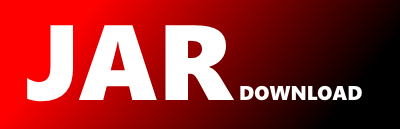
thredds.filesystem.FileSystemProto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netcdf Show documentation
Show all versions of netcdf Show documentation
The NetCDF-Java Library is a Java interface to NetCDF files,
as well as to many other types of scientific data formats.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: thredds/filesystem/FileSystem.proto
package thredds.filesystem;
public final class FileSystemProto {
private FileSystemProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public static final class Directory extends
com.google.protobuf.GeneratedMessage {
// Use Directory.newBuilder() to construct.
private Directory() {
initFields();
}
private Directory(boolean noInit) {}
private static final Directory defaultInstance;
public static Directory getDefaultInstance() {
return defaultInstance;
}
public Directory getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return thredds.filesystem.FileSystemProto.internal_static_filesystem_Directory_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return thredds.filesystem.FileSystemProto.internal_static_filesystem_Directory_fieldAccessorTable;
}
// required string path = 1;
public static final int PATH_FIELD_NUMBER = 1;
private boolean hasPath;
private java.lang.String path_ = "";
public boolean hasPath() { return hasPath; }
public java.lang.String getPath() { return path_; }
// optional uint64 lastModified = 2;
public static final int LASTMODIFIED_FIELD_NUMBER = 2;
private boolean hasLastModified;
private long lastModified_ = 0L;
public boolean hasLastModified() { return hasLastModified; }
public long getLastModified() { return lastModified_; }
// repeated .filesystem.File files = 3;
public static final int FILES_FIELD_NUMBER = 3;
private java.util.List files_ =
java.util.Collections.emptyList();
public java.util.List getFilesList() {
return files_;
}
public int getFilesCount() { return files_.size(); }
public thredds.filesystem.FileSystemProto.File getFiles(int index) {
return files_.get(index);
}
// repeated .filesystem.Attribute atts = 5;
public static final int ATTS_FIELD_NUMBER = 5;
private java.util.List atts_ =
java.util.Collections.emptyList();
public java.util.List getAttsList() {
return atts_;
}
public int getAttsCount() { return atts_.size(); }
public thredds.filesystem.FileSystemProto.Attribute getAtts(int index) {
return atts_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
if (!hasPath) return false;
for (thredds.filesystem.FileSystemProto.File element : getFilesList()) {
if (!element.isInitialized()) return false;
}
for (thredds.filesystem.FileSystemProto.Attribute element : getAttsList()) {
if (!element.isInitialized()) return false;
}
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasPath()) {
output.writeString(1, getPath());
}
if (hasLastModified()) {
output.writeUInt64(2, getLastModified());
}
for (thredds.filesystem.FileSystemProto.File element : getFilesList()) {
output.writeMessage(3, element);
}
for (thredds.filesystem.FileSystemProto.Attribute element : getAttsList()) {
output.writeMessage(5, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasPath()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getPath());
}
if (hasLastModified()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, getLastModified());
}
for (thredds.filesystem.FileSystemProto.File element : getFilesList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, element);
}
for (thredds.filesystem.FileSystemProto.Attribute element : getAttsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static thredds.filesystem.FileSystemProto.Directory parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static thredds.filesystem.FileSystemProto.Directory parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static thredds.filesystem.FileSystemProto.Directory parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static thredds.filesystem.FileSystemProto.Directory parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static thredds.filesystem.FileSystemProto.Directory parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static thredds.filesystem.FileSystemProto.Directory parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static thredds.filesystem.FileSystemProto.Directory parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static thredds.filesystem.FileSystemProto.Directory parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static thredds.filesystem.FileSystemProto.Directory parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static thredds.filesystem.FileSystemProto.Directory parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(thredds.filesystem.FileSystemProto.Directory prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private thredds.filesystem.FileSystemProto.Directory result;
// Construct using thredds.filesystem.FileSystemProto.Directory.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new thredds.filesystem.FileSystemProto.Directory();
return builder;
}
protected thredds.filesystem.FileSystemProto.Directory internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new thredds.filesystem.FileSystemProto.Directory();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return thredds.filesystem.FileSystemProto.Directory.getDescriptor();
}
public thredds.filesystem.FileSystemProto.Directory getDefaultInstanceForType() {
return thredds.filesystem.FileSystemProto.Directory.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public thredds.filesystem.FileSystemProto.Directory build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private thredds.filesystem.FileSystemProto.Directory buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public thredds.filesystem.FileSystemProto.Directory buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.files_ != java.util.Collections.EMPTY_LIST) {
result.files_ =
java.util.Collections.unmodifiableList(result.files_);
}
if (result.atts_ != java.util.Collections.EMPTY_LIST) {
result.atts_ =
java.util.Collections.unmodifiableList(result.atts_);
}
thredds.filesystem.FileSystemProto.Directory returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof thredds.filesystem.FileSystemProto.Directory) {
return mergeFrom((thredds.filesystem.FileSystemProto.Directory)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(thredds.filesystem.FileSystemProto.Directory other) {
if (other == thredds.filesystem.FileSystemProto.Directory.getDefaultInstance()) return this;
if (other.hasPath()) {
setPath(other.getPath());
}
if (other.hasLastModified()) {
setLastModified(other.getLastModified());
}
if (!other.files_.isEmpty()) {
if (result.files_.isEmpty()) {
result.files_ = new java.util.ArrayList();
}
result.files_.addAll(other.files_);
}
if (!other.atts_.isEmpty()) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.addAll(other.atts_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setPath(input.readString());
break;
}
case 16: {
setLastModified(input.readUInt64());
break;
}
case 26: {
thredds.filesystem.FileSystemProto.File.Builder subBuilder = thredds.filesystem.FileSystemProto.File.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addFiles(subBuilder.buildPartial());
break;
}
case 42: {
thredds.filesystem.FileSystemProto.Attribute.Builder subBuilder = thredds.filesystem.FileSystemProto.Attribute.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addAtts(subBuilder.buildPartial());
break;
}
}
}
}
// required string path = 1;
public boolean hasPath() {
return result.hasPath();
}
public java.lang.String getPath() {
return result.getPath();
}
public Builder setPath(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasPath = true;
result.path_ = value;
return this;
}
public Builder clearPath() {
result.hasPath = false;
result.path_ = getDefaultInstance().getPath();
return this;
}
// optional uint64 lastModified = 2;
public boolean hasLastModified() {
return result.hasLastModified();
}
public long getLastModified() {
return result.getLastModified();
}
public Builder setLastModified(long value) {
result.hasLastModified = true;
result.lastModified_ = value;
return this;
}
public Builder clearLastModified() {
result.hasLastModified = false;
result.lastModified_ = 0L;
return this;
}
// repeated .filesystem.File files = 3;
public java.util.List getFilesList() {
return java.util.Collections.unmodifiableList(result.files_);
}
public int getFilesCount() {
return result.getFilesCount();
}
public thredds.filesystem.FileSystemProto.File getFiles(int index) {
return result.getFiles(index);
}
public Builder setFiles(int index, thredds.filesystem.FileSystemProto.File value) {
if (value == null) {
throw new NullPointerException();
}
result.files_.set(index, value);
return this;
}
public Builder setFiles(int index, thredds.filesystem.FileSystemProto.File.Builder builderForValue) {
result.files_.set(index, builderForValue.build());
return this;
}
public Builder addFiles(thredds.filesystem.FileSystemProto.File value) {
if (value == null) {
throw new NullPointerException();
}
if (result.files_.isEmpty()) {
result.files_ = new java.util.ArrayList();
}
result.files_.add(value);
return this;
}
public Builder addFiles(thredds.filesystem.FileSystemProto.File.Builder builderForValue) {
if (result.files_.isEmpty()) {
result.files_ = new java.util.ArrayList();
}
result.files_.add(builderForValue.build());
return this;
}
public Builder addAllFiles(
java.lang.Iterable extends thredds.filesystem.FileSystemProto.File> values) {
if (result.files_.isEmpty()) {
result.files_ = new java.util.ArrayList();
}
super.addAll(values, result.files_);
return this;
}
public Builder clearFiles() {
result.files_ = java.util.Collections.emptyList();
return this;
}
// repeated .filesystem.Attribute atts = 5;
public java.util.List getAttsList() {
return java.util.Collections.unmodifiableList(result.atts_);
}
public int getAttsCount() {
return result.getAttsCount();
}
public thredds.filesystem.FileSystemProto.Attribute getAtts(int index) {
return result.getAtts(index);
}
public Builder setAtts(int index, thredds.filesystem.FileSystemProto.Attribute value) {
if (value == null) {
throw new NullPointerException();
}
result.atts_.set(index, value);
return this;
}
public Builder setAtts(int index, thredds.filesystem.FileSystemProto.Attribute.Builder builderForValue) {
result.atts_.set(index, builderForValue.build());
return this;
}
public Builder addAtts(thredds.filesystem.FileSystemProto.Attribute value) {
if (value == null) {
throw new NullPointerException();
}
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.add(value);
return this;
}
public Builder addAtts(thredds.filesystem.FileSystemProto.Attribute.Builder builderForValue) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.add(builderForValue.build());
return this;
}
public Builder addAllAtts(
java.lang.Iterable extends thredds.filesystem.FileSystemProto.Attribute> values) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
super.addAll(values, result.atts_);
return this;
}
public Builder clearAtts() {
result.atts_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:filesystem.Directory)
}
static {
defaultInstance = new Directory(true);
thredds.filesystem.FileSystemProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:filesystem.Directory)
}
public static final class File extends
com.google.protobuf.GeneratedMessage {
// Use File.newBuilder() to construct.
private File() {
initFields();
}
private File(boolean noInit) {}
private static final File defaultInstance;
public static File getDefaultInstance() {
return defaultInstance;
}
public File getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return thredds.filesystem.FileSystemProto.internal_static_filesystem_File_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return thredds.filesystem.FileSystemProto.internal_static_filesystem_File_fieldAccessorTable;
}
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// optional uint64 lastModified = 2;
public static final int LASTMODIFIED_FIELD_NUMBER = 2;
private boolean hasLastModified;
private long lastModified_ = 0L;
public boolean hasLastModified() { return hasLastModified; }
public long getLastModified() { return lastModified_; }
// optional uint64 length = 3;
public static final int LENGTH_FIELD_NUMBER = 3;
private boolean hasLength;
private long length_ = 0L;
public boolean hasLength() { return hasLength; }
public long getLength() { return length_; }
// required bool isDirectory = 4;
public static final int ISDIRECTORY_FIELD_NUMBER = 4;
private boolean hasIsDirectory;
private boolean isDirectory_ = false;
public boolean hasIsDirectory() { return hasIsDirectory; }
public boolean getIsDirectory() { return isDirectory_; }
// repeated .filesystem.Attribute atts = 5;
public static final int ATTS_FIELD_NUMBER = 5;
private java.util.List atts_ =
java.util.Collections.emptyList();
public java.util.List getAttsList() {
return atts_;
}
public int getAttsCount() { return atts_.size(); }
public thredds.filesystem.FileSystemProto.Attribute getAtts(int index) {
return atts_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
if (!hasName) return false;
if (!hasIsDirectory) return false;
for (thredds.filesystem.FileSystemProto.Attribute element : getAttsList()) {
if (!element.isInitialized()) return false;
}
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasName()) {
output.writeString(1, getName());
}
if (hasLastModified()) {
output.writeUInt64(2, getLastModified());
}
if (hasLength()) {
output.writeUInt64(3, getLength());
}
if (hasIsDirectory()) {
output.writeBool(4, getIsDirectory());
}
for (thredds.filesystem.FileSystemProto.Attribute element : getAttsList()) {
output.writeMessage(5, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
if (hasLastModified()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, getLastModified());
}
if (hasLength()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, getLength());
}
if (hasIsDirectory()) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, getIsDirectory());
}
for (thredds.filesystem.FileSystemProto.Attribute element : getAttsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static thredds.filesystem.FileSystemProto.File parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static thredds.filesystem.FileSystemProto.File parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static thredds.filesystem.FileSystemProto.File parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static thredds.filesystem.FileSystemProto.File parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static thredds.filesystem.FileSystemProto.File parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static thredds.filesystem.FileSystemProto.File parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static thredds.filesystem.FileSystemProto.File parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static thredds.filesystem.FileSystemProto.File parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static thredds.filesystem.FileSystemProto.File parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static thredds.filesystem.FileSystemProto.File parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(thredds.filesystem.FileSystemProto.File prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private thredds.filesystem.FileSystemProto.File result;
// Construct using thredds.filesystem.FileSystemProto.File.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new thredds.filesystem.FileSystemProto.File();
return builder;
}
protected thredds.filesystem.FileSystemProto.File internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new thredds.filesystem.FileSystemProto.File();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return thredds.filesystem.FileSystemProto.File.getDescriptor();
}
public thredds.filesystem.FileSystemProto.File getDefaultInstanceForType() {
return thredds.filesystem.FileSystemProto.File.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public thredds.filesystem.FileSystemProto.File build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private thredds.filesystem.FileSystemProto.File buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public thredds.filesystem.FileSystemProto.File buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.atts_ != java.util.Collections.EMPTY_LIST) {
result.atts_ =
java.util.Collections.unmodifiableList(result.atts_);
}
thredds.filesystem.FileSystemProto.File returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof thredds.filesystem.FileSystemProto.File) {
return mergeFrom((thredds.filesystem.FileSystemProto.File)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(thredds.filesystem.FileSystemProto.File other) {
if (other == thredds.filesystem.FileSystemProto.File.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasLastModified()) {
setLastModified(other.getLastModified());
}
if (other.hasLength()) {
setLength(other.getLength());
}
if (other.hasIsDirectory()) {
setIsDirectory(other.getIsDirectory());
}
if (!other.atts_.isEmpty()) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.addAll(other.atts_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 16: {
setLastModified(input.readUInt64());
break;
}
case 24: {
setLength(input.readUInt64());
break;
}
case 32: {
setIsDirectory(input.readBool());
break;
}
case 42: {
thredds.filesystem.FileSystemProto.Attribute.Builder subBuilder = thredds.filesystem.FileSystemProto.Attribute.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addAtts(subBuilder.buildPartial());
break;
}
}
}
}
// required string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// optional uint64 lastModified = 2;
public boolean hasLastModified() {
return result.hasLastModified();
}
public long getLastModified() {
return result.getLastModified();
}
public Builder setLastModified(long value) {
result.hasLastModified = true;
result.lastModified_ = value;
return this;
}
public Builder clearLastModified() {
result.hasLastModified = false;
result.lastModified_ = 0L;
return this;
}
// optional uint64 length = 3;
public boolean hasLength() {
return result.hasLength();
}
public long getLength() {
return result.getLength();
}
public Builder setLength(long value) {
result.hasLength = true;
result.length_ = value;
return this;
}
public Builder clearLength() {
result.hasLength = false;
result.length_ = 0L;
return this;
}
// required bool isDirectory = 4;
public boolean hasIsDirectory() {
return result.hasIsDirectory();
}
public boolean getIsDirectory() {
return result.getIsDirectory();
}
public Builder setIsDirectory(boolean value) {
result.hasIsDirectory = true;
result.isDirectory_ = value;
return this;
}
public Builder clearIsDirectory() {
result.hasIsDirectory = false;
result.isDirectory_ = false;
return this;
}
// repeated .filesystem.Attribute atts = 5;
public java.util.List getAttsList() {
return java.util.Collections.unmodifiableList(result.atts_);
}
public int getAttsCount() {
return result.getAttsCount();
}
public thredds.filesystem.FileSystemProto.Attribute getAtts(int index) {
return result.getAtts(index);
}
public Builder setAtts(int index, thredds.filesystem.FileSystemProto.Attribute value) {
if (value == null) {
throw new NullPointerException();
}
result.atts_.set(index, value);
return this;
}
public Builder setAtts(int index, thredds.filesystem.FileSystemProto.Attribute.Builder builderForValue) {
result.atts_.set(index, builderForValue.build());
return this;
}
public Builder addAtts(thredds.filesystem.FileSystemProto.Attribute value) {
if (value == null) {
throw new NullPointerException();
}
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.add(value);
return this;
}
public Builder addAtts(thredds.filesystem.FileSystemProto.Attribute.Builder builderForValue) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.add(builderForValue.build());
return this;
}
public Builder addAllAtts(
java.lang.Iterable extends thredds.filesystem.FileSystemProto.Attribute> values) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
super.addAll(values, result.atts_);
return this;
}
public Builder clearAtts() {
result.atts_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:filesystem.File)
}
static {
defaultInstance = new File(true);
thredds.filesystem.FileSystemProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:filesystem.File)
}
public static final class Attribute extends
com.google.protobuf.GeneratedMessage {
// Use Attribute.newBuilder() to construct.
private Attribute() {
initFields();
}
private Attribute(boolean noInit) {}
private static final Attribute defaultInstance;
public static Attribute getDefaultInstance() {
return defaultInstance;
}
public Attribute getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return thredds.filesystem.FileSystemProto.internal_static_filesystem_Attribute_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return thredds.filesystem.FileSystemProto.internal_static_filesystem_Attribute_fieldAccessorTable;
}
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
STRING(0, 0),
BYTE(1, 1),
SHORT(2, 2),
INT(3, 3),
LONG(4, 4),
FLOAT(5, 5),
DOUBLE(6, 6),
;
public final int getNumber() { return value; }
public static Type valueOf(int value) {
switch (value) {
case 0: return STRING;
case 1: return BYTE;
case 2: return SHORT;
case 3: return INT;
case 4: return LONG;
case 5: return FLOAT;
case 6: return DOUBLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.valueOf(number)
; }
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return thredds.filesystem.FileSystemProto.Attribute.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = {
STRING, BYTE, SHORT, INT, LONG, FLOAT, DOUBLE,
};
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private Type(int index, int value) {
this.index = index;
this.value = value;
}
static {
thredds.filesystem.FileSystemProto.getDescriptor();
}
// @@protoc_insertion_point(enum_scope:filesystem.Attribute.Type)
}
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// required .filesystem.Attribute.Type type = 2;
public static final int TYPE_FIELD_NUMBER = 2;
private boolean hasType;
private thredds.filesystem.FileSystemProto.Attribute.Type type_;
public boolean hasType() { return hasType; }
public thredds.filesystem.FileSystemProto.Attribute.Type getType() { return type_; }
// required uint32 len = 3;
public static final int LEN_FIELD_NUMBER = 3;
private boolean hasLen;
private int len_ = 0;
public boolean hasLen() { return hasLen; }
public int getLen() { return len_; }
// required bytes data = 4;
public static final int DATA_FIELD_NUMBER = 4;
private boolean hasData;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
public boolean hasData() { return hasData; }
public com.google.protobuf.ByteString getData() { return data_; }
private void initFields() {
type_ = thredds.filesystem.FileSystemProto.Attribute.Type.STRING;
}
public final boolean isInitialized() {
if (!hasName) return false;
if (!hasType) return false;
if (!hasLen) return false;
if (!hasData) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasName()) {
output.writeString(1, getName());
}
if (hasType()) {
output.writeEnum(2, getType().getNumber());
}
if (hasLen()) {
output.writeUInt32(3, getLen());
}
if (hasData()) {
output.writeBytes(4, getData());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
if (hasType()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, getType().getNumber());
}
if (hasLen()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, getLen());
}
if (hasData()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getData());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static thredds.filesystem.FileSystemProto.Attribute parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static thredds.filesystem.FileSystemProto.Attribute parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static thredds.filesystem.FileSystemProto.Attribute parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static thredds.filesystem.FileSystemProto.Attribute parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static thredds.filesystem.FileSystemProto.Attribute parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static thredds.filesystem.FileSystemProto.Attribute parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static thredds.filesystem.FileSystemProto.Attribute parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static thredds.filesystem.FileSystemProto.Attribute parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static thredds.filesystem.FileSystemProto.Attribute parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static thredds.filesystem.FileSystemProto.Attribute parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(thredds.filesystem.FileSystemProto.Attribute prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private thredds.filesystem.FileSystemProto.Attribute result;
// Construct using thredds.filesystem.FileSystemProto.Attribute.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new thredds.filesystem.FileSystemProto.Attribute();
return builder;
}
protected thredds.filesystem.FileSystemProto.Attribute internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new thredds.filesystem.FileSystemProto.Attribute();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return thredds.filesystem.FileSystemProto.Attribute.getDescriptor();
}
public thredds.filesystem.FileSystemProto.Attribute getDefaultInstanceForType() {
return thredds.filesystem.FileSystemProto.Attribute.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public thredds.filesystem.FileSystemProto.Attribute build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private thredds.filesystem.FileSystemProto.Attribute buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public thredds.filesystem.FileSystemProto.Attribute buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
thredds.filesystem.FileSystemProto.Attribute returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof thredds.filesystem.FileSystemProto.Attribute) {
return mergeFrom((thredds.filesystem.FileSystemProto.Attribute)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(thredds.filesystem.FileSystemProto.Attribute other) {
if (other == thredds.filesystem.FileSystemProto.Attribute.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasLen()) {
setLen(other.getLen());
}
if (other.hasData()) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 16: {
int rawValue = input.readEnum();
thredds.filesystem.FileSystemProto.Attribute.Type value = thredds.filesystem.FileSystemProto.Attribute.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
setType(value);
}
break;
}
case 24: {
setLen(input.readUInt32());
break;
}
case 34: {
setData(input.readBytes());
break;
}
}
}
}
// required string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// required .filesystem.Attribute.Type type = 2;
public boolean hasType() {
return result.hasType();
}
public thredds.filesystem.FileSystemProto.Attribute.Type getType() {
return result.getType();
}
public Builder setType(thredds.filesystem.FileSystemProto.Attribute.Type value) {
if (value == null) {
throw new NullPointerException();
}
result.hasType = true;
result.type_ = value;
return this;
}
public Builder clearType() {
result.hasType = false;
result.type_ = thredds.filesystem.FileSystemProto.Attribute.Type.STRING;
return this;
}
// required uint32 len = 3;
public boolean hasLen() {
return result.hasLen();
}
public int getLen() {
return result.getLen();
}
public Builder setLen(int value) {
result.hasLen = true;
result.len_ = value;
return this;
}
public Builder clearLen() {
result.hasLen = false;
result.len_ = 0;
return this;
}
// required bytes data = 4;
public boolean hasData() {
return result.hasData();
}
public com.google.protobuf.ByteString getData() {
return result.getData();
}
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
result.hasData = true;
result.data_ = value;
return this;
}
public Builder clearData() {
result.hasData = false;
result.data_ = getDefaultInstance().getData();
return this;
}
// @@protoc_insertion_point(builder_scope:filesystem.Attribute)
}
static {
defaultInstance = new Attribute(true);
thredds.filesystem.FileSystemProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:filesystem.Attribute)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_filesystem_Directory_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_filesystem_Directory_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_filesystem_File_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_filesystem_File_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_filesystem_Attribute_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_filesystem_Attribute_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n#thredds/filesystem/FileSystem.proto\022\nf" +
"ilesystem\"u\n\tDirectory\022\014\n\004path\030\001 \002(\t\022\024\n\014" +
"lastModified\030\002 \001(\004\022\037\n\005files\030\003 \003(\0132\020.file" +
"system.File\022#\n\004atts\030\005 \003(\0132\025.filesystem.A" +
"ttribute\"t\n\004File\022\014\n\004name\030\001 \002(\t\022\024\n\014lastMo" +
"dified\030\002 \001(\004\022\016\n\006length\030\003 \001(\004\022\023\n\013isDirect" +
"ory\030\004 \002(\010\022#\n\004atts\030\005 \003(\0132\025.filesystem.Att" +
"ribute\"\261\001\n\tAttribute\022\014\n\004name\030\001 \002(\t\022(\n\004ty" +
"pe\030\002 \002(\0162\032.filesystem.Attribute.Type\022\013\n\003" +
"len\030\003 \002(\r\022\014\n\004data\030\004 \002(\014\"Q\n\004Type\022\n\n\006STRIN",
"G\020\000\022\010\n\004BYTE\020\001\022\t\n\005SHORT\020\002\022\007\n\003INT\020\003\022\010\n\004LON" +
"G\020\004\022\t\n\005FLOAT\020\005\022\n\n\006DOUBLE\020\006B%\n\022thredds.fi" +
"lesystemB\017FileSystemProto"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_filesystem_Directory_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_filesystem_Directory_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_filesystem_Directory_descriptor,
new java.lang.String[] { "Path", "LastModified", "Files", "Atts", },
thredds.filesystem.FileSystemProto.Directory.class,
thredds.filesystem.FileSystemProto.Directory.Builder.class);
internal_static_filesystem_File_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_filesystem_File_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_filesystem_File_descriptor,
new java.lang.String[] { "Name", "LastModified", "Length", "IsDirectory", "Atts", },
thredds.filesystem.FileSystemProto.File.class,
thredds.filesystem.FileSystemProto.File.Builder.class);
internal_static_filesystem_Attribute_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_filesystem_Attribute_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_filesystem_Attribute_descriptor,
new java.lang.String[] { "Name", "Type", "Len", "Data", },
thredds.filesystem.FileSystemProto.Attribute.class,
thredds.filesystem.FileSystemProto.Attribute.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
public static void internalForceInit() {}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy