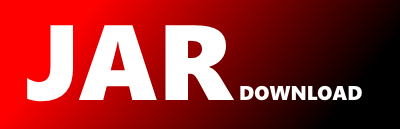
edu.nps.moves.dis7.EntityStateUpdatePdu Maven / Gradle / Ivy
package edu.nps.moves.dis7;
import java.util.*;
import java.io.*;
/**
* Nonstatic information about a particular entity may be communicated by
* issuing an Entity State Update PDU. Section 7.2.5. COMPLETE
*
* Copyright (c) 2008-2016, MOVES Institute, Naval Postgraduate School. All
* rights reserved. This work is licensed under the BSD open source license,
* available at https://www.movesinstitute.org/licenses/bsd.html
*
* @author DMcG
*/
public class EntityStateUpdatePdu extends EntityInformationFamilyPdu implements Serializable {
/**
* This field shall identify the entity issuing the PDU, and shall be
* represented by an Entity Identifier record (see 6.2.28).
*/
protected EntityID entityID = new EntityID();
/**
* Padding
*/
protected byte padding1;
/**
* This field shall specify the number of variable parameters present. This
* field shall be represented by an 8-bit unsigned integer (see Annex I).
*/
protected short numberOfVariableParameters;
/**
* This field shall specify an entity’s linear velocity. The coordinate
* system for an entity’s linear velocity depends on the dead reckoning
* algorithm used. This field shall be represented by a Linear Velocity
* Vector record [see 6.2.95 item c)]).
*/
protected Vector3Float entityLinearVelocity = new Vector3Float();
/**
* This field shall specify an entity’s physical location in the simulated
* world and shall be represented by a World Coordinates record (see
* 6.2.97).
*/
protected Vector3Double entityLocation = new Vector3Double();
/**
* This field shall specify an entity’s orientation and shall be
* represented by an Euler Angles record (see 6.2.33).
*/
protected EulerAngles entityOrientation = new EulerAngles();
/**
* This field shall specify the dynamic changes to the entity’s appearance
* attributes. This field shall be represented by an Entity Appearance
* record (see 6.2.26).
*/
protected long entityAppearance;
/**
* This field shall specify the parameter values for each Variable Parameter
* record that is included (see 6.2.93 and Annex I).
*/
protected List< VariableParameter> variableParameters = new ArrayList< VariableParameter>();
/**
* Constructor
*/
public EntityStateUpdatePdu() {
setPduType((short) 67);
setProtocolFamily((short) 1);
}
public int getMarshalledSize() {
int marshalSize = 0;
marshalSize = super.getMarshalledSize();
marshalSize = marshalSize + entityID.getMarshalledSize(); // entityID
marshalSize = marshalSize + 1; // padding1
marshalSize = marshalSize + 1; // numberOfVariableParameters
marshalSize = marshalSize + entityLinearVelocity.getMarshalledSize(); // entityLinearVelocity
marshalSize = marshalSize + entityLocation.getMarshalledSize(); // entityLocation
marshalSize = marshalSize + entityOrientation.getMarshalledSize(); // entityOrientation
marshalSize = marshalSize + 4; // entityAppearance
for (int idx = 0; idx < variableParameters.size(); idx++) {
VariableParameter listElement = variableParameters.get(idx);
switch (listElement.recordType) {
case 0:
marshalSize = marshalSize + ((ArticulatedParts) listElement).getMarshalledSize();
break;
case 1:
marshalSize = marshalSize + ((AttachedParts) listElement).getMarshalledSize();
break;
case 2:
marshalSize = marshalSize + ((SeparationVP) listElement).getMarshalledSize();
break;
case 3:
marshalSize = marshalSize + ((EntityTypeVP) listElement).getMarshalledSize();
break;
case 4:
marshalSize = marshalSize + ((EntityAssociation) listElement).getMarshalledSize();
break;
}
}
return marshalSize;
}
public void setEntityID(EntityID pEntityID) {
entityID = pEntityID;
}
public EntityID getEntityID() {
return entityID;
}
public void setPadding1(byte pPadding1) {
padding1 = pPadding1;
}
public byte getPadding1() {
return padding1;
}
public short getNumberOfVariableParameters() {
return (short) variableParameters.size();
}
/**
* Note that setting this value will not change the marshalled value. The
* list whose length this describes is used for that purpose. The
* getnumberOfVariableParameters method will also be based on the actual
* list length rather than this value. The method is simply here for java
* bean completeness.
*/
public void setNumberOfVariableParameters(short pNumberOfVariableParameters) {
numberOfVariableParameters = pNumberOfVariableParameters;
}
public void setEntityLinearVelocity(Vector3Float pEntityLinearVelocity) {
entityLinearVelocity = pEntityLinearVelocity;
}
public Vector3Float getEntityLinearVelocity() {
return entityLinearVelocity;
}
public void setEntityLocation(Vector3Double pEntityLocation) {
entityLocation = pEntityLocation;
}
public Vector3Double getEntityLocation() {
return entityLocation;
}
public void setEntityOrientation(EulerAngles pEntityOrientation) {
entityOrientation = pEntityOrientation;
}
public EulerAngles getEntityOrientation() {
return entityOrientation;
}
public void setEntityAppearance(long pEntityAppearance) {
entityAppearance = pEntityAppearance;
}
public long getEntityAppearance() {
return entityAppearance;
}
public void setVariableParameters(List pVariableParameters) {
variableParameters = pVariableParameters;
}
public List getVariableParameters() {
return variableParameters;
}
public void marshal(DataOutputStream dos) {
super.marshal(dos);
try {
entityID.marshal(dos);
dos.writeByte((byte) padding1);
dos.writeByte((byte) variableParameters.size());
entityLinearVelocity.marshal(dos);
entityLocation.marshal(dos);
entityOrientation.marshal(dos);
dos.writeInt((int) entityAppearance);
for (int idx = 0; idx < variableParameters.size(); idx++) {
VariableParameter aVariableParameter = variableParameters.get(idx);
switch (aVariableParameter.recordType) {
case 0:
((ArticulatedParts) aVariableParameter).marshal(dos);
break;
case 1:
((AttachedParts) aVariableParameter).marshal(dos);
break;
case 2:
((SeparationVP) aVariableParameter).marshal(dos);
break;
case 3:
((EntityTypeVP) aVariableParameter).marshal(dos);
break;
case 4:
((EntityAssociation) aVariableParameter).marshal(dos);
break;
}
} // end of list marshalling
} // end try
catch (Exception e) {
System.out.println(e);
}
} // end of marshal method
public void unmarshal(DataInputStream dis) {
super.unmarshal(dis);
try {
entityID.unmarshal(dis);
padding1 = dis.readByte();
numberOfVariableParameters = (short) dis.readUnsignedByte();
entityLinearVelocity.unmarshal(dis);
entityLocation.unmarshal(dis);
entityOrientation.unmarshal(dis);
entityAppearance = dis.readInt();
for (int idx = 0; idx < numberOfVariableParameters; idx++) {
VariableParameter anX = new VariableParameter();
anX.unmarshal(dis);
switch (anX.getRecordType()) {
case 0:
anX = new ArticulatedParts();
((ArticulatedParts) anX).unmarshal(dis);
break;
case 1:
anX = new AttachedParts();
((AttachedParts) anX).unmarshal(dis);
break;
case 2:
anX = new SeparationVP();
((SeparationVP) anX).unmarshal(dis);
break;
case 3:
anX = new EntityTypeVP();
((EntityTypeVP) anX).unmarshal(dis);
break;
case 4:
anX = new EntityAssociation();
((EntityAssociation) anX).unmarshal(dis);
break;
}
variableParameters.add(anX);
}
} // end try
catch (Exception e) {
System.out.println(e);
}
} // end of unmarshal method
/**
* Packs a Pdu into the ByteBuffer.
*
* @throws java.nio.BufferOverflowException if buff is too small
* @throws java.nio.ReadOnlyBufferException if buff is read only
* @see java.nio.ByteBuffer
* @param buff The ByteBuffer at the position to begin writing
* @since ??
*/
public void marshal(java.nio.ByteBuffer buff) {
super.marshal(buff);
entityID.marshal(buff);
buff.put((byte) padding1);
buff.put((byte) variableParameters.size());
entityLinearVelocity.marshal(buff);
entityLocation.marshal(buff);
entityOrientation.marshal(buff);
buff.putInt((int) entityAppearance);
for (int idx = 0; idx < variableParameters.size(); idx++) {
VariableParameter aVariableParameter = (VariableParameter) variableParameters.get(idx);
switch (aVariableParameter.getRecordType()) {
case 0:
((ArticulatedParts) aVariableParameter).marshal(buff);
break;
case 1:
((AttachedParts) aVariableParameter).marshal(buff);
break;
case 2:
((SeparationVP) aVariableParameter).marshal(buff);
break;
case 3:
((EntityTypeVP) aVariableParameter).marshal(buff);
break;
case 4:
((EntityAssociation) aVariableParameter).marshal(buff);
break;
}
} // end of list marshalling
} // end of marshal method
/**
* Unpacks a Pdu from the underlying data.
*
* @throws java.nio.BufferUnderflowException if buff is too small
* @see java.nio.ByteBuffer
* @param buff The ByteBuffer at the position to begin reading
* @since ??
*/
public void unmarshal(java.nio.ByteBuffer buff) {
super.unmarshal(buff);
entityID.unmarshal(buff);
padding1 = buff.get();
numberOfVariableParameters = (short) (buff.get() & 0xFF);
entityLinearVelocity.unmarshal(buff);
entityLocation.unmarshal(buff);
entityOrientation.unmarshal(buff);
entityAppearance = buff.getInt();
for (int idx = 0; idx < numberOfVariableParameters; idx++) {
VariableParameter anX = new VariableParameter();
anX.unmarshal(buff);
switch (anX.getRecordType()) {
case 0:
anX = new ArticulatedParts();
((ArticulatedParts) anX).unmarshal(buff);
break;
case 1:
anX = new AttachedParts();
((AttachedParts) anX).unmarshal(buff);
break;
case 2:
anX = new SeparationVP();
((SeparationVP) anX).unmarshal(buff);
break;
case 3:
anX = new EntityTypeVP();
((EntityTypeVP) anX).unmarshal(buff);
break;
case 4:
anX = new EntityAssociation();
((EntityAssociation) anX).unmarshal(buff);
break;
}
variableParameters.add(anX);
}
} // end of unmarshal method
/*
* The equals method doesn't always work--mostly it works only on classes that consist only of primitives. Be careful.
*/
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
return equalsImpl(obj);
}
@Override
public boolean equalsImpl(Object obj) {
boolean ivarsEqual = true;
if (!(obj instanceof EntityStateUpdatePdu)) {
return false;
}
final EntityStateUpdatePdu rhs = (EntityStateUpdatePdu) obj;
if (!(entityID.equals(rhs.entityID))) {
ivarsEqual = false;
}
if (!(padding1 == rhs.padding1)) {
ivarsEqual = false;
}
if (!(numberOfVariableParameters == rhs.numberOfVariableParameters)) {
ivarsEqual = false;
}
if (!(entityLinearVelocity.equals(rhs.entityLinearVelocity))) {
ivarsEqual = false;
}
if (!(entityLocation.equals(rhs.entityLocation))) {
ivarsEqual = false;
}
if (!(entityOrientation.equals(rhs.entityOrientation))) {
ivarsEqual = false;
}
if (!(entityAppearance == rhs.entityAppearance)) {
ivarsEqual = false;
}
for (int idx = 0; idx < variableParameters.size(); idx++) {
if (variableParameters.get(idx).getRecordType() != rhs.variableParameters.get(idx).getRecordType()) {
ivarsEqual = false;
} else {
switch (variableParameters.get(idx).getRecordType()) {
case 0:
if (!((ArticulatedParts) variableParameters.get(idx)).equalsImpl(((ArticulatedParts) rhs.variableParameters.get(idx)))) {
ivarsEqual = false;
}
break;
case 1:
if (!((AttachedParts) variableParameters.get(idx)).equalsImpl(((AttachedParts) rhs.variableParameters.get(idx)))) {
ivarsEqual = false;
}
break;
case 2:
if (!((SeparationVP) variableParameters.get(idx)).equalsImpl(((AttachedParts) rhs.variableParameters.get(idx)))) {
ivarsEqual = false;
}
break;
case 3:
if (!((EntityTypeVP) variableParameters.get(idx)).equalsImpl(((EntityTypeVP) rhs.variableParameters.get(idx)))) {
ivarsEqual = false;
}
break;
case 4:
if (!((EntityAssociation) variableParameters.get(idx)).equalsImpl(((EntityAssociation) rhs.variableParameters.get(idx)))) {
ivarsEqual = false;
}
break;
}
}
}
return ivarsEqual && super.equalsImpl(rhs);
}
} // end of class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy