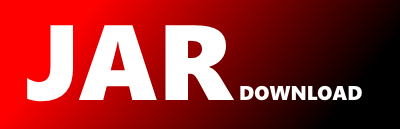
edu.kit.kastel.sdq.artemis4j.grading.model.annotation.Annotation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artemis4j Show documentation
Show all versions of artemis4j Show documentation
Artemis4J is a Java library for interacting with the Artemis teaching system.
/* Licensed under EPL-2.0 2022-2023. */
package edu.kit.kastel.sdq.artemis4j.grading.model.annotation;
import edu.kit.kastel.sdq.artemis4j.api.grading.IAnnotation;
import edu.kit.kastel.sdq.artemis4j.api.grading.IMistakeType;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Optional;
public class Annotation implements IAnnotation {
private final String uuid;
private IMistakeType mistakeType;
private String mistakeTypeId;
private final int startLine;
private final int endLine;
private final String fullyClassifiedClassName;
private final String customMessage;
private final Double customPenalty;
public Annotation(String uuid, IMistakeType mistakeType, int startLine, int endLine, String fullyClassifiedClassName, String customMessage,
Double customPenalty) {
this.uuid = uuid;
this.mistakeType = mistakeType;
this.startLine = startLine;
this.endLine = endLine;
this.fullyClassifiedClassName = fullyClassifiedClassName;
this.customMessage = customMessage;
this.customPenalty = customPenalty;
}
/**
* This Constructor is ONLY FOR DESERIALIZATION!
*/
@JsonCreator
public Annotation(@JsonProperty("uuid") String uuid, @JsonProperty("startLine") int startLine, @JsonProperty("endLine") int endLine,
@JsonProperty("classFilePath") String classFilePath, @JsonProperty("customMessageForJSON") String customMessage,
@JsonProperty("customPenaltyForJSON") Double customPenalty, @JsonProperty("mistakeTypeId") String mistakeTypeId) {
this.uuid = uuid == null ? IAnnotation.createID() : uuid;
this.startLine = startLine;
this.endLine = endLine;
this.fullyClassifiedClassName = classFilePath;
this.mistakeTypeId = mistakeTypeId;
this.customMessage = customMessage;
this.customPenalty = customPenalty;
}
@Override
public String getClassFilePath() {
return this.fullyClassifiedClassName;
}
@Override
@JsonIgnore
public Optional getCustomMessage() {
return this.customMessage == null ? Optional.empty() : Optional.of(this.customMessage);
}
public String getCustomMessageForJSON() {
return this.customMessage;
}
@Override
@JsonIgnore
public Optional getCustomPenalty() {
return this.customPenalty == null ? Optional.empty() : Optional.of(this.customPenalty);
}
public Double getCustomPenaltyForJSON() {
return this.customPenalty;
}
@Override
public int getEndLine() {
return this.endLine;
}
@Override
public String getUUID() {
return this.uuid;
}
@Override
@JsonIgnore
public IMistakeType getMistakeType() {
return this.mistakeType;
}
public String getMistakeTypeId() {
return this.mistakeType != null ? this.mistakeType.getIdentifier() : this.mistakeTypeId;
}
@Override
public int getStartLine() {
return this.startLine;
}
/**
* This Method is ONLY FOR DESERIALIZATION! If mistakeType is already set, this
* has no effect.
*/
public void setMistakeType(IMistakeType mistakeType) {
if (this.mistakeType != null) {
return;
}
this.mistakeType = mistakeType;
}
@Override
public String toString() {
return "Annotation [id=" + this.uuid + ", mistakeType=" + this.mistakeType + ", startLine=" + this.startLine + ", endLine=" + this.endLine
+ ", fullyClassifiedClassName=" + this.fullyClassifiedClassName + ", customMessage=" + this.customMessage + ", customPenalty="
+ this.customPenalty + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy