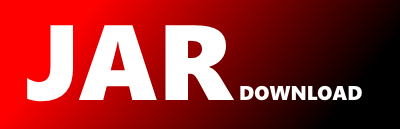
edu.stanford.protege.webprotege.match.MatchingEngineImpl Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.match;
import com.google.common.collect.ImmutableList;
import edu.stanford.protege.webprotege.index.ProjectSignatureIndex;
import edu.stanford.protege.webprotege.criteria.Criteria;
import edu.stanford.protege.webprotege.criteria.RootCriteria;
import org.semanticweb.owlapi.model.OWLEntity;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import java.util.stream.Stream;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.google.common.collect.ImmutableList.toImmutableList;
/**
* Matthew Horridge
* Stanford Center for Biomedical Informatics Research
* 19 Jun 2018
*/
public class MatchingEngineImpl implements MatchingEngine {
@Nonnull
private final ProjectSignatureIndex projectSignatureIndex;
@Nonnull
private final MatcherFactory matcherFactory;
@Inject
public MatchingEngineImpl(@Nonnull ProjectSignatureIndex projectSignatureIndex,
@Nonnull MatcherFactory matcherFactory) {
this.projectSignatureIndex = projectSignatureIndex;
this.matcherFactory = checkNotNull(matcherFactory);
}
@Override
public Stream match(@Nonnull Criteria criteria) {
Matcher matcher = getMatcher(criteria);
return projectSignatureIndex.getSignature()
.filter(matcher::matches);
}
@Override
public Stream matchAny(@Nonnull ImmutableList extends Criteria> criteria) {
ImmutableList> matchers = criteria.stream()
.map(this::getMatcher)
.collect(toImmutableList());
OrMatcher orMatcher = new OrMatcher<>(matchers);
return projectSignatureIndex.getSignature()
.filter(orMatcher::matches);
}
@Override
public boolean matches(@Nonnull OWLEntity entity, @Nonnull Criteria criteria) {
return getMatcher(criteria).matches(entity);
}
@Override
public boolean matchesAny(@Nonnull OWLEntity entity, @Nonnull ImmutableList extends Criteria> criteria) {
return criteria.stream()
.map(this::getMatcher)
.anyMatch(c -> c.matches(entity));
}
private Matcher getMatcher(@Nonnull Criteria rootCriteria) {
return matcherFactory.getMatcher((RootCriteria) rootCriteria);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy