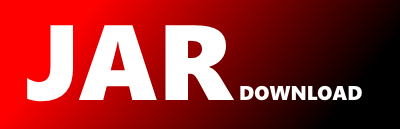
edu.mines.jtk.la.DMatrix Maven / Gradle / Ivy
/****************************************************************************
Copyright 2005, Colorado School of Mines and others.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
****************************************************************************/
package edu.mines.jtk.la;
import static edu.mines.jtk.util.ArrayMath.*;
import edu.mines.jtk.util.Check;
/**
* A double-precision matrix.
* Matrix elements are stored in an array of arrays of doubles a[m][n],
* such that array element a[i][j] corresponds to the i'th row and the
* j'th column of the m-by-n matrix.
*
* This class was adapted from the package Jama, which was developed by
* Joe Hicklin, Cleve Moler, and Peter Webb of The MathWorks, Inc., and by
* Ronald Boisvert, Bruce Miller, Roldan Pozo, and Karin Remington of the
* National Institue of Standards and Technology.
* @author Dave Hale, Colorado School of Mines
* @version 2005.12.01
*/
public class DMatrix {
/**
* Constructs an m-by-n matrix of zeros.
* @param m the number of rows.
* @param n the number of columns.
*/
public DMatrix(int m, int n) {
_m = m;
_n = n;
_a = new double[m][n];
}
/**
* Constructs an m-by-n matrix filled with the specified value.
* @param m the number of rows.
* @param n the number of columns.
* @param v the value.
*/
public DMatrix(int m, int n, double v) {
this(m,n);
fill(v,_a);
}
/**
* Constructs a matrix from the specified array. Does not copy array
* elements into a new array. Rather, the new matrix simply references
* the specified array.
*
* The specified array must be regular. That is, each row much contain
* the same number of columns, and each column must contain the same
* number of rows.
* @param a the array.
*/
public DMatrix(double[][] a) {
Check.argument(isRegular(a),"array a is regular");
_m = a.length;
_n = a[0].length;
_a = a;
}
/**
* Constructs a copy of the specified matrix.
* @param a the matrix.
*/
public DMatrix(DMatrix a) {
this(a._m,a._n, copy(a._a));
}
/**
* Gets the number of rows in this matrix.
* @return the number of rows.
*/
public int getM() {
return _m;
}
/**
* Gets the number of rows in this matrix.
* @return the number of rows.
*/
public int getRowCount() {
return _m;
}
/**
* Gets the number of columns in this matrix.
* @return the number of columns.
*/
public int getN() {
return _n;
}
/**
* Gets the number of columns in this matrix.
* @return the number of columns.
*/
public int getColumnCount() {
return _n;
}
/**
* Gets the array in which matrix elements are stored.
* @return the array; by reference, not by copy.
*/
public double[][] getArray() {
return _a;
}
/**
* Determines whether this matrix is square.
* @return true, if square; false, otherwise.
*/
public boolean isSquare() {
return _m==_n;
}
/**
* Determines whether this matrix is symmetric (and square).
* @return true, if symmetric (and square); false, otherwise.
*/
public boolean isSymmetric() {
if (!isSquare())
return false;
for (int i=0; i<_n; ++i)
for (int j=i+1; j<_n; ++j)
if (_a[i][j]!=_a[j][i])
return false;
return true;
}
/**
* Gets all elements of this matrix into a new array.
* @return the array.
*/
public double[][] get() {
return copy(_a);
}
/**
* Gets all elements of this matrix into the specified array.
* @param a the array.
*/
public void get(double[][] a) {
copy(_a,a);
}
/**
* Gets a matrix element.
* @param i the row index.
* @param j the column index.
* @return the element.
*/
public double get(int i, int j) {
return _a[i][j];
}
/**
* Gets the specified submatrix a[i0:i1][j0:j1] of this matrix.
* @param i0 the index of first row.
* @param i1 the index of last row.
* @param j0 the index of first column.
* @param j1 the index of last column.
* @return the submatrix.
*/
public DMatrix get(int i0, int i1, int j0, int j1) {
checkI(i0,i1);
checkJ(j0,j1);
int m = i1-i0+1;
int n = j1-j0+1;
DMatrix x = new DMatrix(m,n);
copy(n,m,j0,i0,_a,0,0,x._a);
return x;
}
/**
* Gets a new matrix from the specified rows and columns of this matrix.
* @param r the array of row indices; null, for all rows.
* @param c the array of column indices; null, for all columns.
* @return the matrix.
*/
public DMatrix get(int[] r, int[] c) {
if (r==null && c==null) {
return new DMatrix(_m,_n,copy(_a));
} else {
int m = (r!=null)?r.length:_m;
int n = (c!=null)?c.length:_n;
double[][] b = new double[m][n];
if (r==null) {
for (int i=0; i>>32));
}
}
return h;
}
public String toString() {
String ls = System.getProperty("line.separator");
StringBuilder sb = new StringBuilder();
String[][] s = format(_a);
int max = maxlen(s);
String format = "%"+max+"s";
sb.append("[[");
int ncol = 77/(max+2);
if (ncol>=5)
ncol = (ncol/5)*5;
for (int i=0; i<_m; ++i) {
int nrow = 1+(_n-1)/ncol;
if (i>0)
sb.append(" [");
for (int irow=0,j=0; irow=_m)
Check.argument(0<=i && i<_m,"row index i="+i+" is in bounds");
}
private void checkJ(int j) {
if (j<0 || j>=_n)
Check.argument(0<=j && j<_n,"column index j="+j+" is in bounds");
}
private void checkI(int i0, int i1) {
checkI(i0); checkI(i1); Check.argument(i0<=i1,"i0<=i1");
}
private void checkJ(int j0, int j1) {
checkJ(j0); checkJ(j1); Check.argument(j0<=j1,"j0<=j1");
}
// Used in implementation of toString() above.
private static String[][] format(double[][] d) {
int m = d.length;
int n = d[0].length;
int pg = 6;
String fg = "% ."+pg+"g";
int pemax = -1;
int pfmax = -1;
for (int i=0; i7)?ls-7:0;
if (pemax=0)?ls-1-ip:0;
if (pfmax=0) {
if (pfmax>pg-1)
pfmax = pg-1;
int pe = (pemax>pfmax)?pemax:pfmax;
f = "% ."+pe+"e";
} else {
int pf = pfmax;
f = "% ."+pf+"f";
}
for (int i=0; i0) {
while (ibeg>0 && s.charAt(ibeg-1)=='0')
--ibeg;
if (ibeg>0 && s.charAt(ibeg-1)=='.')
--ibeg;
}
if (ibeg