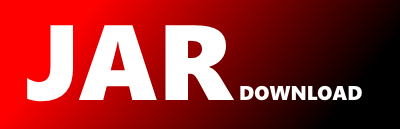
edu.mines.jtk.util.UnitsParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of edu-mines-jtk Show documentation
Show all versions of edu-mines-jtk Show documentation
Java packages for science and engineering
The newest version!
/****************************************************************************
Copyright 2006, Colorado School of Mines and others.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
****************************************************************************/
/****************************************************************************
All classes in this file UnitsParser.java were generated by Dave Hale
in 1988 using JavaCC and the grammar defined in UnitsParser.jj. JavaCC
produced this UnitsParser.java and several other files for classes with
names like Token, ASCII_CharStream, and ParseException. In 2006, Zachary
Pember at the Colorado School of Mines made those other classes static
inner classes of UnitsParser in this file.
****************************************************************************/
package edu.mines.jtk.util;
import java.io.StringReader;
/**
* Parser (generated from UnitsParser.jj via JavaCC) to create Units
* from a string that conforms to the following (extended BNF) grammar:
*
* unit -> expr EOF
* expr -> term ( ("+" | "-") (DOUBLE|INTEGER) )?
* term -> factor ( ("*")? factor | "/" factor )*
* factor -> primary ("^" INTEGER)?
* primary -> NAME | (DOUBLE|INTEGER) | "(" expr ")"
*
*
* @author Dave Hale and Zach Pember, Colorado School of Mines
* @version 1998.07.06, 2006.07.20
*/
final class UnitsParser {
/**
* Parse a String containing a unit definition.
* @param definition the unit definition, as in "coulomb/volt".
* @exception ParseException if the definition is not valid.
*/
static synchronized Units parse(String definition) throws ParseException {
ReInit(new StringReader(definition));
return units();
}
static final public Units units() throws ParseException {
Units e;
e = expr();
jj_consume_token(0);
return e;
//{if (true) return e;}
//throw new Error("Missing return statement in function");
}
static final public Units expr() throws ParseException {
Units t;
Token n;
t = term();
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case PLUS:
case MINUS:
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case PLUS:
jj_consume_token(PLUS);
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case DOUBLE:
n = jj_consume_token(DOUBLE);
break;
case INTEGER:
n = jj_consume_token(INTEGER);
break;
default:
jj_la1[0] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
t.shift(Double.valueOf(n.image).doubleValue());
break;
case MINUS:
jj_consume_token(MINUS);
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case DOUBLE:
n = jj_consume_token(DOUBLE);
break;
case INTEGER:
n = jj_consume_token(INTEGER);
break;
default:
jj_la1[1] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
t.shift(-Double.valueOf(n.image).doubleValue());
break;
default:
jj_la1[2] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
default:
jj_la1[3] = jj_gen;
;
}
return t;
//{if (true) return t;}
//throw new Error("Missing return statement in function");
}
static final public Units term() throws ParseException {
Units f,fb;
f = factor();
label_1:
while (true) {
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case MUL:
case DIV:
case LP:
case NAME:
case INTEGER:
case DOUBLE:
;
break;
default:
jj_la1[4] = jj_gen;
break label_1;
}
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case MUL:
case LP:
case NAME:
case INTEGER:
case DOUBLE:
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case MUL:
jj_consume_token(MUL);
break;
default:
jj_la1[5] = jj_gen;
;
}
fb = factor();
f.mul(fb);
break;
case DIV:
jj_consume_token(DIV);
fb = factor();
f.div(fb);
break;
default:
jj_la1[6] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
return f;
//{if (true) return f;}
//throw new Error("Missing return statement in function");
}
static final public Units factor() throws ParseException {
Units p;
Token n;
p = primary();
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case POW:
jj_consume_token(POW);
n = jj_consume_token(INTEGER);
p.pow(Integer.valueOf(n.image).intValue());
break;
default:
jj_la1[7] = jj_gen;
;
}
return p;
//{if (true) return p;}
//throw new Error("Missing return statement in function");
}
static final public Units primary() throws ParseException {
Units e,p;
Token n;
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case NAME:
n = jj_consume_token(NAME);
p = Units.unitsFromName(n.image);
if (p==null) {if (true) throw new ParseException("Units /" +n.image+
"/ are undefined.");}
return p;
//{if (true) return p;}
// break;
case INTEGER:
case DOUBLE:
switch ((jj_ntk==-1)?jj_ntk():jj_ntk) {
case DOUBLE:
n = jj_consume_token(DOUBLE);
break;
case INTEGER:
n = jj_consume_token(INTEGER);
break;
default:
jj_la1[8] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
double d = (new Double(n.image)).doubleValue();
return (new Units()).scale(d);
//{if (true) return (new Units()).scale(d);}
// break;
case LP:
jj_consume_token(LP);
e = expr();
jj_consume_token(RP);
return e;
//{if (true) return e;}
// break;
default:
jj_la1[9] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
//throw new Error("Missing return statement in function");
}
static private boolean jj_initialized_once = false;
static public UnitsParserTokenManager token_source;
static public ASCII_CharStream jj_input_stream;
static public Token token, jj_nt;
static private int jj_ntk;
static private int jj_gen;
static final private int[] jj_la1 = new int[10];
static final private int[] jj_la1_0 = {0x1800,0x1800,0x18,0x18,0x1d60,0x20,
0x1d60,0x80,0x1800,0x1d00,};
public UnitsParser(java.io.InputStream stream) {
if (jj_initialized_once) {
System.out.println("ERROR: Second call to constructor of static parser. "
+ "You must");
System.out.println(" either use ReInit() or set the JavaCC option "
+ "STATIC to false");
System.out.println(" during parser generation.");
throw new Error();
}
jj_initialized_once = true;
jj_input_stream = new ASCII_CharStream(stream, 1, 1);
token_source = new UnitsParserTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 10; i++) jj_la1[i] = -1;
}
static public void ReInit(java.io.InputStream stream) {
ASCII_CharStream.ReInit(stream, 1, 1);
UnitsParserTokenManager.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 10; i++) jj_la1[i] = -1;
}
public UnitsParser(java.io.Reader stream) {
if (jj_initialized_once) {
System.out.println("ERROR: Second call to constructor of static parser. "
+ "You must");
System.out.println(" either use ReInit() or set the JavaCC option "
+ "STATIC to false");
System.out.println(" during parser generation.");
throw new Error();
}
jj_initialized_once = true;
jj_input_stream = new ASCII_CharStream(stream, 1, 1);
token_source = new UnitsParserTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 10; i++) jj_la1[i] = -1;
}
static public void ReInit(java.io.Reader stream) {
ASCII_CharStream.ReInit(stream, 1, 1);
UnitsParserTokenManager.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 10; i++) jj_la1[i] = -1;
}
public UnitsParser(UnitsParserTokenManager tm) {
if (jj_initialized_once) {
System.out.println("ERROR: Second call to constructor of static parser. "
+ "You must");
System.out.println(" either use ReInit() or set the JavaCC option "
+ "STATIC to false");
System.out.println(" during parser generation.");
throw new Error();
}
jj_initialized_once = true;
token_source = tm;
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 10; i++) jj_la1[i] = -1;
}
public void ReInit(UnitsParserTokenManager tm) {
token_source = tm;
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 10; i++) jj_la1[i] = -1;
}
static final private Token jj_consume_token(int kind) throws ParseException {
Token oldToken;
if ((oldToken = token).next != null) token = token.next;
else token = token.next = UnitsParserTokenManager.getNextToken();
jj_ntk = -1;
if (token.kind == kind) {
jj_gen++;
return token;
}
token = oldToken;
jj_kind = kind;
throw generateParseException();
}
static final public Token getNextToken() {
if (token.next != null) token = token.next;
else token = token.next = UnitsParserTokenManager.getNextToken();
jj_ntk = -1;
jj_gen++;
return token;
}
static final public Token getToken(int index) {
Token t = token;
for (int i = 0; i < index; i++) {
if (t.next != null) t = t.next;
else t = t.next = UnitsParserTokenManager.getNextToken();
}
return t;
}
static final private int jj_ntk() {
if ((jj_nt=token.next) == null)
return (jj_ntk = (token.next=UnitsParserTokenManager.getNextToken()).kind);
else
return (jj_ntk = jj_nt.kind);
}
static private java.util.Vector jj_expentries = new java.util.Vector();
static private int[] jj_expentry;
static private int jj_kind = -1;
static final public ParseException generateParseException() {
jj_expentries.removeAllElements();
boolean[] la1tokens = new boolean[17];
for (int i = 0; i < 17; i++) {
la1tokens[i] = false;
}
if (jj_kind >= 0) {
la1tokens[jj_kind] = true;
jj_kind = -1;
}
for (int i = 0; i < 10; i++) {
if (jj_la1[i] == jj_gen) {
for (int j = 0; j < 32; j++) {
if ((jj_la1_0[i] & (1< 11)
kind = 11;
jjCheckNAddStates(0, 4);
}
else if ((0x440000000000L & l) != 0L)
{
if (kind > 5)
kind = 5;
}
else if (curChar == 45)
jjCheckNAddStates(5, 10);
else if (curChar == 37)
{
if (kind > 10)
kind = 10;
}
else if (curChar == 47)
{
if (kind > 6)
kind = 6;
}
if (curChar == 46)
jjCheckNAddTwoStates(17, 18);
else if (curChar == 42)
jjstateSet[jjnewStateCnt++] = 9;
break;
case 30:
if ((0x3ff000000000000L & l) != 0L)
{
if (kind > 12)
kind = 12;
jjCheckNAddStates(11, 14);
}
else if (curChar == 45)
jjCheckNAddTwoStates(25, 26);
else if (curChar == 46)
jjCheckNAddTwoStates(17, 18);
if ((0x3ff000000000000L & l) != 0L)
{
if (kind > 12)
kind = 12;
jjCheckNAddStates(15, 17);
}
if ((0x3ff000000000000L & l) != 0L)
{
if (kind > 12)
kind = 12;
jjCheckNAddTwoStates(25, 19);
}
if ((0x3ff000000000000L & l) != 0L)
{
if (kind > 11)
kind = 11;
jjCheckNAdd(24);
}
break;
case 1:
if (curChar == 47)
kind = 6;
break;
case 9:
if (curChar == 42 && kind > 7)
kind = 7;
break;
case 10:
if (curChar == 42)
jjstateSet[jjnewStateCnt++] = 9;
break;
case 11:
if (curChar == 37)
kind = 10;
break;
case 14:
if ((0x3ff000000000000L & l) != 0L)
jjAddStates(18, 19);
break;
case 16:
if (curChar == 46)
jjCheckNAddTwoStates(17, 18);
break;
case 17:
if (curChar == 45)
jjCheckNAdd(18);
break;
case 18:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 12)
kind = 12;
jjCheckNAddTwoStates(18, 19);
break;
case 20:
if ((0x280000000000L & l) != 0L)
jjCheckNAddTwoStates(21, 22);
break;
case 21:
if (curChar == 45)
jjCheckNAdd(22);
break;
case 22:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 12)
kind = 12;
jjCheckNAdd(22);
break;
case 23:
if (curChar == 45)
jjCheckNAddStates(5, 10);
break;
case 24:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 11)
kind = 11;
jjCheckNAdd(24);
break;
case 25:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 12)
kind = 12;
jjCheckNAddTwoStates(25, 19);
break;
case 26:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 12)
kind = 12;
jjCheckNAddStates(15, 17);
break;
case 27:
if (curChar == 46)
jjCheckNAddTwoStates(28, 29);
break;
case 28:
if (curChar == 45)
jjCheckNAdd(29);
break;
case 29:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 12)
kind = 12;
jjCheckNAddTwoStates(29, 19);
break;
case 31:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 12)
kind = 12;
jjCheckNAddStates(11, 14);
break;
case 32:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 11)
kind = 11;
jjCheckNAddStates(0, 4);
break;
default : break;
}
} while(i != startsAt);
}
else if (curChar < 128)
{
long l = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
case 0:
if ((0x7fffffe07fffffeL & l) != 0L)
{
if (kind > 10)
kind = 10;
jjCheckNAddTwoStates(13, 14);
}
else if (curChar == 94)
{
if (kind > 7)
kind = 7;
}
if (curChar == 80)
jjstateSet[jjnewStateCnt++] = 6;
else if (curChar == 112)
jjstateSet[jjnewStateCnt++] = 3;
break;
case 2:
if (curChar == 114 && kind > 6)
kind = 6;
break;
case 3:
if (curChar == 101)
jjstateSet[jjnewStateCnt++] = 2;
break;
case 4:
if (curChar == 112)
jjstateSet[jjnewStateCnt++] = 3;
break;
case 5:
if (curChar == 82 && kind > 6)
kind = 6;
break;
case 6:
if (curChar == 69)
jjstateSet[jjnewStateCnt++] = 5;
break;
case 7:
if (curChar == 80)
jjstateSet[jjnewStateCnt++] = 6;
break;
case 8:
if (curChar == 94)
kind = 7;
break;
case 12:
if ((0x7fffffe07fffffeL & l) == 0L)
break;
if (kind > 10)
kind = 10;
jjCheckNAddTwoStates(13, 14);
break;
case 13:
if ((0x7fffffe87fffffeL & l) == 0L)
break;
if (kind > 10)
kind = 10;
jjCheckNAddTwoStates(13, 14);
break;
case 15:
if ((0x7fffffe87fffffeL & l) == 0L)
break;
if (kind > 10)
kind = 10;
jjCheckNAddTwoStates(14, 15);
break;
case 19:
if ((0x2000000020L & l) != 0L)
jjAddStates(20, 22);
break;
default : break;
}
} while(i != startsAt);
}
else
{
//int i2 = (curChar & 0xff) >> 6;
//long l2 = 1L << (curChar & 077);
do
{
switch(jjstateSet[--i])
{
default : break;
}
} while(i != startsAt);
}
if (kind != 0x7fffffff)
{
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 33 - (jjnewStateCnt = startsAt)))
return curPos;
try { curChar = ASCII_CharStream.readChar(); }
catch(java.io.IOException e) { return curPos; }
}
}
static final int[] jjnextStates = {
24, 25, 26, 27, 19, 24, 25, 26, 16, 30, 31, 25, 26, 27, 19, 26,
27, 19, 14, 15, 20, 21, 22,
};
public static final String[] jjstrLiteralImages = {
"", null, null, "\53", "\55", null, null, null, "\50", "\51", null, null, null,
null, null, null, null, };
public static final String[] lexStateNames = {
"DEFAULT",
};
static final long[] jjtoToken = {
0x1ff9L,
};
static final long[] jjtoSkip = {
0x6L,
};
static private ASCII_CharStream input_stream;
static private final int[] jjrounds = new int[33];
static private final int[] jjstateSet = new int[66];
static protected char curChar;
public UnitsParserTokenManager(ASCII_CharStream stream)
{
if (input_stream != null)
throw new TokenMgrError("ERROR: Second call to constructor of static lexer. You must use ReInit() to initialize the static variables.", TokenMgrError.STATIC_LEXER_ERROR);
input_stream = stream;
}
public UnitsParserTokenManager(ASCII_CharStream stream, int lexState)
{
this(stream);
SwitchTo(lexState);
}
static public void ReInit(ASCII_CharStream stream)
{
jjmatchedPos = jjnewStateCnt = 0;
curLexState = defaultLexState;
input_stream = stream;
ReInitRounds();
}
static private final void ReInitRounds()
{
int i;
jjround = 0x80000001;
for (i = 33; i-- > 0;)
jjrounds[i] = 0x80000000;
}
static public void ReInit(ASCII_CharStream stream, int lexState)
{
ReInit(stream);
SwitchTo(lexState);
}
static public void SwitchTo(int lexState)
{
if (lexState >= 1 || lexState < 0)
throw new TokenMgrError("Error: Ignoring invalid lexical state : " + lexState + ". State unchanged.", TokenMgrError.INVALID_LEXICAL_STATE);
else
curLexState = lexState;
}
static private final Token jjFillToken()
{
Token t = Token.newToken(jjmatchedKind);
t.kind = jjmatchedKind;
String im = jjstrLiteralImages[jjmatchedKind];
t.image = (im == null) ? ASCII_CharStream.GetImage() : im;
t.beginLine = ASCII_CharStream.getBeginLine();
t.beginColumn = ASCII_CharStream.getBeginColumn();
t.endLine = ASCII_CharStream.getEndLine();
t.endColumn = ASCII_CharStream.getEndColumn();
return t;
}
static int curLexState = 0;
static int defaultLexState = 0;
static int jjnewStateCnt;
static int jjround;
static int jjmatchedPos;
static int jjmatchedKind;
public static final Token getNextToken()
{
//int kind;
//Token specialToken = null;
Token matchedToken;
int curPos = 0;
EOFLoop :
for (;;)
{
try
{
curChar = ASCII_CharStream.BeginToken();
}
catch(java.io.IOException e)
{
jjmatchedKind = 0;
matchedToken = jjFillToken();
return matchedToken;
}
try { ASCII_CharStream.backup(0);
while (curChar <= 32 && (0x100000200L & (1L << curChar)) != 0L)
curChar = ASCII_CharStream.BeginToken();
}
catch (java.io.IOException e1) { continue EOFLoop; }
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_0();
if (jjmatchedKind != 0x7fffffff)
{
if (jjmatchedPos + 1 < curPos)
ASCII_CharStream.backup(curPos - jjmatchedPos - 1);
if ((jjtoToken[jjmatchedKind >> 6] & (1L << (jjmatchedKind & 077))) != 0L)
{
matchedToken = jjFillToken();
return matchedToken;
}
else
{
continue EOFLoop;
}
}
int error_line = ASCII_CharStream.getEndLine();
int error_column = ASCII_CharStream.getEndColumn();
String error_after = null;
boolean EOFSeen = false;
try { ASCII_CharStream.readChar(); ASCII_CharStream.backup(1); }
catch (java.io.IOException e1) {
EOFSeen = true;
error_after = curPos <= 1 ? "" : ASCII_CharStream.GetImage();
if (curChar == '\n' || curChar == '\r') {
error_line++;
error_column = 0;
}
else
error_column++;
}
if (!EOFSeen) {
ASCII_CharStream.backup(1);
error_after = curPos <= 1 ? "" : ASCII_CharStream.GetImage();
}
throw new TokenMgrError(EOFSeen, curLexState, error_line, error_column, error_after, curChar, TokenMgrError.LEXICAL_ERROR);
}
}
}
////////////////////////////////////////////////////////////////////////////
// TokenMgrError
static private class TokenMgrError extends Error
{
private static final long serialVersionUID = 1L;
/*
* Ordinals for various reasons why an Error of this type can be thrown.
*/
/**
* Lexical error occured.
*/
static final int LEXICAL_ERROR = 0;
/**
* An attempt wass made to create a second instance of a static token
* manager.
*/
static final int STATIC_LEXER_ERROR = 1;
/**
* Tried to change to an invalid lexical state.
*/
static final int INVALID_LEXICAL_STATE = 2;
/**
* Detected (and bailed out of) an infinite loop in the token manager.
*/
static final int LOOP_DETECTED = 3;
/**
* Indicates the reason why the exception is thrown. It will have
* one of the above 4 values.
*/
int errorCode;
/**
* Replaces unprintable characters by their espaced (or unicode escaped)
* equivalents in the given string
*/
protected static final String addEscapes(String str) {
StringBuffer retval = new StringBuffer();
char ch;
for (int i = 0; i < str.length(); i++) {
switch (str.charAt(i))
{
case 0 :
continue;
case '\b':
retval.append("\\b");
continue;
case '\t':
retval.append("\\t");
continue;
case '\n':
retval.append("\\n");
continue;
case '\f':
retval.append("\\f");
continue;
case '\r':
retval.append("\\r");
continue;
case '\"':
retval.append("\\\"");
continue;
case '\'':
retval.append("\\\'");
continue;
case '\\':
retval.append("\\\\");
continue;
default:
if ((ch = str.charAt(i)) < 0x20 || ch > 0x7e) {
String s = "0000" + Integer.toString(ch, 16);
retval.append("\\u" + s.substring(s.length() - 4, s.length()));
} else {
retval.append(ch);
}
continue;
}
}
return retval.toString();
}
/**
* Returns a detailed message for the Error when it is thrown by the
* token manager to indicate a lexical error.
* Parameters :
* EOFSeen : indicates if EOF caused the lexicl error
* curLexState : lexical state in which this error occured
* errorLine : line number when the error occured
* errorColumn : column number when the error occured
* errorAfter : prefix that was seen before this error occured
* curchar : the offending character
* Note: You can customize the lexical error message by modifying this
* method.
*/
private static final String LexicalError(boolean EOFSeen, int lexState,
int errorLine, int errorColumn, String errorAfter,
char curChar) {
return("Lexical error at line " + errorLine + ", column " +
errorColumn + ". Encountered: " +
(EOFSeen ? " " : ("\"" + addEscapes(String.valueOf(curChar)) +
"\"") + " (" + (int)curChar + "), ") +
"after : \"" + addEscapes(errorAfter) + "\"");
}
/**
* You can also modify the body of this method to customize your error
* messages.
* For example, cases like LOOP_DETECTED and INVALID_LEXICAL_STATE are not
* of end-users concern, so you can return something like :
*
* "Internal Error : Please file a bug report .... "
*
* from this method for such cases in the release version of your parser.
*/
public String getMessage() {
return super.getMessage();
}
/*
* Constructors of various flavors follow.
*/
public TokenMgrError() {
}
public TokenMgrError(String message, int reason) {
super(message);
errorCode = reason;
}
public TokenMgrError(boolean EOFSeen, int lexState, int errorLine,
int errorColumn, String errorAfter, char curChar, int reason) {
this(LexicalError(EOFSeen, lexState, errorLine, errorColumn, errorAfter,
curChar), reason);
}
}
////////////////////////////////////////////////////////////////////////////
// UnitsParserConstants
//private static final int EOF = 0;
private static final int PLUS = 3;
private static final int MINUS = 4;
private static final int MUL = 5;
private static final int DIV = 6;
private static final int POW = 7;
private static final int LP = 8;
private static final int RP = 9;
private static final int NAME = 10;
private static final int INTEGER = 11;
private static final int DOUBLE = 12;
//private static final int ALPHA = 13;
//private static final int LETTER = 14;
//private static final int FLOAT = 15;
//private static final int DIGIT = 16;
//private static final int DEFAULT = 0;
/*
private static final String[] tokenImage = {
"",
"\" \"",
"\"\\t\"",
"\"+\"",
"\"-\"",
"",
"",
"",
"\"(\"",
"\")\"",
"",
"",
"",
"",
"",
"",
"",
};
*/
////////////////////////////////////////////////////////////////////////////
// ASCII_CharStream
/**
* An implementation of interface CharStream, where the stream is assumed to
* contain only ASCII characters (without unicode processing).
*/
static private final class ASCII_CharStream
{
public static final boolean staticFlag = true;
static int bufsize;
static int available;
static int tokenBegin;
static public int bufpos = -1;
static private int bufline[];
static private int bufcolumn[];
static private int column = 0;
static private int line = 1;
static private boolean prevCharIsCR = false;
static private boolean prevCharIsLF = false;
static private java.io.Reader inputStream;
static private char[] buffer;
static private int maxNextCharInd = 0;
static private int inBuf = 0;
static private final void ExpandBuff(boolean wrapAround)
{
char[] newbuffer = new char[bufsize + 2048];
int newbufline[] = new int[bufsize + 2048];
int newbufcolumn[] = new int[bufsize + 2048];
try
{
if (wrapAround)
{
System.arraycopy(buffer, tokenBegin, newbuffer, 0, bufsize - tokenBegin);
System.arraycopy(buffer, 0, newbuffer,
bufsize - tokenBegin, bufpos);
buffer = newbuffer;
System.arraycopy(bufline, tokenBegin, newbufline, 0, bufsize - tokenBegin);
System.arraycopy(bufline, 0, newbufline, bufsize - tokenBegin, bufpos);
bufline = newbufline;
System.arraycopy(bufcolumn, tokenBegin, newbufcolumn, 0, bufsize - tokenBegin);
System.arraycopy(bufcolumn, 0, newbufcolumn, bufsize - tokenBegin, bufpos);
bufcolumn = newbufcolumn;
maxNextCharInd = (bufpos += (bufsize - tokenBegin));
}
else
{
System.arraycopy(buffer, tokenBegin, newbuffer, 0, bufsize - tokenBegin);
buffer = newbuffer;
System.arraycopy(bufline, tokenBegin, newbufline, 0, bufsize - tokenBegin);
bufline = newbufline;
System.arraycopy(bufcolumn, tokenBegin, newbufcolumn, 0, bufsize - tokenBegin);
bufcolumn = newbufcolumn;
maxNextCharInd = (bufpos -= tokenBegin);
}
}
catch (Throwable t)
{
throw new Error(t.getMessage());
}
bufsize += 2048;
available = bufsize;
tokenBegin = 0;
}
static private final void FillBuff() throws java.io.IOException
{
if (maxNextCharInd == available)
{
if (available == bufsize)
{
if (tokenBegin > 2048)
{
bufpos = maxNextCharInd = 0;
available = tokenBegin;
}
else if (tokenBegin < 0)
bufpos = maxNextCharInd = 0;
else
ExpandBuff(false);
}
else if (available > tokenBegin)
available = bufsize;
else if ((tokenBegin - available) < 2048)
ExpandBuff(true);
else
available = tokenBegin;
}
int i;
try {
if ((i = inputStream.read(buffer, maxNextCharInd,
available - maxNextCharInd)) == -1)
{
inputStream.close();
throw new java.io.IOException();
}
else
maxNextCharInd += i;
return;
}
catch(java.io.IOException e) {
--bufpos;
backup(0);
if (tokenBegin == -1)
tokenBegin = bufpos;
throw e;
}
}
static public final char BeginToken() throws java.io.IOException
{
tokenBegin = -1;
char c = readChar();
tokenBegin = bufpos;
return c;
}
static private final void UpdateLineColumn(char c)
{
column++;
if (prevCharIsLF)
{
prevCharIsLF = false;
line += (column = 1);
}
else if (prevCharIsCR)
{
prevCharIsCR = false;
if (c == '\n')
{
prevCharIsLF = true;
}
else
line += (column = 1);
}
switch (c)
{
case '\r' :
prevCharIsCR = true;
break;
case '\n' :
prevCharIsLF = true;
break;
case '\t' :
column--;
column += (8 - (column & 07));
break;
default :
break;
}
bufline[bufpos] = line;
bufcolumn[bufpos] = column;
}
static public final char readChar() throws java.io.IOException
{
if (inBuf > 0)
{
--inBuf;
return (char)((char)0xff & buffer[(bufpos == bufsize - 1) ? (bufpos = 0) : ++bufpos]);
}
if (++bufpos >= maxNextCharInd)
FillBuff();
char c = (char)((char)0xff & buffer[bufpos]);
UpdateLineColumn(c);
return (c);
}
/**
* @Deprecated
* @see #getEndColumn
*/
static public final int getColumn() {
return bufcolumn[bufpos];
}
/**
* @Deprecated
* @see #getEndLine
*/
static public final int getLine() {
return bufline[bufpos];
}
static public final int getEndColumn() {
return bufcolumn[bufpos];
}
static public final int getEndLine() {
return bufline[bufpos];
}
static public final int getBeginColumn() {
return bufcolumn[tokenBegin];
}
static public final int getBeginLine() {
return bufline[tokenBegin];
}
static public final void backup(int amount) {
inBuf += amount;
if ((bufpos -= amount) < 0)
bufpos += bufsize;
}
public ASCII_CharStream(java.io.Reader dstream, int startline,
int startcolumn, int buffersize)
{
if (inputStream != null)
throw new Error("\n ERROR: Second call to the constructor of a static ASCII_CharStream. You must\n" +
" either use ReInit() or set the JavaCC option STATIC to false\n" +
" during the generation of this class.");
inputStream = dstream;
line = startline;
column = startcolumn - 1;
available = bufsize = buffersize;
buffer = new char[buffersize];
bufline = new int[buffersize];
bufcolumn = new int[buffersize];
}
public ASCII_CharStream(java.io.Reader dstream, int startline,
int startcolumn)
{
this(dstream, startline, startcolumn, 4096);
}
static public void ReInit(java.io.Reader dstream, int startline,
int startcolumn, int buffersize)
{
inputStream = dstream;
line = startline;
column = startcolumn - 1;
if (buffer == null || buffersize != buffer.length)
{
available = bufsize = buffersize;
buffer = new char[buffersize];
bufline = new int[buffersize];
bufcolumn = new int[buffersize];
}
prevCharIsLF = prevCharIsCR = false;
tokenBegin = inBuf = maxNextCharInd = 0;
bufpos = -1;
}
static public void ReInit(java.io.Reader dstream, int startline,
int startcolumn)
{
ReInit(dstream, startline, startcolumn, 4096);
}
public ASCII_CharStream(java.io.InputStream dstream, int startline,
int startcolumn, int buffersize)
{
this(new java.io.InputStreamReader(dstream), startline, startcolumn, 4096);
}
public ASCII_CharStream(java.io.InputStream dstream, int startline,
int startcolumn)
{
this(dstream, startline, startcolumn, 4096);
}
static public void ReInit(java.io.InputStream dstream, int startline,
int startcolumn, int buffersize)
{
ReInit(new java.io.InputStreamReader(dstream), startline, startcolumn, 4096);
}
static public void ReInit(java.io.InputStream dstream, int startline,
int startcolumn)
{
ReInit(dstream, startline, startcolumn, 4096);
}
static public final String GetImage()
{
if (bufpos >= tokenBegin)
return new String(buffer, tokenBegin, bufpos - tokenBegin + 1);
else
return new String(buffer, tokenBegin, bufsize - tokenBegin) +
new String(buffer, 0, bufpos + 1);
}
static public final char[] GetSuffix(int len)
{
char[] ret = new char[len];
if ((bufpos + 1) >= len)
System.arraycopy(buffer, bufpos - len + 1, ret, 0, len);
else
{
System.arraycopy(buffer, bufsize - (len - bufpos - 1), ret, 0,
len - bufpos - 1);
System.arraycopy(buffer, 0, ret, len - bufpos - 1, bufpos + 1);
}
return ret;
}
static public void Done()
{
buffer = null;
bufline = null;
bufcolumn = null;
}
/**
* Method to adjust line and column numbers for the start of a token.
*/
static public void adjustBeginLineColumn(int newLine, int newCol)
{
int start = tokenBegin;
int len;
if (bufpos >= tokenBegin)
{
len = bufpos - tokenBegin + inBuf + 1;
}
else
{
len = bufsize - tokenBegin + bufpos + 1 + inBuf;
}
int i = 0, j = 0, k = 0;
int nextColDiff = 0, columnDiff = 0;
while (i < len &&
bufline[j = start % bufsize] == bufline[k = ++start % bufsize])
{
bufline[j] = newLine;
nextColDiff = columnDiff + bufcolumn[k] - bufcolumn[j];
bufcolumn[j] = newCol + columnDiff;
columnDiff = nextColDiff;
i++;
}
if (i < len)
{
bufline[j] = newLine++;
bufcolumn[j] = newCol + columnDiff;
while (i++ < len)
{
if (bufline[j = start % bufsize] != bufline[++start % bufsize])
bufline[j] = newLine++;
else
bufline[j] = newLine;
}
}
line = bufline[j];
column = bufcolumn[j];
}
}
////////////////////////////////////////////////////////////////////////////
// ParseException
/**
* This exception is thrown when parse errors are encountered.
* You can explicitly create objects of this exception type by
* calling the method generateParseException in the generated
* parser.
*
* You can modify this class to customize your error reporting
* mechanisms so long as you retain the public fields.
*/
static private class ParseException extends Exception {
private static final long serialVersionUID = 1L;
/**
* This constructor is used by the method "generateParseException"
* in the generated parser. Calling this constructor generates
* a new object of this type with the fields "currentToken",
* "expectedTokenSequences", and "tokenImage" set. The boolean
* flag "specialConstructor" is also set to true to indicate that
* this constructor was used to create this object.
* This constructor calls its super class with the empty string
* to force the "toString" method of parent class "Throwable" to
* print the error message in the form:
* ParseException:
*/
public ParseException(Token currentTokenVal,
int[][] expectedTokenSequencesVal,
String[] tokenImageVal
)
{
super("");
specialConstructor = true;
currentToken = currentTokenVal;
expectedTokenSequences = expectedTokenSequencesVal;
tokenImage = tokenImageVal;
}
/**
* The following constructors are for use by you for whatever
* purpose you can think of. Constructing the exception in this
* manner makes the exception behave in the normal way - i.e., as
* documented in the class "Throwable". The fields "errorToken",
* "expectedTokenSequences", and "tokenImage" do not contain
* relevant information. The JavaCC generated code does not use
* these constructors.
*/
public ParseException() {
super();
specialConstructor = false;
}
public ParseException(String message) {
super(message);
specialConstructor = false;
}
/**
* This variable determines which constructor was used to create
* this object and thereby affects the semantics of the
* "getMessage" method (see below).
*/
protected boolean specialConstructor;
/**
* This is the last token that has been consumed successfully. If
* this object has been created due to a parse error, the token
* followng this token will (therefore) be the first error token.
*/
public Token currentToken;
/**
* Each entry in this array is an array of integers. Each array
* of integers represents a sequence of tokens (by their ordinal
* values) that is expected at this point of the parse.
*/
public int[][] expectedTokenSequences;
/**
* This is a reference to the "tokenImage" array of the generated
* parser within which the parse error occurred. This array is
* defined in the generated ...Constants interface.
*/
public String[] tokenImage;
/**
* This method has the standard behavior when this object has been
* created using the standard constructors. Otherwise, it uses
* "currentToken" and "expectedTokenSequences" to generate a parse
* error message and returns it. If this object has been created
* due to a parse error, and you do not catch it (it gets thrown
* from the parser), then this method is called during the printing
* of the final stack trace, and hence the correct error message
* gets displayed.
*/
public String getMessage() {
if (!specialConstructor) {
return super.getMessage();
}
String expected = "";
int maxSize = 0;
for (int i = 0; i < expectedTokenSequences.length; i++) {
if (maxSize < expectedTokenSequences[i].length) {
maxSize = expectedTokenSequences[i].length;
}
for (int j = 0; j < expectedTokenSequences[i].length; j++) {
expected += tokenImage[expectedTokenSequences[i][j]] + " ";
}
if (expectedTokenSequences[i][expectedTokenSequences[i].length - 1] != 0) {
expected += "...";
}
expected += eol + " ";
}
String retval = "Encountered \"";
Token tok = currentToken.next;
for (int i = 0; i < maxSize; i++) {
if (i != 0) retval += " ";
if (tok.kind == 0) {
retval += tokenImage[0];
break;
}
retval += add_escapes(tok.image);
tok = tok.next;
}
retval += "\" at line " + currentToken.next.beginLine + ", column " + currentToken.next.beginColumn + "." + eol;
if (expectedTokenSequences.length == 1) {
retval += "Was expecting:" + eol + " ";
} else {
retval += "Was expecting one of:" + eol + " ";
}
retval += expected;
return retval;
}
/**
* The end of line string for this machine.
*/
protected String eol = System.getProperty("line.separator", "\n");
/**
* Used to convert raw characters to their escaped version
* when these raw version cannot be used as part of an ASCII
* string literal.
*/
protected String add_escapes(String str) {
StringBuffer retval = new StringBuffer();
char ch;
for (int i = 0; i < str.length(); i++) {
switch (str.charAt(i))
{
case 0 :
continue;
case '\b':
retval.append("\\b");
continue;
case '\t':
retval.append("\\t");
continue;
case '\n':
retval.append("\\n");
continue;
case '\f':
retval.append("\\f");
continue;
case '\r':
retval.append("\\r");
continue;
case '\"':
retval.append("\\\"");
continue;
case '\'':
retval.append("\\\'");
continue;
case '\\':
retval.append("\\\\");
continue;
default:
if ((ch = str.charAt(i)) < 0x20 || ch > 0x7e) {
String s = "0000" + Integer.toString(ch, 16);
retval.append("\\u" + s.substring(s.length() - 4, s.length()));
} else {
retval.append(ch);
}
continue;
}
}
return retval.toString();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy