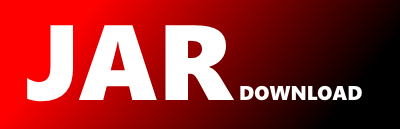
edu.mines.jtk.mosaic.PlotPanelPixels3 Maven / Gradle / Ivy
Show all versions of edu-mines-jtk Show documentation
/****************************************************************************
Copyright 2007, Colorado School of Mines and others.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
****************************************************************************/
package edu.mines.jtk.mosaic;
import java.awt.*;
import java.awt.image.IndexColorModel;
import edu.mines.jtk.awt.ColorMap;
import edu.mines.jtk.dsp.Sampling;
import edu.mines.jtk.util.*;
import static edu.mines.jtk.util.ArrayMath.*;
/**
* A plot panel with three pixels views of slices from a 3-D array.
*
* Pixels views are arranged in one of four ways, depending on the
* orientation of this panel. All arrangements are L-shaped with an
* empty tile in the upper-right corner of a 2x2 mosaic contained in
* this panel.
*
* This class has numerous methods that enable changing various attributes
* of the three pixels views while keeping them consistent. Although such
* attributes can be set independently for each pixels view, one should
* use the methods in this class when possible.
*
* @author Dave Hale, Colorado School of Mines
* @version 2007.08.11
*/
public class PlotPanelPixels3 extends PlotPanel {
private static final long serialVersionUID = 1L;
/**
* Orientations of the plot panel are defined by the orientation of the
* tile in the lower-left corner of the 2x2 mosaic. The four possible
* orientations are X1DOWN_X2RIGHT, X1DOWN_X3RIGHT, X1RIGHT_X2UP, and
* X1RIGHT_X3UP. The other two tiles are oriented so that they share
* axes with the lower-left tile.
*
* The orientations of the x2 and x3 axes can be determined automatically
* from that of the x1 axis to minimize the size of the empty tile in
* the upper-right corner. The two possible orientations of the x1 axis
* are X1DOWN and X1RIGHT. If one of these is specified, then one of the
* other four orientations will automatically be chosen accordingly.
*
* The default orientation is X1DOWN, which will result in either an
* X1DOWN_X2RIGHT or X1DOWN_X3RIGHT orientation being used.
*/
public enum Orientation {
X1DOWN,
X1DOWN_X2RIGHT,
X1DOWN_X3RIGHT,
X1RIGHT,
X1RIGHT_X2UP,
X1RIGHT_X3UP
}
/**
* Placement of labeled axes. The default axes placement is LEFT_BOTTOM.
*/
public enum AxesPlacement {
LEFT_BOTTOM,
NONE
}
/**
* Constructs a plot panel with three pixels views.
* @param orientation the orientation of views.
* @param axesPlacement the placement of axes.
* @param s1 sampling of the 1st dimension.
* @param s2 sampling of the 2nd dimension.
* @param s3 sampling of the 3rd dimension.
* @param f 3-D array of floats.
*/
public PlotPanelPixels3(
Orientation orientation, AxesPlacement axesPlacement,
Sampling s1, Sampling s2, Sampling s3, float[][][] f)
{
this(orientation,axesPlacement,s1,s2,s3,new SimpleFloat3(f));
}
/**
* Constructs a plot panel with three pixels views.
* @param orientation the orientation of views.
* @param axesPlacement the placement of axes.
* @param s1 sampling of the 1st dimension.
* @param s2 sampling of the 2nd dimension.
* @param s3 sampling of the 3rd dimension.
* @param f array of 3-D arrays of floats.
*/
public PlotPanelPixels3(
Orientation orientation, AxesPlacement axesPlacement,
Sampling s1, Sampling s2, Sampling s3, float[][][][] f)
{
this(orientation,axesPlacement,s1,s2,s3,toFloat3(f));
}
private static Float3[] toFloat3(float[][][][] f) {
Float3[] f3 = new Float3[f.length];
for (int i=0; i_n3) ?
Orientation.X1DOWN_X2RIGHT :
Orientation.X1DOWN_X3RIGHT;
} else if (orientation==Orientation.X1RIGHT) {
orientation = (_n2>_n3) ?
Orientation.X1RIGHT_X2UP :
Orientation.X1RIGHT_X3UP;
}
_orientation = orientation;
_axesPlacement = axesPlacement;
if (orientation==Orientation.X1DOWN_X2RIGHT) {
_transpose23 = false;
_p12 = addPixels(1,0,s1,s2,slice12());
_p13 = addPixels(1,1,s1,s3,slice13());
_p23 = addPixels(0,0,s2,s3,slice23());
_p12.setOrientation(PixelsView.Orientation.X1DOWN_X2RIGHT);
_p13.setOrientation(PixelsView.Orientation.X1DOWN_X2RIGHT);
_p23.setOrientation(PixelsView.Orientation.X1RIGHT_X2UP);
_l12 = addPoints(1,0,lines12a(),lines12b());
_l13 = addPoints(1,1,lines13a(),lines13b());
_l23 = addPoints(0,0,lines23a(),lines23b());
_l12.setOrientation(PointsView.Orientation.X1DOWN_X2RIGHT);
_l13.setOrientation(PointsView.Orientation.X1DOWN_X2RIGHT);
_l23.setOrientation(PointsView.Orientation.X1RIGHT_X2UP);
getMosaic().setWidthElastic( 0,100*_n2/_n3);
getMosaic().setHeightElastic(0,100*_n3/_n1);
} else if (orientation==Orientation.X1DOWN_X3RIGHT) {
_transpose23 = true;
_p12 = addPixels(1,1,s1,s2,slice12());
_p13 = addPixels(1,0,s1,s3,slice13());
_p23 = addPixels(0,0,s3,s2,slice23());
_p12.setOrientation(PixelsView.Orientation.X1DOWN_X2RIGHT);
_p13.setOrientation(PixelsView.Orientation.X1DOWN_X2RIGHT);
_p23.setOrientation(PixelsView.Orientation.X1RIGHT_X2UP);
_l12 = addPoints(1,1,lines12a(),lines12b());
_l13 = addPoints(1,0,lines13a(),lines13b());
_l23 = addPoints(0,0,lines23a(),lines23b());
_l12.setOrientation(PointsView.Orientation.X1DOWN_X2RIGHT);
_l13.setOrientation(PointsView.Orientation.X1DOWN_X2RIGHT);
_l23.setOrientation(PointsView.Orientation.X1RIGHT_X2UP);
getMosaic().setWidthElastic( 0,100*_n3/_n2);
getMosaic().setHeightElastic(0,100*_n2/_n1);
} else if (orientation==Orientation.X1RIGHT_X2UP) {
_transpose23 = true;
_p12 = addPixels(1,0,s1,s2,slice12());
_p13 = addPixels(0,0,s1,s3,slice13());
_p23 = addPixels(1,1,s3,s2,slice23());
_p12.setOrientation(PixelsView.Orientation.X1RIGHT_X2UP);
_p13.setOrientation(PixelsView.Orientation.X1RIGHT_X2UP);
_p23.setOrientation(PixelsView.Orientation.X1RIGHT_X2UP);
_l12 = addPoints(1,0,lines12a(),lines12b());
_l13 = addPoints(0,0,lines13a(),lines13b());
_l23 = addPoints(1,1,lines23a(),lines23b());
_l12.setOrientation(PointsView.Orientation.X1RIGHT_X2UP);
_l13.setOrientation(PointsView.Orientation.X1RIGHT_X2UP);
_l23.setOrientation(PointsView.Orientation.X1RIGHT_X2UP);
getMosaic().setWidthElastic( 0,100*_n1/_n3);
getMosaic().setHeightElastic(0,100*_n3/_n2);
} else if (orientation==Orientation.X1RIGHT_X3UP) {
_transpose23 = false;
_p12 = addPixels(0,0,s1,s2,slice12());
_p13 = addPixels(1,0,s1,s3,slice13());
_p23 = addPixels(1,1,s2,s3,slice23());
_p12.setOrientation(PixelsView.Orientation.X1RIGHT_X2UP);
_p13.setOrientation(PixelsView.Orientation.X1RIGHT_X2UP);
_p23.setOrientation(PixelsView.Orientation.X1RIGHT_X2UP);
_l12 = addPoints(0,0,lines12a(),lines12b());
_l13 = addPoints(1,0,lines13a(),lines13b());
_l23 = addPoints(1,1,lines23a(),lines23b());
_l12.setOrientation(PointsView.Orientation.X1RIGHT_X2UP);
_l13.setOrientation(PointsView.Orientation.X1RIGHT_X2UP);
_l23.setOrientation(PointsView.Orientation.X1RIGHT_X2UP);
getMosaic().setWidthElastic( 0,100*_n1/_n2);
getMosaic().setHeightElastic(0,100*_n2/_n3);
}
}
private float[][][] slice12() {
float[][][] x = new float[_nc][_n2][_n1];
for (int ic=0; ic<_nc; ++ic)
_f3[ic].get12(_n1,_n2,0,0,_k3,x[ic]);
return x;
}
private float[][][] slice13() {
float[][][] x = new float[_nc][_n3][_n1];
for (int ic=0; ic<_nc; ++ic)
_f3[ic].get13(_n1,_n3,0,_k2,0,x[ic]);
return x;
}
private float[][][] slice23() {
float[][][] x = new float[_nc][][];
float[][] xic = new float[_n3][_n2];
for (int ic=0; ic<_nc; ++ic) {
_f3[ic].get23(_n2,_n3,_k1,0,0,xic);
x[ic] = (_transpose23)?transpose(xic):copy(xic);
}
return x;
}
private float[][] lines12a() {
float xa1 = (float)_s1.getValue(0);
float xk1 = (float)_s1.getValue(_k1);
float xb1 = (float)_s1.getValue(_n1-1);
return new float[][]{{xk1,xk1},{xa1,xb1}};
}
private float[][] lines12b() {
float xa2 = (float)_s2.getValue(0);
float xk2 = (float)_s2.getValue(_k2);
float xb2 = (float)_s2.getValue(_n2-1);
return new float[][]{{xa2,xb2},{xk2,xk2}};
}
private float[][] lines13a() {
float xa1 = (float)_s1.getValue(0);
float xk1 = (float)_s1.getValue(_k1);
float xb1 = (float)_s1.getValue(_n1-1);
return new float[][]{{xk1,xk1},{xa1,xb1}};
}
private float[][] lines13b() {
float xa3 = (float)_s3.getValue(0);
float xk3 = (float)_s3.getValue(_k3);
float xb3 = (float)_s3.getValue(_n3-1);
return new float[][]{{xa3,xb3},{xk3,xk3}};
}
private float[][] lines23a() {
if (_transpose23) {
float xa3 = (float)_s3.getValue(0);
float xk3 = (float)_s3.getValue(_k3);
float xb3 = (float)_s3.getValue(_n3-1);
return new float[][]{{xk3,xk3},{xa3,xb3}};
} else {
float xa2 = (float)_s2.getValue(0);
float xk2 = (float)_s2.getValue(_k2);
float xb2 = (float)_s2.getValue(_n2-1);
return new float[][]{{xk2,xk2},{xa2,xb2}};
}
}
private float[][] lines23b() {
if (_transpose23) {
float xa2 = (float)_s2.getValue(0);
float xk2 = (float)_s2.getValue(_k2);
float xb2 = (float)_s2.getValue(_n2-1);
return new float[][]{{xa2,xb2},{xk2,xk2}};
} else {
float xa3 = (float)_s3.getValue(0);
float xk3 = (float)_s3.getValue(_k3);
float xb3 = (float)_s3.getValue(_n3-1);
return new float[][]{{xa3,xb3},{xk3,xk3}};
}
}
private void showLines() {
Mosaic mosaic = getMosaic();
Tile t00 = mosaic.getTile(0,0);
Tile t10 = mosaic.getTile(1,0);
Tile t11 = mosaic.getTile(1,1);
if (_orientation==Orientation.X1DOWN_X2RIGHT) {
t00.addTiledView(_l23);
t10.addTiledView(_l12);
t11.addTiledView(_l13);
} else if (_orientation==Orientation.X1DOWN_X3RIGHT) {
t00.addTiledView(_l23);
t10.addTiledView(_l13);
t11.addTiledView(_l12);
} else if (_orientation==Orientation.X1RIGHT_X2UP) {
t00.addTiledView(_l13);
t10.addTiledView(_l12);
t11.addTiledView(_l23);
} else if (_orientation==Orientation.X1RIGHT_X3UP) {
t00.addTiledView(_l12);
t10.addTiledView(_l13);
t11.addTiledView(_l23);
}
}
private void hideLines() {
Mosaic mosaic = getMosaic();
Tile t00 = mosaic.getTile(0,0);
Tile t10 = mosaic.getTile(1,0);
Tile t11 = mosaic.getTile(1,1);
if (_orientation==Orientation.X1DOWN_X2RIGHT) {
t00.removeTiledView(_l23);
t10.removeTiledView(_l12);
t11.removeTiledView(_l13);
} else if (_orientation==Orientation.X1DOWN_X3RIGHT) {
t00.removeTiledView(_l23);
t10.removeTiledView(_l13);
t11.removeTiledView(_l12);
} else if (_orientation==Orientation.X1RIGHT_X2UP) {
t00.removeTiledView(_l13);
t10.removeTiledView(_l12);
t11.removeTiledView(_l23);
} else if (_orientation==Orientation.X1RIGHT_X3UP) {
t00.removeTiledView(_l12);
t10.removeTiledView(_l13);
t11.removeTiledView(_l23);
}
}
TileAxis[] getAxes(int i) {
Mosaic mosaic = getMosaic();
TileAxis al0 = mosaic.getTileAxisLeft(0);
TileAxis al1 = mosaic.getTileAxisLeft(1);
TileAxis ab0 = mosaic.getTileAxisBottom(0);
TileAxis ab1 = mosaic.getTileAxisBottom(1);
TileAxis[] a = null;
if (_axesPlacement==AxesPlacement.NONE) {
a = new TileAxis[]{};
} else if (_orientation==Orientation.X1DOWN_X2RIGHT) {
if (i==1) a = new TileAxis[]{al1};
if (i==2) a = new TileAxis[]{ab0};
if (i==3) a = new TileAxis[]{al0,ab1};
} else if (_orientation==Orientation.X1DOWN_X3RIGHT) {
if (i==1) a = new TileAxis[]{al1};
if (i==2) a = new TileAxis[]{al0,ab1};
if (i==3) a = new TileAxis[]{ab0};
} else if (_orientation==Orientation.X1RIGHT_X2UP) {
if (i==1) a = new TileAxis[]{ab0};
if (i==2) a = new TileAxis[]{al1};
if (i==3) a = new TileAxis[]{al0,ab1};
} else if (_orientation==Orientation.X1RIGHT_X3UP) {
if (i==1) a = new TileAxis[]{ab0};
if (i==2) a = new TileAxis[]{al0,ab1};
if (i==3) a = new TileAxis[]{al1};
}
return a;
}
}