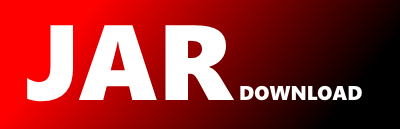
edu.stanford.protege.webprotege.viz.AutoValue_EntityGraph Maven / Gradle / Ivy
package edu.stanford.protege.webprotege.viz;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.ImmutableSetMultimap;
import edu.stanford.protege.webprotege.entity.OWLEntityData;
import javax.annotation.Nonnull;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EntityGraph extends EntityGraph {
private final OWLEntityData root;
private final int nodeCount;
private final ImmutableSet nodes;
private final int edgeCount;
private final ImmutableSet edges;
private final boolean prunedToEdgeLimit;
private final ImmutableSetMultimap edgesByTailNode;
AutoValue_EntityGraph(
OWLEntityData root,
int nodeCount,
ImmutableSet nodes,
int edgeCount,
ImmutableSet edges,
boolean prunedToEdgeLimit,
ImmutableSetMultimap edgesByTailNode) {
if (root == null) {
throw new NullPointerException("Null root");
}
this.root = root;
this.nodeCount = nodeCount;
if (nodes == null) {
throw new NullPointerException("Null nodes");
}
this.nodes = nodes;
this.edgeCount = edgeCount;
if (edges == null) {
throw new NullPointerException("Null edges");
}
this.edges = edges;
this.prunedToEdgeLimit = prunedToEdgeLimit;
if (edgesByTailNode == null) {
throw new NullPointerException("Null edgesByTailNode");
}
this.edgesByTailNode = edgesByTailNode;
}
@Nonnull
@Override
public OWLEntityData getRoot() {
return root;
}
@JsonIgnore
@Override
public int getNodeCount() {
return nodeCount;
}
@JsonIgnore
@Nonnull
@Override
public ImmutableSet getNodes() {
return nodes;
}
@JsonIgnore
@Override
public int getEdgeCount() {
return edgeCount;
}
@Nonnull
@Override
public ImmutableSet getEdges() {
return edges;
}
@Override
public boolean isPrunedToEdgeLimit() {
return prunedToEdgeLimit;
}
@JsonIgnore
@Nonnull
@Override
public ImmutableSetMultimap getEdgesByTailNode() {
return edgesByTailNode;
}
@Override
public String toString() {
return "EntityGraph{"
+ "root=" + root + ", "
+ "nodeCount=" + nodeCount + ", "
+ "nodes=" + nodes + ", "
+ "edgeCount=" + edgeCount + ", "
+ "edges=" + edges + ", "
+ "prunedToEdgeLimit=" + prunedToEdgeLimit + ", "
+ "edgesByTailNode=" + edgesByTailNode
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EntityGraph) {
EntityGraph that = (EntityGraph) o;
return this.root.equals(that.getRoot())
&& this.nodeCount == that.getNodeCount()
&& this.nodes.equals(that.getNodes())
&& this.edgeCount == that.getEdgeCount()
&& this.edges.equals(that.getEdges())
&& this.prunedToEdgeLimit == that.isPrunedToEdgeLimit()
&& this.edgesByTailNode.equals(that.getEdgesByTailNode());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= root.hashCode();
h$ *= 1000003;
h$ ^= nodeCount;
h$ *= 1000003;
h$ ^= nodes.hashCode();
h$ *= 1000003;
h$ ^= edgeCount;
h$ *= 1000003;
h$ ^= edges.hashCode();
h$ *= 1000003;
h$ ^= prunedToEdgeLimit ? 1231 : 1237;
h$ *= 1000003;
h$ ^= edgesByTailNode.hashCode();
return h$;
}
}