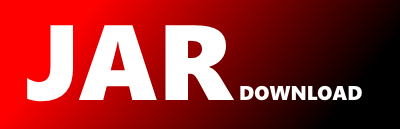
edu.stanford.protege.webprotege.access.AccessManager Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.access;
import edu.stanford.protege.webprotege.authorization.*;
import edu.stanford.protege.webprotege.ipc.ExecutionContext;
import javax.annotation.Nonnull;
import java.util.Collection;
import java.util.Set;
/**
* Matthew Horridge
* Stanford Center for Biomedical Informatics Research
* 4 Jan 2017
*/
public interface AccessManager {
/**
* Get the role ids that have been assigned to the specified subject for the specified resource.
* @param subject The subject.
* @param resource The resoruce.
* @return The assigned role ids for the subject and resource pair.
*/
@Nonnull
Collection getAssignedRoles(@Nonnull Subject subject,
@Nonnull Resource resource);
/**
* Sets the assigned roles for the specified subject and resource pair.
* @param subject The subject.
* @param resource The resource.
* @param roleIds The role ids to be assigned. These will replace any existing assigned role ids for the
* specified subject and resource pair.
*/
void setAssignedRoles(@Nonnull Subject subject,
@Nonnull Resource resource,
@Nonnull Collection roleIds);
/**
* Gets the role closure for the specified subject and resource pair.
* @param subject The subject.
* @param resource The resource.
* @return A collection of role ids that are in the role closure for the specified subject and resource pair.
*/
@Nonnull
Collection getRoleClosure(@Nonnull Subject subject,
@Nonnull Resource resource);
/**
* Gets the action closure for the specified subject and resource pair.
* @param subject The subject.
* @param resource The resource.
* @return A collection of action ids that belong to the role closure of the specified subject and resource pair.
*/
@Nonnull
Set getActionClosure(@Nonnull Subject subject,
@Nonnull Resource resource,
ExecutionContext executionContext);
/**
* Tests to see if the specified subject has permission to execute the specified action on the specified resource.
* @param subject The subject.
* @param resource The resource on which the action should be executed.
* @param actionId The action to be executed.
* @return {@code true} if the subject has permission to execute the specified action on the specified resource,
* otherwise {@code false}.
*/
boolean hasPermission(@Nonnull Subject subject,
@Nonnull Resource resource,
@Nonnull ActionId actionId);
boolean hasPermission(@Nonnull Subject subject,
@Nonnull ApplicationResource resource,
@Nonnull ActionId actionId,
ExecutionContext executionContext);
/**
* Tests to see if the specified subject has permission to execute the specified action on the specified resource.
* @param subject The subject.
* @param resource The resource on which the action should be executed.
* @param builtInAction The action to be executed.
* @return {@code true} if the subject has permission to execute the specified action on the specified resource,
* otherwise {@code false}.
*/
boolean hasPermission(@Nonnull Subject subject,
@Nonnull Resource resource,
@Nonnull BuiltInAction builtInAction);
Collection getSubjectsWithAccessToResource(Resource resource);
Collection getSubjectsWithAccessToResource(Resource resource, BuiltInAction action);
Collection getResourcesAccessibleToSubject(Subject subject, ActionId actionId, ExecutionContext executionContext);
/**
* Rebuilds the role and action closure for all subjects and resources.
*/
void rebuild();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy