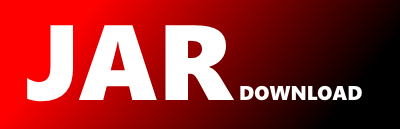
edu.sc.seis.seisFile.fdsnws.FDSNStationQueryParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of seisFile Show documentation
Show all versions of seisFile Show documentation
A library for reading and writing seismic file formats in java.
The newest version!
package edu.sc.seis.seisFile.fdsnws;
import java.time.Instant;
import edu.sc.seis.seisFile.BoxArea;
import edu.sc.seis.seisFile.DonutArea;
import edu.sc.seis.seisFile.fdsnws.stationxml.Channel;
/** Autogenerated by groovy FDSNQueryParamGenerator.groovy in src/metacode/groovy
*/
public class FDSNStationQueryParams extends AbstractQueryParams implements Cloneable {
public FDSNStationQueryParams() {
this(DEFAULT_HOST);
}
public FDSNStationQueryParams(String host) {
super(host==null ? DEFAULT_HOST : host);
}
public FDSNStationQueryParams clone() {
FDSNStationQueryParams out = new FDSNStationQueryParams(getHost());
out.cloneNonParams(this);
for (String key : params.keySet()) {
out.setParam(key, params.get(key));
}
return out;
}
public FDSNStationQueryParams setHost(String host) {
this.host = host;
return this;
}
public FDSNStationQueryParams setPort(int port) {
this.port = port;
return this;
}
public static final String STARTTIME = "starttime";
public static final String STARTTIME_SHORT = "start";
public FDSNStationQueryParams clearStartTime() {
clearParam(STARTTIME);
return this;
}
/** Limit to metadata epochs starting on or after the specified start time.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setStartTime(Instant value) {
setParam(STARTTIME, value);
return this;
}
public static final String ENDTIME = "endtime";
public static final String ENDTIME_SHORT = "end";
public FDSNStationQueryParams clearEndTime() {
clearParam(ENDTIME);
return this;
}
/** Limit to metadata epochs ending on or before the specified end time.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setEndTime(Instant value) {
setParam(ENDTIME, value);
return this;
}
public static final String STARTBEFORE = "startbefore";
public FDSNStationQueryParams clearStartBefore() {
clearParam(STARTBEFORE);
return this;
}
/** Limit to metadata epochs starting before specified time.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setStartBefore(Instant value) {
setParam(STARTBEFORE, value);
return this;
}
public static final String STARTAFTER = "startafter";
public FDSNStationQueryParams clearStartAfter() {
clearParam(STARTAFTER);
return this;
}
/** Limit to metadata epochs starting after specified time.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setStartAfter(Instant value) {
setParam(STARTAFTER, value);
return this;
}
public static final String ENDBEFORE = "endbefore";
public FDSNStationQueryParams clearEndBefore() {
clearParam(ENDBEFORE);
return this;
}
/** Limit to metadata epochs ending before specified time.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setEndBefore(Instant value) {
setParam(ENDBEFORE, value);
return this;
}
public static final String ENDAFTER = "endafter";
public FDSNStationQueryParams clearEndAfter() {
clearParam(ENDAFTER);
return this;
}
/** Limit to metadata epochs ending after specified time.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setEndAfter(Instant value) {
setParam(ENDAFTER, value);
return this;
}
public static final String NETWORK = "network";
public static final String NETWORK_SHORT = "net";
public FDSNStationQueryParams clearNetwork() {
clearParam(NETWORK);
return this;
}
/** Select one or more network codes. Can be SEED network codes or data center defined codes. Multiple codes are comma-separated.
*/
public FDSNStationQueryParams setNetwork(String[] value) {
clearNetwork();
for(String v: value) appendToNetwork(v);
return this;
}
public FDSNStationQueryParams appendToNetwork(String value) {
appendToParam(NETWORK, value);
return this;
}
public static final String STATION = "station";
public static final String STATION_SHORT = "sta";
public FDSNStationQueryParams clearStation() {
clearParam(STATION);
return this;
}
/** Select one or more SEED station codes. Multiple codes are comma-separated.
*/
public FDSNStationQueryParams setStation(String[] value) {
clearStation();
for(String v: value) appendToStation(v);
return this;
}
public FDSNStationQueryParams appendToStation(String value) {
appendToParam(STATION, value);
return this;
}
public static final String LOCATION = "location";
public static final String LOCATION_SHORT = "loc";
public FDSNStationQueryParams clearLocation() {
clearParam(LOCATION);
return this;
}
/** Select one or more SEED location identifiers. Multiple identifiers are comma-separated. As a special case -- (two dashes) will be translated to a string of two space characters to match blank location IDs.
*/
public FDSNStationQueryParams setLocation(String[] value) {
clearLocation();
for(String v: value) appendToLocation(v);
return this;
}
public FDSNStationQueryParams appendToLocation(String value) {
if (value == null || Channel.EMPTY_LOC_CODE.equals(value.trim())) { value = "--";}
appendToParam(LOCATION, value);
return this;
}
public static final String CHANNEL = "channel";
public static final String CHANNEL_SHORT = "cha";
public FDSNStationQueryParams clearChannel() {
clearParam(CHANNEL);
return this;
}
/** Select one or more SEED channel codes. Multiple codes are comma-separated.
*/
public FDSNStationQueryParams setChannel(String[] value) {
clearChannel();
for(String v: value) appendToChannel(v);
return this;
}
public FDSNStationQueryParams appendToChannel(String value) {
appendToParam(CHANNEL, value);
return this;
}
public static final String MINLATITUDE = "minlatitude";
public static final String MINLATITUDE_SHORT = "minlat";
public FDSNStationQueryParams clearMinLatitude() {
clearParam(MINLATITUDE);
return this;
}
/** Limit to stations with a latitude larger than the specified minimum.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setMinLatitude(float value) {
setParam(MINLATITUDE, value);
return this;
}
public static final String MAXLATITUDE = "maxlatitude";
public static final String MAXLATITUDE_SHORT = "maxlat";
public FDSNStationQueryParams clearMaxLatitude() {
clearParam(MAXLATITUDE);
return this;
}
/** Limit to stations with a latitude smaller than the specified maximum.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setMaxLatitude(float value) {
setParam(MAXLATITUDE, value);
return this;
}
public static final String MINLONGITUDE = "minlongitude";
public static final String MINLONGITUDE_SHORT = "minlon";
public FDSNStationQueryParams clearMinLongitude() {
clearParam(MINLONGITUDE);
return this;
}
/** Limit to stations with a longitude larger than the specified minimum.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setMinLongitude(float value) {
setParam(MINLONGITUDE, value);
return this;
}
public static final String MAXLONGITUDE = "maxlongitude";
public static final String MAXLONGITUDE_SHORT = "maxlon";
public FDSNStationQueryParams clearMaxLongitude() {
clearParam(MAXLONGITUDE);
return this;
}
/** Limit to stations with a longitude smaller than the specified maximum.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setMaxLongitude(float value) {
setParam(MAXLONGITUDE, value);
return this;
}
public static final String LATITUDE = "latitude";
public static final String LATITUDE_SHORT = "lat";
public FDSNStationQueryParams clearLatitude() {
clearParam(LATITUDE);
return this;
}
/** Specify the latitude to be used for a radius search.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setLatitude(float value) {
setParam(LATITUDE, value);
return this;
}
public static final String LONGITUDE = "longitude";
public static final String LONGITUDE_SHORT = "lon";
public FDSNStationQueryParams clearLongitude() {
clearParam(LONGITUDE);
return this;
}
/** Specify the longitude to the used for a radius search.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setLongitude(float value) {
setParam(LONGITUDE, value);
return this;
}
public static final String MINRADIUS = "minradius";
public FDSNStationQueryParams clearMinRadius() {
clearParam(MINRADIUS);
return this;
}
/** Limit results to stations within the specified minimum number of degrees from the geographic point defined by the latitude and longitude parameters.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setMinRadius(float value) {
setParam(MINRADIUS, value);
return this;
}
public static final String MAXRADIUS = "maxradius";
public FDSNStationQueryParams clearMaxRadius() {
clearParam(MAXRADIUS);
return this;
}
/** Limit results to stations within the specified maximum number of degrees from the geographic point defined by the latitude and longitude parameters.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setMaxRadius(float value) {
setParam(MAXRADIUS, value);
return this;
}
public static final String LEVEL = "level";
public FDSNStationQueryParams clearLevel() {
clearParam(LEVEL);
return this;
}
/** Specify the level of detail for the results.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setLevel(String value) {
setParam(LEVEL, value);
return this;
}
public static final String INCLUDERESTRICTED = "includerestricted";
public FDSNStationQueryParams clearIncludeRestricted() {
clearParam(INCLUDERESTRICTED);
return this;
}
/** Specify if results should include information for restricted stations.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setIncludeRestricted(boolean value) {
setParam(INCLUDERESTRICTED, value);
return this;
}
public static final String INCLUDEAVAILABILITY = "includeavailability";
public FDSNStationQueryParams clearIncludeAvailability() {
clearParam(INCLUDEAVAILABILITY);
return this;
}
/** Specify if results should include information about time series data availability.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setIncludeAvailability(boolean value) {
setParam(INCLUDEAVAILABILITY, value);
return this;
}
public static final String MATCHTIMESERIES = "matchtimeseries";
public FDSNStationQueryParams clearMatchTimeseries() {
clearParam(MATCHTIMESERIES);
return this;
}
/** Limit to metadata where selection criteria matches time series data availability.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setMatchTimeseries(boolean value) {
setParam(MATCHTIMESERIES, value);
return this;
}
public static final String UPDATEDAFTER = "updatedafter";
public FDSNStationQueryParams clearUpdatedAfter() {
clearParam(UPDATEDAFTER);
return this;
}
/** Limit to metadata updated after specified date; updates are data center specific.
* @param value value to set
* @return the queryParams for method chaining
*/
public FDSNStationQueryParams setUpdatedAfter(Instant value) {
setParam(UPDATEDAFTER, value);
return this;
}
public FDSNStationQueryParams boxArea(BoxArea box) {
return area(box.south, box.north, box.west, box.east);
}
public FDSNStationQueryParams area(float minLat, float maxLat, float minLon, float maxLon) {
return setMinLatitude(minLat).setMaxLatitude(maxLat).setMinLongitude(minLon).setMaxLongitude(maxLon);
}
public FDSNStationQueryParams ring(float lat, float lon, float maxRadius) {
return setLatitude(lat).setLongitude(lon).setMaxRadius(maxRadius);
}
public FDSNStationQueryParams donut(DonutArea donut) {
return ring(donut.latitude, donut.longitude, donut.maxradius).setMinRadius(donut.minradius);
}
public static final String LEVEL_NETWORK = QueryLevel.network.name();
public static final String LEVEL_STATION = QueryLevel.station.name();
public static final String LEVEL_CHANNEL = QueryLevel.channel.name();
public static final String LEVEL_RESPONSE = QueryLevel.response.name();
@Override
public String getServiceName() {
return STATION_SERVICE;
}
public static final String STATION_SERVICE = "station";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy