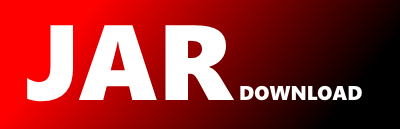
edu.stanford.protege.webprotege.match.LiteralAnnotationValueMatcher Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.match;
import org.semanticweb.owlapi.model.OWLAnnotationValue;
import org.semanticweb.owlapi.model.OWLLiteral;
import javax.annotation.Nonnull;
import java.util.function.Predicate;
import java.util.regex.Pattern;
import static com.google.common.base.Preconditions.checkNotNull;
/**
* Matthew Horridge
* Stanford Center for Biomedical Informatics Research
* 7 Jun 2018
*/
public class LiteralAnnotationValueMatcher implements Matcher {
@Nonnull
private final Matcher literalMatcher;
public LiteralAnnotationValueMatcher(@Nonnull Matcher literalMatcher) {
this.literalMatcher = checkNotNull(literalMatcher);
}
@Override
public boolean matches(@Nonnull OWLAnnotationValue value) {
return value instanceof OWLLiteral && literalMatcher.matches((OWLLiteral) value);
}
public static Matcher forLexicalPattern(@Nonnull Pattern pattern) {
return new LiteralAnnotationValueMatcher(LiteralMatcher.forLexicalPattern(pattern));
}
public static Matcher forLexicalPredicate(Predicate predicate) {
return new LiteralAnnotationValueMatcher(LiteralMatcher.forPredicate(predicate));
}
public static Matcher forHasAnyLangTag() {
return new LiteralAnnotationValueMatcher(LiteralMatcher.forHasAnyLangTag());
}
public static Matcher forIsXsdBooleanTrue() {
return new LiteralAnnotationValueMatcher(LiteralMatcher.forXsdBooleanTrue());
}
/**
* Returns a matcher for annotation values that matches if the datatype is an OWL 2 datatype
* and the lexical value is in the lexical space of the datatype.
*/
public static Matcher forIsNotInLexicalSpace() {
return new LiteralAnnotationValueMatcher(new NotMatcher<>(new LiteralInLexicalSpaceMatcher()));
}
public static Matcher forHasTrailingWhiteSpace() {
return forLexicalPattern(Pattern.compile("\\s+$"));
}
public static Matcher forHasRepeatedWhiteSpace() {
return forLexicalPattern(Pattern.compile("\\s+"));
}
public static Matcher forAnyValue() {
return OWLAnnotationValue::isLiteral;
}
public static Matcher forLangTagMatcher(Matcher langTagMatcher) {
return new LiteralAnnotationValueMatcher(new LiteralMatcher(
lex -> true,
langTagMatcher,
dt -> true
));
}
public static Matcher forLexicalValueMatcher(Matcher lexicalValueMatcher) {
return new LiteralAnnotationValueMatcher(
new LiteralMatcher(
lexicalValueMatcher,
langTag -> true,
dt -> true
)
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy