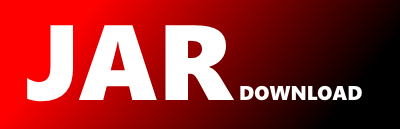
edu.stanford.nlp.util.PropertiesUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stanford-parser Show documentation
Show all versions of stanford-parser Show documentation
Stanford Parser processes raw text in English, Chinese, German, Arabic, and French, and extracts constituency parse trees.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy