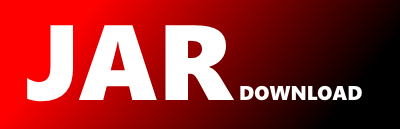
thredds.catalog.dl.DCWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdm Show documentation
Show all versions of cdm Show documentation
The NetCDF-Java Library is a Java interface to NetCDF files,
as well as to many other types of scientific data formats.
The newest version!
// $Id: DCWriter.java 48 2006-07-12 16:15:40Z caron $
/*
* Copyright 1998-2009 University Corporation for Atmospheric Research/Unidata
*
* Portions of this software were developed by the Unidata Program at the
* University Corporation for Atmospheric Research.
*
* Access and use of this software shall impose the following obligations
* and understandings on the user. The user is granted the right, without
* any fee or cost, to use, copy, modify, alter, enhance and distribute
* this software, and any derivative works thereof, and its supporting
* documentation for any purpose whatsoever, provided that this entire
* notice appears in all copies of the software, derivative works and
* supporting documentation. Further, UCAR requests that the user credit
* UCAR/Unidata in any publications that result from the use of this
* software or in any product that includes this software. The names UCAR
* and/or Unidata, however, may not be used in any advertising or publicity
* to endorse or promote any products or commercial entity unless specific
* written permission is obtained from UCAR/Unidata. The user also
* understands that UCAR/Unidata is not obligated to provide the user with
* any support, consulting, training or assistance of any kind with regard
* to the use, operation and performance of this software nor to provide
* the user with any updates, revisions, new versions or "bug fixes."
*
* THIS SOFTWARE IS PROVIDED BY UCAR/UNIDATA "AS IS" AND ANY EXPRESS OR
* IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL UCAR/UNIDATA BE LIABLE FOR ANY SPECIAL,
* INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING
* FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT,
* NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION
* WITH THE ACCESS, USE OR PERFORMANCE OF THIS SOFTWARE.
*/
package thredds.catalog.dl;
import thredds.catalog.*;
import ucar.nc2.time.CalendarDateRange;
import ucar.nc2.units.DateType;
import org.jdom2.*;
import org.jdom2.output.*;
import java.io.*;
import java.util.*;
public class DCWriter {
private static final Namespace defNS = Namespace.getNamespace("http://purl.org/dc/elements/1.1/");
private static final String schemaLocation = defNS.getURI() + " http://www.unidata.ucar.edu/schemas/other/dc/dc.xsd";
private static final String threddsServerURL = "http://localhost:8080/thredds/subset.html";
private InvCatalog cat;
public DCWriter( InvCatalog cat) {
this.cat = cat;
}
public void writeItems( String fileDir) {
File dir = new File(fileDir);
if (!dir.exists()) {
boolean ret = dir.mkdirs();
assert ret;
}
for (InvDataset dataset : cat.getDatasets()) {
doDataset(dataset, fileDir);
}
}
private void doDataset( InvDataset ds, String fileDir) {
if (ds.isHarvest() && (ds.getID() != null)) {
String fileOutName = fileDir+"/"+ds.getID()+".dc.xml";
try ( OutputStream out = new BufferedOutputStream(new FileOutputStream(fileOutName))) {
writeOneItem(ds, System.out);
writeOneItem(ds, out);
return;
} catch (IOException ioe) {
ioe.printStackTrace();
}
}
for (InvDataset nested : ds.getDatasets()) {
doDataset( nested, fileDir);
}
}
private void writeOneItem( InvDataset ds, OutputStream out) throws IOException {
Element rootElem = new Element("dc", defNS);
Document doc = new Document(rootElem);
writeDataset( ds, rootElem);
rootElem.addNamespaceDeclaration(XMLEntityResolver.xsiNS);
// rootElem.setAttribute("schemaLocation", schemaLocation, XMLEntityResolver.xsiNS);
rootElem.setAttribute("schemaLocation", defNS.getURI()+" "+schemaLocation, XMLEntityResolver.xsiNS);
// Output the document, use standard formatter
//XMLOutputter fmt = new XMLOutputter(" ", true);
XMLOutputter fmt = new XMLOutputter( Format.getPrettyFormat());
fmt.output( doc, out);
}
/*
*/
public void writeDataset(InvDataset ds, Element rootElem) {
rootElem.addContent( new Element("title", defNS).addContent(ds.getName()));
rootElem.addContent( new Element("Entry_ID", defNS).addContent(ds.getUniqueID()));
// keywords
List list = ds.getKeywords();
if (list.size() > 0) {
for (ThreddsMetadata.Vocab k : list) {
rootElem.addContent( new Element("Keyword", defNS).addContent( k.getText()));
}
}
//temporal
CalendarDateRange tm = ds.getCalendarDateCoverage();
Element tmElem = new Element("Temporal_Coverage", defNS);
rootElem.addContent( tmElem);
tmElem.addContent( new Element("Start_Date", defNS).addContent( tm.getStart().toString()));
tmElem.addContent( new Element("End_Date", defNS).addContent( tm.getEnd().toString()));
//geospatial
ThreddsMetadata.GeospatialCoverage geo = ds.getGeospatialCoverage();
Element geoElem = new Element("Spatial_Coverage", defNS);
rootElem.addContent( geoElem);
geoElem.addContent( new Element("Southernmost_Latitude", defNS).addContent( Double.toString(geo.getLatSouth())));
geoElem.addContent( new Element("Northernmost_Latitude", defNS).addContent(Double.toString(geo.getLatNorth())));
geoElem.addContent( new Element("Westernmost_Latitude", defNS).addContent(Double.toString(geo.getLonWest())));
geoElem.addContent( new Element("Easternmost_Latitude", defNS).addContent(Double.toString(geo.getLonEast())));
rootElem.addContent( new Element("Use_Constraints", defNS).addContent(ds.getDocumentation("rights")));
// data center
List slist = ds.getPublishers();
if (list.size() > 0) {
for ( ThreddsMetadata.Source p : slist) {
Element dataCenter = new Element("Data_Center", defNS);
rootElem.addContent( dataCenter);
writePublisher(p, dataCenter);
}
}
rootElem.addContent( new Element("Summary", defNS).addContent(ds.getDocumentation("summary")));
Element primaryURLelem = new Element("Related_URL", defNS);
rootElem.addContent( primaryURLelem);
String primaryURL = threddsServerURL +
"?catalog="+((InvCatalogImpl)ds.getParentCatalog()).getBaseURI().toString() +
"&dataset="+ds.getID();
primaryURLelem.addContent( new Element("URL_Content_Type", defNS).addContent("THREDDS access page"));
primaryURLelem.addContent( new Element("URL", defNS).addContent(primaryURL));
DateType today = new DateType(false, new Date());
rootElem.addContent(new Element("DIF_Creation_Date", defNS).addContent(today.toDateTimeStringISO()));
}
protected void writePublisher(ThreddsMetadata.Source p, Element dataCenter) {
Element name = new Element("Data_Center_Name", defNS);
dataCenter.addContent( name);
name.addContent( new Element("Short_Name", defNS).addContent(p.getName()));
//name.addContent( new Element("Long_Name", defNS).addContent(p.getLongName()));
if ((p.getUrl() != null) && p.getUrl().length() > 0)
dataCenter.addContent( new Element("Data_Center_URL", defNS).addContent(p.getUrl()));
Element person = new Element("Personnel", defNS);
dataCenter.addContent( person);
person.addContent( new Element("Role", defNS).addContent("DATA CENTER CONTACT"));
person.addContent( new Element("Email", defNS).addContent(p.getEmail()));
}
private void writeVariable( Element param, ThreddsMetadata.Variable v) {
String vname = v.getVocabularyName();
StringTokenizer stoker = new StringTokenizer(vname,">");
if (stoker.hasMoreTokens())
param.addContent( new Element("Category", defNS).addContent(stoker.nextToken().trim()));
if (stoker.hasMoreTokens())
param.addContent( new Element("Topic", defNS).addContent(stoker.nextToken().trim()));
if (stoker.hasMoreTokens())
param.addContent( new Element("Term", defNS).addContent(stoker.nextToken().trim()));
if (stoker.hasMoreTokens())
param.addContent( new Element("Variable", defNS).addContent(stoker.nextToken().trim()));
if (stoker.hasMoreTokens())
param.addContent( new Element("Detailed_Variable", defNS).addContent(stoker.nextToken().trim()));
}
// test
private static void doOne( InvCatalogFactory fac, String url) {
System.out.println("***read "+url);
try {
InvCatalogImpl cat = fac.readXML(url);
StringBuilder buff = new StringBuilder();
boolean isValid = cat.check( buff, false);
System.out.println("catalog <" + cat.getName()+ "> "+ (isValid ? "is" : "is not") + " valid");
System.out.println(" validation output=\n" + buff);
// System.out.println(" catalog=\n" + fac.writeXML(cat));
DCWriter w = new DCWriter( cat);
w.writeItems( "C:/temp/dif");
} catch (Exception e) {
e.printStackTrace();
}
}
/** testing */
public static void main (String[] args) throws Exception {
InvCatalogFactory catFactory = InvCatalogFactory.getDefaultFactory(true);
doOne(catFactory, "file:///C:/dev/thredds/catalog/test/data/TestHarvest.xml");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy