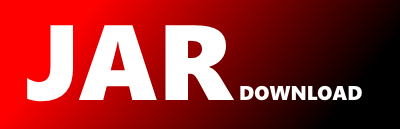
edu.stanford.protege.webprotege.frame.AutoValue_GetManchesterSyntaxFrameCompletionsAction Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.frame;
import edu.stanford.protege.webprotege.common.ProjectId;
import edu.stanford.protege.webprotege.entity.OWLEntityData;
import edu.stanford.protege.webprotege.mansyntax.EditorPosition;
import java.util.Set;
import javax.annotation.Nonnull;
import javax.annotation.processing.Generated;
import org.semanticweb.owlapi.model.OWLEntity;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_GetManchesterSyntaxFrameCompletionsAction extends GetManchesterSyntaxFrameCompletionsAction {
private final ProjectId projectId;
private final OWLEntity getSubject;
private final String getSyntax;
private final EditorPosition getFromPos;
private final int getFrom;
private final Set freshEntities;
private final int getEntityTypeSuggestLimit;
AutoValue_GetManchesterSyntaxFrameCompletionsAction(
ProjectId projectId,
OWLEntity getSubject,
String getSyntax,
EditorPosition getFromPos,
int getFrom,
Set freshEntities,
int getEntityTypeSuggestLimit) {
if (projectId == null) {
throw new NullPointerException("Null projectId");
}
this.projectId = projectId;
if (getSubject == null) {
throw new NullPointerException("Null getSubject");
}
this.getSubject = getSubject;
if (getSyntax == null) {
throw new NullPointerException("Null getSyntax");
}
this.getSyntax = getSyntax;
if (getFromPos == null) {
throw new NullPointerException("Null getFromPos");
}
this.getFromPos = getFromPos;
this.getFrom = getFrom;
if (freshEntities == null) {
throw new NullPointerException("Null freshEntities");
}
this.freshEntities = freshEntities;
this.getEntityTypeSuggestLimit = getEntityTypeSuggestLimit;
}
@Nonnull
@Override
public ProjectId projectId() {
return projectId;
}
@Override
public OWLEntity getSubject() {
return getSubject;
}
@Override
public String getSyntax() {
return getSyntax;
}
@Override
public EditorPosition getFromPos() {
return getFromPos;
}
@Override
public int getFrom() {
return getFrom;
}
@Override
public Set freshEntities() {
return freshEntities;
}
@Override
public int getEntityTypeSuggestLimit() {
return getEntityTypeSuggestLimit;
}
@Override
public String toString() {
return "GetManchesterSyntaxFrameCompletionsAction{"
+ "projectId=" + projectId + ", "
+ "getSubject=" + getSubject + ", "
+ "getSyntax=" + getSyntax + ", "
+ "getFromPos=" + getFromPos + ", "
+ "getFrom=" + getFrom + ", "
+ "freshEntities=" + freshEntities + ", "
+ "getEntityTypeSuggestLimit=" + getEntityTypeSuggestLimit
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof GetManchesterSyntaxFrameCompletionsAction) {
GetManchesterSyntaxFrameCompletionsAction that = (GetManchesterSyntaxFrameCompletionsAction) o;
return this.projectId.equals(that.projectId())
&& this.getSubject.equals(that.getSubject())
&& this.getSyntax.equals(that.getSyntax())
&& this.getFromPos.equals(that.getFromPos())
&& this.getFrom == that.getFrom()
&& this.freshEntities.equals(that.freshEntities())
&& this.getEntityTypeSuggestLimit == that.getEntityTypeSuggestLimit();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= projectId.hashCode();
h$ *= 1000003;
h$ ^= getSubject.hashCode();
h$ *= 1000003;
h$ ^= getSyntax.hashCode();
h$ *= 1000003;
h$ ^= getFromPos.hashCode();
h$ *= 1000003;
h$ ^= getFrom;
h$ *= 1000003;
h$ ^= freshEntities.hashCode();
h$ *= 1000003;
h$ ^= getEntityTypeSuggestLimit;
return h$;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy