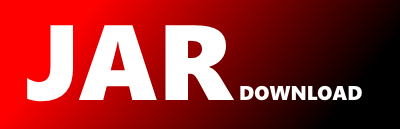
ucar.nc2.stream.NcStreamProto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdm Show documentation
Show all versions of cdm Show documentation
The NetCDF-Java Library is a Java interface to NetCDF files,
as well as to many other types of scientific data formats.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ucar/nc2/stream/ncStream.proto
package ucar.nc2.stream;
public final class NcStreamProto {
private NcStreamProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
/**
* Protobuf enum {@code ncstream.DataType}
*/
public enum DataType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* CHAR = 0;
*/
CHAR(0, 0),
/**
* BYTE = 1;
*/
BYTE(1, 1),
/**
* SHORT = 2;
*/
SHORT(2, 2),
/**
* INT = 3;
*/
INT(3, 3),
/**
* LONG = 4;
*/
LONG(4, 4),
/**
* FLOAT = 5;
*/
FLOAT(5, 5),
/**
* DOUBLE = 6;
*/
DOUBLE(6, 6),
/**
* STRING = 7;
*/
STRING(7, 7),
/**
* STRUCTURE = 8;
*/
STRUCTURE(8, 8),
/**
* SEQUENCE = 9;
*/
SEQUENCE(9, 9),
/**
* ENUM1 = 10;
*/
ENUM1(10, 10),
/**
* ENUM2 = 11;
*/
ENUM2(11, 11),
/**
* ENUM4 = 12;
*/
ENUM4(12, 12),
/**
* OPAQUE = 13;
*/
OPAQUE(13, 13),
;
/**
* CHAR = 0;
*/
public static final int CHAR_VALUE = 0;
/**
* BYTE = 1;
*/
public static final int BYTE_VALUE = 1;
/**
* SHORT = 2;
*/
public static final int SHORT_VALUE = 2;
/**
* INT = 3;
*/
public static final int INT_VALUE = 3;
/**
* LONG = 4;
*/
public static final int LONG_VALUE = 4;
/**
* FLOAT = 5;
*/
public static final int FLOAT_VALUE = 5;
/**
* DOUBLE = 6;
*/
public static final int DOUBLE_VALUE = 6;
/**
* STRING = 7;
*/
public static final int STRING_VALUE = 7;
/**
* STRUCTURE = 8;
*/
public static final int STRUCTURE_VALUE = 8;
/**
* SEQUENCE = 9;
*/
public static final int SEQUENCE_VALUE = 9;
/**
* ENUM1 = 10;
*/
public static final int ENUM1_VALUE = 10;
/**
* ENUM2 = 11;
*/
public static final int ENUM2_VALUE = 11;
/**
* ENUM4 = 12;
*/
public static final int ENUM4_VALUE = 12;
/**
* OPAQUE = 13;
*/
public static final int OPAQUE_VALUE = 13;
public final int getNumber() { return value; }
public static DataType valueOf(int value) {
switch (value) {
case 0: return CHAR;
case 1: return BYTE;
case 2: return SHORT;
case 3: return INT;
case 4: return LONG;
case 5: return FLOAT;
case 6: return DOUBLE;
case 7: return STRING;
case 8: return STRUCTURE;
case 9: return SEQUENCE;
case 10: return ENUM1;
case 11: return ENUM2;
case 12: return ENUM4;
case 13: return OPAQUE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DataType findValueByNumber(int number) {
return DataType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.getDescriptor().getEnumTypes().get(0);
}
private static final DataType[] VALUES = values();
public static DataType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private DataType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:ncstream.DataType)
}
/**
* Protobuf enum {@code ncstream.Compress}
*/
public enum Compress
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NONE = 0;
*/
NONE(0, 0),
/**
* DEFLATE = 1;
*/
DEFLATE(1, 1),
;
/**
* NONE = 0;
*/
public static final int NONE_VALUE = 0;
/**
* DEFLATE = 1;
*/
public static final int DEFLATE_VALUE = 1;
public final int getNumber() { return value; }
public static Compress valueOf(int value) {
switch (value) {
case 0: return NONE;
case 1: return DEFLATE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Compress findValueByNumber(int number) {
return Compress.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.getDescriptor().getEnumTypes().get(1);
}
private static final Compress[] VALUES = values();
public static Compress valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private Compress(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:ncstream.Compress)
}
public interface AttributeOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
/**
* required string name = 1;
*/
boolean hasName();
/**
* required string name = 1;
*/
java.lang.String getName();
/**
* required string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
// required .ncstream.Attribute.Type type = 2;
/**
* required .ncstream.Attribute.Type type = 2;
*/
boolean hasType();
/**
* required .ncstream.Attribute.Type type = 2;
*/
ucar.nc2.stream.NcStreamProto.Attribute.Type getType();
// required uint32 len = 3;
/**
* required uint32 len = 3;
*/
boolean hasLen();
/**
* required uint32 len = 3;
*/
int getLen();
// optional bytes data = 4;
/**
* optional bytes data = 4;
*
*
* not needed if len == 0
*
*/
boolean hasData();
/**
* optional bytes data = 4;
*
*
* not needed if len == 0
*
*/
com.google.protobuf.ByteString getData();
// repeated string sdata = 5;
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
java.util.List
getSdataList();
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
int getSdataCount();
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
java.lang.String getSdata(int index);
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
com.google.protobuf.ByteString
getSdataBytes(int index);
// optional bool unsigned = 6 [default = false];
/**
* optional bool unsigned = 6 [default = false];
*/
boolean hasUnsigned();
/**
* optional bool unsigned = 6 [default = false];
*/
boolean getUnsigned();
}
/**
* Protobuf type {@code ncstream.Attribute}
*/
public static final class Attribute extends
com.google.protobuf.GeneratedMessage
implements AttributeOrBuilder {
// Use Attribute.newBuilder() to construct.
private Attribute(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Attribute(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Attribute defaultInstance;
public static Attribute getDefaultInstance() {
return defaultInstance;
}
public Attribute getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Attribute(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
ucar.nc2.stream.NcStreamProto.Attribute.Type value = ucar.nc2.stream.NcStreamProto.Attribute.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
type_ = value;
}
break;
}
case 24: {
bitField0_ |= 0x00000004;
len_ = input.readUInt32();
break;
}
case 34: {
bitField0_ |= 0x00000008;
data_ = input.readBytes();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
sdata_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000010;
}
sdata_.add(input.readBytes());
break;
}
case 48: {
bitField0_ |= 0x00000010;
unsigned_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
sdata_ = new com.google.protobuf.UnmodifiableLazyStringList(sdata_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Attribute_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Attribute_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Attribute.class, ucar.nc2.stream.NcStreamProto.Attribute.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Attribute parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Attribute(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code ncstream.Attribute.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* STRING = 0;
*/
STRING(0, 0),
/**
* BYTE = 1;
*/
BYTE(1, 1),
/**
* SHORT = 2;
*/
SHORT(2, 2),
/**
* INT = 3;
*/
INT(3, 3),
/**
* LONG = 4;
*/
LONG(4, 4),
/**
* FLOAT = 5;
*/
FLOAT(5, 5),
/**
* DOUBLE = 6;
*/
DOUBLE(6, 6),
;
/**
* STRING = 0;
*/
public static final int STRING_VALUE = 0;
/**
* BYTE = 1;
*/
public static final int BYTE_VALUE = 1;
/**
* SHORT = 2;
*/
public static final int SHORT_VALUE = 2;
/**
* INT = 3;
*/
public static final int INT_VALUE = 3;
/**
* LONG = 4;
*/
public static final int LONG_VALUE = 4;
/**
* FLOAT = 5;
*/
public static final int FLOAT_VALUE = 5;
/**
* DOUBLE = 6;
*/
public static final int DOUBLE_VALUE = 6;
public final int getNumber() { return value; }
public static Type valueOf(int value) {
switch (value) {
case 0: return STRING;
case 1: return BYTE;
case 2: return SHORT;
case 3: return INT;
case 4: return LONG;
case 5: return FLOAT;
case 6: return DOUBLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.Attribute.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private Type(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:ncstream.Attribute.Type)
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required .ncstream.Attribute.Type type = 2;
public static final int TYPE_FIELD_NUMBER = 2;
private ucar.nc2.stream.NcStreamProto.Attribute.Type type_;
/**
* required .ncstream.Attribute.Type type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .ncstream.Attribute.Type type = 2;
*/
public ucar.nc2.stream.NcStreamProto.Attribute.Type getType() {
return type_;
}
// required uint32 len = 3;
public static final int LEN_FIELD_NUMBER = 3;
private int len_;
/**
* required uint32 len = 3;
*/
public boolean hasLen() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required uint32 len = 3;
*/
public int getLen() {
return len_;
}
// optional bytes data = 4;
public static final int DATA_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString data_;
/**
* optional bytes data = 4;
*
*
* not needed if len == 0
*
*/
public boolean hasData() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes data = 4;
*
*
* not needed if len == 0
*
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
// repeated string sdata = 5;
public static final int SDATA_FIELD_NUMBER = 5;
private com.google.protobuf.LazyStringList sdata_;
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public java.util.List
getSdataList() {
return sdata_;
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public int getSdataCount() {
return sdata_.size();
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public java.lang.String getSdata(int index) {
return sdata_.get(index);
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public com.google.protobuf.ByteString
getSdataBytes(int index) {
return sdata_.getByteString(index);
}
// optional bool unsigned = 6 [default = false];
public static final int UNSIGNED_FIELD_NUMBER = 6;
private boolean unsigned_;
/**
* optional bool unsigned = 6 [default = false];
*/
public boolean hasUnsigned() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool unsigned = 6 [default = false];
*/
public boolean getUnsigned() {
return unsigned_;
}
private void initFields() {
name_ = "";
type_ = ucar.nc2.stream.NcStreamProto.Attribute.Type.STRING;
len_ = 0;
data_ = com.google.protobuf.ByteString.EMPTY;
sdata_ = com.google.protobuf.LazyStringArrayList.EMPTY;
unsigned_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasLen()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, type_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt32(3, len_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, data_);
}
for (int i = 0; i < sdata_.size(); i++) {
output.writeBytes(5, sdata_.getByteString(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(6, unsigned_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, len_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, data_);
}
{
int dataSize = 0;
for (int i = 0; i < sdata_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(sdata_.getByteString(i));
}
size += dataSize;
size += 1 * getSdataList().size();
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, unsigned_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Attribute prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.Attribute}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.AttributeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Attribute_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Attribute_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Attribute.class, ucar.nc2.stream.NcStreamProto.Attribute.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.Attribute.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
type_ = ucar.nc2.stream.NcStreamProto.Attribute.Type.STRING;
bitField0_ = (bitField0_ & ~0x00000002);
len_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
data_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
sdata_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
unsigned_ = false;
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Attribute_descriptor;
}
public ucar.nc2.stream.NcStreamProto.Attribute getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Attribute.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.Attribute build() {
ucar.nc2.stream.NcStreamProto.Attribute result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.Attribute buildPartial() {
ucar.nc2.stream.NcStreamProto.Attribute result = new ucar.nc2.stream.NcStreamProto.Attribute(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.len_ = len_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.data_ = data_;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
sdata_ = new com.google.protobuf.UnmodifiableLazyStringList(
sdata_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.sdata_ = sdata_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000010;
}
result.unsigned_ = unsigned_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Attribute) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Attribute)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Attribute other) {
if (other == ucar.nc2.stream.NcStreamProto.Attribute.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasLen()) {
setLen(other.getLen());
}
if (other.hasData()) {
setData(other.getData());
}
if (!other.sdata_.isEmpty()) {
if (sdata_.isEmpty()) {
sdata_ = other.sdata_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureSdataIsMutable();
sdata_.addAll(other.sdata_);
}
onChanged();
}
if (other.hasUnsigned()) {
setUnsigned(other.getUnsigned());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasType()) {
return false;
}
if (!hasLen()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.Attribute parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.Attribute) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// required .ncstream.Attribute.Type type = 2;
private ucar.nc2.stream.NcStreamProto.Attribute.Type type_ = ucar.nc2.stream.NcStreamProto.Attribute.Type.STRING;
/**
* required .ncstream.Attribute.Type type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .ncstream.Attribute.Type type = 2;
*/
public ucar.nc2.stream.NcStreamProto.Attribute.Type getType() {
return type_;
}
/**
* required .ncstream.Attribute.Type type = 2;
*/
public Builder setType(ucar.nc2.stream.NcStreamProto.Attribute.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* required .ncstream.Attribute.Type type = 2;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = ucar.nc2.stream.NcStreamProto.Attribute.Type.STRING;
onChanged();
return this;
}
// required uint32 len = 3;
private int len_ ;
/**
* required uint32 len = 3;
*/
public boolean hasLen() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required uint32 len = 3;
*/
public int getLen() {
return len_;
}
/**
* required uint32 len = 3;
*/
public Builder setLen(int value) {
bitField0_ |= 0x00000004;
len_ = value;
onChanged();
return this;
}
/**
* required uint32 len = 3;
*/
public Builder clearLen() {
bitField0_ = (bitField0_ & ~0x00000004);
len_ = 0;
onChanged();
return this;
}
// optional bytes data = 4;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes data = 4;
*
*
* not needed if len == 0
*
*/
public boolean hasData() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes data = 4;
*
*
* not needed if len == 0
*
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* optional bytes data = 4;
*
*
* not needed if len == 0
*
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
data_ = value;
onChanged();
return this;
}
/**
* optional bytes data = 4;
*
*
* not needed if len == 0
*
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000008);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
// repeated string sdata = 5;
private com.google.protobuf.LazyStringList sdata_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSdataIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
sdata_ = new com.google.protobuf.LazyStringArrayList(sdata_);
bitField0_ |= 0x00000010;
}
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public java.util.List
getSdataList() {
return java.util.Collections.unmodifiableList(sdata_);
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public int getSdataCount() {
return sdata_.size();
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public java.lang.String getSdata(int index) {
return sdata_.get(index);
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public com.google.protobuf.ByteString
getSdataBytes(int index) {
return sdata_.getByteString(index);
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public Builder setSdata(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSdataIsMutable();
sdata_.set(index, value);
onChanged();
return this;
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public Builder addSdata(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSdataIsMutable();
sdata_.add(value);
onChanged();
return this;
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public Builder addAllSdata(
java.lang.Iterable values) {
ensureSdataIsMutable();
super.addAll(values, sdata_);
onChanged();
return this;
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public Builder clearSdata() {
sdata_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* repeated string sdata = 5;
*
*
* used for string data
*
*/
public Builder addSdataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSdataIsMutable();
sdata_.add(value);
onChanged();
return this;
}
// optional bool unsigned = 6 [default = false];
private boolean unsigned_ ;
/**
* optional bool unsigned = 6 [default = false];
*/
public boolean hasUnsigned() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bool unsigned = 6 [default = false];
*/
public boolean getUnsigned() {
return unsigned_;
}
/**
* optional bool unsigned = 6 [default = false];
*/
public Builder setUnsigned(boolean value) {
bitField0_ |= 0x00000020;
unsigned_ = value;
onChanged();
return this;
}
/**
* optional bool unsigned = 6 [default = false];
*/
public Builder clearUnsigned() {
bitField0_ = (bitField0_ & ~0x00000020);
unsigned_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Attribute)
}
static {
defaultInstance = new Attribute(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Attribute)
}
public interface DimensionOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string name = 1;
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
boolean hasName();
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
java.lang.String getName();
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
com.google.protobuf.ByteString
getNameBytes();
// optional uint64 length = 2;
/**
* optional uint64 length = 2;
*
*
* optional when vlen
*
*/
boolean hasLength();
/**
* optional uint64 length = 2;
*
*
* optional when vlen
*
*/
long getLength();
// optional bool isUnlimited = 3 [default = false];
/**
* optional bool isUnlimited = 3 [default = false];
*
*
* is this needed ??
*
*/
boolean hasIsUnlimited();
/**
* optional bool isUnlimited = 3 [default = false];
*
*
* is this needed ??
*
*/
boolean getIsUnlimited();
// optional bool isVlen = 4 [default = false];
/**
* optional bool isVlen = 4 [default = false];
*/
boolean hasIsVlen();
/**
* optional bool isVlen = 4 [default = false];
*/
boolean getIsVlen();
// optional bool isPrivate = 5 [default = false];
/**
* optional bool isPrivate = 5 [default = false];
*/
boolean hasIsPrivate();
/**
* optional bool isPrivate = 5 [default = false];
*/
boolean getIsPrivate();
}
/**
* Protobuf type {@code ncstream.Dimension}
*/
public static final class Dimension extends
com.google.protobuf.GeneratedMessage
implements DimensionOrBuilder {
// Use Dimension.newBuilder() to construct.
private Dimension(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Dimension(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Dimension defaultInstance;
public static Dimension getDefaultInstance() {
return defaultInstance;
}
public Dimension getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Dimension(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
length_ = input.readUInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
isUnlimited_ = input.readBool();
break;
}
case 32: {
bitField0_ |= 0x00000008;
isVlen_ = input.readBool();
break;
}
case 40: {
bitField0_ |= 0x00000010;
isPrivate_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Dimension_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Dimension_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Dimension.class, ucar.nc2.stream.NcStreamProto.Dimension.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Dimension parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Dimension(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional uint64 length = 2;
public static final int LENGTH_FIELD_NUMBER = 2;
private long length_;
/**
* optional uint64 length = 2;
*
*
* optional when vlen
*
*/
public boolean hasLength() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint64 length = 2;
*
*
* optional when vlen
*
*/
public long getLength() {
return length_;
}
// optional bool isUnlimited = 3 [default = false];
public static final int ISUNLIMITED_FIELD_NUMBER = 3;
private boolean isUnlimited_;
/**
* optional bool isUnlimited = 3 [default = false];
*
*
* is this needed ??
*
*/
public boolean hasIsUnlimited() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool isUnlimited = 3 [default = false];
*
*
* is this needed ??
*
*/
public boolean getIsUnlimited() {
return isUnlimited_;
}
// optional bool isVlen = 4 [default = false];
public static final int ISVLEN_FIELD_NUMBER = 4;
private boolean isVlen_;
/**
* optional bool isVlen = 4 [default = false];
*/
public boolean hasIsVlen() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool isVlen = 4 [default = false];
*/
public boolean getIsVlen() {
return isVlen_;
}
// optional bool isPrivate = 5 [default = false];
public static final int ISPRIVATE_FIELD_NUMBER = 5;
private boolean isPrivate_;
/**
* optional bool isPrivate = 5 [default = false];
*/
public boolean hasIsPrivate() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool isPrivate = 5 [default = false];
*/
public boolean getIsPrivate() {
return isPrivate_;
}
private void initFields() {
name_ = "";
length_ = 0L;
isUnlimited_ = false;
isVlen_ = false;
isPrivate_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt64(2, length_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBool(3, isUnlimited_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(4, isVlen_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(5, isPrivate_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, length_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, isUnlimited_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, isVlen_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, isPrivate_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Dimension prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.Dimension}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.DimensionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Dimension_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Dimension_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Dimension.class, ucar.nc2.stream.NcStreamProto.Dimension.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.Dimension.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
length_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
isUnlimited_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
isVlen_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
isPrivate_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Dimension_descriptor;
}
public ucar.nc2.stream.NcStreamProto.Dimension getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Dimension.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.Dimension build() {
ucar.nc2.stream.NcStreamProto.Dimension result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.Dimension buildPartial() {
ucar.nc2.stream.NcStreamProto.Dimension result = new ucar.nc2.stream.NcStreamProto.Dimension(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.length_ = length_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.isUnlimited_ = isUnlimited_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.isVlen_ = isVlen_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.isPrivate_ = isPrivate_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Dimension) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Dimension)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Dimension other) {
if (other == ucar.nc2.stream.NcStreamProto.Dimension.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasLength()) {
setLength(other.getLength());
}
if (other.hasIsUnlimited()) {
setIsUnlimited(other.getIsUnlimited());
}
if (other.hasIsVlen()) {
setIsVlen(other.getIsVlen());
}
if (other.hasIsPrivate()) {
setIsPrivate(other.getIsPrivate());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.Dimension parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.Dimension) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string name = 1;
private java.lang.Object name_ = "";
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 1;
*
*
* short name - optional when private
*
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// optional uint64 length = 2;
private long length_ ;
/**
* optional uint64 length = 2;
*
*
* optional when vlen
*
*/
public boolean hasLength() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint64 length = 2;
*
*
* optional when vlen
*
*/
public long getLength() {
return length_;
}
/**
* optional uint64 length = 2;
*
*
* optional when vlen
*
*/
public Builder setLength(long value) {
bitField0_ |= 0x00000002;
length_ = value;
onChanged();
return this;
}
/**
* optional uint64 length = 2;
*
*
* optional when vlen
*
*/
public Builder clearLength() {
bitField0_ = (bitField0_ & ~0x00000002);
length_ = 0L;
onChanged();
return this;
}
// optional bool isUnlimited = 3 [default = false];
private boolean isUnlimited_ ;
/**
* optional bool isUnlimited = 3 [default = false];
*
*
* is this needed ??
*
*/
public boolean hasIsUnlimited() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool isUnlimited = 3 [default = false];
*
*
* is this needed ??
*
*/
public boolean getIsUnlimited() {
return isUnlimited_;
}
/**
* optional bool isUnlimited = 3 [default = false];
*
*
* is this needed ??
*
*/
public Builder setIsUnlimited(boolean value) {
bitField0_ |= 0x00000004;
isUnlimited_ = value;
onChanged();
return this;
}
/**
* optional bool isUnlimited = 3 [default = false];
*
*
* is this needed ??
*
*/
public Builder clearIsUnlimited() {
bitField0_ = (bitField0_ & ~0x00000004);
isUnlimited_ = false;
onChanged();
return this;
}
// optional bool isVlen = 4 [default = false];
private boolean isVlen_ ;
/**
* optional bool isVlen = 4 [default = false];
*/
public boolean hasIsVlen() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool isVlen = 4 [default = false];
*/
public boolean getIsVlen() {
return isVlen_;
}
/**
* optional bool isVlen = 4 [default = false];
*/
public Builder setIsVlen(boolean value) {
bitField0_ |= 0x00000008;
isVlen_ = value;
onChanged();
return this;
}
/**
* optional bool isVlen = 4 [default = false];
*/
public Builder clearIsVlen() {
bitField0_ = (bitField0_ & ~0x00000008);
isVlen_ = false;
onChanged();
return this;
}
// optional bool isPrivate = 5 [default = false];
private boolean isPrivate_ ;
/**
* optional bool isPrivate = 5 [default = false];
*/
public boolean hasIsPrivate() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool isPrivate = 5 [default = false];
*/
public boolean getIsPrivate() {
return isPrivate_;
}
/**
* optional bool isPrivate = 5 [default = false];
*/
public Builder setIsPrivate(boolean value) {
bitField0_ |= 0x00000010;
isPrivate_ = value;
onChanged();
return this;
}
/**
* optional bool isPrivate = 5 [default = false];
*/
public Builder clearIsPrivate() {
bitField0_ = (bitField0_ & ~0x00000010);
isPrivate_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Dimension)
}
static {
defaultInstance = new Dimension(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Dimension)
}
public interface VariableOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
/**
* required string name = 1;
*
*
* short name
*
*/
boolean hasName();
/**
* required string name = 1;
*
*
* short name
*
*/
java.lang.String getName();
/**
* required string name = 1;
*
*
* short name
*
*/
com.google.protobuf.ByteString
getNameBytes();
// required .ncstream.DataType dataType = 2;
/**
* required .ncstream.DataType dataType = 2;
*/
boolean hasDataType();
/**
* required .ncstream.DataType dataType = 2;
*/
ucar.nc2.stream.NcStreamProto.DataType getDataType();
// repeated .ncstream.Dimension shape = 3;
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
java.util.List
getShapeList();
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
ucar.nc2.stream.NcStreamProto.Dimension getShape(int index);
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
int getShapeCount();
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getShapeOrBuilderList();
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
ucar.nc2.stream.NcStreamProto.DimensionOrBuilder getShapeOrBuilder(
int index);
// repeated .ncstream.Attribute atts = 4;
/**
* repeated .ncstream.Attribute atts = 4;
*/
java.util.List
getAttsList();
/**
* repeated .ncstream.Attribute atts = 4;
*/
ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index);
/**
* repeated .ncstream.Attribute atts = 4;
*/
int getAttsCount();
/**
* repeated .ncstream.Attribute atts = 4;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsOrBuilderList();
/**
* repeated .ncstream.Attribute atts = 4;
*/
ucar.nc2.stream.NcStreamProto.AttributeOrBuilder getAttsOrBuilder(
int index);
// optional bool unsigned = 5 [default = false];
/**
* optional bool unsigned = 5 [default = false];
*/
boolean hasUnsigned();
/**
* optional bool unsigned = 5 [default = false];
*/
boolean getUnsigned();
// optional bytes data = 6;
/**
* optional bytes data = 6;
*
*
* "immediate" - store small data in header
*
*/
boolean hasData();
/**
* optional bytes data = 6;
*
*
* "immediate" - store small data in header
*
*/
com.google.protobuf.ByteString getData();
// optional string enumType = 7;
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
boolean hasEnumType();
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
java.lang.String getEnumType();
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
com.google.protobuf.ByteString
getEnumTypeBytes();
}
/**
* Protobuf type {@code ncstream.Variable}
*/
public static final class Variable extends
com.google.protobuf.GeneratedMessage
implements VariableOrBuilder {
// Use Variable.newBuilder() to construct.
private Variable(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Variable(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Variable defaultInstance;
public static Variable getDefaultInstance() {
return defaultInstance;
}
public Variable getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Variable(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
ucar.nc2.stream.NcStreamProto.DataType value = ucar.nc2.stream.NcStreamProto.DataType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
dataType_ = value;
}
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
shape_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
shape_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Dimension.PARSER, extensionRegistry));
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
atts_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
atts_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Attribute.PARSER, extensionRegistry));
break;
}
case 40: {
bitField0_ |= 0x00000004;
unsigned_ = input.readBool();
break;
}
case 50: {
bitField0_ |= 0x00000008;
data_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000010;
enumType_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
shape_ = java.util.Collections.unmodifiableList(shape_);
}
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
atts_ = java.util.Collections.unmodifiableList(atts_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Variable_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Variable_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Variable.class, ucar.nc2.stream.NcStreamProto.Variable.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Variable parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Variable(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* required string name = 1;
*
*
* short name
*
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*
*
* short name
*
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*
*
* short name
*
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required .ncstream.DataType dataType = 2;
public static final int DATATYPE_FIELD_NUMBER = 2;
private ucar.nc2.stream.NcStreamProto.DataType dataType_;
/**
* required .ncstream.DataType dataType = 2;
*/
public boolean hasDataType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .ncstream.DataType dataType = 2;
*/
public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
return dataType_;
}
// repeated .ncstream.Dimension shape = 3;
public static final int SHAPE_FIELD_NUMBER = 3;
private java.util.List shape_;
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public java.util.List getShapeList() {
return shape_;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getShapeOrBuilderList() {
return shape_;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public int getShapeCount() {
return shape_.size();
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public ucar.nc2.stream.NcStreamProto.Dimension getShape(int index) {
return shape_.get(index);
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public ucar.nc2.stream.NcStreamProto.DimensionOrBuilder getShapeOrBuilder(
int index) {
return shape_.get(index);
}
// repeated .ncstream.Attribute atts = 4;
public static final int ATTS_FIELD_NUMBER = 4;
private java.util.List atts_;
/**
* repeated .ncstream.Attribute atts = 4;
*/
public java.util.List getAttsList() {
return atts_;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsOrBuilderList() {
return atts_;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public int getAttsCount() {
return atts_.size();
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
return atts_.get(index);
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.AttributeOrBuilder getAttsOrBuilder(
int index) {
return atts_.get(index);
}
// optional bool unsigned = 5 [default = false];
public static final int UNSIGNED_FIELD_NUMBER = 5;
private boolean unsigned_;
/**
* optional bool unsigned = 5 [default = false];
*/
public boolean hasUnsigned() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool unsigned = 5 [default = false];
*/
public boolean getUnsigned() {
return unsigned_;
}
// optional bytes data = 6;
public static final int DATA_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString data_;
/**
* optional bytes data = 6;
*
*
* "immediate" - store small data in header
*
*/
public boolean hasData() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes data = 6;
*
*
* "immediate" - store small data in header
*
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
// optional string enumType = 7;
public static final int ENUMTYPE_FIELD_NUMBER = 7;
private java.lang.Object enumType_;
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
public boolean hasEnumType() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
public java.lang.String getEnumType() {
java.lang.Object ref = enumType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
enumType_ = s;
}
return s;
}
}
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
public com.google.protobuf.ByteString
getEnumTypeBytes() {
java.lang.Object ref = enumType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
enumType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
name_ = "";
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
shape_ = java.util.Collections.emptyList();
atts_ = java.util.Collections.emptyList();
unsigned_ = false;
data_ = com.google.protobuf.ByteString.EMPTY;
enumType_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasDataType()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getAttsCount(); i++) {
if (!getAtts(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, dataType_.getNumber());
}
for (int i = 0; i < shape_.size(); i++) {
output.writeMessage(3, shape_.get(i));
}
for (int i = 0; i < atts_.size(); i++) {
output.writeMessage(4, atts_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBool(5, unsigned_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(6, data_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(7, getEnumTypeBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, dataType_.getNumber());
}
for (int i = 0; i < shape_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, shape_.get(i));
}
for (int i = 0; i < atts_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, atts_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, unsigned_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, data_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, getEnumTypeBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Variable parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Variable parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Variable prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.Variable}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.VariableOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Variable_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Variable_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Variable.class, ucar.nc2.stream.NcStreamProto.Variable.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.Variable.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getShapeFieldBuilder();
getAttsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
bitField0_ = (bitField0_ & ~0x00000002);
if (shapeBuilder_ == null) {
shape_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
shapeBuilder_.clear();
}
if (attsBuilder_ == null) {
atts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
attsBuilder_.clear();
}
unsigned_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
data_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
enumType_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Variable_descriptor;
}
public ucar.nc2.stream.NcStreamProto.Variable getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Variable.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.Variable build() {
ucar.nc2.stream.NcStreamProto.Variable result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.Variable buildPartial() {
ucar.nc2.stream.NcStreamProto.Variable result = new ucar.nc2.stream.NcStreamProto.Variable(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.dataType_ = dataType_;
if (shapeBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
shape_ = java.util.Collections.unmodifiableList(shape_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.shape_ = shape_;
} else {
result.shape_ = shapeBuilder_.build();
}
if (attsBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
atts_ = java.util.Collections.unmodifiableList(atts_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.atts_ = atts_;
} else {
result.atts_ = attsBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000004;
}
result.unsigned_ = unsigned_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000008;
}
result.data_ = data_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000010;
}
result.enumType_ = enumType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Variable) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Variable)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Variable other) {
if (other == ucar.nc2.stream.NcStreamProto.Variable.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasDataType()) {
setDataType(other.getDataType());
}
if (shapeBuilder_ == null) {
if (!other.shape_.isEmpty()) {
if (shape_.isEmpty()) {
shape_ = other.shape_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureShapeIsMutable();
shape_.addAll(other.shape_);
}
onChanged();
}
} else {
if (!other.shape_.isEmpty()) {
if (shapeBuilder_.isEmpty()) {
shapeBuilder_.dispose();
shapeBuilder_ = null;
shape_ = other.shape_;
bitField0_ = (bitField0_ & ~0x00000004);
shapeBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getShapeFieldBuilder() : null;
} else {
shapeBuilder_.addAllMessages(other.shape_);
}
}
}
if (attsBuilder_ == null) {
if (!other.atts_.isEmpty()) {
if (atts_.isEmpty()) {
atts_ = other.atts_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureAttsIsMutable();
atts_.addAll(other.atts_);
}
onChanged();
}
} else {
if (!other.atts_.isEmpty()) {
if (attsBuilder_.isEmpty()) {
attsBuilder_.dispose();
attsBuilder_ = null;
atts_ = other.atts_;
bitField0_ = (bitField0_ & ~0x00000008);
attsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getAttsFieldBuilder() : null;
} else {
attsBuilder_.addAllMessages(other.atts_);
}
}
}
if (other.hasUnsigned()) {
setUnsigned(other.getUnsigned());
}
if (other.hasData()) {
setData(other.getData());
}
if (other.hasEnumType()) {
bitField0_ |= 0x00000040;
enumType_ = other.enumType_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasDataType()) {
return false;
}
for (int i = 0; i < getAttsCount(); i++) {
if (!getAtts(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.Variable parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.Variable) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*
*
* short name
*
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*
*
* short name
*
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*
*
* short name
*
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*
*
* short name
*
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*
*
* short name
*
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*
*
* short name
*
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// required .ncstream.DataType dataType = 2;
private ucar.nc2.stream.NcStreamProto.DataType dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
/**
* required .ncstream.DataType dataType = 2;
*/
public boolean hasDataType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .ncstream.DataType dataType = 2;
*/
public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
return dataType_;
}
/**
* required .ncstream.DataType dataType = 2;
*/
public Builder setDataType(ucar.nc2.stream.NcStreamProto.DataType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
dataType_ = value;
onChanged();
return this;
}
/**
* required .ncstream.DataType dataType = 2;
*/
public Builder clearDataType() {
bitField0_ = (bitField0_ & ~0x00000002);
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
onChanged();
return this;
}
// repeated .ncstream.Dimension shape = 3;
private java.util.List shape_ =
java.util.Collections.emptyList();
private void ensureShapeIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
shape_ = new java.util.ArrayList(shape_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Dimension, ucar.nc2.stream.NcStreamProto.Dimension.Builder, ucar.nc2.stream.NcStreamProto.DimensionOrBuilder> shapeBuilder_;
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public java.util.List getShapeList() {
if (shapeBuilder_ == null) {
return java.util.Collections.unmodifiableList(shape_);
} else {
return shapeBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public int getShapeCount() {
if (shapeBuilder_ == null) {
return shape_.size();
} else {
return shapeBuilder_.getCount();
}
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public ucar.nc2.stream.NcStreamProto.Dimension getShape(int index) {
if (shapeBuilder_ == null) {
return shape_.get(index);
} else {
return shapeBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public Builder setShape(
int index, ucar.nc2.stream.NcStreamProto.Dimension value) {
if (shapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShapeIsMutable();
shape_.set(index, value);
onChanged();
} else {
shapeBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public Builder setShape(
int index, ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (shapeBuilder_ == null) {
ensureShapeIsMutable();
shape_.set(index, builderForValue.build());
onChanged();
} else {
shapeBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public Builder addShape(ucar.nc2.stream.NcStreamProto.Dimension value) {
if (shapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShapeIsMutable();
shape_.add(value);
onChanged();
} else {
shapeBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public Builder addShape(
int index, ucar.nc2.stream.NcStreamProto.Dimension value) {
if (shapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShapeIsMutable();
shape_.add(index, value);
onChanged();
} else {
shapeBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public Builder addShape(
ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (shapeBuilder_ == null) {
ensureShapeIsMutable();
shape_.add(builderForValue.build());
onChanged();
} else {
shapeBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public Builder addShape(
int index, ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (shapeBuilder_ == null) {
ensureShapeIsMutable();
shape_.add(index, builderForValue.build());
onChanged();
} else {
shapeBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public Builder addAllShape(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Dimension> values) {
if (shapeBuilder_ == null) {
ensureShapeIsMutable();
super.addAll(values, shape_);
onChanged();
} else {
shapeBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public Builder clearShape() {
if (shapeBuilder_ == null) {
shape_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
shapeBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public Builder removeShape(int index) {
if (shapeBuilder_ == null) {
ensureShapeIsMutable();
shape_.remove(index);
onChanged();
} else {
shapeBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public ucar.nc2.stream.NcStreamProto.Dimension.Builder getShapeBuilder(
int index) {
return getShapeFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public ucar.nc2.stream.NcStreamProto.DimensionOrBuilder getShapeOrBuilder(
int index) {
if (shapeBuilder_ == null) {
return shape_.get(index); } else {
return shapeBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getShapeOrBuilderList() {
if (shapeBuilder_ != null) {
return shapeBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(shape_);
}
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public ucar.nc2.stream.NcStreamProto.Dimension.Builder addShapeBuilder() {
return getShapeFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Dimension.getDefaultInstance());
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public ucar.nc2.stream.NcStreamProto.Dimension.Builder addShapeBuilder(
int index) {
return getShapeFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Dimension.getDefaultInstance());
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference; could use hash id
*
*/
public java.util.List
getShapeBuilderList() {
return getShapeFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Dimension, ucar.nc2.stream.NcStreamProto.Dimension.Builder, ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getShapeFieldBuilder() {
if (shapeBuilder_ == null) {
shapeBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Dimension, ucar.nc2.stream.NcStreamProto.Dimension.Builder, ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>(
shape_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
shape_ = null;
}
return shapeBuilder_;
}
// repeated .ncstream.Attribute atts = 4;
private java.util.List atts_ =
java.util.Collections.emptyList();
private void ensureAttsIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
atts_ = new java.util.ArrayList(atts_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Attribute, ucar.nc2.stream.NcStreamProto.Attribute.Builder, ucar.nc2.stream.NcStreamProto.AttributeOrBuilder> attsBuilder_;
/**
* repeated .ncstream.Attribute atts = 4;
*/
public java.util.List getAttsList() {
if (attsBuilder_ == null) {
return java.util.Collections.unmodifiableList(atts_);
} else {
return attsBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public int getAttsCount() {
if (attsBuilder_ == null) {
return atts_.size();
} else {
return attsBuilder_.getCount();
}
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
if (attsBuilder_ == null) {
return atts_.get(index);
} else {
return attsBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder setAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute value) {
if (attsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttsIsMutable();
atts_.set(index, value);
onChanged();
} else {
attsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder setAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.set(index, builderForValue.build());
onChanged();
} else {
attsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder addAtts(ucar.nc2.stream.NcStreamProto.Attribute value) {
if (attsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttsIsMutable();
atts_.add(value);
onChanged();
} else {
attsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder addAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute value) {
if (attsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttsIsMutable();
atts_.add(index, value);
onChanged();
} else {
attsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder addAtts(
ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.add(builderForValue.build());
onChanged();
} else {
attsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder addAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.add(index, builderForValue.build());
onChanged();
} else {
attsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder addAllAtts(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Attribute> values) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
super.addAll(values, atts_);
onChanged();
} else {
attsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder clearAtts() {
if (attsBuilder_ == null) {
atts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
attsBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder removeAtts(int index) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.remove(index);
onChanged();
} else {
attsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.Attribute.Builder getAttsBuilder(
int index) {
return getAttsFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.AttributeOrBuilder getAttsOrBuilder(
int index) {
if (attsBuilder_ == null) {
return atts_.get(index); } else {
return attsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsOrBuilderList() {
if (attsBuilder_ != null) {
return attsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(atts_);
}
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.Attribute.Builder addAttsBuilder() {
return getAttsFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Attribute.getDefaultInstance());
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.Attribute.Builder addAttsBuilder(
int index) {
return getAttsFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Attribute.getDefaultInstance());
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public java.util.List
getAttsBuilderList() {
return getAttsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Attribute, ucar.nc2.stream.NcStreamProto.Attribute.Builder, ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsFieldBuilder() {
if (attsBuilder_ == null) {
attsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Attribute, ucar.nc2.stream.NcStreamProto.Attribute.Builder, ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>(
atts_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
atts_ = null;
}
return attsBuilder_;
}
// optional bool unsigned = 5 [default = false];
private boolean unsigned_ ;
/**
* optional bool unsigned = 5 [default = false];
*/
public boolean hasUnsigned() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool unsigned = 5 [default = false];
*/
public boolean getUnsigned() {
return unsigned_;
}
/**
* optional bool unsigned = 5 [default = false];
*/
public Builder setUnsigned(boolean value) {
bitField0_ |= 0x00000010;
unsigned_ = value;
onChanged();
return this;
}
/**
* optional bool unsigned = 5 [default = false];
*/
public Builder clearUnsigned() {
bitField0_ = (bitField0_ & ~0x00000010);
unsigned_ = false;
onChanged();
return this;
}
// optional bytes data = 6;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes data = 6;
*
*
* "immediate" - store small data in header
*
*/
public boolean hasData() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bytes data = 6;
*
*
* "immediate" - store small data in header
*
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* optional bytes data = 6;
*
*
* "immediate" - store small data in header
*
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
data_ = value;
onChanged();
return this;
}
/**
* optional bytes data = 6;
*
*
* "immediate" - store small data in header
*
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000020);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
// optional string enumType = 7;
private java.lang.Object enumType_ = "";
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
public boolean hasEnumType() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
public java.lang.String getEnumType() {
java.lang.Object ref = enumType_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
enumType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
public com.google.protobuf.ByteString
getEnumTypeBytes() {
java.lang.Object ref = enumType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
enumType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
public Builder setEnumType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
enumType_ = value;
onChanged();
return this;
}
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
public Builder clearEnumType() {
bitField0_ = (bitField0_ & ~0x00000040);
enumType_ = getDefaultInstance().getEnumType();
onChanged();
return this;
}
/**
* optional string enumType = 7;
*
*
* only for enum types
*
*/
public Builder setEnumTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
enumType_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Variable)
}
static {
defaultInstance = new Variable(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Variable)
}
public interface StructureOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
/**
* required string name = 1;
*
*
* short name
*
*/
boolean hasName();
/**
* required string name = 1;
*
*
* short name
*
*/
java.lang.String getName();
/**
* required string name = 1;
*
*
* short name
*
*/
com.google.protobuf.ByteString
getNameBytes();
// required .ncstream.DataType dataType = 2;
/**
* required .ncstream.DataType dataType = 2;
*
*
* struct or seq
*
*/
boolean hasDataType();
/**
* required .ncstream.DataType dataType = 2;
*
*
* struct or seq
*
*/
ucar.nc2.stream.NcStreamProto.DataType getDataType();
// repeated .ncstream.Dimension shape = 3;
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
java.util.List
getShapeList();
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
ucar.nc2.stream.NcStreamProto.Dimension getShape(int index);
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
int getShapeCount();
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getShapeOrBuilderList();
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
ucar.nc2.stream.NcStreamProto.DimensionOrBuilder getShapeOrBuilder(
int index);
// repeated .ncstream.Attribute atts = 4;
/**
* repeated .ncstream.Attribute atts = 4;
*/
java.util.List
getAttsList();
/**
* repeated .ncstream.Attribute atts = 4;
*/
ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index);
/**
* repeated .ncstream.Attribute atts = 4;
*/
int getAttsCount();
/**
* repeated .ncstream.Attribute atts = 4;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsOrBuilderList();
/**
* repeated .ncstream.Attribute atts = 4;
*/
ucar.nc2.stream.NcStreamProto.AttributeOrBuilder getAttsOrBuilder(
int index);
// repeated .ncstream.Variable vars = 5;
/**
* repeated .ncstream.Variable vars = 5;
*/
java.util.List
getVarsList();
/**
* repeated .ncstream.Variable vars = 5;
*/
ucar.nc2.stream.NcStreamProto.Variable getVars(int index);
/**
* repeated .ncstream.Variable vars = 5;
*/
int getVarsCount();
/**
* repeated .ncstream.Variable vars = 5;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.VariableOrBuilder>
getVarsOrBuilderList();
/**
* repeated .ncstream.Variable vars = 5;
*/
ucar.nc2.stream.NcStreamProto.VariableOrBuilder getVarsOrBuilder(
int index);
// repeated .ncstream.Structure structs = 6;
/**
* repeated .ncstream.Structure structs = 6;
*/
java.util.List
getStructsList();
/**
* repeated .ncstream.Structure structs = 6;
*/
ucar.nc2.stream.NcStreamProto.Structure getStructs(int index);
/**
* repeated .ncstream.Structure structs = 6;
*/
int getStructsCount();
/**
* repeated .ncstream.Structure structs = 6;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.StructureOrBuilder>
getStructsOrBuilderList();
/**
* repeated .ncstream.Structure structs = 6;
*/
ucar.nc2.stream.NcStreamProto.StructureOrBuilder getStructsOrBuilder(
int index);
}
/**
* Protobuf type {@code ncstream.Structure}
*/
public static final class Structure extends
com.google.protobuf.GeneratedMessage
implements StructureOrBuilder {
// Use Structure.newBuilder() to construct.
private Structure(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Structure(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Structure defaultInstance;
public static Structure getDefaultInstance() {
return defaultInstance;
}
public Structure getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Structure(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
ucar.nc2.stream.NcStreamProto.DataType value = ucar.nc2.stream.NcStreamProto.DataType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
dataType_ = value;
}
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
shape_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
shape_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Dimension.PARSER, extensionRegistry));
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
atts_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
atts_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Attribute.PARSER, extensionRegistry));
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
vars_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
vars_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Variable.PARSER, extensionRegistry));
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
structs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
structs_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Structure.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
shape_ = java.util.Collections.unmodifiableList(shape_);
}
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
atts_ = java.util.Collections.unmodifiableList(atts_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
vars_ = java.util.Collections.unmodifiableList(vars_);
}
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
structs_ = java.util.Collections.unmodifiableList(structs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Structure_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Structure_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Structure.class, ucar.nc2.stream.NcStreamProto.Structure.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Structure parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Structure(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* required string name = 1;
*
*
* short name
*
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*
*
* short name
*
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*
*
* short name
*
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required .ncstream.DataType dataType = 2;
public static final int DATATYPE_FIELD_NUMBER = 2;
private ucar.nc2.stream.NcStreamProto.DataType dataType_;
/**
* required .ncstream.DataType dataType = 2;
*
*
* struct or seq
*
*/
public boolean hasDataType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .ncstream.DataType dataType = 2;
*
*
* struct or seq
*
*/
public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
return dataType_;
}
// repeated .ncstream.Dimension shape = 3;
public static final int SHAPE_FIELD_NUMBER = 3;
private java.util.List shape_;
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public java.util.List getShapeList() {
return shape_;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getShapeOrBuilderList() {
return shape_;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public int getShapeCount() {
return shape_.size();
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public ucar.nc2.stream.NcStreamProto.Dimension getShape(int index) {
return shape_.get(index);
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public ucar.nc2.stream.NcStreamProto.DimensionOrBuilder getShapeOrBuilder(
int index) {
return shape_.get(index);
}
// repeated .ncstream.Attribute atts = 4;
public static final int ATTS_FIELD_NUMBER = 4;
private java.util.List atts_;
/**
* repeated .ncstream.Attribute atts = 4;
*/
public java.util.List getAttsList() {
return atts_;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsOrBuilderList() {
return atts_;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public int getAttsCount() {
return atts_.size();
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
return atts_.get(index);
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.AttributeOrBuilder getAttsOrBuilder(
int index) {
return atts_.get(index);
}
// repeated .ncstream.Variable vars = 5;
public static final int VARS_FIELD_NUMBER = 5;
private java.util.List vars_;
/**
* repeated .ncstream.Variable vars = 5;
*/
public java.util.List getVarsList() {
return vars_;
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.VariableOrBuilder>
getVarsOrBuilderList() {
return vars_;
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public int getVarsCount() {
return vars_.size();
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public ucar.nc2.stream.NcStreamProto.Variable getVars(int index) {
return vars_.get(index);
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public ucar.nc2.stream.NcStreamProto.VariableOrBuilder getVarsOrBuilder(
int index) {
return vars_.get(index);
}
// repeated .ncstream.Structure structs = 6;
public static final int STRUCTS_FIELD_NUMBER = 6;
private java.util.List structs_;
/**
* repeated .ncstream.Structure structs = 6;
*/
public java.util.List getStructsList() {
return structs_;
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.StructureOrBuilder>
getStructsOrBuilderList() {
return structs_;
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public int getStructsCount() {
return structs_.size();
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public ucar.nc2.stream.NcStreamProto.Structure getStructs(int index) {
return structs_.get(index);
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public ucar.nc2.stream.NcStreamProto.StructureOrBuilder getStructsOrBuilder(
int index) {
return structs_.get(index);
}
private void initFields() {
name_ = "";
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
shape_ = java.util.Collections.emptyList();
atts_ = java.util.Collections.emptyList();
vars_ = java.util.Collections.emptyList();
structs_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasDataType()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getAttsCount(); i++) {
if (!getAtts(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getVarsCount(); i++) {
if (!getVars(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getStructsCount(); i++) {
if (!getStructs(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, dataType_.getNumber());
}
for (int i = 0; i < shape_.size(); i++) {
output.writeMessage(3, shape_.get(i));
}
for (int i = 0; i < atts_.size(); i++) {
output.writeMessage(4, atts_.get(i));
}
for (int i = 0; i < vars_.size(); i++) {
output.writeMessage(5, vars_.get(i));
}
for (int i = 0; i < structs_.size(); i++) {
output.writeMessage(6, structs_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, dataType_.getNumber());
}
for (int i = 0; i < shape_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, shape_.get(i));
}
for (int i = 0; i < atts_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, atts_.get(i));
}
for (int i = 0; i < vars_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, vars_.get(i));
}
for (int i = 0; i < structs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, structs_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Structure parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Structure parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Structure prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.Structure}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.StructureOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Structure_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Structure_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Structure.class, ucar.nc2.stream.NcStreamProto.Structure.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.Structure.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getShapeFieldBuilder();
getAttsFieldBuilder();
getVarsFieldBuilder();
getStructsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
bitField0_ = (bitField0_ & ~0x00000002);
if (shapeBuilder_ == null) {
shape_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
shapeBuilder_.clear();
}
if (attsBuilder_ == null) {
atts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
attsBuilder_.clear();
}
if (varsBuilder_ == null) {
vars_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
varsBuilder_.clear();
}
if (structsBuilder_ == null) {
structs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
structsBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Structure_descriptor;
}
public ucar.nc2.stream.NcStreamProto.Structure getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Structure.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.Structure build() {
ucar.nc2.stream.NcStreamProto.Structure result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.Structure buildPartial() {
ucar.nc2.stream.NcStreamProto.Structure result = new ucar.nc2.stream.NcStreamProto.Structure(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.dataType_ = dataType_;
if (shapeBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
shape_ = java.util.Collections.unmodifiableList(shape_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.shape_ = shape_;
} else {
result.shape_ = shapeBuilder_.build();
}
if (attsBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
atts_ = java.util.Collections.unmodifiableList(atts_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.atts_ = atts_;
} else {
result.atts_ = attsBuilder_.build();
}
if (varsBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
vars_ = java.util.Collections.unmodifiableList(vars_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.vars_ = vars_;
} else {
result.vars_ = varsBuilder_.build();
}
if (structsBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
structs_ = java.util.Collections.unmodifiableList(structs_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.structs_ = structs_;
} else {
result.structs_ = structsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Structure) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Structure)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Structure other) {
if (other == ucar.nc2.stream.NcStreamProto.Structure.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasDataType()) {
setDataType(other.getDataType());
}
if (shapeBuilder_ == null) {
if (!other.shape_.isEmpty()) {
if (shape_.isEmpty()) {
shape_ = other.shape_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureShapeIsMutable();
shape_.addAll(other.shape_);
}
onChanged();
}
} else {
if (!other.shape_.isEmpty()) {
if (shapeBuilder_.isEmpty()) {
shapeBuilder_.dispose();
shapeBuilder_ = null;
shape_ = other.shape_;
bitField0_ = (bitField0_ & ~0x00000004);
shapeBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getShapeFieldBuilder() : null;
} else {
shapeBuilder_.addAllMessages(other.shape_);
}
}
}
if (attsBuilder_ == null) {
if (!other.atts_.isEmpty()) {
if (atts_.isEmpty()) {
atts_ = other.atts_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureAttsIsMutable();
atts_.addAll(other.atts_);
}
onChanged();
}
} else {
if (!other.atts_.isEmpty()) {
if (attsBuilder_.isEmpty()) {
attsBuilder_.dispose();
attsBuilder_ = null;
atts_ = other.atts_;
bitField0_ = (bitField0_ & ~0x00000008);
attsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getAttsFieldBuilder() : null;
} else {
attsBuilder_.addAllMessages(other.atts_);
}
}
}
if (varsBuilder_ == null) {
if (!other.vars_.isEmpty()) {
if (vars_.isEmpty()) {
vars_ = other.vars_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureVarsIsMutable();
vars_.addAll(other.vars_);
}
onChanged();
}
} else {
if (!other.vars_.isEmpty()) {
if (varsBuilder_.isEmpty()) {
varsBuilder_.dispose();
varsBuilder_ = null;
vars_ = other.vars_;
bitField0_ = (bitField0_ & ~0x00000010);
varsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getVarsFieldBuilder() : null;
} else {
varsBuilder_.addAllMessages(other.vars_);
}
}
}
if (structsBuilder_ == null) {
if (!other.structs_.isEmpty()) {
if (structs_.isEmpty()) {
structs_ = other.structs_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureStructsIsMutable();
structs_.addAll(other.structs_);
}
onChanged();
}
} else {
if (!other.structs_.isEmpty()) {
if (structsBuilder_.isEmpty()) {
structsBuilder_.dispose();
structsBuilder_ = null;
structs_ = other.structs_;
bitField0_ = (bitField0_ & ~0x00000020);
structsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getStructsFieldBuilder() : null;
} else {
structsBuilder_.addAllMessages(other.structs_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasDataType()) {
return false;
}
for (int i = 0; i < getAttsCount(); i++) {
if (!getAtts(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getVarsCount(); i++) {
if (!getVars(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getStructsCount(); i++) {
if (!getStructs(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.Structure parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.Structure) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*
*
* short name
*
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*
*
* short name
*
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*
*
* short name
*
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*
*
* short name
*
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*
*
* short name
*
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*
*
* short name
*
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// required .ncstream.DataType dataType = 2;
private ucar.nc2.stream.NcStreamProto.DataType dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
/**
* required .ncstream.DataType dataType = 2;
*
*
* struct or seq
*
*/
public boolean hasDataType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .ncstream.DataType dataType = 2;
*
*
* struct or seq
*
*/
public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
return dataType_;
}
/**
* required .ncstream.DataType dataType = 2;
*
*
* struct or seq
*
*/
public Builder setDataType(ucar.nc2.stream.NcStreamProto.DataType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
dataType_ = value;
onChanged();
return this;
}
/**
* required .ncstream.DataType dataType = 2;
*
*
* struct or seq
*
*/
public Builder clearDataType() {
bitField0_ = (bitField0_ & ~0x00000002);
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
onChanged();
return this;
}
// repeated .ncstream.Dimension shape = 3;
private java.util.List shape_ =
java.util.Collections.emptyList();
private void ensureShapeIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
shape_ = new java.util.ArrayList(shape_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Dimension, ucar.nc2.stream.NcStreamProto.Dimension.Builder, ucar.nc2.stream.NcStreamProto.DimensionOrBuilder> shapeBuilder_;
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public java.util.List getShapeList() {
if (shapeBuilder_ == null) {
return java.util.Collections.unmodifiableList(shape_);
} else {
return shapeBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public int getShapeCount() {
if (shapeBuilder_ == null) {
return shape_.size();
} else {
return shapeBuilder_.getCount();
}
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public ucar.nc2.stream.NcStreamProto.Dimension getShape(int index) {
if (shapeBuilder_ == null) {
return shape_.get(index);
} else {
return shapeBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public Builder setShape(
int index, ucar.nc2.stream.NcStreamProto.Dimension value) {
if (shapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShapeIsMutable();
shape_.set(index, value);
onChanged();
} else {
shapeBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public Builder setShape(
int index, ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (shapeBuilder_ == null) {
ensureShapeIsMutable();
shape_.set(index, builderForValue.build());
onChanged();
} else {
shapeBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public Builder addShape(ucar.nc2.stream.NcStreamProto.Dimension value) {
if (shapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShapeIsMutable();
shape_.add(value);
onChanged();
} else {
shapeBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public Builder addShape(
int index, ucar.nc2.stream.NcStreamProto.Dimension value) {
if (shapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureShapeIsMutable();
shape_.add(index, value);
onChanged();
} else {
shapeBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public Builder addShape(
ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (shapeBuilder_ == null) {
ensureShapeIsMutable();
shape_.add(builderForValue.build());
onChanged();
} else {
shapeBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public Builder addShape(
int index, ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (shapeBuilder_ == null) {
ensureShapeIsMutable();
shape_.add(index, builderForValue.build());
onChanged();
} else {
shapeBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public Builder addAllShape(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Dimension> values) {
if (shapeBuilder_ == null) {
ensureShapeIsMutable();
super.addAll(values, shape_);
onChanged();
} else {
shapeBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public Builder clearShape() {
if (shapeBuilder_ == null) {
shape_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
shapeBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public Builder removeShape(int index) {
if (shapeBuilder_ == null) {
ensureShapeIsMutable();
shape_.remove(index);
onChanged();
} else {
shapeBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public ucar.nc2.stream.NcStreamProto.Dimension.Builder getShapeBuilder(
int index) {
return getShapeFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public ucar.nc2.stream.NcStreamProto.DimensionOrBuilder getShapeOrBuilder(
int index) {
if (shapeBuilder_ == null) {
return shape_.get(index); } else {
return shapeBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getShapeOrBuilderList() {
if (shapeBuilder_ != null) {
return shapeBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(shape_);
}
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public ucar.nc2.stream.NcStreamProto.Dimension.Builder addShapeBuilder() {
return getShapeFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Dimension.getDefaultInstance());
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public ucar.nc2.stream.NcStreamProto.Dimension.Builder addShapeBuilder(
int index) {
return getShapeFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Dimension.getDefaultInstance());
}
/**
* repeated .ncstream.Dimension shape = 3;
*
*
* actual dimension instead of reference
*
*/
public java.util.List
getShapeBuilderList() {
return getShapeFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Dimension, ucar.nc2.stream.NcStreamProto.Dimension.Builder, ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getShapeFieldBuilder() {
if (shapeBuilder_ == null) {
shapeBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Dimension, ucar.nc2.stream.NcStreamProto.Dimension.Builder, ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>(
shape_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
shape_ = null;
}
return shapeBuilder_;
}
// repeated .ncstream.Attribute atts = 4;
private java.util.List atts_ =
java.util.Collections.emptyList();
private void ensureAttsIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
atts_ = new java.util.ArrayList(atts_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Attribute, ucar.nc2.stream.NcStreamProto.Attribute.Builder, ucar.nc2.stream.NcStreamProto.AttributeOrBuilder> attsBuilder_;
/**
* repeated .ncstream.Attribute atts = 4;
*/
public java.util.List getAttsList() {
if (attsBuilder_ == null) {
return java.util.Collections.unmodifiableList(atts_);
} else {
return attsBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public int getAttsCount() {
if (attsBuilder_ == null) {
return atts_.size();
} else {
return attsBuilder_.getCount();
}
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
if (attsBuilder_ == null) {
return atts_.get(index);
} else {
return attsBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder setAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute value) {
if (attsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttsIsMutable();
atts_.set(index, value);
onChanged();
} else {
attsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder setAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.set(index, builderForValue.build());
onChanged();
} else {
attsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder addAtts(ucar.nc2.stream.NcStreamProto.Attribute value) {
if (attsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttsIsMutable();
atts_.add(value);
onChanged();
} else {
attsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder addAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute value) {
if (attsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttsIsMutable();
atts_.add(index, value);
onChanged();
} else {
attsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder addAtts(
ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.add(builderForValue.build());
onChanged();
} else {
attsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder addAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.add(index, builderForValue.build());
onChanged();
} else {
attsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder addAllAtts(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Attribute> values) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
super.addAll(values, atts_);
onChanged();
} else {
attsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder clearAtts() {
if (attsBuilder_ == null) {
atts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
attsBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public Builder removeAtts(int index) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.remove(index);
onChanged();
} else {
attsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.Attribute.Builder getAttsBuilder(
int index) {
return getAttsFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.AttributeOrBuilder getAttsOrBuilder(
int index) {
if (attsBuilder_ == null) {
return atts_.get(index); } else {
return attsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsOrBuilderList() {
if (attsBuilder_ != null) {
return attsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(atts_);
}
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.Attribute.Builder addAttsBuilder() {
return getAttsFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Attribute.getDefaultInstance());
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public ucar.nc2.stream.NcStreamProto.Attribute.Builder addAttsBuilder(
int index) {
return getAttsFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Attribute.getDefaultInstance());
}
/**
* repeated .ncstream.Attribute atts = 4;
*/
public java.util.List
getAttsBuilderList() {
return getAttsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Attribute, ucar.nc2.stream.NcStreamProto.Attribute.Builder, ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsFieldBuilder() {
if (attsBuilder_ == null) {
attsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Attribute, ucar.nc2.stream.NcStreamProto.Attribute.Builder, ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>(
atts_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
atts_ = null;
}
return attsBuilder_;
}
// repeated .ncstream.Variable vars = 5;
private java.util.List vars_ =
java.util.Collections.emptyList();
private void ensureVarsIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
vars_ = new java.util.ArrayList(vars_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Variable, ucar.nc2.stream.NcStreamProto.Variable.Builder, ucar.nc2.stream.NcStreamProto.VariableOrBuilder> varsBuilder_;
/**
* repeated .ncstream.Variable vars = 5;
*/
public java.util.List getVarsList() {
if (varsBuilder_ == null) {
return java.util.Collections.unmodifiableList(vars_);
} else {
return varsBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public int getVarsCount() {
if (varsBuilder_ == null) {
return vars_.size();
} else {
return varsBuilder_.getCount();
}
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public ucar.nc2.stream.NcStreamProto.Variable getVars(int index) {
if (varsBuilder_ == null) {
return vars_.get(index);
} else {
return varsBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public Builder setVars(
int index, ucar.nc2.stream.NcStreamProto.Variable value) {
if (varsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVarsIsMutable();
vars_.set(index, value);
onChanged();
} else {
varsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public Builder setVars(
int index, ucar.nc2.stream.NcStreamProto.Variable.Builder builderForValue) {
if (varsBuilder_ == null) {
ensureVarsIsMutable();
vars_.set(index, builderForValue.build());
onChanged();
} else {
varsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public Builder addVars(ucar.nc2.stream.NcStreamProto.Variable value) {
if (varsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVarsIsMutable();
vars_.add(value);
onChanged();
} else {
varsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public Builder addVars(
int index, ucar.nc2.stream.NcStreamProto.Variable value) {
if (varsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVarsIsMutable();
vars_.add(index, value);
onChanged();
} else {
varsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public Builder addVars(
ucar.nc2.stream.NcStreamProto.Variable.Builder builderForValue) {
if (varsBuilder_ == null) {
ensureVarsIsMutable();
vars_.add(builderForValue.build());
onChanged();
} else {
varsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public Builder addVars(
int index, ucar.nc2.stream.NcStreamProto.Variable.Builder builderForValue) {
if (varsBuilder_ == null) {
ensureVarsIsMutable();
vars_.add(index, builderForValue.build());
onChanged();
} else {
varsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public Builder addAllVars(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Variable> values) {
if (varsBuilder_ == null) {
ensureVarsIsMutable();
super.addAll(values, vars_);
onChanged();
} else {
varsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public Builder clearVars() {
if (varsBuilder_ == null) {
vars_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
varsBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public Builder removeVars(int index) {
if (varsBuilder_ == null) {
ensureVarsIsMutable();
vars_.remove(index);
onChanged();
} else {
varsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public ucar.nc2.stream.NcStreamProto.Variable.Builder getVarsBuilder(
int index) {
return getVarsFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public ucar.nc2.stream.NcStreamProto.VariableOrBuilder getVarsOrBuilder(
int index) {
if (varsBuilder_ == null) {
return vars_.get(index); } else {
return varsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.VariableOrBuilder>
getVarsOrBuilderList() {
if (varsBuilder_ != null) {
return varsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(vars_);
}
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public ucar.nc2.stream.NcStreamProto.Variable.Builder addVarsBuilder() {
return getVarsFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Variable.getDefaultInstance());
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public ucar.nc2.stream.NcStreamProto.Variable.Builder addVarsBuilder(
int index) {
return getVarsFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Variable.getDefaultInstance());
}
/**
* repeated .ncstream.Variable vars = 5;
*/
public java.util.List
getVarsBuilderList() {
return getVarsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Variable, ucar.nc2.stream.NcStreamProto.Variable.Builder, ucar.nc2.stream.NcStreamProto.VariableOrBuilder>
getVarsFieldBuilder() {
if (varsBuilder_ == null) {
varsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Variable, ucar.nc2.stream.NcStreamProto.Variable.Builder, ucar.nc2.stream.NcStreamProto.VariableOrBuilder>(
vars_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
vars_ = null;
}
return varsBuilder_;
}
// repeated .ncstream.Structure structs = 6;
private java.util.List structs_ =
java.util.Collections.emptyList();
private void ensureStructsIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
structs_ = new java.util.ArrayList(structs_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Structure, ucar.nc2.stream.NcStreamProto.Structure.Builder, ucar.nc2.stream.NcStreamProto.StructureOrBuilder> structsBuilder_;
/**
* repeated .ncstream.Structure structs = 6;
*/
public java.util.List getStructsList() {
if (structsBuilder_ == null) {
return java.util.Collections.unmodifiableList(structs_);
} else {
return structsBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public int getStructsCount() {
if (structsBuilder_ == null) {
return structs_.size();
} else {
return structsBuilder_.getCount();
}
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public ucar.nc2.stream.NcStreamProto.Structure getStructs(int index) {
if (structsBuilder_ == null) {
return structs_.get(index);
} else {
return structsBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public Builder setStructs(
int index, ucar.nc2.stream.NcStreamProto.Structure value) {
if (structsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStructsIsMutable();
structs_.set(index, value);
onChanged();
} else {
structsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public Builder setStructs(
int index, ucar.nc2.stream.NcStreamProto.Structure.Builder builderForValue) {
if (structsBuilder_ == null) {
ensureStructsIsMutable();
structs_.set(index, builderForValue.build());
onChanged();
} else {
structsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public Builder addStructs(ucar.nc2.stream.NcStreamProto.Structure value) {
if (structsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStructsIsMutable();
structs_.add(value);
onChanged();
} else {
structsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public Builder addStructs(
int index, ucar.nc2.stream.NcStreamProto.Structure value) {
if (structsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStructsIsMutable();
structs_.add(index, value);
onChanged();
} else {
structsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public Builder addStructs(
ucar.nc2.stream.NcStreamProto.Structure.Builder builderForValue) {
if (structsBuilder_ == null) {
ensureStructsIsMutable();
structs_.add(builderForValue.build());
onChanged();
} else {
structsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public Builder addStructs(
int index, ucar.nc2.stream.NcStreamProto.Structure.Builder builderForValue) {
if (structsBuilder_ == null) {
ensureStructsIsMutable();
structs_.add(index, builderForValue.build());
onChanged();
} else {
structsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public Builder addAllStructs(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Structure> values) {
if (structsBuilder_ == null) {
ensureStructsIsMutable();
super.addAll(values, structs_);
onChanged();
} else {
structsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public Builder clearStructs() {
if (structsBuilder_ == null) {
structs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
structsBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public Builder removeStructs(int index) {
if (structsBuilder_ == null) {
ensureStructsIsMutable();
structs_.remove(index);
onChanged();
} else {
structsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public ucar.nc2.stream.NcStreamProto.Structure.Builder getStructsBuilder(
int index) {
return getStructsFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public ucar.nc2.stream.NcStreamProto.StructureOrBuilder getStructsOrBuilder(
int index) {
if (structsBuilder_ == null) {
return structs_.get(index); } else {
return structsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.StructureOrBuilder>
getStructsOrBuilderList() {
if (structsBuilder_ != null) {
return structsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(structs_);
}
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public ucar.nc2.stream.NcStreamProto.Structure.Builder addStructsBuilder() {
return getStructsFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Structure.getDefaultInstance());
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public ucar.nc2.stream.NcStreamProto.Structure.Builder addStructsBuilder(
int index) {
return getStructsFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Structure.getDefaultInstance());
}
/**
* repeated .ncstream.Structure structs = 6;
*/
public java.util.List
getStructsBuilderList() {
return getStructsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Structure, ucar.nc2.stream.NcStreamProto.Structure.Builder, ucar.nc2.stream.NcStreamProto.StructureOrBuilder>
getStructsFieldBuilder() {
if (structsBuilder_ == null) {
structsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Structure, ucar.nc2.stream.NcStreamProto.Structure.Builder, ucar.nc2.stream.NcStreamProto.StructureOrBuilder>(
structs_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
structs_ = null;
}
return structsBuilder_;
}
// @@protoc_insertion_point(builder_scope:ncstream.Structure)
}
static {
defaultInstance = new Structure(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Structure)
}
public interface EnumTypedefOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
/**
* required string name = 1;
*/
boolean hasName();
/**
* required string name = 1;
*/
java.lang.String getName();
/**
* required string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
// repeated .ncstream.EnumTypedef.EnumType map = 2;
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
java.util.List
getMapList();
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType getMap(int index);
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
int getMapCount();
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumTypeOrBuilder>
getMapOrBuilderList();
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumTypeOrBuilder getMapOrBuilder(
int index);
}
/**
* Protobuf type {@code ncstream.EnumTypedef}
*/
public static final class EnumTypedef extends
com.google.protobuf.GeneratedMessage
implements EnumTypedefOrBuilder {
// Use EnumTypedef.newBuilder() to construct.
private EnumTypedef(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private EnumTypedef(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final EnumTypedef defaultInstance;
public static EnumTypedef getDefaultInstance() {
return defaultInstance;
}
public EnumTypedef getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EnumTypedef(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
map_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
map_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
map_ = java.util.Collections.unmodifiableList(map_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.EnumTypedef.class, ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public EnumTypedef parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EnumTypedef(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public interface EnumTypeOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required uint32 code = 1;
/**
* required uint32 code = 1;
*/
boolean hasCode();
/**
* required uint32 code = 1;
*/
int getCode();
// required string value = 2;
/**
* required string value = 2;
*/
boolean hasValue();
/**
* required string value = 2;
*/
java.lang.String getValue();
/**
* required string value = 2;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
* Protobuf type {@code ncstream.EnumTypedef.EnumType}
*/
public static final class EnumType extends
com.google.protobuf.GeneratedMessage
implements EnumTypeOrBuilder {
// Use EnumType.newBuilder() to construct.
private EnumType(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private EnumType(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final EnumType defaultInstance;
public static EnumType getDefaultInstance() {
return defaultInstance;
}
public EnumType getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EnumType(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
code_ = input.readUInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
value_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_EnumType_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_EnumType_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.class, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public EnumType parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EnumType(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required uint32 code = 1;
public static final int CODE_FIELD_NUMBER = 1;
private int code_;
/**
* required uint32 code = 1;
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required uint32 code = 1;
*/
public int getCode() {
return code_;
}
// required string value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private java.lang.Object value_;
/**
* required string value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
code_ = 0;
value_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCode()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(1, code_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getValueBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, code_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getValueBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.EnumTypedef.EnumType}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumTypeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_EnumType_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_EnumType_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.class, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
code_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
value_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_EnumType_descriptor;
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType build() {
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType buildPartial() {
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType result = new ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.code_ = code_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType other) {
if (other == ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.getDefaultInstance()) return this;
if (other.hasCode()) {
setCode(other.getCode());
}
if (other.hasValue()) {
bitField0_ |= 0x00000002;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCode()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required uint32 code = 1;
private int code_ ;
/**
* required uint32 code = 1;
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required uint32 code = 1;
*/
public int getCode() {
return code_;
}
/**
* required uint32 code = 1;
*/
public Builder setCode(int value) {
bitField0_ |= 0x00000001;
code_ = value;
onChanged();
return this;
}
/**
* required uint32 code = 1;
*/
public Builder clearCode() {
bitField0_ = (bitField0_ & ~0x00000001);
code_ = 0;
onChanged();
return this;
}
// required string value = 2;
private java.lang.Object value_ = "";
/**
* required string value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
value_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 2;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 2;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 2;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.EnumTypedef.EnumType)
}
static {
defaultInstance = new EnumType(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.EnumTypedef.EnumType)
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// repeated .ncstream.EnumTypedef.EnumType map = 2;
public static final int MAP_FIELD_NUMBER = 2;
private java.util.List map_;
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public java.util.List getMapList() {
return map_;
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumTypeOrBuilder>
getMapOrBuilderList() {
return map_;
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public int getMapCount() {
return map_.size();
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType getMap(int index) {
return map_.get(index);
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumTypeOrBuilder getMapOrBuilder(
int index) {
return map_.get(index);
}
private void initFields() {
name_ = "";
map_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getMapCount(); i++) {
if (!getMap(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
for (int i = 0; i < map_.size(); i++) {
output.writeMessage(2, map_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
for (int i = 0; i < map_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, map_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.EnumTypedef prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.EnumTypedef}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.EnumTypedefOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.EnumTypedef.class, ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.EnumTypedef.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getMapFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
if (mapBuilder_ == null) {
map_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
mapBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_descriptor;
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.EnumTypedef.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef build() {
ucar.nc2.stream.NcStreamProto.EnumTypedef result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef buildPartial() {
ucar.nc2.stream.NcStreamProto.EnumTypedef result = new ucar.nc2.stream.NcStreamProto.EnumTypedef(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (mapBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
map_ = java.util.Collections.unmodifiableList(map_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.map_ = map_;
} else {
result.map_ = mapBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.EnumTypedef) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.EnumTypedef)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.EnumTypedef other) {
if (other == ucar.nc2.stream.NcStreamProto.EnumTypedef.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (mapBuilder_ == null) {
if (!other.map_.isEmpty()) {
if (map_.isEmpty()) {
map_ = other.map_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureMapIsMutable();
map_.addAll(other.map_);
}
onChanged();
}
} else {
if (!other.map_.isEmpty()) {
if (mapBuilder_.isEmpty()) {
mapBuilder_.dispose();
mapBuilder_ = null;
map_ = other.map_;
bitField0_ = (bitField0_ & ~0x00000002);
mapBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getMapFieldBuilder() : null;
} else {
mapBuilder_.addAllMessages(other.map_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
for (int i = 0; i < getMapCount(); i++) {
if (!getMap(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.EnumTypedef parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.EnumTypedef) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// repeated .ncstream.EnumTypedef.EnumType map = 2;
private java.util.List map_ =
java.util.Collections.emptyList();
private void ensureMapIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
map_ = new java.util.ArrayList(map_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumTypeOrBuilder> mapBuilder_;
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public java.util.List getMapList() {
if (mapBuilder_ == null) {
return java.util.Collections.unmodifiableList(map_);
} else {
return mapBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public int getMapCount() {
if (mapBuilder_ == null) {
return map_.size();
} else {
return mapBuilder_.getCount();
}
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType getMap(int index) {
if (mapBuilder_ == null) {
return map_.get(index);
} else {
return mapBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public Builder setMap(
int index, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType value) {
if (mapBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapIsMutable();
map_.set(index, value);
onChanged();
} else {
mapBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public Builder setMap(
int index, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder builderForValue) {
if (mapBuilder_ == null) {
ensureMapIsMutable();
map_.set(index, builderForValue.build());
onChanged();
} else {
mapBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public Builder addMap(ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType value) {
if (mapBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapIsMutable();
map_.add(value);
onChanged();
} else {
mapBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public Builder addMap(
int index, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType value) {
if (mapBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapIsMutable();
map_.add(index, value);
onChanged();
} else {
mapBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public Builder addMap(
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder builderForValue) {
if (mapBuilder_ == null) {
ensureMapIsMutable();
map_.add(builderForValue.build());
onChanged();
} else {
mapBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public Builder addMap(
int index, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder builderForValue) {
if (mapBuilder_ == null) {
ensureMapIsMutable();
map_.add(index, builderForValue.build());
onChanged();
} else {
mapBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public Builder addAllMap(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType> values) {
if (mapBuilder_ == null) {
ensureMapIsMutable();
super.addAll(values, map_);
onChanged();
} else {
mapBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public Builder clearMap() {
if (mapBuilder_ == null) {
map_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
mapBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public Builder removeMap(int index) {
if (mapBuilder_ == null) {
ensureMapIsMutable();
map_.remove(index);
onChanged();
} else {
mapBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder getMapBuilder(
int index) {
return getMapFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumTypeOrBuilder getMapOrBuilder(
int index) {
if (mapBuilder_ == null) {
return map_.get(index); } else {
return mapBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumTypeOrBuilder>
getMapOrBuilderList() {
if (mapBuilder_ != null) {
return mapBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(map_);
}
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder addMapBuilder() {
return getMapFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.getDefaultInstance());
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder addMapBuilder(
int index) {
return getMapFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.getDefaultInstance());
}
/**
* repeated .ncstream.EnumTypedef.EnumType map = 2;
*/
public java.util.List
getMapBuilderList() {
return getMapFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumTypeOrBuilder>
getMapFieldBuilder() {
if (mapBuilder_ == null) {
mapBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumTypeOrBuilder>(
map_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
map_ = null;
}
return mapBuilder_;
}
// @@protoc_insertion_point(builder_scope:ncstream.EnumTypedef)
}
static {
defaultInstance = new EnumTypedef(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.EnumTypedef)
}
public interface GroupOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
/**
* required string name = 1;
*/
boolean hasName();
/**
* required string name = 1;
*/
java.lang.String getName();
/**
* required string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
// repeated .ncstream.Dimension dims = 2;
/**
* repeated .ncstream.Dimension dims = 2;
*/
java.util.List
getDimsList();
/**
* repeated .ncstream.Dimension dims = 2;
*/
ucar.nc2.stream.NcStreamProto.Dimension getDims(int index);
/**
* repeated .ncstream.Dimension dims = 2;
*/
int getDimsCount();
/**
* repeated .ncstream.Dimension dims = 2;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getDimsOrBuilderList();
/**
* repeated .ncstream.Dimension dims = 2;
*/
ucar.nc2.stream.NcStreamProto.DimensionOrBuilder getDimsOrBuilder(
int index);
// repeated .ncstream.Variable vars = 3;
/**
* repeated .ncstream.Variable vars = 3;
*/
java.util.List
getVarsList();
/**
* repeated .ncstream.Variable vars = 3;
*/
ucar.nc2.stream.NcStreamProto.Variable getVars(int index);
/**
* repeated .ncstream.Variable vars = 3;
*/
int getVarsCount();
/**
* repeated .ncstream.Variable vars = 3;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.VariableOrBuilder>
getVarsOrBuilderList();
/**
* repeated .ncstream.Variable vars = 3;
*/
ucar.nc2.stream.NcStreamProto.VariableOrBuilder getVarsOrBuilder(
int index);
// repeated .ncstream.Structure structs = 4;
/**
* repeated .ncstream.Structure structs = 4;
*/
java.util.List
getStructsList();
/**
* repeated .ncstream.Structure structs = 4;
*/
ucar.nc2.stream.NcStreamProto.Structure getStructs(int index);
/**
* repeated .ncstream.Structure structs = 4;
*/
int getStructsCount();
/**
* repeated .ncstream.Structure structs = 4;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.StructureOrBuilder>
getStructsOrBuilderList();
/**
* repeated .ncstream.Structure structs = 4;
*/
ucar.nc2.stream.NcStreamProto.StructureOrBuilder getStructsOrBuilder(
int index);
// repeated .ncstream.Attribute atts = 5;
/**
* repeated .ncstream.Attribute atts = 5;
*/
java.util.List
getAttsList();
/**
* repeated .ncstream.Attribute atts = 5;
*/
ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index);
/**
* repeated .ncstream.Attribute atts = 5;
*/
int getAttsCount();
/**
* repeated .ncstream.Attribute atts = 5;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsOrBuilderList();
/**
* repeated .ncstream.Attribute atts = 5;
*/
ucar.nc2.stream.NcStreamProto.AttributeOrBuilder getAttsOrBuilder(
int index);
// repeated .ncstream.Group groups = 6;
/**
* repeated .ncstream.Group groups = 6;
*/
java.util.List
getGroupsList();
/**
* repeated .ncstream.Group groups = 6;
*/
ucar.nc2.stream.NcStreamProto.Group getGroups(int index);
/**
* repeated .ncstream.Group groups = 6;
*/
int getGroupsCount();
/**
* repeated .ncstream.Group groups = 6;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.GroupOrBuilder>
getGroupsOrBuilderList();
/**
* repeated .ncstream.Group groups = 6;
*/
ucar.nc2.stream.NcStreamProto.GroupOrBuilder getGroupsOrBuilder(
int index);
// repeated .ncstream.EnumTypedef enumTypes = 7;
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
java.util.List
getEnumTypesList();
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
ucar.nc2.stream.NcStreamProto.EnumTypedef getEnumTypes(int index);
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
int getEnumTypesCount();
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.EnumTypedefOrBuilder>
getEnumTypesOrBuilderList();
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
ucar.nc2.stream.NcStreamProto.EnumTypedefOrBuilder getEnumTypesOrBuilder(
int index);
}
/**
* Protobuf type {@code ncstream.Group}
*/
public static final class Group extends
com.google.protobuf.GeneratedMessage
implements GroupOrBuilder {
// Use Group.newBuilder() to construct.
private Group(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Group(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Group defaultInstance;
public static Group getDefaultInstance() {
return defaultInstance;
}
public Group getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Group(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
dims_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
dims_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Dimension.PARSER, extensionRegistry));
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
vars_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
vars_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Variable.PARSER, extensionRegistry));
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
structs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
structs_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Structure.PARSER, extensionRegistry));
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
atts_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
atts_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Attribute.PARSER, extensionRegistry));
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
groups_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
groups_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Group.PARSER, extensionRegistry));
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
enumTypes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
enumTypes_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.EnumTypedef.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
dims_ = java.util.Collections.unmodifiableList(dims_);
}
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
vars_ = java.util.Collections.unmodifiableList(vars_);
}
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
structs_ = java.util.Collections.unmodifiableList(structs_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
atts_ = java.util.Collections.unmodifiableList(atts_);
}
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
groups_ = java.util.Collections.unmodifiableList(groups_);
}
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
enumTypes_ = java.util.Collections.unmodifiableList(enumTypes_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Group_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Group_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Group.class, ucar.nc2.stream.NcStreamProto.Group.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Group parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Group(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// repeated .ncstream.Dimension dims = 2;
public static final int DIMS_FIELD_NUMBER = 2;
private java.util.List dims_;
/**
* repeated .ncstream.Dimension dims = 2;
*/
public java.util.List getDimsList() {
return dims_;
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getDimsOrBuilderList() {
return dims_;
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public int getDimsCount() {
return dims_.size();
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public ucar.nc2.stream.NcStreamProto.Dimension getDims(int index) {
return dims_.get(index);
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public ucar.nc2.stream.NcStreamProto.DimensionOrBuilder getDimsOrBuilder(
int index) {
return dims_.get(index);
}
// repeated .ncstream.Variable vars = 3;
public static final int VARS_FIELD_NUMBER = 3;
private java.util.List vars_;
/**
* repeated .ncstream.Variable vars = 3;
*/
public java.util.List getVarsList() {
return vars_;
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.VariableOrBuilder>
getVarsOrBuilderList() {
return vars_;
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public int getVarsCount() {
return vars_.size();
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public ucar.nc2.stream.NcStreamProto.Variable getVars(int index) {
return vars_.get(index);
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public ucar.nc2.stream.NcStreamProto.VariableOrBuilder getVarsOrBuilder(
int index) {
return vars_.get(index);
}
// repeated .ncstream.Structure structs = 4;
public static final int STRUCTS_FIELD_NUMBER = 4;
private java.util.List structs_;
/**
* repeated .ncstream.Structure structs = 4;
*/
public java.util.List getStructsList() {
return structs_;
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.StructureOrBuilder>
getStructsOrBuilderList() {
return structs_;
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public int getStructsCount() {
return structs_.size();
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public ucar.nc2.stream.NcStreamProto.Structure getStructs(int index) {
return structs_.get(index);
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public ucar.nc2.stream.NcStreamProto.StructureOrBuilder getStructsOrBuilder(
int index) {
return structs_.get(index);
}
// repeated .ncstream.Attribute atts = 5;
public static final int ATTS_FIELD_NUMBER = 5;
private java.util.List atts_;
/**
* repeated .ncstream.Attribute atts = 5;
*/
public java.util.List getAttsList() {
return atts_;
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsOrBuilderList() {
return atts_;
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public int getAttsCount() {
return atts_.size();
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
return atts_.get(index);
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public ucar.nc2.stream.NcStreamProto.AttributeOrBuilder getAttsOrBuilder(
int index) {
return atts_.get(index);
}
// repeated .ncstream.Group groups = 6;
public static final int GROUPS_FIELD_NUMBER = 6;
private java.util.List groups_;
/**
* repeated .ncstream.Group groups = 6;
*/
public java.util.List getGroupsList() {
return groups_;
}
/**
* repeated .ncstream.Group groups = 6;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.GroupOrBuilder>
getGroupsOrBuilderList() {
return groups_;
}
/**
* repeated .ncstream.Group groups = 6;
*/
public int getGroupsCount() {
return groups_.size();
}
/**
* repeated .ncstream.Group groups = 6;
*/
public ucar.nc2.stream.NcStreamProto.Group getGroups(int index) {
return groups_.get(index);
}
/**
* repeated .ncstream.Group groups = 6;
*/
public ucar.nc2.stream.NcStreamProto.GroupOrBuilder getGroupsOrBuilder(
int index) {
return groups_.get(index);
}
// repeated .ncstream.EnumTypedef enumTypes = 7;
public static final int ENUMTYPES_FIELD_NUMBER = 7;
private java.util.List enumTypes_;
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public java.util.List getEnumTypesList() {
return enumTypes_;
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.EnumTypedefOrBuilder>
getEnumTypesOrBuilderList() {
return enumTypes_;
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public int getEnumTypesCount() {
return enumTypes_.size();
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef getEnumTypes(int index) {
return enumTypes_.get(index);
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedefOrBuilder getEnumTypesOrBuilder(
int index) {
return enumTypes_.get(index);
}
private void initFields() {
name_ = "";
dims_ = java.util.Collections.emptyList();
vars_ = java.util.Collections.emptyList();
structs_ = java.util.Collections.emptyList();
atts_ = java.util.Collections.emptyList();
groups_ = java.util.Collections.emptyList();
enumTypes_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getVarsCount(); i++) {
if (!getVars(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getStructsCount(); i++) {
if (!getStructs(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getAttsCount(); i++) {
if (!getAtts(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getGroupsCount(); i++) {
if (!getGroups(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getEnumTypesCount(); i++) {
if (!getEnumTypes(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
for (int i = 0; i < dims_.size(); i++) {
output.writeMessage(2, dims_.get(i));
}
for (int i = 0; i < vars_.size(); i++) {
output.writeMessage(3, vars_.get(i));
}
for (int i = 0; i < structs_.size(); i++) {
output.writeMessage(4, structs_.get(i));
}
for (int i = 0; i < atts_.size(); i++) {
output.writeMessage(5, atts_.get(i));
}
for (int i = 0; i < groups_.size(); i++) {
output.writeMessage(6, groups_.get(i));
}
for (int i = 0; i < enumTypes_.size(); i++) {
output.writeMessage(7, enumTypes_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
for (int i = 0; i < dims_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, dims_.get(i));
}
for (int i = 0; i < vars_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, vars_.get(i));
}
for (int i = 0; i < structs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, structs_.get(i));
}
for (int i = 0; i < atts_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, atts_.get(i));
}
for (int i = 0; i < groups_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, groups_.get(i));
}
for (int i = 0; i < enumTypes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, enumTypes_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Group parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Group parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Group prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.Group}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.GroupOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Group_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Group_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Group.class, ucar.nc2.stream.NcStreamProto.Group.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.Group.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getDimsFieldBuilder();
getVarsFieldBuilder();
getStructsFieldBuilder();
getAttsFieldBuilder();
getGroupsFieldBuilder();
getEnumTypesFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
if (dimsBuilder_ == null) {
dims_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
dimsBuilder_.clear();
}
if (varsBuilder_ == null) {
vars_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
varsBuilder_.clear();
}
if (structsBuilder_ == null) {
structs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
structsBuilder_.clear();
}
if (attsBuilder_ == null) {
atts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
attsBuilder_.clear();
}
if (groupsBuilder_ == null) {
groups_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
groupsBuilder_.clear();
}
if (enumTypesBuilder_ == null) {
enumTypes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
enumTypesBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Group_descriptor;
}
public ucar.nc2.stream.NcStreamProto.Group getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.Group build() {
ucar.nc2.stream.NcStreamProto.Group result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.Group buildPartial() {
ucar.nc2.stream.NcStreamProto.Group result = new ucar.nc2.stream.NcStreamProto.Group(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (dimsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
dims_ = java.util.Collections.unmodifiableList(dims_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.dims_ = dims_;
} else {
result.dims_ = dimsBuilder_.build();
}
if (varsBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
vars_ = java.util.Collections.unmodifiableList(vars_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.vars_ = vars_;
} else {
result.vars_ = varsBuilder_.build();
}
if (structsBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
structs_ = java.util.Collections.unmodifiableList(structs_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.structs_ = structs_;
} else {
result.structs_ = structsBuilder_.build();
}
if (attsBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
atts_ = java.util.Collections.unmodifiableList(atts_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.atts_ = atts_;
} else {
result.atts_ = attsBuilder_.build();
}
if (groupsBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
groups_ = java.util.Collections.unmodifiableList(groups_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.groups_ = groups_;
} else {
result.groups_ = groupsBuilder_.build();
}
if (enumTypesBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040)) {
enumTypes_ = java.util.Collections.unmodifiableList(enumTypes_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.enumTypes_ = enumTypes_;
} else {
result.enumTypes_ = enumTypesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Group) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Group)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Group other) {
if (other == ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (dimsBuilder_ == null) {
if (!other.dims_.isEmpty()) {
if (dims_.isEmpty()) {
dims_ = other.dims_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureDimsIsMutable();
dims_.addAll(other.dims_);
}
onChanged();
}
} else {
if (!other.dims_.isEmpty()) {
if (dimsBuilder_.isEmpty()) {
dimsBuilder_.dispose();
dimsBuilder_ = null;
dims_ = other.dims_;
bitField0_ = (bitField0_ & ~0x00000002);
dimsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getDimsFieldBuilder() : null;
} else {
dimsBuilder_.addAllMessages(other.dims_);
}
}
}
if (varsBuilder_ == null) {
if (!other.vars_.isEmpty()) {
if (vars_.isEmpty()) {
vars_ = other.vars_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureVarsIsMutable();
vars_.addAll(other.vars_);
}
onChanged();
}
} else {
if (!other.vars_.isEmpty()) {
if (varsBuilder_.isEmpty()) {
varsBuilder_.dispose();
varsBuilder_ = null;
vars_ = other.vars_;
bitField0_ = (bitField0_ & ~0x00000004);
varsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getVarsFieldBuilder() : null;
} else {
varsBuilder_.addAllMessages(other.vars_);
}
}
}
if (structsBuilder_ == null) {
if (!other.structs_.isEmpty()) {
if (structs_.isEmpty()) {
structs_ = other.structs_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureStructsIsMutable();
structs_.addAll(other.structs_);
}
onChanged();
}
} else {
if (!other.structs_.isEmpty()) {
if (structsBuilder_.isEmpty()) {
structsBuilder_.dispose();
structsBuilder_ = null;
structs_ = other.structs_;
bitField0_ = (bitField0_ & ~0x00000008);
structsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getStructsFieldBuilder() : null;
} else {
structsBuilder_.addAllMessages(other.structs_);
}
}
}
if (attsBuilder_ == null) {
if (!other.atts_.isEmpty()) {
if (atts_.isEmpty()) {
atts_ = other.atts_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureAttsIsMutable();
atts_.addAll(other.atts_);
}
onChanged();
}
} else {
if (!other.atts_.isEmpty()) {
if (attsBuilder_.isEmpty()) {
attsBuilder_.dispose();
attsBuilder_ = null;
atts_ = other.atts_;
bitField0_ = (bitField0_ & ~0x00000010);
attsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getAttsFieldBuilder() : null;
} else {
attsBuilder_.addAllMessages(other.atts_);
}
}
}
if (groupsBuilder_ == null) {
if (!other.groups_.isEmpty()) {
if (groups_.isEmpty()) {
groups_ = other.groups_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureGroupsIsMutable();
groups_.addAll(other.groups_);
}
onChanged();
}
} else {
if (!other.groups_.isEmpty()) {
if (groupsBuilder_.isEmpty()) {
groupsBuilder_.dispose();
groupsBuilder_ = null;
groups_ = other.groups_;
bitField0_ = (bitField0_ & ~0x00000020);
groupsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getGroupsFieldBuilder() : null;
} else {
groupsBuilder_.addAllMessages(other.groups_);
}
}
}
if (enumTypesBuilder_ == null) {
if (!other.enumTypes_.isEmpty()) {
if (enumTypes_.isEmpty()) {
enumTypes_ = other.enumTypes_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureEnumTypesIsMutable();
enumTypes_.addAll(other.enumTypes_);
}
onChanged();
}
} else {
if (!other.enumTypes_.isEmpty()) {
if (enumTypesBuilder_.isEmpty()) {
enumTypesBuilder_.dispose();
enumTypesBuilder_ = null;
enumTypes_ = other.enumTypes_;
bitField0_ = (bitField0_ & ~0x00000040);
enumTypesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getEnumTypesFieldBuilder() : null;
} else {
enumTypesBuilder_.addAllMessages(other.enumTypes_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
for (int i = 0; i < getVarsCount(); i++) {
if (!getVars(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getStructsCount(); i++) {
if (!getStructs(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getAttsCount(); i++) {
if (!getAtts(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getGroupsCount(); i++) {
if (!getGroups(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getEnumTypesCount(); i++) {
if (!getEnumTypes(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.Group parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.Group) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// repeated .ncstream.Dimension dims = 2;
private java.util.List dims_ =
java.util.Collections.emptyList();
private void ensureDimsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
dims_ = new java.util.ArrayList(dims_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Dimension, ucar.nc2.stream.NcStreamProto.Dimension.Builder, ucar.nc2.stream.NcStreamProto.DimensionOrBuilder> dimsBuilder_;
/**
* repeated .ncstream.Dimension dims = 2;
*/
public java.util.List getDimsList() {
if (dimsBuilder_ == null) {
return java.util.Collections.unmodifiableList(dims_);
} else {
return dimsBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public int getDimsCount() {
if (dimsBuilder_ == null) {
return dims_.size();
} else {
return dimsBuilder_.getCount();
}
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public ucar.nc2.stream.NcStreamProto.Dimension getDims(int index) {
if (dimsBuilder_ == null) {
return dims_.get(index);
} else {
return dimsBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public Builder setDims(
int index, ucar.nc2.stream.NcStreamProto.Dimension value) {
if (dimsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDimsIsMutable();
dims_.set(index, value);
onChanged();
} else {
dimsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public Builder setDims(
int index, ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (dimsBuilder_ == null) {
ensureDimsIsMutable();
dims_.set(index, builderForValue.build());
onChanged();
} else {
dimsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public Builder addDims(ucar.nc2.stream.NcStreamProto.Dimension value) {
if (dimsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDimsIsMutable();
dims_.add(value);
onChanged();
} else {
dimsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public Builder addDims(
int index, ucar.nc2.stream.NcStreamProto.Dimension value) {
if (dimsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDimsIsMutable();
dims_.add(index, value);
onChanged();
} else {
dimsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public Builder addDims(
ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (dimsBuilder_ == null) {
ensureDimsIsMutable();
dims_.add(builderForValue.build());
onChanged();
} else {
dimsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public Builder addDims(
int index, ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (dimsBuilder_ == null) {
ensureDimsIsMutable();
dims_.add(index, builderForValue.build());
onChanged();
} else {
dimsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public Builder addAllDims(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Dimension> values) {
if (dimsBuilder_ == null) {
ensureDimsIsMutable();
super.addAll(values, dims_);
onChanged();
} else {
dimsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public Builder clearDims() {
if (dimsBuilder_ == null) {
dims_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
dimsBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public Builder removeDims(int index) {
if (dimsBuilder_ == null) {
ensureDimsIsMutable();
dims_.remove(index);
onChanged();
} else {
dimsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public ucar.nc2.stream.NcStreamProto.Dimension.Builder getDimsBuilder(
int index) {
return getDimsFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public ucar.nc2.stream.NcStreamProto.DimensionOrBuilder getDimsOrBuilder(
int index) {
if (dimsBuilder_ == null) {
return dims_.get(index); } else {
return dimsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getDimsOrBuilderList() {
if (dimsBuilder_ != null) {
return dimsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(dims_);
}
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public ucar.nc2.stream.NcStreamProto.Dimension.Builder addDimsBuilder() {
return getDimsFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Dimension.getDefaultInstance());
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public ucar.nc2.stream.NcStreamProto.Dimension.Builder addDimsBuilder(
int index) {
return getDimsFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Dimension.getDefaultInstance());
}
/**
* repeated .ncstream.Dimension dims = 2;
*/
public java.util.List
getDimsBuilderList() {
return getDimsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Dimension, ucar.nc2.stream.NcStreamProto.Dimension.Builder, ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>
getDimsFieldBuilder() {
if (dimsBuilder_ == null) {
dimsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Dimension, ucar.nc2.stream.NcStreamProto.Dimension.Builder, ucar.nc2.stream.NcStreamProto.DimensionOrBuilder>(
dims_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
dims_ = null;
}
return dimsBuilder_;
}
// repeated .ncstream.Variable vars = 3;
private java.util.List vars_ =
java.util.Collections.emptyList();
private void ensureVarsIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
vars_ = new java.util.ArrayList(vars_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Variable, ucar.nc2.stream.NcStreamProto.Variable.Builder, ucar.nc2.stream.NcStreamProto.VariableOrBuilder> varsBuilder_;
/**
* repeated .ncstream.Variable vars = 3;
*/
public java.util.List getVarsList() {
if (varsBuilder_ == null) {
return java.util.Collections.unmodifiableList(vars_);
} else {
return varsBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public int getVarsCount() {
if (varsBuilder_ == null) {
return vars_.size();
} else {
return varsBuilder_.getCount();
}
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public ucar.nc2.stream.NcStreamProto.Variable getVars(int index) {
if (varsBuilder_ == null) {
return vars_.get(index);
} else {
return varsBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public Builder setVars(
int index, ucar.nc2.stream.NcStreamProto.Variable value) {
if (varsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVarsIsMutable();
vars_.set(index, value);
onChanged();
} else {
varsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public Builder setVars(
int index, ucar.nc2.stream.NcStreamProto.Variable.Builder builderForValue) {
if (varsBuilder_ == null) {
ensureVarsIsMutable();
vars_.set(index, builderForValue.build());
onChanged();
} else {
varsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public Builder addVars(ucar.nc2.stream.NcStreamProto.Variable value) {
if (varsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVarsIsMutable();
vars_.add(value);
onChanged();
} else {
varsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public Builder addVars(
int index, ucar.nc2.stream.NcStreamProto.Variable value) {
if (varsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVarsIsMutable();
vars_.add(index, value);
onChanged();
} else {
varsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public Builder addVars(
ucar.nc2.stream.NcStreamProto.Variable.Builder builderForValue) {
if (varsBuilder_ == null) {
ensureVarsIsMutable();
vars_.add(builderForValue.build());
onChanged();
} else {
varsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public Builder addVars(
int index, ucar.nc2.stream.NcStreamProto.Variable.Builder builderForValue) {
if (varsBuilder_ == null) {
ensureVarsIsMutable();
vars_.add(index, builderForValue.build());
onChanged();
} else {
varsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public Builder addAllVars(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Variable> values) {
if (varsBuilder_ == null) {
ensureVarsIsMutable();
super.addAll(values, vars_);
onChanged();
} else {
varsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public Builder clearVars() {
if (varsBuilder_ == null) {
vars_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
varsBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public Builder removeVars(int index) {
if (varsBuilder_ == null) {
ensureVarsIsMutable();
vars_.remove(index);
onChanged();
} else {
varsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public ucar.nc2.stream.NcStreamProto.Variable.Builder getVarsBuilder(
int index) {
return getVarsFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public ucar.nc2.stream.NcStreamProto.VariableOrBuilder getVarsOrBuilder(
int index) {
if (varsBuilder_ == null) {
return vars_.get(index); } else {
return varsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.VariableOrBuilder>
getVarsOrBuilderList() {
if (varsBuilder_ != null) {
return varsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(vars_);
}
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public ucar.nc2.stream.NcStreamProto.Variable.Builder addVarsBuilder() {
return getVarsFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Variable.getDefaultInstance());
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public ucar.nc2.stream.NcStreamProto.Variable.Builder addVarsBuilder(
int index) {
return getVarsFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Variable.getDefaultInstance());
}
/**
* repeated .ncstream.Variable vars = 3;
*/
public java.util.List
getVarsBuilderList() {
return getVarsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Variable, ucar.nc2.stream.NcStreamProto.Variable.Builder, ucar.nc2.stream.NcStreamProto.VariableOrBuilder>
getVarsFieldBuilder() {
if (varsBuilder_ == null) {
varsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Variable, ucar.nc2.stream.NcStreamProto.Variable.Builder, ucar.nc2.stream.NcStreamProto.VariableOrBuilder>(
vars_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
vars_ = null;
}
return varsBuilder_;
}
// repeated .ncstream.Structure structs = 4;
private java.util.List structs_ =
java.util.Collections.emptyList();
private void ensureStructsIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
structs_ = new java.util.ArrayList(structs_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Structure, ucar.nc2.stream.NcStreamProto.Structure.Builder, ucar.nc2.stream.NcStreamProto.StructureOrBuilder> structsBuilder_;
/**
* repeated .ncstream.Structure structs = 4;
*/
public java.util.List getStructsList() {
if (structsBuilder_ == null) {
return java.util.Collections.unmodifiableList(structs_);
} else {
return structsBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public int getStructsCount() {
if (structsBuilder_ == null) {
return structs_.size();
} else {
return structsBuilder_.getCount();
}
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public ucar.nc2.stream.NcStreamProto.Structure getStructs(int index) {
if (structsBuilder_ == null) {
return structs_.get(index);
} else {
return structsBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public Builder setStructs(
int index, ucar.nc2.stream.NcStreamProto.Structure value) {
if (structsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStructsIsMutable();
structs_.set(index, value);
onChanged();
} else {
structsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public Builder setStructs(
int index, ucar.nc2.stream.NcStreamProto.Structure.Builder builderForValue) {
if (structsBuilder_ == null) {
ensureStructsIsMutable();
structs_.set(index, builderForValue.build());
onChanged();
} else {
structsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public Builder addStructs(ucar.nc2.stream.NcStreamProto.Structure value) {
if (structsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStructsIsMutable();
structs_.add(value);
onChanged();
} else {
structsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public Builder addStructs(
int index, ucar.nc2.stream.NcStreamProto.Structure value) {
if (structsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStructsIsMutable();
structs_.add(index, value);
onChanged();
} else {
structsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public Builder addStructs(
ucar.nc2.stream.NcStreamProto.Structure.Builder builderForValue) {
if (structsBuilder_ == null) {
ensureStructsIsMutable();
structs_.add(builderForValue.build());
onChanged();
} else {
structsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public Builder addStructs(
int index, ucar.nc2.stream.NcStreamProto.Structure.Builder builderForValue) {
if (structsBuilder_ == null) {
ensureStructsIsMutable();
structs_.add(index, builderForValue.build());
onChanged();
} else {
structsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public Builder addAllStructs(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Structure> values) {
if (structsBuilder_ == null) {
ensureStructsIsMutable();
super.addAll(values, structs_);
onChanged();
} else {
structsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public Builder clearStructs() {
if (structsBuilder_ == null) {
structs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
structsBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public Builder removeStructs(int index) {
if (structsBuilder_ == null) {
ensureStructsIsMutable();
structs_.remove(index);
onChanged();
} else {
structsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public ucar.nc2.stream.NcStreamProto.Structure.Builder getStructsBuilder(
int index) {
return getStructsFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public ucar.nc2.stream.NcStreamProto.StructureOrBuilder getStructsOrBuilder(
int index) {
if (structsBuilder_ == null) {
return structs_.get(index); } else {
return structsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.StructureOrBuilder>
getStructsOrBuilderList() {
if (structsBuilder_ != null) {
return structsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(structs_);
}
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public ucar.nc2.stream.NcStreamProto.Structure.Builder addStructsBuilder() {
return getStructsFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Structure.getDefaultInstance());
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public ucar.nc2.stream.NcStreamProto.Structure.Builder addStructsBuilder(
int index) {
return getStructsFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Structure.getDefaultInstance());
}
/**
* repeated .ncstream.Structure structs = 4;
*/
public java.util.List
getStructsBuilderList() {
return getStructsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Structure, ucar.nc2.stream.NcStreamProto.Structure.Builder, ucar.nc2.stream.NcStreamProto.StructureOrBuilder>
getStructsFieldBuilder() {
if (structsBuilder_ == null) {
structsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Structure, ucar.nc2.stream.NcStreamProto.Structure.Builder, ucar.nc2.stream.NcStreamProto.StructureOrBuilder>(
structs_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
structs_ = null;
}
return structsBuilder_;
}
// repeated .ncstream.Attribute atts = 5;
private java.util.List atts_ =
java.util.Collections.emptyList();
private void ensureAttsIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
atts_ = new java.util.ArrayList(atts_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Attribute, ucar.nc2.stream.NcStreamProto.Attribute.Builder, ucar.nc2.stream.NcStreamProto.AttributeOrBuilder> attsBuilder_;
/**
* repeated .ncstream.Attribute atts = 5;
*/
public java.util.List getAttsList() {
if (attsBuilder_ == null) {
return java.util.Collections.unmodifiableList(atts_);
} else {
return attsBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public int getAttsCount() {
if (attsBuilder_ == null) {
return atts_.size();
} else {
return attsBuilder_.getCount();
}
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
if (attsBuilder_ == null) {
return atts_.get(index);
} else {
return attsBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public Builder setAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute value) {
if (attsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttsIsMutable();
atts_.set(index, value);
onChanged();
} else {
attsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public Builder setAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.set(index, builderForValue.build());
onChanged();
} else {
attsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public Builder addAtts(ucar.nc2.stream.NcStreamProto.Attribute value) {
if (attsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttsIsMutable();
atts_.add(value);
onChanged();
} else {
attsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public Builder addAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute value) {
if (attsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttsIsMutable();
atts_.add(index, value);
onChanged();
} else {
attsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public Builder addAtts(
ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.add(builderForValue.build());
onChanged();
} else {
attsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public Builder addAtts(
int index, ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.add(index, builderForValue.build());
onChanged();
} else {
attsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public Builder addAllAtts(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Attribute> values) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
super.addAll(values, atts_);
onChanged();
} else {
attsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public Builder clearAtts() {
if (attsBuilder_ == null) {
atts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
attsBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public Builder removeAtts(int index) {
if (attsBuilder_ == null) {
ensureAttsIsMutable();
atts_.remove(index);
onChanged();
} else {
attsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public ucar.nc2.stream.NcStreamProto.Attribute.Builder getAttsBuilder(
int index) {
return getAttsFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public ucar.nc2.stream.NcStreamProto.AttributeOrBuilder getAttsOrBuilder(
int index) {
if (attsBuilder_ == null) {
return atts_.get(index); } else {
return attsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsOrBuilderList() {
if (attsBuilder_ != null) {
return attsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(atts_);
}
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public ucar.nc2.stream.NcStreamProto.Attribute.Builder addAttsBuilder() {
return getAttsFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Attribute.getDefaultInstance());
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public ucar.nc2.stream.NcStreamProto.Attribute.Builder addAttsBuilder(
int index) {
return getAttsFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Attribute.getDefaultInstance());
}
/**
* repeated .ncstream.Attribute atts = 5;
*/
public java.util.List
getAttsBuilderList() {
return getAttsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Attribute, ucar.nc2.stream.NcStreamProto.Attribute.Builder, ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>
getAttsFieldBuilder() {
if (attsBuilder_ == null) {
attsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Attribute, ucar.nc2.stream.NcStreamProto.Attribute.Builder, ucar.nc2.stream.NcStreamProto.AttributeOrBuilder>(
atts_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
atts_ = null;
}
return attsBuilder_;
}
// repeated .ncstream.Group groups = 6;
private java.util.List groups_ =
java.util.Collections.emptyList();
private void ensureGroupsIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
groups_ = new java.util.ArrayList(groups_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Group, ucar.nc2.stream.NcStreamProto.Group.Builder, ucar.nc2.stream.NcStreamProto.GroupOrBuilder> groupsBuilder_;
/**
* repeated .ncstream.Group groups = 6;
*/
public java.util.List getGroupsList() {
if (groupsBuilder_ == null) {
return java.util.Collections.unmodifiableList(groups_);
} else {
return groupsBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Group groups = 6;
*/
public int getGroupsCount() {
if (groupsBuilder_ == null) {
return groups_.size();
} else {
return groupsBuilder_.getCount();
}
}
/**
* repeated .ncstream.Group groups = 6;
*/
public ucar.nc2.stream.NcStreamProto.Group getGroups(int index) {
if (groupsBuilder_ == null) {
return groups_.get(index);
} else {
return groupsBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Group groups = 6;
*/
public Builder setGroups(
int index, ucar.nc2.stream.NcStreamProto.Group value) {
if (groupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupsIsMutable();
groups_.set(index, value);
onChanged();
} else {
groupsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Group groups = 6;
*/
public Builder setGroups(
int index, ucar.nc2.stream.NcStreamProto.Group.Builder builderForValue) {
if (groupsBuilder_ == null) {
ensureGroupsIsMutable();
groups_.set(index, builderForValue.build());
onChanged();
} else {
groupsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Group groups = 6;
*/
public Builder addGroups(ucar.nc2.stream.NcStreamProto.Group value) {
if (groupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupsIsMutable();
groups_.add(value);
onChanged();
} else {
groupsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Group groups = 6;
*/
public Builder addGroups(
int index, ucar.nc2.stream.NcStreamProto.Group value) {
if (groupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupsIsMutable();
groups_.add(index, value);
onChanged();
} else {
groupsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Group groups = 6;
*/
public Builder addGroups(
ucar.nc2.stream.NcStreamProto.Group.Builder builderForValue) {
if (groupsBuilder_ == null) {
ensureGroupsIsMutable();
groups_.add(builderForValue.build());
onChanged();
} else {
groupsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Group groups = 6;
*/
public Builder addGroups(
int index, ucar.nc2.stream.NcStreamProto.Group.Builder builderForValue) {
if (groupsBuilder_ == null) {
ensureGroupsIsMutable();
groups_.add(index, builderForValue.build());
onChanged();
} else {
groupsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Group groups = 6;
*/
public Builder addAllGroups(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Group> values) {
if (groupsBuilder_ == null) {
ensureGroupsIsMutable();
super.addAll(values, groups_);
onChanged();
} else {
groupsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Group groups = 6;
*/
public Builder clearGroups() {
if (groupsBuilder_ == null) {
groups_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
groupsBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Group groups = 6;
*/
public Builder removeGroups(int index) {
if (groupsBuilder_ == null) {
ensureGroupsIsMutable();
groups_.remove(index);
onChanged();
} else {
groupsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Group groups = 6;
*/
public ucar.nc2.stream.NcStreamProto.Group.Builder getGroupsBuilder(
int index) {
return getGroupsFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Group groups = 6;
*/
public ucar.nc2.stream.NcStreamProto.GroupOrBuilder getGroupsOrBuilder(
int index) {
if (groupsBuilder_ == null) {
return groups_.get(index); } else {
return groupsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Group groups = 6;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.GroupOrBuilder>
getGroupsOrBuilderList() {
if (groupsBuilder_ != null) {
return groupsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(groups_);
}
}
/**
* repeated .ncstream.Group groups = 6;
*/
public ucar.nc2.stream.NcStreamProto.Group.Builder addGroupsBuilder() {
return getGroupsFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance());
}
/**
* repeated .ncstream.Group groups = 6;
*/
public ucar.nc2.stream.NcStreamProto.Group.Builder addGroupsBuilder(
int index) {
return getGroupsFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance());
}
/**
* repeated .ncstream.Group groups = 6;
*/
public java.util.List
getGroupsBuilderList() {
return getGroupsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Group, ucar.nc2.stream.NcStreamProto.Group.Builder, ucar.nc2.stream.NcStreamProto.GroupOrBuilder>
getGroupsFieldBuilder() {
if (groupsBuilder_ == null) {
groupsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Group, ucar.nc2.stream.NcStreamProto.Group.Builder, ucar.nc2.stream.NcStreamProto.GroupOrBuilder>(
groups_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
groups_ = null;
}
return groupsBuilder_;
}
// repeated .ncstream.EnumTypedef enumTypes = 7;
private java.util.List enumTypes_ =
java.util.Collections.emptyList();
private void ensureEnumTypesIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
enumTypes_ = new java.util.ArrayList(enumTypes_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.EnumTypedef, ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder, ucar.nc2.stream.NcStreamProto.EnumTypedefOrBuilder> enumTypesBuilder_;
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public java.util.List getEnumTypesList() {
if (enumTypesBuilder_ == null) {
return java.util.Collections.unmodifiableList(enumTypes_);
} else {
return enumTypesBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public int getEnumTypesCount() {
if (enumTypesBuilder_ == null) {
return enumTypes_.size();
} else {
return enumTypesBuilder_.getCount();
}
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef getEnumTypes(int index) {
if (enumTypesBuilder_ == null) {
return enumTypes_.get(index);
} else {
return enumTypesBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public Builder setEnumTypes(
int index, ucar.nc2.stream.NcStreamProto.EnumTypedef value) {
if (enumTypesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEnumTypesIsMutable();
enumTypes_.set(index, value);
onChanged();
} else {
enumTypesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public Builder setEnumTypes(
int index, ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder builderForValue) {
if (enumTypesBuilder_ == null) {
ensureEnumTypesIsMutable();
enumTypes_.set(index, builderForValue.build());
onChanged();
} else {
enumTypesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public Builder addEnumTypes(ucar.nc2.stream.NcStreamProto.EnumTypedef value) {
if (enumTypesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEnumTypesIsMutable();
enumTypes_.add(value);
onChanged();
} else {
enumTypesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public Builder addEnumTypes(
int index, ucar.nc2.stream.NcStreamProto.EnumTypedef value) {
if (enumTypesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEnumTypesIsMutable();
enumTypes_.add(index, value);
onChanged();
} else {
enumTypesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public Builder addEnumTypes(
ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder builderForValue) {
if (enumTypesBuilder_ == null) {
ensureEnumTypesIsMutable();
enumTypes_.add(builderForValue.build());
onChanged();
} else {
enumTypesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public Builder addEnumTypes(
int index, ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder builderForValue) {
if (enumTypesBuilder_ == null) {
ensureEnumTypesIsMutable();
enumTypes_.add(index, builderForValue.build());
onChanged();
} else {
enumTypesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public Builder addAllEnumTypes(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.EnumTypedef> values) {
if (enumTypesBuilder_ == null) {
ensureEnumTypesIsMutable();
super.addAll(values, enumTypes_);
onChanged();
} else {
enumTypesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public Builder clearEnumTypes() {
if (enumTypesBuilder_ == null) {
enumTypes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
enumTypesBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public Builder removeEnumTypes(int index) {
if (enumTypesBuilder_ == null) {
ensureEnumTypesIsMutable();
enumTypes_.remove(index);
onChanged();
} else {
enumTypesBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder getEnumTypesBuilder(
int index) {
return getEnumTypesFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedefOrBuilder getEnumTypesOrBuilder(
int index) {
if (enumTypesBuilder_ == null) {
return enumTypes_.get(index); } else {
return enumTypesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.EnumTypedefOrBuilder>
getEnumTypesOrBuilderList() {
if (enumTypesBuilder_ != null) {
return enumTypesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(enumTypes_);
}
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder addEnumTypesBuilder() {
return getEnumTypesFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.EnumTypedef.getDefaultInstance());
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder addEnumTypesBuilder(
int index) {
return getEnumTypesFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.EnumTypedef.getDefaultInstance());
}
/**
* repeated .ncstream.EnumTypedef enumTypes = 7;
*/
public java.util.List
getEnumTypesBuilderList() {
return getEnumTypesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.EnumTypedef, ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder, ucar.nc2.stream.NcStreamProto.EnumTypedefOrBuilder>
getEnumTypesFieldBuilder() {
if (enumTypesBuilder_ == null) {
enumTypesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.EnumTypedef, ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder, ucar.nc2.stream.NcStreamProto.EnumTypedefOrBuilder>(
enumTypes_,
((bitField0_ & 0x00000040) == 0x00000040),
getParentForChildren(),
isClean());
enumTypes_ = null;
}
return enumTypesBuilder_;
}
// @@protoc_insertion_point(builder_scope:ncstream.Group)
}
static {
defaultInstance = new Group(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Group)
}
public interface HeaderOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string location = 1;
/**
* optional string location = 1;
*/
boolean hasLocation();
/**
* optional string location = 1;
*/
java.lang.String getLocation();
/**
* optional string location = 1;
*/
com.google.protobuf.ByteString
getLocationBytes();
// optional string title = 2;
/**
* optional string title = 2;
*
*
* ??
*
*/
boolean hasTitle();
/**
* optional string title = 2;
*
*
* ??
*
*/
java.lang.String getTitle();
/**
* optional string title = 2;
*
*
* ??
*
*/
com.google.protobuf.ByteString
getTitleBytes();
// optional string id = 3;
/**
* optional string id = 3;
*
*
* ??
*
*/
boolean hasId();
/**
* optional string id = 3;
*
*
* ??
*
*/
java.lang.String getId();
/**
* optional string id = 3;
*
*
* ??
*
*/
com.google.protobuf.ByteString
getIdBytes();
// required .ncstream.Group root = 4;
/**
* required .ncstream.Group root = 4;
*/
boolean hasRoot();
/**
* required .ncstream.Group root = 4;
*/
ucar.nc2.stream.NcStreamProto.Group getRoot();
/**
* required .ncstream.Group root = 4;
*/
ucar.nc2.stream.NcStreamProto.GroupOrBuilder getRootOrBuilder();
// optional uint32 version = 5 [default = 0];
/**
* optional uint32 version = 5 [default = 0];
*/
boolean hasVersion();
/**
* optional uint32 version = 5 [default = 0];
*/
int getVersion();
}
/**
* Protobuf type {@code ncstream.Header}
*/
public static final class Header extends
com.google.protobuf.GeneratedMessage
implements HeaderOrBuilder {
// Use Header.newBuilder() to construct.
private Header(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Header(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Header defaultInstance;
public static Header getDefaultInstance() {
return defaultInstance;
}
public Header getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Header(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
location_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
title_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
id_ = input.readBytes();
break;
}
case 34: {
ucar.nc2.stream.NcStreamProto.Group.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = root_.toBuilder();
}
root_ = input.readMessage(ucar.nc2.stream.NcStreamProto.Group.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(root_);
root_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 40: {
bitField0_ |= 0x00000010;
version_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Header_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Header_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Header.class, ucar.nc2.stream.NcStreamProto.Header.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Header parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Header(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string location = 1;
public static final int LOCATION_FIELD_NUMBER = 1;
private java.lang.Object location_;
/**
* optional string location = 1;
*/
public boolean hasLocation() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string location = 1;
*/
public java.lang.String getLocation() {
java.lang.Object ref = location_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
location_ = s;
}
return s;
}
}
/**
* optional string location = 1;
*/
public com.google.protobuf.ByteString
getLocationBytes() {
java.lang.Object ref = location_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
location_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string title = 2;
public static final int TITLE_FIELD_NUMBER = 2;
private java.lang.Object title_;
/**
* optional string title = 2;
*
*
* ??
*
*/
public boolean hasTitle() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string title = 2;
*
*
* ??
*
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
title_ = s;
}
return s;
}
}
/**
* optional string title = 2;
*
*
* ??
*
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string id = 3;
public static final int ID_FIELD_NUMBER = 3;
private java.lang.Object id_;
/**
* optional string id = 3;
*
*
* ??
*
*/
public boolean hasId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string id = 3;
*
*
* ??
*
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
* optional string id = 3;
*
*
* ??
*
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required .ncstream.Group root = 4;
public static final int ROOT_FIELD_NUMBER = 4;
private ucar.nc2.stream.NcStreamProto.Group root_;
/**
* required .ncstream.Group root = 4;
*/
public boolean hasRoot() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required .ncstream.Group root = 4;
*/
public ucar.nc2.stream.NcStreamProto.Group getRoot() {
return root_;
}
/**
* required .ncstream.Group root = 4;
*/
public ucar.nc2.stream.NcStreamProto.GroupOrBuilder getRootOrBuilder() {
return root_;
}
// optional uint32 version = 5 [default = 0];
public static final int VERSION_FIELD_NUMBER = 5;
private int version_;
/**
* optional uint32 version = 5 [default = 0];
*/
public boolean hasVersion() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional uint32 version = 5 [default = 0];
*/
public int getVersion() {
return version_;
}
private void initFields() {
location_ = "";
title_ = "";
id_ = "";
root_ = ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance();
version_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasRoot()) {
memoizedIsInitialized = 0;
return false;
}
if (!getRoot().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getLocationBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getTitleBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getIdBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, root_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeUInt32(5, version_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getLocationBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getTitleBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getIdBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, root_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, version_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Header parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Header parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Header prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.Header}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.HeaderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Header_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Header_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Header.class, ucar.nc2.stream.NcStreamProto.Header.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.Header.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getRootFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
location_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
title_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
id_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
if (rootBuilder_ == null) {
root_ = ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance();
} else {
rootBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
version_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Header_descriptor;
}
public ucar.nc2.stream.NcStreamProto.Header getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Header.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.Header build() {
ucar.nc2.stream.NcStreamProto.Header result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.Header buildPartial() {
ucar.nc2.stream.NcStreamProto.Header result = new ucar.nc2.stream.NcStreamProto.Header(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.location_ = location_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.title_ = title_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (rootBuilder_ == null) {
result.root_ = root_;
} else {
result.root_ = rootBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.version_ = version_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Header) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Header)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Header other) {
if (other == ucar.nc2.stream.NcStreamProto.Header.getDefaultInstance()) return this;
if (other.hasLocation()) {
bitField0_ |= 0x00000001;
location_ = other.location_;
onChanged();
}
if (other.hasTitle()) {
bitField0_ |= 0x00000002;
title_ = other.title_;
onChanged();
}
if (other.hasId()) {
bitField0_ |= 0x00000004;
id_ = other.id_;
onChanged();
}
if (other.hasRoot()) {
mergeRoot(other.getRoot());
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasRoot()) {
return false;
}
if (!getRoot().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.Header parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.Header) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string location = 1;
private java.lang.Object location_ = "";
/**
* optional string location = 1;
*/
public boolean hasLocation() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string location = 1;
*/
public java.lang.String getLocation() {
java.lang.Object ref = location_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
location_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string location = 1;
*/
public com.google.protobuf.ByteString
getLocationBytes() {
java.lang.Object ref = location_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
location_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string location = 1;
*/
public Builder setLocation(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
location_ = value;
onChanged();
return this;
}
/**
* optional string location = 1;
*/
public Builder clearLocation() {
bitField0_ = (bitField0_ & ~0x00000001);
location_ = getDefaultInstance().getLocation();
onChanged();
return this;
}
/**
* optional string location = 1;
*/
public Builder setLocationBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
location_ = value;
onChanged();
return this;
}
// optional string title = 2;
private java.lang.Object title_ = "";
/**
* optional string title = 2;
*
*
* ??
*
*/
public boolean hasTitle() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string title = 2;
*
*
* ??
*
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string title = 2;
*
*
* ??
*
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string title = 2;
*
*
* ??
*
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
title_ = value;
onChanged();
return this;
}
/**
* optional string title = 2;
*
*
* ??
*
*/
public Builder clearTitle() {
bitField0_ = (bitField0_ & ~0x00000002);
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
* optional string title = 2;
*
*
* ??
*
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
title_ = value;
onChanged();
return this;
}
// optional string id = 3;
private java.lang.Object id_ = "";
/**
* optional string id = 3;
*
*
* ??
*
*/
public boolean hasId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string id = 3;
*
*
* ??
*
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string id = 3;
*
*
* ??
*
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string id = 3;
*
*
* ??
*
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
id_ = value;
onChanged();
return this;
}
/**
* optional string id = 3;
*
*
* ??
*
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000004);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* optional string id = 3;
*
*
* ??
*
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
id_ = value;
onChanged();
return this;
}
// required .ncstream.Group root = 4;
private ucar.nc2.stream.NcStreamProto.Group root_ = ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
ucar.nc2.stream.NcStreamProto.Group, ucar.nc2.stream.NcStreamProto.Group.Builder, ucar.nc2.stream.NcStreamProto.GroupOrBuilder> rootBuilder_;
/**
* required .ncstream.Group root = 4;
*/
public boolean hasRoot() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required .ncstream.Group root = 4;
*/
public ucar.nc2.stream.NcStreamProto.Group getRoot() {
if (rootBuilder_ == null) {
return root_;
} else {
return rootBuilder_.getMessage();
}
}
/**
* required .ncstream.Group root = 4;
*/
public Builder setRoot(ucar.nc2.stream.NcStreamProto.Group value) {
if (rootBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
root_ = value;
onChanged();
} else {
rootBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* required .ncstream.Group root = 4;
*/
public Builder setRoot(
ucar.nc2.stream.NcStreamProto.Group.Builder builderForValue) {
if (rootBuilder_ == null) {
root_ = builderForValue.build();
onChanged();
} else {
rootBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* required .ncstream.Group root = 4;
*/
public Builder mergeRoot(ucar.nc2.stream.NcStreamProto.Group value) {
if (rootBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
root_ != ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance()) {
root_ =
ucar.nc2.stream.NcStreamProto.Group.newBuilder(root_).mergeFrom(value).buildPartial();
} else {
root_ = value;
}
onChanged();
} else {
rootBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* required .ncstream.Group root = 4;
*/
public Builder clearRoot() {
if (rootBuilder_ == null) {
root_ = ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance();
onChanged();
} else {
rootBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* required .ncstream.Group root = 4;
*/
public ucar.nc2.stream.NcStreamProto.Group.Builder getRootBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getRootFieldBuilder().getBuilder();
}
/**
* required .ncstream.Group root = 4;
*/
public ucar.nc2.stream.NcStreamProto.GroupOrBuilder getRootOrBuilder() {
if (rootBuilder_ != null) {
return rootBuilder_.getMessageOrBuilder();
} else {
return root_;
}
}
/**
* required .ncstream.Group root = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
ucar.nc2.stream.NcStreamProto.Group, ucar.nc2.stream.NcStreamProto.Group.Builder, ucar.nc2.stream.NcStreamProto.GroupOrBuilder>
getRootFieldBuilder() {
if (rootBuilder_ == null) {
rootBuilder_ = new com.google.protobuf.SingleFieldBuilder<
ucar.nc2.stream.NcStreamProto.Group, ucar.nc2.stream.NcStreamProto.Group.Builder, ucar.nc2.stream.NcStreamProto.GroupOrBuilder>(
root_,
getParentForChildren(),
isClean());
root_ = null;
}
return rootBuilder_;
}
// optional uint32 version = 5 [default = 0];
private int version_ ;
/**
* optional uint32 version = 5 [default = 0];
*/
public boolean hasVersion() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional uint32 version = 5 [default = 0];
*/
public int getVersion() {
return version_;
}
/**
* optional uint32 version = 5 [default = 0];
*/
public Builder setVersion(int value) {
bitField0_ |= 0x00000010;
version_ = value;
onChanged();
return this;
}
/**
* optional uint32 version = 5 [default = 0];
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000010);
version_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Header)
}
static {
defaultInstance = new Header(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Header)
}
public interface DataOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string varName = 1;
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
boolean hasVarName();
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
java.lang.String getVarName();
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
com.google.protobuf.ByteString
getVarNameBytes();
// required .ncstream.DataType dataType = 2;
/**
* required .ncstream.DataType dataType = 2;
*/
boolean hasDataType();
/**
* required .ncstream.DataType dataType = 2;
*/
ucar.nc2.stream.NcStreamProto.DataType getDataType();
// optional .ncstream.Section section = 3;
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
boolean hasSection();
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
ucar.nc2.stream.NcStreamProto.Section getSection();
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
ucar.nc2.stream.NcStreamProto.SectionOrBuilder getSectionOrBuilder();
// optional bool bigend = 4 [default = true];
/**
* optional bool bigend = 4 [default = true];
*/
boolean hasBigend();
/**
* optional bool bigend = 4 [default = true];
*/
boolean getBigend();
// optional uint32 version = 5 [default = 0];
/**
* optional uint32 version = 5 [default = 0];
*/
boolean hasVersion();
/**
* optional uint32 version = 5 [default = 0];
*/
int getVersion();
// optional .ncstream.Compress compress = 6 [default = NONE];
/**
* optional .ncstream.Compress compress = 6 [default = NONE];
*/
boolean hasCompress();
/**
* optional .ncstream.Compress compress = 6 [default = NONE];
*/
ucar.nc2.stream.NcStreamProto.Compress getCompress();
// optional bool vdata = 7 [default = false];
/**
* optional bool vdata = 7 [default = false];
*/
boolean hasVdata();
/**
* optional bool vdata = 7 [default = false];
*/
boolean getVdata();
// optional uint32 uncompressedSize = 8;
/**
* optional uint32 uncompressedSize = 8;
*/
boolean hasUncompressedSize();
/**
* optional uint32 uncompressedSize = 8;
*/
int getUncompressedSize();
}
/**
* Protobuf type {@code ncstream.Data}
*/
public static final class Data extends
com.google.protobuf.GeneratedMessage
implements DataOrBuilder {
// Use Data.newBuilder() to construct.
private Data(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Data(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Data defaultInstance;
public static Data getDefaultInstance() {
return defaultInstance;
}
public Data getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Data(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
varName_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
ucar.nc2.stream.NcStreamProto.DataType value = ucar.nc2.stream.NcStreamProto.DataType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
dataType_ = value;
}
break;
}
case 26: {
ucar.nc2.stream.NcStreamProto.Section.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = section_.toBuilder();
}
section_ = input.readMessage(ucar.nc2.stream.NcStreamProto.Section.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(section_);
section_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 32: {
bitField0_ |= 0x00000008;
bigend_ = input.readBool();
break;
}
case 40: {
bitField0_ |= 0x00000010;
version_ = input.readUInt32();
break;
}
case 48: {
int rawValue = input.readEnum();
ucar.nc2.stream.NcStreamProto.Compress value = ucar.nc2.stream.NcStreamProto.Compress.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(6, rawValue);
} else {
bitField0_ |= 0x00000020;
compress_ = value;
}
break;
}
case 56: {
bitField0_ |= 0x00000040;
vdata_ = input.readBool();
break;
}
case 64: {
bitField0_ |= 0x00000080;
uncompressedSize_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Data_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Data_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Data.class, ucar.nc2.stream.NcStreamProto.Data.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Data parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Data(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string varName = 1;
public static final int VARNAME_FIELD_NUMBER = 1;
private java.lang.Object varName_;
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
public boolean hasVarName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
public java.lang.String getVarName() {
java.lang.Object ref = varName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
varName_ = s;
}
return s;
}
}
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
public com.google.protobuf.ByteString
getVarNameBytes() {
java.lang.Object ref = varName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
varName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required .ncstream.DataType dataType = 2;
public static final int DATATYPE_FIELD_NUMBER = 2;
private ucar.nc2.stream.NcStreamProto.DataType dataType_;
/**
* required .ncstream.DataType dataType = 2;
*/
public boolean hasDataType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .ncstream.DataType dataType = 2;
*/
public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
return dataType_;
}
// optional .ncstream.Section section = 3;
public static final int SECTION_FIELD_NUMBER = 3;
private ucar.nc2.stream.NcStreamProto.Section section_;
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
public boolean hasSection() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
public ucar.nc2.stream.NcStreamProto.Section getSection() {
return section_;
}
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
public ucar.nc2.stream.NcStreamProto.SectionOrBuilder getSectionOrBuilder() {
return section_;
}
// optional bool bigend = 4 [default = true];
public static final int BIGEND_FIELD_NUMBER = 4;
private boolean bigend_;
/**
* optional bool bigend = 4 [default = true];
*/
public boolean hasBigend() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool bigend = 4 [default = true];
*/
public boolean getBigend() {
return bigend_;
}
// optional uint32 version = 5 [default = 0];
public static final int VERSION_FIELD_NUMBER = 5;
private int version_;
/**
* optional uint32 version = 5 [default = 0];
*/
public boolean hasVersion() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional uint32 version = 5 [default = 0];
*/
public int getVersion() {
return version_;
}
// optional .ncstream.Compress compress = 6 [default = NONE];
public static final int COMPRESS_FIELD_NUMBER = 6;
private ucar.nc2.stream.NcStreamProto.Compress compress_;
/**
* optional .ncstream.Compress compress = 6 [default = NONE];
*/
public boolean hasCompress() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .ncstream.Compress compress = 6 [default = NONE];
*/
public ucar.nc2.stream.NcStreamProto.Compress getCompress() {
return compress_;
}
// optional bool vdata = 7 [default = false];
public static final int VDATA_FIELD_NUMBER = 7;
private boolean vdata_;
/**
* optional bool vdata = 7 [default = false];
*/
public boolean hasVdata() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool vdata = 7 [default = false];
*/
public boolean getVdata() {
return vdata_;
}
// optional uint32 uncompressedSize = 8;
public static final int UNCOMPRESSEDSIZE_FIELD_NUMBER = 8;
private int uncompressedSize_;
/**
* optional uint32 uncompressedSize = 8;
*/
public boolean hasUncompressedSize() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional uint32 uncompressedSize = 8;
*/
public int getUncompressedSize() {
return uncompressedSize_;
}
private void initFields() {
varName_ = "";
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
section_ = ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance();
bigend_ = true;
version_ = 0;
compress_ = ucar.nc2.stream.NcStreamProto.Compress.NONE;
vdata_ = false;
uncompressedSize_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasVarName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasDataType()) {
memoizedIsInitialized = 0;
return false;
}
if (hasSection()) {
if (!getSection().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getVarNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, dataType_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, section_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(4, bigend_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeUInt32(5, version_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeEnum(6, compress_.getNumber());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBool(7, vdata_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeUInt32(8, uncompressedSize_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getVarNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, dataType_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, section_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, bigend_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, version_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, compress_.getNumber());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, vdata_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(8, uncompressedSize_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Data parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Data parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Data prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.Data}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.DataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Data_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Data_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Data.class, ucar.nc2.stream.NcStreamProto.Data.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.Data.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getSectionFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
varName_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
bitField0_ = (bitField0_ & ~0x00000002);
if (sectionBuilder_ == null) {
section_ = ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance();
} else {
sectionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
bigend_ = true;
bitField0_ = (bitField0_ & ~0x00000008);
version_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
compress_ = ucar.nc2.stream.NcStreamProto.Compress.NONE;
bitField0_ = (bitField0_ & ~0x00000020);
vdata_ = false;
bitField0_ = (bitField0_ & ~0x00000040);
uncompressedSize_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Data_descriptor;
}
public ucar.nc2.stream.NcStreamProto.Data getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Data.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.Data build() {
ucar.nc2.stream.NcStreamProto.Data result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.Data buildPartial() {
ucar.nc2.stream.NcStreamProto.Data result = new ucar.nc2.stream.NcStreamProto.Data(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.varName_ = varName_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.dataType_ = dataType_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (sectionBuilder_ == null) {
result.section_ = section_;
} else {
result.section_ = sectionBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.bigend_ = bigend_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.version_ = version_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.compress_ = compress_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.vdata_ = vdata_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.uncompressedSize_ = uncompressedSize_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Data) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Data)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Data other) {
if (other == ucar.nc2.stream.NcStreamProto.Data.getDefaultInstance()) return this;
if (other.hasVarName()) {
bitField0_ |= 0x00000001;
varName_ = other.varName_;
onChanged();
}
if (other.hasDataType()) {
setDataType(other.getDataType());
}
if (other.hasSection()) {
mergeSection(other.getSection());
}
if (other.hasBigend()) {
setBigend(other.getBigend());
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
if (other.hasCompress()) {
setCompress(other.getCompress());
}
if (other.hasVdata()) {
setVdata(other.getVdata());
}
if (other.hasUncompressedSize()) {
setUncompressedSize(other.getUncompressedSize());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasVarName()) {
return false;
}
if (!hasDataType()) {
return false;
}
if (hasSection()) {
if (!getSection().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.Data parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.Data) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string varName = 1;
private java.lang.Object varName_ = "";
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
public boolean hasVarName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
public java.lang.String getVarName() {
java.lang.Object ref = varName_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
varName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
public com.google.protobuf.ByteString
getVarNameBytes() {
java.lang.Object ref = varName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
varName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
public Builder setVarName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
varName_ = value;
onChanged();
return this;
}
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
public Builder clearVarName() {
bitField0_ = (bitField0_ & ~0x00000001);
varName_ = getDefaultInstance().getVarName();
onChanged();
return this;
}
/**
* required string varName = 1;
*
*
* full escaped name. change to hash or index to save space ??
*
*/
public Builder setVarNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
varName_ = value;
onChanged();
return this;
}
// required .ncstream.DataType dataType = 2;
private ucar.nc2.stream.NcStreamProto.DataType dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
/**
* required .ncstream.DataType dataType = 2;
*/
public boolean hasDataType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .ncstream.DataType dataType = 2;
*/
public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
return dataType_;
}
/**
* required .ncstream.DataType dataType = 2;
*/
public Builder setDataType(ucar.nc2.stream.NcStreamProto.DataType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
dataType_ = value;
onChanged();
return this;
}
/**
* required .ncstream.DataType dataType = 2;
*/
public Builder clearDataType() {
bitField0_ = (bitField0_ & ~0x00000002);
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
onChanged();
return this;
}
// optional .ncstream.Section section = 3;
private ucar.nc2.stream.NcStreamProto.Section section_ = ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
ucar.nc2.stream.NcStreamProto.Section, ucar.nc2.stream.NcStreamProto.Section.Builder, ucar.nc2.stream.NcStreamProto.SectionOrBuilder> sectionBuilder_;
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
public boolean hasSection() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
public ucar.nc2.stream.NcStreamProto.Section getSection() {
if (sectionBuilder_ == null) {
return section_;
} else {
return sectionBuilder_.getMessage();
}
}
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
public Builder setSection(ucar.nc2.stream.NcStreamProto.Section value) {
if (sectionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
section_ = value;
onChanged();
} else {
sectionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
public Builder setSection(
ucar.nc2.stream.NcStreamProto.Section.Builder builderForValue) {
if (sectionBuilder_ == null) {
section_ = builderForValue.build();
onChanged();
} else {
sectionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
public Builder mergeSection(ucar.nc2.stream.NcStreamProto.Section value) {
if (sectionBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
section_ != ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance()) {
section_ =
ucar.nc2.stream.NcStreamProto.Section.newBuilder(section_).mergeFrom(value).buildPartial();
} else {
section_ = value;
}
onChanged();
} else {
sectionBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
public Builder clearSection() {
if (sectionBuilder_ == null) {
section_ = ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance();
onChanged();
} else {
sectionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
public ucar.nc2.stream.NcStreamProto.Section.Builder getSectionBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getSectionFieldBuilder().getBuilder();
}
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
public ucar.nc2.stream.NcStreamProto.SectionOrBuilder getSectionOrBuilder() {
if (sectionBuilder_ != null) {
return sectionBuilder_.getMessageOrBuilder();
} else {
return section_;
}
}
/**
* optional .ncstream.Section section = 3;
*
*
* not required for Sequence
*
*/
private com.google.protobuf.SingleFieldBuilder<
ucar.nc2.stream.NcStreamProto.Section, ucar.nc2.stream.NcStreamProto.Section.Builder, ucar.nc2.stream.NcStreamProto.SectionOrBuilder>
getSectionFieldBuilder() {
if (sectionBuilder_ == null) {
sectionBuilder_ = new com.google.protobuf.SingleFieldBuilder<
ucar.nc2.stream.NcStreamProto.Section, ucar.nc2.stream.NcStreamProto.Section.Builder, ucar.nc2.stream.NcStreamProto.SectionOrBuilder>(
section_,
getParentForChildren(),
isClean());
section_ = null;
}
return sectionBuilder_;
}
// optional bool bigend = 4 [default = true];
private boolean bigend_ = true;
/**
* optional bool bigend = 4 [default = true];
*/
public boolean hasBigend() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool bigend = 4 [default = true];
*/
public boolean getBigend() {
return bigend_;
}
/**
* optional bool bigend = 4 [default = true];
*/
public Builder setBigend(boolean value) {
bitField0_ |= 0x00000008;
bigend_ = value;
onChanged();
return this;
}
/**
* optional bool bigend = 4 [default = true];
*/
public Builder clearBigend() {
bitField0_ = (bitField0_ & ~0x00000008);
bigend_ = true;
onChanged();
return this;
}
// optional uint32 version = 5 [default = 0];
private int version_ ;
/**
* optional uint32 version = 5 [default = 0];
*/
public boolean hasVersion() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional uint32 version = 5 [default = 0];
*/
public int getVersion() {
return version_;
}
/**
* optional uint32 version = 5 [default = 0];
*/
public Builder setVersion(int value) {
bitField0_ |= 0x00000010;
version_ = value;
onChanged();
return this;
}
/**
* optional uint32 version = 5 [default = 0];
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000010);
version_ = 0;
onChanged();
return this;
}
// optional .ncstream.Compress compress = 6 [default = NONE];
private ucar.nc2.stream.NcStreamProto.Compress compress_ = ucar.nc2.stream.NcStreamProto.Compress.NONE;
/**
* optional .ncstream.Compress compress = 6 [default = NONE];
*/
public boolean hasCompress() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .ncstream.Compress compress = 6 [default = NONE];
*/
public ucar.nc2.stream.NcStreamProto.Compress getCompress() {
return compress_;
}
/**
* optional .ncstream.Compress compress = 6 [default = NONE];
*/
public Builder setCompress(ucar.nc2.stream.NcStreamProto.Compress value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
compress_ = value;
onChanged();
return this;
}
/**
* optional .ncstream.Compress compress = 6 [default = NONE];
*/
public Builder clearCompress() {
bitField0_ = (bitField0_ & ~0x00000020);
compress_ = ucar.nc2.stream.NcStreamProto.Compress.NONE;
onChanged();
return this;
}
// optional bool vdata = 7 [default = false];
private boolean vdata_ ;
/**
* optional bool vdata = 7 [default = false];
*/
public boolean hasVdata() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool vdata = 7 [default = false];
*/
public boolean getVdata() {
return vdata_;
}
/**
* optional bool vdata = 7 [default = false];
*/
public Builder setVdata(boolean value) {
bitField0_ |= 0x00000040;
vdata_ = value;
onChanged();
return this;
}
/**
* optional bool vdata = 7 [default = false];
*/
public Builder clearVdata() {
bitField0_ = (bitField0_ & ~0x00000040);
vdata_ = false;
onChanged();
return this;
}
// optional uint32 uncompressedSize = 8;
private int uncompressedSize_ ;
/**
* optional uint32 uncompressedSize = 8;
*/
public boolean hasUncompressedSize() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional uint32 uncompressedSize = 8;
*/
public int getUncompressedSize() {
return uncompressedSize_;
}
/**
* optional uint32 uncompressedSize = 8;
*/
public Builder setUncompressedSize(int value) {
bitField0_ |= 0x00000080;
uncompressedSize_ = value;
onChanged();
return this;
}
/**
* optional uint32 uncompressedSize = 8;
*/
public Builder clearUncompressedSize() {
bitField0_ = (bitField0_ & ~0x00000080);
uncompressedSize_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Data)
}
static {
defaultInstance = new Data(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Data)
}
public interface RangeOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint64 start = 1 [default = 0];
/**
* optional uint64 start = 1 [default = 0];
*/
boolean hasStart();
/**
* optional uint64 start = 1 [default = 0];
*/
long getStart();
// required uint64 size = 2;
/**
* required uint64 size = 2;
*/
boolean hasSize();
/**
* required uint64 size = 2;
*/
long getSize();
// optional uint64 stride = 3 [default = 1];
/**
* optional uint64 stride = 3 [default = 1];
*/
boolean hasStride();
/**
* optional uint64 stride = 3 [default = 1];
*/
long getStride();
}
/**
* Protobuf type {@code ncstream.Range}
*/
public static final class Range extends
com.google.protobuf.GeneratedMessage
implements RangeOrBuilder {
// Use Range.newBuilder() to construct.
private Range(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Range(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Range defaultInstance;
public static Range getDefaultInstance() {
return defaultInstance;
}
public Range getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Range(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
start_ = input.readUInt64();
break;
}
case 16: {
bitField0_ |= 0x00000002;
size_ = input.readUInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
stride_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Range_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Range_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Range.class, ucar.nc2.stream.NcStreamProto.Range.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Range parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Range(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional uint64 start = 1 [default = 0];
public static final int START_FIELD_NUMBER = 1;
private long start_;
/**
* optional uint64 start = 1 [default = 0];
*/
public boolean hasStart() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint64 start = 1 [default = 0];
*/
public long getStart() {
return start_;
}
// required uint64 size = 2;
public static final int SIZE_FIELD_NUMBER = 2;
private long size_;
/**
* required uint64 size = 2;
*/
public boolean hasSize() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint64 size = 2;
*/
public long getSize() {
return size_;
}
// optional uint64 stride = 3 [default = 1];
public static final int STRIDE_FIELD_NUMBER = 3;
private long stride_;
/**
* optional uint64 stride = 3 [default = 1];
*/
public boolean hasStride() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 stride = 3 [default = 1];
*/
public long getStride() {
return stride_;
}
private void initFields() {
start_ = 0L;
size_ = 0L;
stride_ = 1L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasSize()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt64(1, start_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt64(2, size_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt64(3, stride_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, start_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, size_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, stride_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Range parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Range parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Range prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.Range}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.RangeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Range_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Range_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Range.class, ucar.nc2.stream.NcStreamProto.Range.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.Range.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
start_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
size_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
stride_ = 1L;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Range_descriptor;
}
public ucar.nc2.stream.NcStreamProto.Range getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Range.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.Range build() {
ucar.nc2.stream.NcStreamProto.Range result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.Range buildPartial() {
ucar.nc2.stream.NcStreamProto.Range result = new ucar.nc2.stream.NcStreamProto.Range(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.start_ = start_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.size_ = size_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.stride_ = stride_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Range) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Range)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Range other) {
if (other == ucar.nc2.stream.NcStreamProto.Range.getDefaultInstance()) return this;
if (other.hasStart()) {
setStart(other.getStart());
}
if (other.hasSize()) {
setSize(other.getSize());
}
if (other.hasStride()) {
setStride(other.getStride());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasSize()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.Range parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.Range) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional uint64 start = 1 [default = 0];
private long start_ ;
/**
* optional uint64 start = 1 [default = 0];
*/
public boolean hasStart() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint64 start = 1 [default = 0];
*/
public long getStart() {
return start_;
}
/**
* optional uint64 start = 1 [default = 0];
*/
public Builder setStart(long value) {
bitField0_ |= 0x00000001;
start_ = value;
onChanged();
return this;
}
/**
* optional uint64 start = 1 [default = 0];
*/
public Builder clearStart() {
bitField0_ = (bitField0_ & ~0x00000001);
start_ = 0L;
onChanged();
return this;
}
// required uint64 size = 2;
private long size_ ;
/**
* required uint64 size = 2;
*/
public boolean hasSize() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint64 size = 2;
*/
public long getSize() {
return size_;
}
/**
* required uint64 size = 2;
*/
public Builder setSize(long value) {
bitField0_ |= 0x00000002;
size_ = value;
onChanged();
return this;
}
/**
* required uint64 size = 2;
*/
public Builder clearSize() {
bitField0_ = (bitField0_ & ~0x00000002);
size_ = 0L;
onChanged();
return this;
}
// optional uint64 stride = 3 [default = 1];
private long stride_ = 1L;
/**
* optional uint64 stride = 3 [default = 1];
*/
public boolean hasStride() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 stride = 3 [default = 1];
*/
public long getStride() {
return stride_;
}
/**
* optional uint64 stride = 3 [default = 1];
*/
public Builder setStride(long value) {
bitField0_ |= 0x00000004;
stride_ = value;
onChanged();
return this;
}
/**
* optional uint64 stride = 3 [default = 1];
*/
public Builder clearStride() {
bitField0_ = (bitField0_ & ~0x00000004);
stride_ = 1L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Range)
}
static {
defaultInstance = new Range(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Range)
}
public interface SectionOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated .ncstream.Range range = 1;
/**
* repeated .ncstream.Range range = 1;
*/
java.util.List
getRangeList();
/**
* repeated .ncstream.Range range = 1;
*/
ucar.nc2.stream.NcStreamProto.Range getRange(int index);
/**
* repeated .ncstream.Range range = 1;
*/
int getRangeCount();
/**
* repeated .ncstream.Range range = 1;
*/
java.util.List extends ucar.nc2.stream.NcStreamProto.RangeOrBuilder>
getRangeOrBuilderList();
/**
* repeated .ncstream.Range range = 1;
*/
ucar.nc2.stream.NcStreamProto.RangeOrBuilder getRangeOrBuilder(
int index);
}
/**
* Protobuf type {@code ncstream.Section}
*/
public static final class Section extends
com.google.protobuf.GeneratedMessage
implements SectionOrBuilder {
// Use Section.newBuilder() to construct.
private Section(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Section(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Section defaultInstance;
public static Section getDefaultInstance() {
return defaultInstance;
}
public Section getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Section(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
range_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
range_.add(input.readMessage(ucar.nc2.stream.NcStreamProto.Range.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
range_ = java.util.Collections.unmodifiableList(range_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Section_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Section_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Section.class, ucar.nc2.stream.NcStreamProto.Section.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Section parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Section(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
// repeated .ncstream.Range range = 1;
public static final int RANGE_FIELD_NUMBER = 1;
private java.util.List range_;
/**
* repeated .ncstream.Range range = 1;
*/
public java.util.List getRangeList() {
return range_;
}
/**
* repeated .ncstream.Range range = 1;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.RangeOrBuilder>
getRangeOrBuilderList() {
return range_;
}
/**
* repeated .ncstream.Range range = 1;
*/
public int getRangeCount() {
return range_.size();
}
/**
* repeated .ncstream.Range range = 1;
*/
public ucar.nc2.stream.NcStreamProto.Range getRange(int index) {
return range_.get(index);
}
/**
* repeated .ncstream.Range range = 1;
*/
public ucar.nc2.stream.NcStreamProto.RangeOrBuilder getRangeOrBuilder(
int index) {
return range_.get(index);
}
private void initFields() {
range_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getRangeCount(); i++) {
if (!getRange(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < range_.size(); i++) {
output.writeMessage(1, range_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < range_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, range_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Section parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Section parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Section prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.Section}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.SectionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Section_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Section_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Section.class, ucar.nc2.stream.NcStreamProto.Section.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.Section.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getRangeFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (rangeBuilder_ == null) {
range_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
rangeBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Section_descriptor;
}
public ucar.nc2.stream.NcStreamProto.Section getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.Section build() {
ucar.nc2.stream.NcStreamProto.Section result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.Section buildPartial() {
ucar.nc2.stream.NcStreamProto.Section result = new ucar.nc2.stream.NcStreamProto.Section(this);
int from_bitField0_ = bitField0_;
if (rangeBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
range_ = java.util.Collections.unmodifiableList(range_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.range_ = range_;
} else {
result.range_ = rangeBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Section) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Section)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Section other) {
if (other == ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance()) return this;
if (rangeBuilder_ == null) {
if (!other.range_.isEmpty()) {
if (range_.isEmpty()) {
range_ = other.range_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRangeIsMutable();
range_.addAll(other.range_);
}
onChanged();
}
} else {
if (!other.range_.isEmpty()) {
if (rangeBuilder_.isEmpty()) {
rangeBuilder_.dispose();
rangeBuilder_ = null;
range_ = other.range_;
bitField0_ = (bitField0_ & ~0x00000001);
rangeBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getRangeFieldBuilder() : null;
} else {
rangeBuilder_.addAllMessages(other.range_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getRangeCount(); i++) {
if (!getRange(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.Section parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.Section) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .ncstream.Range range = 1;
private java.util.List range_ =
java.util.Collections.emptyList();
private void ensureRangeIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
range_ = new java.util.ArrayList(range_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Range, ucar.nc2.stream.NcStreamProto.Range.Builder, ucar.nc2.stream.NcStreamProto.RangeOrBuilder> rangeBuilder_;
/**
* repeated .ncstream.Range range = 1;
*/
public java.util.List getRangeList() {
if (rangeBuilder_ == null) {
return java.util.Collections.unmodifiableList(range_);
} else {
return rangeBuilder_.getMessageList();
}
}
/**
* repeated .ncstream.Range range = 1;
*/
public int getRangeCount() {
if (rangeBuilder_ == null) {
return range_.size();
} else {
return rangeBuilder_.getCount();
}
}
/**
* repeated .ncstream.Range range = 1;
*/
public ucar.nc2.stream.NcStreamProto.Range getRange(int index) {
if (rangeBuilder_ == null) {
return range_.get(index);
} else {
return rangeBuilder_.getMessage(index);
}
}
/**
* repeated .ncstream.Range range = 1;
*/
public Builder setRange(
int index, ucar.nc2.stream.NcStreamProto.Range value) {
if (rangeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRangeIsMutable();
range_.set(index, value);
onChanged();
} else {
rangeBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Range range = 1;
*/
public Builder setRange(
int index, ucar.nc2.stream.NcStreamProto.Range.Builder builderForValue) {
if (rangeBuilder_ == null) {
ensureRangeIsMutable();
range_.set(index, builderForValue.build());
onChanged();
} else {
rangeBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Range range = 1;
*/
public Builder addRange(ucar.nc2.stream.NcStreamProto.Range value) {
if (rangeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRangeIsMutable();
range_.add(value);
onChanged();
} else {
rangeBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ncstream.Range range = 1;
*/
public Builder addRange(
int index, ucar.nc2.stream.NcStreamProto.Range value) {
if (rangeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRangeIsMutable();
range_.add(index, value);
onChanged();
} else {
rangeBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ncstream.Range range = 1;
*/
public Builder addRange(
ucar.nc2.stream.NcStreamProto.Range.Builder builderForValue) {
if (rangeBuilder_ == null) {
ensureRangeIsMutable();
range_.add(builderForValue.build());
onChanged();
} else {
rangeBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Range range = 1;
*/
public Builder addRange(
int index, ucar.nc2.stream.NcStreamProto.Range.Builder builderForValue) {
if (rangeBuilder_ == null) {
ensureRangeIsMutable();
range_.add(index, builderForValue.build());
onChanged();
} else {
rangeBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ncstream.Range range = 1;
*/
public Builder addAllRange(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Range> values) {
if (rangeBuilder_ == null) {
ensureRangeIsMutable();
super.addAll(values, range_);
onChanged();
} else {
rangeBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ncstream.Range range = 1;
*/
public Builder clearRange() {
if (rangeBuilder_ == null) {
range_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
rangeBuilder_.clear();
}
return this;
}
/**
* repeated .ncstream.Range range = 1;
*/
public Builder removeRange(int index) {
if (rangeBuilder_ == null) {
ensureRangeIsMutable();
range_.remove(index);
onChanged();
} else {
rangeBuilder_.remove(index);
}
return this;
}
/**
* repeated .ncstream.Range range = 1;
*/
public ucar.nc2.stream.NcStreamProto.Range.Builder getRangeBuilder(
int index) {
return getRangeFieldBuilder().getBuilder(index);
}
/**
* repeated .ncstream.Range range = 1;
*/
public ucar.nc2.stream.NcStreamProto.RangeOrBuilder getRangeOrBuilder(
int index) {
if (rangeBuilder_ == null) {
return range_.get(index); } else {
return rangeBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ncstream.Range range = 1;
*/
public java.util.List extends ucar.nc2.stream.NcStreamProto.RangeOrBuilder>
getRangeOrBuilderList() {
if (rangeBuilder_ != null) {
return rangeBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(range_);
}
}
/**
* repeated .ncstream.Range range = 1;
*/
public ucar.nc2.stream.NcStreamProto.Range.Builder addRangeBuilder() {
return getRangeFieldBuilder().addBuilder(
ucar.nc2.stream.NcStreamProto.Range.getDefaultInstance());
}
/**
* repeated .ncstream.Range range = 1;
*/
public ucar.nc2.stream.NcStreamProto.Range.Builder addRangeBuilder(
int index) {
return getRangeFieldBuilder().addBuilder(
index, ucar.nc2.stream.NcStreamProto.Range.getDefaultInstance());
}
/**
* repeated .ncstream.Range range = 1;
*/
public java.util.List
getRangeBuilderList() {
return getRangeFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Range, ucar.nc2.stream.NcStreamProto.Range.Builder, ucar.nc2.stream.NcStreamProto.RangeOrBuilder>
getRangeFieldBuilder() {
if (rangeBuilder_ == null) {
rangeBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
ucar.nc2.stream.NcStreamProto.Range, ucar.nc2.stream.NcStreamProto.Range.Builder, ucar.nc2.stream.NcStreamProto.RangeOrBuilder>(
range_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
range_ = null;
}
return rangeBuilder_;
}
// @@protoc_insertion_point(builder_scope:ncstream.Section)
}
static {
defaultInstance = new Section(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Section)
}
public interface StructureDataOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated uint32 member = 1;
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
java.util.List getMemberList();
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
int getMemberCount();
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
int getMember(int index);
// required bytes data = 2;
/**
* required bytes data = 2;
*
*
* fixed length data
*
*/
boolean hasData();
/**
* required bytes data = 2;
*
*
* fixed length data
*
*/
com.google.protobuf.ByteString getData();
// repeated uint32 heapCount = 3;
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
java.util.List getHeapCountList();
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
int getHeapCountCount();
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
int getHeapCount(int index);
// repeated string sdata = 4;
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
java.util.List
getSdataList();
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
int getSdataCount();
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
java.lang.String getSdata(int index);
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
com.google.protobuf.ByteString
getSdataBytes(int index);
// optional uint64 nrows = 5 [default = 1];
/**
* optional uint64 nrows = 5 [default = 1];
*/
boolean hasNrows();
/**
* optional uint64 nrows = 5 [default = 1];
*/
long getNrows();
}
/**
* Protobuf type {@code ncstream.StructureData}
*/
public static final class StructureData extends
com.google.protobuf.GeneratedMessage
implements StructureDataOrBuilder {
// Use StructureData.newBuilder() to construct.
private StructureData(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private StructureData(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final StructureData defaultInstance;
public static StructureData getDefaultInstance() {
return defaultInstance;
}
public StructureData getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StructureData(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
member_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
member_.add(input.readUInt32());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001) && input.getBytesUntilLimit() > 0) {
member_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
member_.add(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 18: {
bitField0_ |= 0x00000001;
data_ = input.readBytes();
break;
}
case 24: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
heapCount_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
heapCount_.add(input.readUInt32());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004) && input.getBytesUntilLimit() > 0) {
heapCount_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
while (input.getBytesUntilLimit() > 0) {
heapCount_.add(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
sdata_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000008;
}
sdata_.add(input.readBytes());
break;
}
case 40: {
bitField0_ |= 0x00000002;
nrows_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
member_ = java.util.Collections.unmodifiableList(member_);
}
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
heapCount_ = java.util.Collections.unmodifiableList(heapCount_);
}
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
sdata_ = new com.google.protobuf.UnmodifiableLazyStringList(sdata_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_StructureData_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_StructureData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.StructureData.class, ucar.nc2.stream.NcStreamProto.StructureData.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public StructureData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StructureData(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// repeated uint32 member = 1;
public static final int MEMBER_FIELD_NUMBER = 1;
private java.util.List member_;
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
public java.util.List
getMemberList() {
return member_;
}
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
public int getMemberCount() {
return member_.size();
}
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
public int getMember(int index) {
return member_.get(index);
}
// required bytes data = 2;
public static final int DATA_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString data_;
/**
* required bytes data = 2;
*
*
* fixed length data
*
*/
public boolean hasData() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes data = 2;
*
*
* fixed length data
*
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
// repeated uint32 heapCount = 3;
public static final int HEAPCOUNT_FIELD_NUMBER = 3;
private java.util.List heapCount_;
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
public java.util.List
getHeapCountList() {
return heapCount_;
}
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
public int getHeapCountCount() {
return heapCount_.size();
}
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
public int getHeapCount(int index) {
return heapCount_.get(index);
}
// repeated string sdata = 4;
public static final int SDATA_FIELD_NUMBER = 4;
private com.google.protobuf.LazyStringList sdata_;
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public java.util.List
getSdataList() {
return sdata_;
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public int getSdataCount() {
return sdata_.size();
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public java.lang.String getSdata(int index) {
return sdata_.get(index);
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public com.google.protobuf.ByteString
getSdataBytes(int index) {
return sdata_.getByteString(index);
}
// optional uint64 nrows = 5 [default = 1];
public static final int NROWS_FIELD_NUMBER = 5;
private long nrows_;
/**
* optional uint64 nrows = 5 [default = 1];
*/
public boolean hasNrows() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint64 nrows = 5 [default = 1];
*/
public long getNrows() {
return nrows_;
}
private void initFields() {
member_ = java.util.Collections.emptyList();
data_ = com.google.protobuf.ByteString.EMPTY;
heapCount_ = java.util.Collections.emptyList();
sdata_ = com.google.protobuf.LazyStringArrayList.EMPTY;
nrows_ = 1L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasData()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < member_.size(); i++) {
output.writeUInt32(1, member_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(2, data_);
}
for (int i = 0; i < heapCount_.size(); i++) {
output.writeUInt32(3, heapCount_.get(i));
}
for (int i = 0; i < sdata_.size(); i++) {
output.writeBytes(4, sdata_.getByteString(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt64(5, nrows_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < member_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(member_.get(i));
}
size += dataSize;
size += 1 * getMemberList().size();
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, data_);
}
{
int dataSize = 0;
for (int i = 0; i < heapCount_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(heapCount_.get(i));
}
size += dataSize;
size += 1 * getHeapCountList().size();
}
{
int dataSize = 0;
for (int i = 0; i < sdata_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(sdata_.getByteString(i));
}
size += dataSize;
size += 1 * getSdataList().size();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, nrows_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.StructureData prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.StructureData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.StructureDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_StructureData_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_StructureData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.StructureData.class, ucar.nc2.stream.NcStreamProto.StructureData.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.StructureData.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
member_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
data_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
heapCount_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
sdata_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
nrows_ = 1L;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_StructureData_descriptor;
}
public ucar.nc2.stream.NcStreamProto.StructureData getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.StructureData.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.StructureData build() {
ucar.nc2.stream.NcStreamProto.StructureData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.StructureData buildPartial() {
ucar.nc2.stream.NcStreamProto.StructureData result = new ucar.nc2.stream.NcStreamProto.StructureData(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
member_ = java.util.Collections.unmodifiableList(member_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.member_ = member_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.data_ = data_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
heapCount_ = java.util.Collections.unmodifiableList(heapCount_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.heapCount_ = heapCount_;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
sdata_ = new com.google.protobuf.UnmodifiableLazyStringList(
sdata_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.sdata_ = sdata_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000002;
}
result.nrows_ = nrows_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.StructureData) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.StructureData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.StructureData other) {
if (other == ucar.nc2.stream.NcStreamProto.StructureData.getDefaultInstance()) return this;
if (!other.member_.isEmpty()) {
if (member_.isEmpty()) {
member_ = other.member_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureMemberIsMutable();
member_.addAll(other.member_);
}
onChanged();
}
if (other.hasData()) {
setData(other.getData());
}
if (!other.heapCount_.isEmpty()) {
if (heapCount_.isEmpty()) {
heapCount_ = other.heapCount_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureHeapCountIsMutable();
heapCount_.addAll(other.heapCount_);
}
onChanged();
}
if (!other.sdata_.isEmpty()) {
if (sdata_.isEmpty()) {
sdata_ = other.sdata_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureSdataIsMutable();
sdata_.addAll(other.sdata_);
}
onChanged();
}
if (other.hasNrows()) {
setNrows(other.getNrows());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasData()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.StructureData parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.StructureData) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated uint32 member = 1;
private java.util.List member_ = java.util.Collections.emptyList();
private void ensureMemberIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
member_ = new java.util.ArrayList(member_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
public java.util.List
getMemberList() {
return java.util.Collections.unmodifiableList(member_);
}
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
public int getMemberCount() {
return member_.size();
}
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
public int getMember(int index) {
return member_.get(index);
}
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
public Builder setMember(
int index, int value) {
ensureMemberIsMutable();
member_.set(index, value);
onChanged();
return this;
}
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
public Builder addMember(int value) {
ensureMemberIsMutable();
member_.add(value);
onChanged();
return this;
}
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
public Builder addAllMember(
java.lang.Iterable extends java.lang.Integer> values) {
ensureMemberIsMutable();
super.addAll(values, member_);
onChanged();
return this;
}
/**
* repeated uint32 member = 1;
*
*
* optional list of members present
*
*/
public Builder clearMember() {
member_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
// required bytes data = 2;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes data = 2;
*
*
* fixed length data
*
*/
public boolean hasData() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes data = 2;
*
*
* fixed length data
*
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* required bytes data = 2;
*
*
* fixed length data
*
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
data_ = value;
onChanged();
return this;
}
/**
* required bytes data = 2;
*
*
* fixed length data
*
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000002);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
// repeated uint32 heapCount = 3;
private java.util.List heapCount_ = java.util.Collections.emptyList();
private void ensureHeapCountIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
heapCount_ = new java.util.ArrayList(heapCount_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
public java.util.List
getHeapCountList() {
return java.util.Collections.unmodifiableList(heapCount_);
}
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
public int getHeapCountCount() {
return heapCount_.size();
}
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
public int getHeapCount(int index) {
return heapCount_.get(index);
}
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
public Builder setHeapCount(
int index, int value) {
ensureHeapCountIsMutable();
heapCount_.set(index, value);
onChanged();
return this;
}
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
public Builder addHeapCount(int value) {
ensureHeapCountIsMutable();
heapCount_.add(value);
onChanged();
return this;
}
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
public Builder addAllHeapCount(
java.lang.Iterable extends java.lang.Integer> values) {
ensureHeapCountIsMutable();
super.addAll(values, heapCount_);
onChanged();
return this;
}
/**
* repeated uint32 heapCount = 3;
*
*
* heap String count
*
*/
public Builder clearHeapCount() {
heapCount_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
// repeated string sdata = 4;
private com.google.protobuf.LazyStringList sdata_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSdataIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
sdata_ = new com.google.protobuf.LazyStringArrayList(sdata_);
bitField0_ |= 0x00000008;
}
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public java.util.List
getSdataList() {
return java.util.Collections.unmodifiableList(sdata_);
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public int getSdataCount() {
return sdata_.size();
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public java.lang.String getSdata(int index) {
return sdata_.get(index);
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public com.google.protobuf.ByteString
getSdataBytes(int index) {
return sdata_.getByteString(index);
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public Builder setSdata(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSdataIsMutable();
sdata_.set(index, value);
onChanged();
return this;
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public Builder addSdata(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSdataIsMutable();
sdata_.add(value);
onChanged();
return this;
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public Builder addAllSdata(
java.lang.Iterable values) {
ensureSdataIsMutable();
super.addAll(values, sdata_);
onChanged();
return this;
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public Builder clearSdata() {
sdata_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* repeated string sdata = 4;
*
*
* Strings
*
*/
public Builder addSdataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSdataIsMutable();
sdata_.add(value);
onChanged();
return this;
}
// optional uint64 nrows = 5 [default = 1];
private long nrows_ = 1L;
/**
* optional uint64 nrows = 5 [default = 1];
*/
public boolean hasNrows() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional uint64 nrows = 5 [default = 1];
*/
public long getNrows() {
return nrows_;
}
/**
* optional uint64 nrows = 5 [default = 1];
*/
public Builder setNrows(long value) {
bitField0_ |= 0x00000010;
nrows_ = value;
onChanged();
return this;
}
/**
* optional uint64 nrows = 5 [default = 1];
*/
public Builder clearNrows() {
bitField0_ = (bitField0_ & ~0x00000010);
nrows_ = 1L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.StructureData)
}
static {
defaultInstance = new StructureData(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.StructureData)
}
public interface ErrorOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string message = 1;
/**
* required string message = 1;
*/
boolean hasMessage();
/**
* required string message = 1;
*/
java.lang.String getMessage();
/**
* required string message = 1;
*/
com.google.protobuf.ByteString
getMessageBytes();
}
/**
* Protobuf type {@code ncstream.Error}
*/
public static final class Error extends
com.google.protobuf.GeneratedMessage
implements ErrorOrBuilder {
// Use Error.newBuilder() to construct.
private Error(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Error(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Error defaultInstance;
public static Error getDefaultInstance() {
return defaultInstance;
}
public Error getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Error(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
message_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Error_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Error_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Error.class, ucar.nc2.stream.NcStreamProto.Error.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Error parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Error(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string message = 1;
public static final int MESSAGE_FIELD_NUMBER = 1;
private java.lang.Object message_;
/**
* required string message = 1;
*/
public boolean hasMessage() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string message = 1;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
message_ = s;
}
return s;
}
}
/**
* required string message = 1;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
message_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasMessage()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getMessageBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getMessageBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Error parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Error parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Error prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ncstream.Error}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements ucar.nc2.stream.NcStreamProto.ErrorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Error_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Error_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.stream.NcStreamProto.Error.class, ucar.nc2.stream.NcStreamProto.Error.Builder.class);
}
// Construct using ucar.nc2.stream.NcStreamProto.Error.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
message_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Error_descriptor;
}
public ucar.nc2.stream.NcStreamProto.Error getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Error.getDefaultInstance();
}
public ucar.nc2.stream.NcStreamProto.Error build() {
ucar.nc2.stream.NcStreamProto.Error result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public ucar.nc2.stream.NcStreamProto.Error buildPartial() {
ucar.nc2.stream.NcStreamProto.Error result = new ucar.nc2.stream.NcStreamProto.Error(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.message_ = message_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Error) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Error)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Error other) {
if (other == ucar.nc2.stream.NcStreamProto.Error.getDefaultInstance()) return this;
if (other.hasMessage()) {
bitField0_ |= 0x00000001;
message_ = other.message_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasMessage()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ucar.nc2.stream.NcStreamProto.Error parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ucar.nc2.stream.NcStreamProto.Error) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string message = 1;
private java.lang.Object message_ = "";
/**
* required string message = 1;
*/
public boolean hasMessage() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string message = 1;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string message = 1;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string message = 1;
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
message_ = value;
onChanged();
return this;
}
/**
* required string message = 1;
*/
public Builder clearMessage() {
bitField0_ = (bitField0_ & ~0x00000001);
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
* required string message = 1;
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
message_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Error)
}
static {
defaultInstance = new Error(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Error)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Attribute_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Attribute_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Dimension_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Dimension_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Variable_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Variable_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Structure_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Structure_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_EnumTypedef_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_EnumTypedef_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_EnumTypedef_EnumType_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_EnumTypedef_EnumType_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Group_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Group_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Header_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Header_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Data_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Data_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Range_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Range_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Section_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Section_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_StructureData_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_StructureData_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Error_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Error_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\036ucar/nc2/stream/ncStream.proto\022\010ncstre" +
"am\"\327\001\n\tAttribute\022\014\n\004name\030\001 \002(\t\022&\n\004type\030\002" +
" \002(\0162\030.ncstream.Attribute.Type\022\013\n\003len\030\003 " +
"\002(\r\022\014\n\004data\030\004 \001(\014\022\r\n\005sdata\030\005 \003(\t\022\027\n\010unsi" +
"gned\030\006 \001(\010:\005false\"Q\n\004Type\022\n\n\006STRING\020\000\022\010\n" +
"\004BYTE\020\001\022\t\n\005SHORT\020\002\022\007\n\003INT\020\003\022\010\n\004LONG\020\004\022\t\n" +
"\005FLOAT\020\005\022\n\n\006DOUBLE\020\006\"v\n\tDimension\022\014\n\004nam" +
"e\030\001 \001(\t\022\016\n\006length\030\002 \001(\004\022\032\n\013isUnlimited\030\003" +
" \001(\010:\005false\022\025\n\006isVlen\030\004 \001(\010:\005false\022\030\n\tis" +
"Private\030\005 \001(\010:\005false\"\276\001\n\010Variable\022\014\n\004nam",
"e\030\001 \002(\t\022$\n\010dataType\030\002 \002(\0162\022.ncstream.Dat" +
"aType\022\"\n\005shape\030\003 \003(\0132\023.ncstream.Dimensio" +
"n\022!\n\004atts\030\004 \003(\0132\023.ncstream.Attribute\022\027\n\010" +
"unsigned\030\005 \001(\010:\005false\022\014\n\004data\030\006 \001(\014\022\020\n\010e" +
"numType\030\007 \001(\t\"\316\001\n\tStructure\022\014\n\004name\030\001 \002(" +
"\t\022$\n\010dataType\030\002 \002(\0162\022.ncstream.DataType\022" +
"\"\n\005shape\030\003 \003(\0132\023.ncstream.Dimension\022!\n\004a" +
"tts\030\004 \003(\0132\023.ncstream.Attribute\022 \n\004vars\030\005" +
" \003(\0132\022.ncstream.Variable\022$\n\007structs\030\006 \003(" +
"\0132\023.ncstream.Structure\"q\n\013EnumTypedef\022\014\n",
"\004name\030\001 \002(\t\022+\n\003map\030\002 \003(\0132\036.ncstream.Enum" +
"Typedef.EnumType\032\'\n\010EnumType\022\014\n\004code\030\001 \002" +
"(\r\022\r\n\005value\030\002 \002(\t\"\356\001\n\005Group\022\014\n\004name\030\001 \002(" +
"\t\022!\n\004dims\030\002 \003(\0132\023.ncstream.Dimension\022 \n\004" +
"vars\030\003 \003(\0132\022.ncstream.Variable\022$\n\007struct" +
"s\030\004 \003(\0132\023.ncstream.Structure\022!\n\004atts\030\005 \003" +
"(\0132\023.ncstream.Attribute\022\037\n\006groups\030\006 \003(\0132" +
"\017.ncstream.Group\022(\n\tenumTypes\030\007 \003(\0132\025.nc" +
"stream.EnumTypedef\"h\n\006Header\022\020\n\010location" +
"\030\001 \001(\t\022\r\n\005title\030\002 \001(\t\022\n\n\002id\030\003 \001(\t\022\035\n\004roo",
"t\030\004 \002(\0132\017.ncstream.Group\022\022\n\007version\030\005 \001(" +
"\r:\0010\"\347\001\n\004Data\022\017\n\007varName\030\001 \002(\t\022$\n\010dataTy" +
"pe\030\002 \002(\0162\022.ncstream.DataType\022\"\n\007section\030" +
"\003 \001(\0132\021.ncstream.Section\022\024\n\006bigend\030\004 \001(\010" +
":\004true\022\022\n\007version\030\005 \001(\r:\0010\022*\n\010compress\030\006" +
" \001(\0162\022.ncstream.Compress:\004NONE\022\024\n\005vdata\030" +
"\007 \001(\010:\005false\022\030\n\020uncompressedSize\030\010 \001(\r\":" +
"\n\005Range\022\020\n\005start\030\001 \001(\004:\0010\022\014\n\004size\030\002 \002(\004\022" +
"\021\n\006stride\030\003 \001(\004:\0011\")\n\007Section\022\036\n\005range\030\001" +
" \003(\0132\017.ncstream.Range\"a\n\rStructureData\022\016",
"\n\006member\030\001 \003(\r\022\014\n\004data\030\002 \002(\014\022\021\n\theapCoun" +
"t\030\003 \003(\r\022\r\n\005sdata\030\004 \003(\t\022\020\n\005nrows\030\005 \001(\004:\0011" +
"\"\030\n\005Error\022\017\n\007message\030\001 \002(\t*\251\001\n\010DataType\022" +
"\010\n\004CHAR\020\000\022\010\n\004BYTE\020\001\022\t\n\005SHORT\020\002\022\007\n\003INT\020\003\022" +
"\010\n\004LONG\020\004\022\t\n\005FLOAT\020\005\022\n\n\006DOUBLE\020\006\022\n\n\006STRI" +
"NG\020\007\022\r\n\tSTRUCTURE\020\010\022\014\n\010SEQUENCE\020\t\022\t\n\005ENU" +
"M1\020\n\022\t\n\005ENUM2\020\013\022\t\n\005ENUM4\020\014\022\n\n\006OPAQUE\020\r*!" +
"\n\010Compress\022\010\n\004NONE\020\000\022\013\n\007DEFLATE\020\001B \n\017uca" +
"r.nc2.streamB\rNcStreamProto"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_ncstream_Attribute_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_ncstream_Attribute_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Attribute_descriptor,
new java.lang.String[] { "Name", "Type", "Len", "Data", "Sdata", "Unsigned", });
internal_static_ncstream_Dimension_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_ncstream_Dimension_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Dimension_descriptor,
new java.lang.String[] { "Name", "Length", "IsUnlimited", "IsVlen", "IsPrivate", });
internal_static_ncstream_Variable_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_ncstream_Variable_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Variable_descriptor,
new java.lang.String[] { "Name", "DataType", "Shape", "Atts", "Unsigned", "Data", "EnumType", });
internal_static_ncstream_Structure_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_ncstream_Structure_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Structure_descriptor,
new java.lang.String[] { "Name", "DataType", "Shape", "Atts", "Vars", "Structs", });
internal_static_ncstream_EnumTypedef_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_ncstream_EnumTypedef_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_EnumTypedef_descriptor,
new java.lang.String[] { "Name", "Map", });
internal_static_ncstream_EnumTypedef_EnumType_descriptor =
internal_static_ncstream_EnumTypedef_descriptor.getNestedTypes().get(0);
internal_static_ncstream_EnumTypedef_EnumType_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_EnumTypedef_EnumType_descriptor,
new java.lang.String[] { "Code", "Value", });
internal_static_ncstream_Group_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_ncstream_Group_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Group_descriptor,
new java.lang.String[] { "Name", "Dims", "Vars", "Structs", "Atts", "Groups", "EnumTypes", });
internal_static_ncstream_Header_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_ncstream_Header_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Header_descriptor,
new java.lang.String[] { "Location", "Title", "Id", "Root", "Version", });
internal_static_ncstream_Data_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_ncstream_Data_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Data_descriptor,
new java.lang.String[] { "VarName", "DataType", "Section", "Bigend", "Version", "Compress", "Vdata", "UncompressedSize", });
internal_static_ncstream_Range_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_ncstream_Range_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Range_descriptor,
new java.lang.String[] { "Start", "Size", "Stride", });
internal_static_ncstream_Section_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_ncstream_Section_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Section_descriptor,
new java.lang.String[] { "Range", });
internal_static_ncstream_StructureData_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_ncstream_StructureData_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_StructureData_descriptor,
new java.lang.String[] { "Member", "Data", "HeapCount", "Sdata", "Nrows", });
internal_static_ncstream_Error_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_ncstream_Error_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Error_descriptor,
new java.lang.String[] { "Message", });
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy