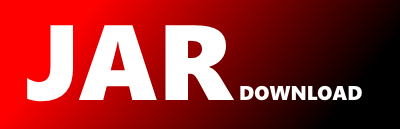
edu.stanford.protege.webprotege.util.ReferenceFinder Maven / Gradle / Ivy
The newest version!
package edu.stanford.protege.webprotege.util;
import com.google.common.collect.ImmutableCollection;
import com.google.common.collect.ImmutableSet;
import edu.stanford.protege.webprotege.index.AxiomsByReferenceIndex;
import edu.stanford.protege.webprotege.index.OntologyAnnotationsIndex;
import org.semanticweb.owlapi.model.*;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
import static com.google.common.base.Preconditions.checkNotNull;
/**
* Matthew Horridge
* Stanford Center for Biomedical Informatics Research
* 2019-08-06
*/
public class ReferenceFinder {
@Nonnull
private final AxiomsByReferenceIndex axiomsIndex;
@Nonnull
private final OntologyAnnotationsIndex ontologyAnnotationsIndex;
@Inject
public ReferenceFinder(@Nonnull AxiomsByReferenceIndex axiomsIndex,
@Nonnull OntologyAnnotationsIndex ontologyAnnotationsIndex) {
this.axiomsIndex = checkNotNull(axiomsIndex);
this.ontologyAnnotationsIndex = checkNotNull(ontologyAnnotationsIndex);
}
/**
* Gets the references set for the specified entities in the specified ontology.
*
* @param entities The entities whose references are to be retrieved. Not {@code null}.
* @param ontologyId The ontology. Not {@code null}.
* @return The ReferenceSet that contains axiomsSource that reference the specified entities and ontology annotations
* that reference the specified entities. Note that, since annotation assertions have subjects that may be IRIs and
* values that may be IRIs, and ontology annotation have values that may be IRIs, the reference set includes these
* axiomsSource where the IRI is the IRI of one or more of the specified entities.
*/
public ReferenceSet getReferenceSet(@Nonnull Collection entities,
@Nonnull OWLOntologyID ontologyId) {
checkNotNull(entities);
checkNotNull(ontologyId);
ImmutableSet.Builder axiomSetBuilder = ImmutableSet.builder();
ImmutableSet.Builder ontologyAnnotationSetBuilder = ImmutableSet.builder();
final Set entityIRIs = new HashSet<>(entities.size());
axiomsIndex.getReferencingAxioms(entities, ontologyId)
.forEach(axiomSetBuilder::add);
entities.stream().map(OWLNamedObject::getIRI).forEach(entityIRIs::add);
ontologyAnnotationsIndex.getOntologyAnnotations(ontologyId)
.forEach(annotation -> {
processAnnotation(entities, ontologyAnnotationSetBuilder, entityIRIs, annotation, annotation);
});
return new ReferenceSet(ontologyId, axiomSetBuilder.build(), ontologyAnnotationSetBuilder.build());
}
private void processAnnotation(@Nonnull Collection entities,
ImmutableSet.Builder ontologyAnnotationSetBuilder,
Set entityIRIs,
OWLAnnotation rootAnnotation,
OWLAnnotation annotation) {
var value = annotation.getValue();
if(value instanceof IRI && entityIRIs.contains(value)) {
ontologyAnnotationSetBuilder.add(rootAnnotation);
}
else {
if(entities.contains(annotation.getProperty())) {
ontologyAnnotationSetBuilder.add(rootAnnotation);
}
}
annotation.getAnnotations().forEach(annoAnno -> processAnnotation(entities,
ontologyAnnotationSetBuilder,
entityIRIs,
rootAnnotation,
annoAnno));
}
public static class ReferenceSet {
private final OWLOntologyID ontologyId;
private final ImmutableCollection referencingAxioms;
private final ImmutableCollection referencingOntologyAnnotations;
public ReferenceSet(OWLOntologyID ontologyId,
ImmutableCollection referencingAxioms,
ImmutableCollection referencingOntologyAnnotations) {
this.ontologyId = checkNotNull(ontologyId);
this.referencingAxioms = checkNotNull(referencingAxioms);
this.referencingOntologyAnnotations = checkNotNull(referencingOntologyAnnotations);
}
public OWLOntologyID getOntologyId() {
return ontologyId;
}
public ImmutableCollection getReferencingAxioms() {
return referencingAxioms;
}
public ImmutableCollection getReferencingOntologyAnnotations() {
return referencingOntologyAnnotations;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy