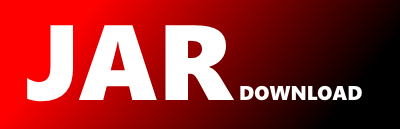
edu.stanford.protege.webprotege.access.RoleAssignment Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.access;
import com.google.common.base.Objects;
import com.google.common.collect.ImmutableList;
import org.bson.types.ObjectId;
import org.springframework.data.mongodb.core.index.CompoundIndex;
import org.springframework.data.mongodb.core.index.CompoundIndexes;
import org.springframework.data.mongodb.core.mapping.Document;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.List;
import java.util.Optional;
import static com.google.common.base.MoreObjects.toStringHelper;
import static com.google.common.base.Preconditions.checkNotNull;
/**
* Matthew Horridge
* Stanford Center for Biomedical Informatics Research
* 7 Jan 2017
*
* A persistence structure for role assignments. This assumes the persistence is provided by
* MongoDb, which is access via Morphia.
*/
@Document(collection = "RoleAssignments")
@CompoundIndexes({
@CompoundIndex(def = "{'userName':1, 'projectId':1}", unique = true)
})
public class RoleAssignment {
public static final String USER_NAME = "userName";
public static final String PROJECT_ID = "projectId";
public static final String ACTION_CLOSURE = "actionClosure";
public static final String ROLE_CLOSURE = "roleClosure";
@Nullable
@SuppressWarnings("unused")
private ObjectId id;
@Nullable
private String userName;
@Nullable
private String projectId;
private List assignedRoles = ImmutableList.of();
private List roleClosure = ImmutableList.of();
private List actionClosure = ImmutableList.of();
private RoleAssignment() {
}
public RoleAssignment(@Nullable String userName,
@Nullable String projectId,
@Nonnull List assignedRoles,
@Nonnull List roleClosure,
@Nonnull List actionClosure) {
this.userName = userName;
this.projectId = projectId;
this.assignedRoles = ImmutableList.copyOf(checkNotNull(assignedRoles));
this.roleClosure = ImmutableList.copyOf(checkNotNull(roleClosure));
this.actionClosure = ImmutableList.copyOf(checkNotNull(actionClosure));
}
@Nonnull
public Optional getProjectId() {
return Optional.ofNullable(projectId);
}
@Nonnull
public Optional getUserName() {
return Optional.ofNullable(userName);
}
@Nonnull
public List getAssignedRoles() {
return ImmutableList.copyOf(assignedRoles);
}
@Nonnull
public List getRoleClosure() {
return ImmutableList.copyOf(roleClosure);
}
@Nonnull
public List getActionClosure() {
return ImmutableList.copyOf(actionClosure);
}
@Override
public int hashCode() {
return Objects.hashCode(userName, projectId, assignedRoles, roleClosure, actionClosure);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof RoleAssignment)) {
return false;
}
RoleAssignment other = (RoleAssignment) obj;
return Objects.equal(userName, other.userName)
&& Objects.equal(projectId, other.projectId)
&& this.assignedRoles.equals(other.assignedRoles)
&& this.roleClosure.equals(other.roleClosure)
&& this.actionClosure.equals(other.actionClosure);
}
@Override
public String toString() {
return toStringHelper("RoleAssignment")
.add("userName", userName)
.add("projectId", projectId)
.add("assignedRoles", assignedRoles)
.add("roleClosure", roleClosure)
.add("actionClosure", actionClosure)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy