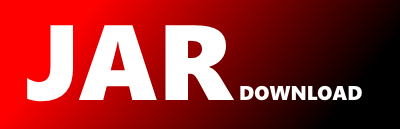
org.protege.owl.codegeneration.property.JavaPropertyDeclarationCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of code-generation Show documentation
Show all versions of code-generation Show documentation
Generate Java code from an OWL ontology in the Protege Desktop editing environment.
The newest version!
package org.protege.owl.codegeneration.property;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.TreeSet;
import org.protege.owl.codegeneration.inference.CodeGenerationInference;
import org.protege.owl.codegeneration.names.CodeGenerationNames;
import org.semanticweb.owlapi.model.OWLClass;
import org.semanticweb.owlapi.model.OWLDataFactory;
import org.semanticweb.owlapi.model.OWLDataProperty;
import org.semanticweb.owlapi.model.OWLEntity;
import org.semanticweb.owlapi.model.OWLObject;
import org.semanticweb.owlapi.model.OWLObjectProperty;
/**
* This class provides a java property declarations object for any class and property. The
* primary responsibility of this class is to ensure that if
*
* - A class or interface X has a method m,
*
- A class or interface Y extends/implements the class X
*
* then it must be true that the class or interface Y has a method that specializes the method m. By
* putting the code to ensure this property here we release other classes (such as the code generation inference)
* from concerning themselves with this issue.
*
*
*/
public class JavaPropertyDeclarationCache {
private CodeGenerationInference inference;
private CodeGenerationNames names;;
private Map> class2Property2DeclarationMap
= new HashMap>();
public JavaPropertyDeclarationCache(CodeGenerationInference inference, CodeGenerationNames names) {
this.inference = inference;
this.names = names;
generateCache();
}
private void generateCache() {
OWLDataFactory factory = inference.getOWLOntology().getOWLOntologyManager().getOWLDataFactory();
Set explored = new HashSet();
OWLClass thing = factory.getOWLThing();
for (JavaPropertyDeclaration declarations : inference.getJavaPropertyDeclarations(thing, names)) {
add(thing, declarations.getOwlProperty(), declarations);
}
generateChildren(thing, explored);
}
private void generateChildren(OWLClass parent, Set explored) {
if (explored.contains(parent)) {
return;
}
explored.add(parent);
Map parentProperty2DeclarationMap = class2Property2DeclarationMap.get(parent);
if (parentProperty2DeclarationMap == null) {
parentProperty2DeclarationMap = Collections.emptyMap();
}
for (OWLClass child : inference.getSubClasses(parent)) {
copyDeclarations(parentProperty2DeclarationMap, parent, child);
generateChildren(child, explored);
}
}
private void copyDeclarations(Map parentProperty2DeclarationMap,
OWLClass parent,
OWLClass child) {
for (Entry entry : parentProperty2DeclarationMap.entrySet()) {
OWLEntity property = entry.getKey();
JavaPropertyDeclaration declarations = entry.getValue();
add(child, property, declarations.specializeTo(child));
}
for (JavaPropertyDeclaration childDeclarations : inference.getJavaPropertyDeclarations(child, names)) {
OWLEntity property = childDeclarations.getOwlProperty();
if (!parentProperty2DeclarationMap.containsKey(property)) {
add(child, property, childDeclarations);
}
}
}
private void add(OWLClass owlClass, OWLEntity property, JavaPropertyDeclaration declarations) {
Map property2DeclarationsMap = class2Property2DeclarationMap.get(owlClass);
if (property2DeclarationsMap == null) {
property2DeclarationsMap = new HashMap();
class2Property2DeclarationMap.put(owlClass, property2DeclarationsMap);
}
property2DeclarationsMap.put(property, declarations);
}
public JavaPropertyDeclaration get(OWLClass clazz, OWLEntity property) {
Map property2DeclarationMap = class2Property2DeclarationMap.get(clazz);
JavaPropertyDeclaration decls = null;
if (property2DeclarationMap != null) {
decls = property2DeclarationMap.get(property);
}
return decls;
}
public Set getObjectPropertiesForClass(OWLClass owlClass) {
return getPropertiesForClass(owlClass, OWLObjectProperty.class);
}
public Set getDataPropertiesForClass(OWLClass owlClass) {
return getPropertiesForClass(owlClass, OWLDataProperty.class);
}
private > Set getPropertiesForClass(OWLClass owlClass, Class extends X> javaClass) {
Map property2DeclarationMap = class2Property2DeclarationMap.get(owlClass);
if (property2DeclarationMap == null) {
return Collections.emptySet();
}
Set properties = new TreeSet();
for (OWLEntity property : property2DeclarationMap.keySet()) {
if (javaClass.isAssignableFrom(property.getClass())) {
properties.add(javaClass.cast(property));
}
}
return properties;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy