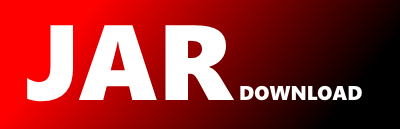
edu.nps.moves.dis.StartResumeReliablePdu Maven / Gradle / Ivy
package edu.nps.moves.dis;
import java.io.*;
/**
* Section 5.3.12.3: Start resume simulation, relaible. COMPLETE
*
* Copyright (c) 2008-2016, MOVES Institute, Naval Postgraduate School. All
* rights reserved. This work is licensed under the BSD open source license,
* available at https://www.movesinstitute.org/licenses/bsd.html
*
* @author DMcG
*/
public class StartResumeReliablePdu extends SimulationManagementWithReliabilityFamilyPdu implements Serializable {
/**
* time in real world for this operation to happen
*/
protected ClockTime realWorldTime = new ClockTime();
/**
* time in simulation for the simulation to resume
*/
protected ClockTime simulationTime = new ClockTime();
/**
* level of reliability service used for this transaction
*/
protected short requiredReliabilityService;
/**
* padding
*/
protected int pad1;
/**
* padding
*/
protected short pad2;
/**
* Request ID
*/
protected long requestID;
/**
* Constructor
*/
public StartResumeReliablePdu() {
setPduType((short) 53);
}
public int getMarshalledSize() {
int marshalSize = 0;
marshalSize = super.getMarshalledSize();
marshalSize = marshalSize + realWorldTime.getMarshalledSize(); // realWorldTime
marshalSize = marshalSize + simulationTime.getMarshalledSize(); // simulationTime
marshalSize = marshalSize + 1; // requiredReliabilityService
marshalSize = marshalSize + 2; // pad1
marshalSize = marshalSize + 1; // pad2
marshalSize = marshalSize + 4; // requestID
return marshalSize;
}
public void setRealWorldTime(ClockTime pRealWorldTime) {
realWorldTime = pRealWorldTime;
}
public ClockTime getRealWorldTime() {
return realWorldTime;
}
public void setSimulationTime(ClockTime pSimulationTime) {
simulationTime = pSimulationTime;
}
public ClockTime getSimulationTime() {
return simulationTime;
}
public void setRequiredReliabilityService(short pRequiredReliabilityService) {
requiredReliabilityService = pRequiredReliabilityService;
}
public short getRequiredReliabilityService() {
return requiredReliabilityService;
}
public void setPad1(int pPad1) {
pad1 = pPad1;
}
public int getPad1() {
return pad1;
}
public void setPad2(short pPad2) {
pad2 = pPad2;
}
public short getPad2() {
return pad2;
}
public void setRequestID(long pRequestID) {
requestID = pRequestID;
}
public long getRequestID() {
return requestID;
}
/**
* Packs a Pdu into the ByteBuffer.
*
* @throws java.nio.BufferOverflowException if buff is too small
* @throws java.nio.ReadOnlyBufferException if buff is read only
* @see java.nio.ByteBuffer
* @param buff The ByteBuffer at the position to begin writing
* @since ??
*/
public void marshal(java.nio.ByteBuffer buff) {
super.marshal(buff);
realWorldTime.marshal(buff);
simulationTime.marshal(buff);
buff.put((byte) requiredReliabilityService);
buff.putShort((short) pad1);
buff.put((byte) pad2);
buff.putInt((int) requestID);
} // end of marshal method
/**
* Unpacks a Pdu from the underlying data.
*
* @throws java.nio.BufferUnderflowException if buff is too small
* @see java.nio.ByteBuffer
* @param buff The ByteBuffer at the position to begin reading
* @since ??
*/
public void unmarshal(java.nio.ByteBuffer buff) {
super.unmarshal(buff);
realWorldTime.unmarshal(buff);
simulationTime.unmarshal(buff);
requiredReliabilityService = (short) (buff.get() & 0xFF);
pad1 = (int) (buff.getShort() & 0xFFFF);
pad2 = (short) (buff.get() & 0xFF);
requestID = buff.getInt();
} // end of unmarshal method
/*
* The equals method doesn't always work--mostly it works only on classes that consist only of primitives. Be careful.
*/
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
return equalsImpl(obj);
}
@Override
public boolean equalsImpl(Object obj) {
boolean ivarsEqual = true;
if (!(obj instanceof StartResumeReliablePdu)) {
return false;
}
final StartResumeReliablePdu rhs = (StartResumeReliablePdu) obj;
if (!(realWorldTime.equals(rhs.realWorldTime))) {
ivarsEqual = false;
}
if (!(simulationTime.equals(rhs.simulationTime))) {
ivarsEqual = false;
}
if (!(requiredReliabilityService == rhs.requiredReliabilityService)) {
ivarsEqual = false;
}
if (!(pad1 == rhs.pad1)) {
ivarsEqual = false;
}
if (!(pad2 == rhs.pad2)) {
ivarsEqual = false;
}
if (!(requestID == rhs.requestID)) {
ivarsEqual = false;
}
return ivarsEqual && super.equalsImpl(rhs);
}
} // end of class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy