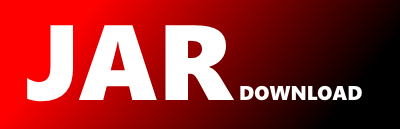
edu.stanford.protege.webprotege.projectsettings.AutoValue_EntityDeprecationSettings Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.projectsettings;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import edu.stanford.protege.webprotege.criteria.CompositeRootCriteria;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.semanticweb.owlapi.model.IRI;
import org.semanticweb.owlapi.model.OWLAnnotationProperty;
import org.semanticweb.owlapi.model.OWLClass;
import org.semanticweb.owlapi.model.OWLDataProperty;
import org.semanticweb.owlapi.model.OWLObjectProperty;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EntityDeprecationSettings extends EntityDeprecationSettings {
private final IRI replacedByPropertyIriInternal;
private final CompositeRootCriteria replacedByFilterInternal;
private final OWLClass deprecatedClassesParentInternal;
private final OWLObjectProperty deprecatedObjectPropertiesParentInternal;
private final OWLDataProperty deprecatedDataPropertiesParentInternal;
private final OWLAnnotationProperty deprecatedAnnotationPropertiesParentInternal;
private final OWLClass deprecatedIndividualsParentInternal;
AutoValue_EntityDeprecationSettings(
@Nullable IRI replacedByPropertyIriInternal,
@Nullable CompositeRootCriteria replacedByFilterInternal,
@Nullable OWLClass deprecatedClassesParentInternal,
@Nullable OWLObjectProperty deprecatedObjectPropertiesParentInternal,
@Nullable OWLDataProperty deprecatedDataPropertiesParentInternal,
@Nullable OWLAnnotationProperty deprecatedAnnotationPropertiesParentInternal,
@Nullable OWLClass deprecatedIndividualsParentInternal) {
this.replacedByPropertyIriInternal = replacedByPropertyIriInternal;
this.replacedByFilterInternal = replacedByFilterInternal;
this.deprecatedClassesParentInternal = deprecatedClassesParentInternal;
this.deprecatedObjectPropertiesParentInternal = deprecatedObjectPropertiesParentInternal;
this.deprecatedDataPropertiesParentInternal = deprecatedDataPropertiesParentInternal;
this.deprecatedAnnotationPropertiesParentInternal = deprecatedAnnotationPropertiesParentInternal;
this.deprecatedIndividualsParentInternal = deprecatedIndividualsParentInternal;
}
@JsonProperty("replacedByPropertyIri")
@Nullable
@Override
IRI getReplacedByPropertyIriInternal() {
return replacedByPropertyIriInternal;
}
@JsonIgnore
@Nullable
@Override
CompositeRootCriteria getReplacedByFilterInternal() {
return replacedByFilterInternal;
}
@JsonProperty("deprecatedClassesParent")
@Nullable
@Override
OWLClass getDeprecatedClassesParentInternal() {
return deprecatedClassesParentInternal;
}
@JsonProperty("deprecatedObjectPropertiesParent")
@Nullable
@Override
OWLObjectProperty getDeprecatedObjectPropertiesParentInternal() {
return deprecatedObjectPropertiesParentInternal;
}
@JsonProperty("deprecatedDataPropertiesParent")
@Nullable
@Override
OWLDataProperty getDeprecatedDataPropertiesParentInternal() {
return deprecatedDataPropertiesParentInternal;
}
@JsonProperty("deprecatedAnnotationPropertiesParent")
@Nullable
@Override
OWLAnnotationProperty getDeprecatedAnnotationPropertiesParentInternal() {
return deprecatedAnnotationPropertiesParentInternal;
}
@JsonProperty("deprecatedIndividualsParent")
@Nullable
@Override
OWLClass getDeprecatedIndividualsParentInternal() {
return deprecatedIndividualsParentInternal;
}
@Override
public String toString() {
return "EntityDeprecationSettings{"
+ "replacedByPropertyIriInternal=" + replacedByPropertyIriInternal + ", "
+ "replacedByFilterInternal=" + replacedByFilterInternal + ", "
+ "deprecatedClassesParentInternal=" + deprecatedClassesParentInternal + ", "
+ "deprecatedObjectPropertiesParentInternal=" + deprecatedObjectPropertiesParentInternal + ", "
+ "deprecatedDataPropertiesParentInternal=" + deprecatedDataPropertiesParentInternal + ", "
+ "deprecatedAnnotationPropertiesParentInternal=" + deprecatedAnnotationPropertiesParentInternal + ", "
+ "deprecatedIndividualsParentInternal=" + deprecatedIndividualsParentInternal
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EntityDeprecationSettings) {
EntityDeprecationSettings that = (EntityDeprecationSettings) o;
return (this.replacedByPropertyIriInternal == null ? that.getReplacedByPropertyIriInternal() == null : this.replacedByPropertyIriInternal.equals(that.getReplacedByPropertyIriInternal()))
&& (this.replacedByFilterInternal == null ? that.getReplacedByFilterInternal() == null : this.replacedByFilterInternal.equals(that.getReplacedByFilterInternal()))
&& (this.deprecatedClassesParentInternal == null ? that.getDeprecatedClassesParentInternal() == null : this.deprecatedClassesParentInternal.equals(that.getDeprecatedClassesParentInternal()))
&& (this.deprecatedObjectPropertiesParentInternal == null ? that.getDeprecatedObjectPropertiesParentInternal() == null : this.deprecatedObjectPropertiesParentInternal.equals(that.getDeprecatedObjectPropertiesParentInternal()))
&& (this.deprecatedDataPropertiesParentInternal == null ? that.getDeprecatedDataPropertiesParentInternal() == null : this.deprecatedDataPropertiesParentInternal.equals(that.getDeprecatedDataPropertiesParentInternal()))
&& (this.deprecatedAnnotationPropertiesParentInternal == null ? that.getDeprecatedAnnotationPropertiesParentInternal() == null : this.deprecatedAnnotationPropertiesParentInternal.equals(that.getDeprecatedAnnotationPropertiesParentInternal()))
&& (this.deprecatedIndividualsParentInternal == null ? that.getDeprecatedIndividualsParentInternal() == null : this.deprecatedIndividualsParentInternal.equals(that.getDeprecatedIndividualsParentInternal()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (replacedByPropertyIriInternal == null) ? 0 : replacedByPropertyIriInternal.hashCode();
h$ *= 1000003;
h$ ^= (replacedByFilterInternal == null) ? 0 : replacedByFilterInternal.hashCode();
h$ *= 1000003;
h$ ^= (deprecatedClassesParentInternal == null) ? 0 : deprecatedClassesParentInternal.hashCode();
h$ *= 1000003;
h$ ^= (deprecatedObjectPropertiesParentInternal == null) ? 0 : deprecatedObjectPropertiesParentInternal.hashCode();
h$ *= 1000003;
h$ ^= (deprecatedDataPropertiesParentInternal == null) ? 0 : deprecatedDataPropertiesParentInternal.hashCode();
h$ *= 1000003;
h$ ^= (deprecatedAnnotationPropertiesParentInternal == null) ? 0 : deprecatedAnnotationPropertiesParentInternal.hashCode();
h$ *= 1000003;
h$ ^= (deprecatedIndividualsParentInternal == null) ? 0 : deprecatedIndividualsParentInternal.hashCode();
return h$;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy