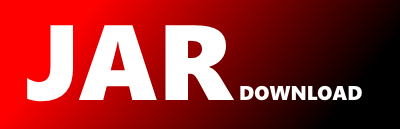
edu.stanford.protege.webprotege.change.ReverseEngineeredChangeDescriptionGenerator Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.change;
import edu.stanford.protege.webprotege.change.matcher.ChangeMatcher;
import edu.stanford.protege.webprotege.change.matcher.ChangeSummary;
import edu.stanford.protege.webprotege.owlapi.OWLObjectStringFormatter;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import static com.google.common.base.Preconditions.checkNotNull;
/**
* Matthew Horridge
* Stanford Center for Biomedical Informatics Research
* 16/03/16
*/
public class ReverseEngineeredChangeDescriptionGenerator implements ChangeDescriptionGenerator {
@Nonnull
private final String defaultDescription;
@Nonnull
private final List matchers;
@Nonnull
private final OWLObjectStringFormatter formatter;
@Inject
public ReverseEngineeredChangeDescriptionGenerator(@Nonnull String defaultDescription,
@Nonnull Set matchers,
@Nonnull OWLObjectStringFormatter formatter) {
this.defaultDescription = checkNotNull(defaultDescription);
this.matchers = new ArrayList<>(matchers);
this.formatter = checkNotNull(formatter);
}
@Override
public String generateChangeDescription(ChangeApplicationResult result) {
var changes = result.getChangeList();
for(ChangeMatcher matcher : matchers) {
Optional description = matcher.getDescription(changes);
if(description.isPresent()) {
return description.get().getDescription().formatDescription(formatter);
}
}
return defaultDescription;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy