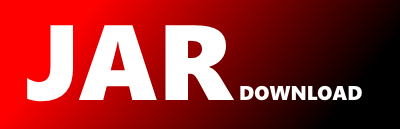
edu.stanford.protege.webprotege.mansyntax.ShellOwlOntology Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.mansyntax;
import org.semanticweb.owlapi.io.OWLOntologyDocumentTarget;
import org.semanticweb.owlapi.model.*;
import org.semanticweb.owlapi.model.parameters.AxiomAnnotations;
import org.semanticweb.owlapi.model.parameters.Imports;
import org.semanticweb.owlapi.model.parameters.Navigation;
import org.semanticweb.owlapi.util.OWLAxiomSearchFilter;
import javax.annotation.Nonnull;
import java.io.OutputStream;
import java.util.Collection;
import java.util.Collections;
import java.util.Set;
import static autovalue.shaded.com.google$.common.base.$MoreObjects.toStringHelper;
import static com.google.common.base.Preconditions.checkNotNull;
/**
* Matthew Horridge
* Stanford Center for Biomedical Informatics Research
* 2019-08-22
*
* The purpose of this class is to fool the Manchester syntax parser into thinking
* that is has a reference to some {@link OWLOntology}. In fact, the parser only
* requires equals, hashCode and getOWLOntologyID.
*/
public class ShellOwlOntology implements OWLOntology {
@Nonnull
private final OWLOntologyID ontologyID;
@Nonnull
public static ShellOwlOntology get(@Nonnull OWLOntologyID ontologyId) {
return new ShellOwlOntology(ontologyId);
}
public ShellOwlOntology(@Nonnull OWLOntologyID ontologyID) {
this.ontologyID = checkNotNull(ontologyID);
}
@Nonnull
@Override
public OWLOntologyID getOntologyID() {
return ontologyID;
}
@Override
public int hashCode() {
return ontologyID.hashCode();
}
@Override
public String toString() {
return toStringHelper("ShellOwlOntology")
.addValue(ontologyID)
.toString();
}
@Override
public boolean equals(Object obj) {
if(obj == this) {
return true;
}
if(!(obj instanceof OWLOntology)) {
return false;
}
OWLOntology other = (OWLOntology) obj;
return this.ontologyID.equals(other.getOntologyID());
}
@Override
public void accept(@Nonnull OWLNamedObjectVisitor visitor) {
}
@Nonnull
@Override
public O accept(@Nonnull OWLNamedObjectVisitorEx visitor) {
throw new RuntimeException("Cannot accept visits");
}
@Nonnull
@Override
public OWLOntologyManager getOWLOntologyManager() {
throw new RuntimeException("Cannot provide OWLOntologyManager");
}
@Override
public void setOWLOntologyManager(OWLOntologyManager manager) {
}
@Override
public boolean isAnonymous() {
return false;
}
@Nonnull
@Override
public Set getAnnotations() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDirectImportsDocuments() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDirectImports() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getImports() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getImportsClosure() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getImportsDeclarations() {
return Collections.emptySet();
}
@Override
public boolean isEmpty() {
return false;
}
@Nonnull
@Override
public Set getTBoxAxioms(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getABoxAxioms(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getRBoxAxioms(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getGeneralClassAxioms() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getSignature() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getSignature(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Override
public boolean isDeclared(@Nonnull OWLEntity owlEntity) {
return false;
}
@Override
public boolean isDeclared(@Nonnull OWLEntity owlEntity, @Nonnull Imports includeImportsClosure) {
return false;
}
@Override
public void saveOntology() {
}
@Override
public void saveOntology(@Nonnull IRI documentIRI) {
}
@Override
public void saveOntology(@Nonnull OutputStream outputStream) {
}
@Override
public void saveOntology(@Nonnull OWLDocumentFormat ontologyFormat) {
}
@Override
public void saveOntology(@Nonnull OWLDocumentFormat ontologyFormat,
@Nonnull IRI documentIRI) {
}
@Override
public void saveOntology(@Nonnull OWLDocumentFormat ontologyFormat,
@Nonnull OutputStream outputStream) {
}
@Override
public void saveOntology(@Nonnull OWLOntologyDocumentTarget documentTarget) {
}
@Override
public void saveOntology(@Nonnull OWLDocumentFormat ontologyFormat,
@Nonnull OWLOntologyDocumentTarget documentTarget) {
}
@Nonnull
@Override
public Set getEntitiesInSignature(@Nonnull IRI entityIRI) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Override
public int getAxiomCount(@Nonnull Imports includeImportsClosure) {
return 0;
}
@Nonnull
@Override
public Set getLogicalAxioms(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Override
public int getLogicalAxiomCount(@Nonnull Imports includeImportsClosure) {
return 0;
}
@Nonnull
@Override
public Set getAxioms(@Nonnull AxiomType axiomType,
@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Override
public int getAxiomCount(@Nonnull AxiomType axiomType,
@Nonnull Imports includeImportsClosure) {
return 0;
}
@Override
public boolean containsAxiom(@Nonnull OWLAxiom axiom,
@Nonnull Imports includeImportsClosure,
@Nonnull AxiomAnnotations ignoreAnnotations) {
return false;
}
@Nonnull
@Override
public Set getAxiomsIgnoreAnnotations(@Nonnull OWLAxiom axiom, @Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getReferencingAxioms(@Nonnull OWLPrimitive owlEntity, @Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLClass cls, @Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLObjectPropertyExpression property,
@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLDataProperty property,
@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLIndividual individual,
@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLAnnotationProperty property,
@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLDatatype datatype,
@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(boolean b) {
return Collections.emptySet();
}
@Override
public int getAxiomCount(boolean includeImportsClosure) {
return 0;
}
@Nonnull
@Override
public Set getLogicalAxioms(boolean includeImportsClosure) {
return Collections.emptySet();
}
@Override
public int getLogicalAxiomCount(boolean includeImportsClosure) {
return 0;
}
@Nonnull
@Override
public Set getAxioms(@Nonnull AxiomType axiomType, boolean includeImportsClosure) {
return Collections.emptySet();
}
@Override
public int getAxiomCount(@Nonnull AxiomType axiomType, boolean includeImportsClosure) {
return 0;
}
@Override
public boolean containsAxiom(@Nonnull OWLAxiom axiom, boolean includeImportsClosure) {
return false;
}
@Override
public boolean containsAxiomIgnoreAnnotations(@Nonnull OWLAxiom axiom, boolean includeImportsClosure) {
return false;
}
@Nonnull
@Override
public Set getAxiomsIgnoreAnnotations(@Nonnull OWLAxiom axiom, boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getReferencingAxioms(@Nonnull OWLPrimitive owlEntity, boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLClass cls, boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLObjectPropertyExpression property,
boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLDataProperty property, boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLIndividual individual, boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLAnnotationProperty property, boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLDatatype datatype, boolean includeImportsClosure) {
return Collections.emptySet();
}
@Override
public int getAxiomCount() {
return 0;
}
@Override
public int getLogicalAxiomCount() {
return 0;
}
@Override
public int getAxiomCount(@Nonnull AxiomType axiomType) {
return 0;
}
@Override
public boolean containsAxiomIgnoreAnnotations(@Nonnull OWLAxiom axiom) {
return false;
}
@Nonnull
@Override
public Set getAxiomsIgnoreAnnotations(@Nonnull OWLAxiom axiom) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getReferencingAxioms(@Nonnull OWLPrimitive owlEntity) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLClass cls) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLDataProperty property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLIndividual individual) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLAnnotationProperty property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull OWLDatatype datatype) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull AxiomType axiomType) {
return Collections.emptySet();
}
@Override
public boolean containsAxiom(@Nonnull OWLAxiom axiom) {
return false;
}
@Nonnull
@Override
public Set getLogicalAxioms() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAxioms(@Nonnull Class type,
@Nonnull OWLObject entity,
@Nonnull Imports includeImports,
@Nonnull Navigation forSubPosition) {
return Collections.emptySet();
}
@Nonnull
@Override
public Collection filterAxioms(@Nonnull OWLAxiomSearchFilter filter,
@Nonnull Object key,
@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Override
public boolean contains(@Nonnull OWLAxiomSearchFilter filter,
@Nonnull Object key,
@Nonnull Imports includeImportsClosure) {
return false;
}
@Nonnull
@Override
public Set getAxioms(@Nonnull Class type,
@Nonnull Class extends OWLObject> explicitClass,
@Nonnull OWLObject entity,
@Nonnull Imports includeImports,
@Nonnull Navigation forSubPosition) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getSubAnnotationPropertyOfAxioms(@Nonnull OWLAnnotationProperty subProperty) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAnnotationPropertyDomainAxioms(@Nonnull OWLAnnotationProperty property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAnnotationPropertyRangeAxioms(@Nonnull OWLAnnotationProperty property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDeclarationAxioms(@Nonnull OWLEntity subject) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAnnotationAssertionAxioms(@Nonnull OWLAnnotationSubject entity) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getSubClassAxiomsForSubClass(@Nonnull OWLClass cls) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getSubClassAxiomsForSuperClass(@Nonnull OWLClass cls) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getEquivalentClassesAxioms(@Nonnull OWLClass cls) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDisjointClassesAxioms(@Nonnull OWLClass cls) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDisjointUnionAxioms(@Nonnull OWLClass owlClass) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getHasKeyAxioms(@Nonnull OWLClass cls) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getObjectSubPropertyAxiomsForSubProperty(@Nonnull OWLObjectPropertyExpression subProperty) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getObjectSubPropertyAxiomsForSuperProperty(@Nonnull OWLObjectPropertyExpression superProperty) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getObjectPropertyDomainAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getObjectPropertyRangeAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getInverseObjectPropertyAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getEquivalentObjectPropertiesAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDisjointObjectPropertiesAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getFunctionalObjectPropertyAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getInverseFunctionalObjectPropertyAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getSymmetricObjectPropertyAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAsymmetricObjectPropertyAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getReflexiveObjectPropertyAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getIrreflexiveObjectPropertyAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getTransitiveObjectPropertyAxioms(@Nonnull OWLObjectPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDataSubPropertyAxiomsForSubProperty(@Nonnull OWLDataProperty subProperty) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDataSubPropertyAxiomsForSuperProperty(@Nonnull OWLDataPropertyExpression superProperty) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDataPropertyDomainAxioms(@Nonnull OWLDataProperty property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDataPropertyRangeAxioms(@Nonnull OWLDataProperty property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getEquivalentDataPropertiesAxioms(@Nonnull OWLDataProperty property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDisjointDataPropertiesAxioms(@Nonnull OWLDataProperty property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getFunctionalDataPropertyAxioms(@Nonnull OWLDataPropertyExpression property) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getClassAssertionAxioms(@Nonnull OWLIndividual individual) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getClassAssertionAxioms(@Nonnull OWLClassExpression ce) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDataPropertyAssertionAxioms(@Nonnull OWLIndividual individual) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getObjectPropertyAssertionAxioms(@Nonnull OWLIndividual individual) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getNegativeObjectPropertyAssertionAxioms(@Nonnull OWLIndividual individual) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getNegativeDataPropertyAssertionAxioms(@Nonnull OWLIndividual individual) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getSameIndividualAxioms(@Nonnull OWLIndividual individual) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDifferentIndividualAxioms(@Nonnull OWLIndividual individual) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDatatypeDefinitions(@Nonnull OWLDatatype datatype) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getNestedClassExpressions() {
return Collections.emptySet();
}
@Override
public void accept(@Nonnull OWLObjectVisitor visitor) {
throw new RuntimeException("Cannot accept visits");
}
@Nonnull
@Override
public O accept(@Nonnull OWLObjectVisitorEx visitor) {
throw new RuntimeException("Cannot accept visits");
}
@Override
public boolean isTopEntity() {
return false;
}
@Override
public boolean isBottomEntity() {
return false;
}
@Override
public int compareTo(OWLObject o) {
return 0;
}
@Nonnull
@Override
public Set getAnnotationPropertiesInSignature() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAnonymousIndividuals() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getClassesInSignature(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getObjectPropertiesInSignature(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDataPropertiesInSignature(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getIndividualsInSignature(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getReferencedAnonymousIndividuals(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDatatypesInSignature(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAnnotationPropertiesInSignature(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Override
public boolean containsEntityInSignature(@Nonnull OWLEntity owlEntity, @Nonnull Imports includeImportsClosure) {
return false;
}
@Override
public boolean containsEntityInSignature(@Nonnull IRI entityIRI, @Nonnull Imports includeImportsClosure) {
return false;
}
@Override
public boolean containsClassInSignature(@Nonnull IRI owlClassIRI, @Nonnull Imports includeImportsClosure) {
return false;
}
@Override
public boolean containsObjectPropertyInSignature(@Nonnull IRI owlObjectPropertyIRI,
@Nonnull Imports includeImportsClosure) {
return false;
}
@Override
public boolean containsDataPropertyInSignature(@Nonnull IRI owlDataPropertyIRI,
@Nonnull Imports includeImportsClosure) {
return false;
}
@Override
public boolean containsAnnotationPropertyInSignature(@Nonnull IRI owlAnnotationPropertyIRI,
@Nonnull Imports includeImportsClosure) {
return false;
}
@Override
public boolean containsDatatypeInSignature(@Nonnull IRI owlDatatypeIRI, @Nonnull Imports includeImportsClosure) {
return false;
}
@Override
public boolean containsIndividualInSignature(@Nonnull IRI owlIndividualIRI,
@Nonnull Imports includeImportsClosure) {
return false;
}
@Override
public boolean containsDatatypeInSignature(@Nonnull IRI owlDatatypeIRI) {
return false;
}
@Override
public boolean containsEntityInSignature(@Nonnull IRI entityIRI) {
return false;
}
@Override
public boolean containsClassInSignature(@Nonnull IRI owlClassIRI) {
return false;
}
@Override
public boolean containsObjectPropertyInSignature(@Nonnull IRI owlObjectPropertyIRI) {
return false;
}
@Override
public boolean containsDataPropertyInSignature(@Nonnull IRI owlDataPropertyIRI) {
return false;
}
@Override
public boolean containsAnnotationPropertyInSignature(@Nonnull IRI owlAnnotationPropertyIRI) {
return false;
}
@Override
public boolean containsIndividualInSignature(@Nonnull IRI owlIndividualIRI) {
return false;
}
@Nonnull
@Override
public Set getEntitiesInSignature(@Nonnull IRI iri, @Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Override
public Set getPunnedIRIs(@Nonnull Imports includeImportsClosure) {
return Collections.emptySet();
}
@Override
public boolean containsReference(@Nonnull OWLEntity entity, @Nonnull Imports includeImportsClosure) {
return false;
}
@Override
public boolean containsReference(@Nonnull OWLEntity entity) {
return false;
}
@Nonnull
@Override
public Set getClassesInSignature(boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getObjectPropertiesInSignature(boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDataPropertiesInSignature(boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getIndividualsInSignature(boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getReferencedAnonymousIndividuals(boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDatatypesInSignature(boolean includeImportsClosure) {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getAnnotationPropertiesInSignature(boolean includeImportsClosure) {
return Collections.emptySet();
}
@Override
public boolean containsEntityInSignature(@Nonnull OWLEntity owlEntity, boolean includeImportsClosure) {
return false;
}
@Override
public boolean containsEntityInSignature(@Nonnull IRI entityIRI, boolean includeImportsClosure) {
return false;
}
@Override
public boolean containsClassInSignature(@Nonnull IRI owlClassIRI, boolean includeImportsClosure) {
return false;
}
@Override
public boolean containsObjectPropertyInSignature(@Nonnull IRI owlObjectPropertyIRI, boolean includeImportsClosure) {
return false;
}
@Override
public boolean containsDataPropertyInSignature(@Nonnull IRI owlDataPropertyIRI, boolean includeImportsClosure) {
return false;
}
@Override
public boolean containsAnnotationPropertyInSignature(@Nonnull IRI owlAnnotationPropertyIRI,
boolean includeImportsClosure) {
return false;
}
@Override
public boolean containsDatatypeInSignature(@Nonnull IRI owlDatatypeIRI, boolean includeImportsClosure) {
return false;
}
@Override
public boolean containsIndividualInSignature(@Nonnull IRI owlIndividualIRI, boolean includeImportsClosure) {
return false;
}
@Nonnull
@Override
public Set getEntitiesInSignature(@Nonnull IRI iri, boolean includeImportsClosure) {
return Collections.emptySet();
}
@Override
public boolean containsReference(@Nonnull OWLEntity entity, boolean includeImportsClosure) {
return false;
}
@Nonnull
@Override
public Set getClassesInSignature() {
return Collections.emptySet();
}
@Override
public boolean containsEntityInSignature(@Nonnull OWLEntity owlEntity) {
return false;
}
@Nonnull
@Override
public Set getDataPropertiesInSignature() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getDatatypesInSignature() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getIndividualsInSignature() {
return Collections.emptySet();
}
@Nonnull
@Override
public Set getObjectPropertiesInSignature() {
return Collections.emptySet();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy