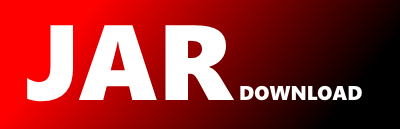
thredds.inventory.MCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdm Show documentation
Show all versions of cdm Show documentation
The NetCDF-Java Library is a Java interface to NetCDF files,
as well as to many other types of scientific data formats.
The newest version!
package thredds.inventory;
import ucar.nc2.time.CalendarDate;
import ucar.nc2.util.CloseableIterator;
import java.io.IOException;
import java.util.List;
/**
* Collection of MFiles or Partitions
*
* @author caron
* @since 11/11/13
*/
public interface MCollection extends AutoCloseable {
/**
* Consider MCollections as a tree. At the leaves are a single mfile or mfile collection.
* A leaf MCollection will be a GC if it has a single runtime, or a PofGC otherwise.
* A non-leaf is a directory with other directories under it. It will either be a PofGC (Partition of GC) or PofP (Partition of Partition).
* @return if leaf collection
*/
public boolean isLeaf();
/**
* The name of the collection
* @return name of the collection
*/
public String getCollectionName();
/**
* Get common root directory of all MFiles in the collection - may be null
*
* @return root directory name, or null.
*/
public String getRoot();
/**
* Use the date extractor to extract the date from the filename.
* Only call if hasDateExtractor() == true.
*
* @param mfile extract from here
* @return Date, or null if none
*/
public CalendarDate extractDate(MFile mfile);
/**
* Does this CollectionManager have the ability to extract a date from the MFile ?
* @return true if CollectionManager has a DateExtractor
*/
public boolean hasDateExtractor();
/**
* The starting date of the collection.
* Only call if hasDateExtractor() == true.
* @return starting date of the collection
*/
public CalendarDate getStartCollection();
/**
* Close and release any resources. Do not make further calls on this object.
*/
public void close();
/**
* Choose Proto dataset as index from [0..n-1], based on configuration.
* @param n size to choose from
* @return index within range [0..n-1]
*/
public int getProtoIndex(int n);
/**
* last time this collection was modified
* @return msess since epoch
*/
public long getLastModified();
// not in cache
public String getIndexFilename();
//////////////////////////////////////////////////////////////////////////////////////
/**
* Get the current collection of MFile.
* if hasDateExtractor() == true, these will be sorted by Date, otherwise by path.
*
* @return current collection of MFile as an Iterable.
*/
public Iterable getFilesSorted() throws IOException;
/**
* Sorted filename
* @return Sorted filename
* @throws IOException
*/
public List getFilenames() throws IOException;
/**
* The latest file in the collection.
* Only call if hasDateExtractor() == true.
* @return latest file in the collection
*/
public MFile getLatestFile() throws IOException;
/**
* Get the current collection of MFile, no guaranteed order.
* May be faster for large collections, use when order is not important.
*
try (CloseableIterator iter = getFileIterator()) {
while (iter.hasNext()) {
MFile file = iter.next();
}
}
*
* @return current collection of MFile as an CloseableIterator.
*/
public CloseableIterator getFileIterator() throws IOException;
////////////////////////////////////////////////////
// ability to pass arbitrary information to users of the collection manager.
public Object getAuxInfo(String key);
public void putAuxInfo(String key, Object value);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy