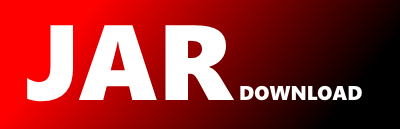
edu.stanford.protege.webprotege.owlapi.RenameMap Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.owlapi;
import edu.stanford.protege.webprotege.DataFactory;
import edu.stanford.protege.webprotege.entity.OWLEntityData;
import edu.stanford.protege.webprotege.renderer.RenderingManager;
import org.semanticweb.owlapi.model.IRI;
import org.semanticweb.owlapi.model.OWLDataFactory;
import org.semanticweb.owlapi.model.OWLEntity;
import javax.annotation.Nonnull;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import static com.google.common.base.Preconditions.checkNotNull;
/**
* Author: Matthew Horridge
* Stanford University
* Bio-Medical Informatics Research Group
* Date: 22/02/2013
*
* An object that records renamings of IRIs.
*
*/
public class RenameMap {
@Nonnull
private final OWLDataFactory dataFactory;
@Nonnull
private final RenderingManager renderingManager;
@Nonnull
private final Map map = new HashMap<>();
/**
* Constructs a {@link RenameMap} from the specified IRI map.
* @param map The map from which to construct this rename map. Not {@code null}.
* @param dataFactory
* @param renderingManager
* @throws NullPointerException if {@code map} is {@code null}.
*/
public RenameMap(@Nonnull Map map,
@Nonnull OWLDataFactory dataFactory,
@Nonnull RenderingManager renderingManager) {
this.dataFactory = dataFactory;
this.renderingManager = checkNotNull(renderingManager);
this.map.putAll(checkNotNull(map));
}
/**
* Gets renamed version of the specified entity.
* @param entity The entity.
* @param The entity type.
* @return An entity of the same type that is a renaming for the specified entity, or the specified entity if
* no renaming exists within this map. Not {@code null}.
*/
@SuppressWarnings("unchecked")
public E getRenamedEntity(E entity) {
IRI renamedIRI = map.get(entity.getEntity().getIRI());
if(renamedIRI == null) {
return entity;
}
OWLEntity renamedEntity = DataFactory.getOWLEntity(entity.getEntity().getEntityType(), renamedIRI);
return (E) renderingManager.getRendering(renamedEntity);
}
public E getRenamedEntity(E entity) {
IRI renamedIRI = map.get(entity.getIRI());
if(renamedIRI == null) {
return entity;
}
return (E) dataFactory.getOWLEntity(entity.getEntityType(), renamedIRI);
}
/**
* Gets a set containing renamed versions of the entities in the specified set.
* @param entities The set of entities. Not {@code null}.
* @param The entity type.
* @return A set of entities (of the same cardinality as the specified set) that contains renamed versions of the
* entities in the specfied set. Not {@code null}.
*/
public Set getRenamedEntityData(Set entities) {
Set result = new HashSet<>(entities.size());
for(E entity : entities) {
result.add(getRenamedEntity(entity));
}
return result;
}
public Set getRenamedEntities(Set entities) {
Set result = new HashSet<>(entities.size());
for(E entity : entities) {
result.add(getRenamedEntity(entity));
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy