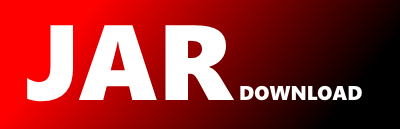
edu.nps.moves.dis.RemoveEntityPdu Maven / Gradle / Ivy
package edu.nps.moves.dis;
import java.io.*;
/**
* Section 5.3.6.2. Remove an entity. COMPLETE
*
* Copyright (c) 2008-2016, MOVES Institute, Naval Postgraduate School. All
* rights reserved. This work is licensed under the BSD open source license,
* available at https://www.movesinstitute.org/licenses/bsd.html
*
* @author DMcG
*/
public class RemoveEntityPdu extends SimulationManagementFamilyPdu implements Serializable {
/**
* Identifier for the request
*/
protected long requestID;
/**
* Constructor
*/
public RemoveEntityPdu() {
setPduType((short) 12);
}
public int getMarshalledSize() {
int marshalSize = 0;
marshalSize = super.getMarshalledSize();
marshalSize = marshalSize + 4; // requestID
return marshalSize;
}
public void setRequestID(long pRequestID) {
requestID = pRequestID;
}
public long getRequestID() {
return requestID;
}
/**
* Packs a Pdu into the ByteBuffer.
*
* @throws java.nio.BufferOverflowException if buff is too small
* @throws java.nio.ReadOnlyBufferException if buff is read only
* @see java.nio.ByteBuffer
* @param buff The ByteBuffer at the position to begin writing
* @since ??
*/
public void marshal(java.nio.ByteBuffer buff) {
super.marshal(buff);
buff.putInt((int) requestID);
} // end of marshal method
/**
* Unpacks a Pdu from the underlying data.
*
* @throws java.nio.BufferUnderflowException if buff is too small
* @see java.nio.ByteBuffer
* @param buff The ByteBuffer at the position to begin reading
* @since ??
*/
public void unmarshal(java.nio.ByteBuffer buff) {
super.unmarshal(buff);
requestID = buff.getInt();
} // end of unmarshal method
/*
* The equals method doesn't always work--mostly it works only on classes that consist only of primitives. Be careful.
*/
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
return equalsImpl(obj);
}
@Override
public boolean equalsImpl(Object obj) {
boolean ivarsEqual = true;
if (!(obj instanceof RemoveEntityPdu)) {
return false;
}
final RemoveEntityPdu rhs = (RemoveEntityPdu) obj;
if (!(requestID == rhs.requestID)) {
ivarsEqual = false;
}
return ivarsEqual && super.equalsImpl(rhs);
}
} // end of class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy