static void increment(Map map, X key, Integer incr) {
if (map.containsKey(key)) {
Integer value = map.get(key);
map.put(key, value + incr);
} else {
map.put(key, incr);
}
}
public static void increment(Map map, X key, Double incr) {
if (map.containsKey(key)) {
Double value = map.get(key);
map.put(key, value + incr);
} else {
map.put(key, incr);
}
}
public static void increment(Map> map, X key1, Y key2, Integer incr) {
if (map.containsKey(key1)) {
Map subMap = map.get(key1);
increment(subMap, key2, incr);
} else {
Map subMap = new HashMap();
increment(subMap, key2, incr);
map.put(key1, subMap);
}
}
/**
* @return The resulting set
*/
public static Set addToSet(Map> map, X key, Y value) {
Set values;
if (map.containsKey(key)) {
values = map.get(key);
values.add(value);
} else {
values = new HashSet();
values.add(value);
map.put(key, values);
}
return values;
}
/**
* @return The resulting list.
*/
public static List addToList(Map> map, X key, Y value) {
List values;
if (map.containsKey(key)) {
values = map.get(key);
values.add(value);
} else {
values = new ArrayList();
values.add(value);
map.put(key, values);
}
return values;
}
public static List safeGetList(Map> map, X key) {
List list = map.get(key);
if (list == null) {
return Collections.emptyList();
} else {
return list;
}
}
/**
* Choose the with the greatest number of votes.
* @param The type of the thing being voted for.
* @param votes Maps to a Double representing the number of votes
* it received.
* @return The that received the most votes.
*/
public static List mostVotedFor(Map votes) {
// Choose the label with the greatest number of votes
// If there is a tie, choose the one with the least index
double maxTalley = Double.NEGATIVE_INFINITY;
List maxTickets = new ArrayList();
for(Entry entry : votes.entrySet()) {
X ticket = entry.getKey();
double talley = entry.getValue();
if (talley > maxTalley) {
maxTickets = new ArrayList();
maxTickets.add(ticket);
maxTalley = talley;
} else if (talley == maxTalley) {
maxTickets.add(ticket);
}
}
return maxTickets;
}
/**
* Creates a new list containing a slice of the original list.
* @param list The original list.
* @param start The index of the first element of the slice (inclusive).
* @param end The index of the last element of the slice (exclusive).
* @return A sublist containing elements [start, end).
*/
public static ArrayList sublist(List list, int start, int end) {
ArrayList sublist = new ArrayList();
for (int i=start; i= y.
*
* @param x log(p)
* @param y log(q)
* @return log(p - q) = log(exp(x) - exp(y))
* @throws IllegalStateException if x < y
*/
public static double logSubtract(double x, double y) {
return logSubtractExact(x,y);
}
public static double logAddExact(double x, double y) {
// p = 0 or q = 0, where x = log(p), y = log(q)
if (Double.NEGATIVE_INFINITY == x) {
return y;
} else if (Double.NEGATIVE_INFINITY == y) {
return x;
}
// p != 0 && q != 0
if (y <= x) {
return x + Math.log1p(exp(y - x));
} else {
return y + Math.log1p(exp(x - y));
}
}
/**
* Subtracts two probabilities that are stored as log probabilities.
* Note that x >= y.
*
* @param x log(p)
* @param y log(q)
* @return log(p - q) = log(exp(p) + exp(q))
* @throws IllegalStateException if x < y
*/
public static double logSubtractExact(double x, double y) {
if (x < y) {
throw new IllegalStateException("x must be >= y. x=" + x + " y=" + y);
}
// p = 0 or q = 0, where x = log(p), y = log(q)
if (Double.NEGATIVE_INFINITY == y) {
return x;
} else if (Double.NEGATIVE_INFINITY == x) {
return y;
}
// p != 0 && q != 0
return x + Math.log1p(-exp(y - x));
}
public static double log(double d) {
return Math.log(d);
}
public static double exp(double d) {
return Math.exp(d);
}
public static double log2(double d) {
return log(d) / LOG2;
}
public static double[][][][] copyOf(double[][][][] array) {
double[][][][] clone = new double[array.length][][][];
for (int i = 0; i < clone.length; i++) {
clone[i] = copyOf(array[i]);
}
return clone;
}
public static double[][][] copyOf(double[][][] array) {
double[][][] clone = new double[array.length][][];
for (int i = 0; i < clone.length; i++) {
clone[i] = copyOf(array[i]);
}
return clone;
}
public static double[][] copyOf(double[][] array) {
double[][] newArray = new double[array.length][];
for (int i = 0; i < array.length; i++) {
newArray[i] = Utilities.copyOf(array[i], array[i].length);
}
return newArray;
}
public static double[] copyOf(double[] original, int newLength) {
return Arrays.copyOf(original, newLength);
}
public static double[] copyOf(double[] original) {
return Arrays.copyOf(original, original.length);
}
public static boolean[][][][] copyOf(boolean[][][][] array) {
boolean[][][][] clone = new boolean[array.length][][][];
for (int i = 0; i < clone.length; i++) {
clone[i] = copyOf(array[i]);
}
return clone;
}
public static boolean[][][] copyOf(boolean[][][] array) {
boolean[][][] clone = new boolean[array.length][][];
for (int i = 0; i < clone.length; i++) {
clone[i] = copyOf(array[i]);
}
return clone;
}
public static boolean[][] copyOf(boolean[][] array) {
boolean[][] newArray = new boolean[array.length][];
for (int i = 0; i < array.length; i++) {
newArray[i] = Utilities.copyOf(array[i], array[i].length);
}
return newArray;
}
public static boolean[] copyOf(boolean[] original, int newLength) {
return Arrays.copyOf(original, newLength);
}
public static boolean[] copyOf(boolean[] original) {
return Arrays.copyOf(original, original.length);
}
public static int[][] copyOf(int[][] array) {
int[][] newArray = new int[array.length][];
for (int i = 0; i < array.length; i++) {
newArray[i] = Utilities.copyOf(array[i], array[i].length);
}
return newArray;
}
public static int[] copyOf(int[] original, int newLength) {
return Arrays.copyOf(original, newLength);
}
public static int[] copyOf(int[] original) {
return Arrays.copyOf(original, original.length);
}
public static long[] copyOf(long[] original, int newLength) {
return Arrays.copyOf(original, newLength);
}
public static long[] copyOf(long[] original) {
return Arrays.copyOf(original, original.length);
}
public static void copy(int[][] array, int[][] clone) {
assert (array.length == clone.length);
for (int i = 0; i < array.length; i++) {
copy(array[i], clone[i]);
}
}
public static void copy(int[] array, int[] clone) {
assert (array.length == clone.length);
System.arraycopy(array, 0, clone, 0, array.length);
}
public static void copy(double[] array, double[] clone) {
assert (array.length == clone.length);
System.arraycopy(array, 0, clone, 0, array.length);
}
public static void copy(double[][] array, double[][] clone) {
assert (array.length == clone.length);
for (int i = 0; i < clone.length; i++) {
copy(array[i], clone[i]);
}
}
public static void copy(double[][][] array, double[][][] clone) {
assert (array.length == clone.length);
for (int i = 0; i < clone.length; i++) {
copy(array[i], clone[i]);
}
}
public static void copy(double[][][][] array, double[][][][] clone) {
assert (array.length == clone.length);
for (int i = 0; i < clone.length; i++) {
copy(array[i], clone[i]);
}
}
public static double infinityNorm(double[][] gradient) {
double maxIN = 0.0;
for (int i=0; i maxIN) {
maxIN = tempVal;
}
}
return maxIN;
}
private static double infinityNorm(double[] gradient) {
double maxAbs = 0.0;
for (int i=0; i maxAbs) {
maxAbs = tempVal;
}
}
return maxAbs;
}
/**
* Fisher-Yates shuffle randomly reorders the elements in array. This
* is O(n) in the length of the array.
*/
public static void shuffle(int[] array) {
for (int i=array.length-1; i > 0; i--) {
int j = Prng.nextInt(i+1);
// Swap array[i] and array[j]
int tmp = array[i];
array[i] = array[j];
array[j] = tmp;
}
}
/**
* Samples a set of m integers without replacement from the range [0,...,n-1].
* @param m The number of integers to return.
* @param n The number of integers from which to sample.
* @return The sample as an unsorted integer array.
*/
public static int[] sampleWithoutReplacement(int m, int n) {
// This implements a modified form of the genshuf() function from
// Programming Pearls pg. 129.
// TODO: Design a faster method that only generates min(m, n-m) integers.
int[] array = getIndexArray(n);
for (int i=0; i b, and a value less than 0 if a < b.
*/
public static int compare(double a, double b, double delta) {
if (equals(a, b, delta)) {
return 0;
}
return Double.compare(a, b);
}
public static int compare(int a, int b) {
return a - b;
}
public static int compare(int[] x, int[] y) {
for (int i=0; i y.length) {
return 1;
} else {
return 0;
}
}
public static boolean lte(double a, double b) {
return a <= b + DEFAULT_DELTA;
}
public static boolean lte(double a, double b, double delta) {
return a <= b + delta;
}
public static boolean gte(double a, double b) {
return a + DEFAULT_DELTA >= b;
}
public static boolean gte(double a, double b, double delta) {
return a + delta >= b;
}
public static String deepToString(double[][] array) {
StringBuilder sb = new StringBuilder();
sb.append("[");
for (double[] arr : array) {
sb.append("[");
for (double a : arr) {
sb.append(String.format("%10.3g, ", a));
}
sb.append("], ");
}
sb.append("]");
return sb.toString();
}
/**
* Gets an array where array[i] = i.
* @param length The length of the array.
* @return The new index array.
*/
public static int[] getIndexArray(int length) {
int[] index = new int[length];
for (int i=0; i getMatchingFiles(File file, String regexStr) {
Pattern regex = Pattern.compile(regexStr);
return getMatchingFiles(file, regex);
}
private static List getMatchingFiles(File file, Pattern regex) {
ArrayList files = new ArrayList();
if (file.exists()) {
if (file.isFile()) {
if (regex.matcher(file.getName()).matches()) {
files.add(file);
}
} else {
for (File subFile : file.listFiles()) {
files.addAll(getMatchingFiles(subFile, regex));
}
}
}
return files;
}
public static void addAll(ArrayList list, T[] array) {
if (array == null) {
return;
}
list.addAll(Arrays.asList(array));
}
public static void addAll(ArrayList list, T[][] array) {
for (int i=0; i void addAll(ArrayList list, T[][][] array) {
for (int i=0; i void addAll(ArrayList list, T[][][][] array) {
for (int i=0; i 0) {
// array[0] = value;
// }
// for (int i = 1; i < n; i += i) {
// System.arraycopy(array, 0, array, i, ((n - i) < i) ? (n - i) : i);
// }
for (int i=0; i 0) {
// array[0] = value;
// }
// for (int i = 1; i < n; i += i) {
// System.arraycopy(array, 0, array, i, ((n - i) < i) ? (n - i) : i);
// }
for (int i=0; i 0) {
// array[0] = value;
// }
// for (int i = 1; i < n; i += i) {
// System.arraycopy(array, 0, array, i, ((n - i) < i) ? (n - i) : i);
// }
for (int i=0; i List getList(T... args) {
return Arrays.asList(args);
}
public static String toString(double[] array, String formatString) {
StringBuilder sb = new StringBuilder();
sb.append("{");
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy