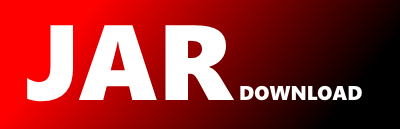
edu.stanford.protege.webprotege.trigger.TriggerRunner Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.trigger;
import com.google.common.collect.ImmutableList;
import edu.stanford.protege.webprotege.match.Matcher;
import org.semanticweb.owlapi.model.OWLEntity;
import javax.annotation.Nonnull;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Stream;
import static com.google.common.base.Preconditions.checkNotNull;
/**
* Matthew Horridge
* Stanford Center for Biomedical Informatics Research
* 7 Jun 2018
*/
public class TriggerRunner {
@Nonnull
private final ImmutableList triggers;
@Nonnull
private final List> contexts = new ArrayList<>();
public TriggerRunner(@Nonnull ImmutableList triggers) {
this.triggers = checkNotNull(triggers);
}
public void execute(@Nonnull Stream entities) {
checkNotNull(entities);
begin();
entities.forEach(this::execute);
end();
}
private void begin() {
contexts.clear();
triggers.forEach(trigger -> {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy