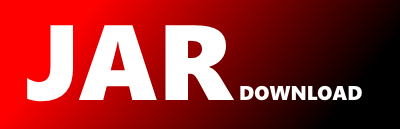
edu.stanford.protege.webprotege.index.BuiltInOwlEntitiesIndexImpl Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.index;
import com.google.common.collect.ImmutableSet;
import org.semanticweb.owlapi.model.*;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import java.util.Collection;
import java.util.stream.Stream;
import static com.google.common.collect.ImmutableSet.toImmutableSet;
/**
* Matthew Horridge
* Stanford Center for Biomedical Informatics Research
* 2020-07-27
*/
public class BuiltInOwlEntitiesIndexImpl implements BuiltInOwlEntitiesIndex {
@Nonnull
private final ImmutableSet classes;
@Nonnull
private final ImmutableSet objectProperties;
@Nonnull
private final ImmutableSet dataProperties;
@Nonnull
private final ImmutableSet annotationProperties;
@Nonnull
private final ImmutableSet builtInEntities;
@Inject
public BuiltInOwlEntitiesIndexImpl(@Nonnull OWLDataFactory dataFactory) {
classes = ImmutableSet.of(
dataFactory.getOWLThing(),
dataFactory.getOWLNothing()
);
objectProperties = ImmutableSet.of(
dataFactory.getOWLTopObjectProperty(),
dataFactory.getOWLBottomObjectProperty()
);
dataProperties = ImmutableSet.of(
dataFactory.getOWLTopDataProperty(),
dataFactory.getOWLBottomDataProperty()
);
annotationProperties = ImmutableSet.of(
dataFactory.getRDFSLabel(),
dataFactory.getRDFSComment(),
dataFactory.getRDFSIsDefinedBy(),
dataFactory.getRDFSSeeAlso(),
dataFactory.getOWLBackwardCompatibleWith(),
dataFactory.getOWLIncompatibleWith()
);
builtInEntities = ImmutableSet.of(classes, objectProperties, dataProperties, annotationProperties)
.stream()
.flatMap(Collection::stream)
.collect(toImmutableSet());
}
@Nonnull
@Override
public Stream getBuiltInEntities() {
return builtInEntities.stream();
}
@Nonnull
@Override
public Stream getAnnotationProperties() {
return annotationProperties.stream();
}
@Nonnull
@Override
public Stream getClasses() {
return classes.stream();
}
@Nonnull
@Override
public Stream getObjectProperties() {
return objectProperties.stream();
}
@Nonnull
@Override
public Stream getDataProperties() {
return dataProperties.stream();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy