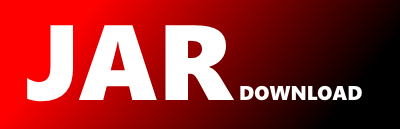
ucar.nc2.ft.point.remote.PointStreamProto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netcdf Show documentation
Show all versions of netcdf Show documentation
The NetCDF-Java Library is a Java interface to NetCDF files,
as well as to many other types of scientific data formats.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ucar/nc2/ft/point/remote/pointStream.proto
package ucar.nc2.ft.point.remote;
public final class PointStreamProto {
private PointStreamProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public static final class Location extends
com.google.protobuf.GeneratedMessage {
// Use Location.newBuilder() to construct.
private Location() {
initFields();
}
private Location(boolean noInit) {}
private static final Location defaultInstance;
public static Location getDefaultInstance() {
return defaultInstance;
}
public Location getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_Location_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_Location_fieldAccessorTable;
}
// required double time = 1;
public static final int TIME_FIELD_NUMBER = 1;
private boolean hasTime;
private double time_ = 0D;
public boolean hasTime() { return hasTime; }
public double getTime() { return time_; }
// required double lat = 2;
public static final int LAT_FIELD_NUMBER = 2;
private boolean hasLat;
private double lat_ = 0D;
public boolean hasLat() { return hasLat; }
public double getLat() { return lat_; }
// required double lon = 3;
public static final int LON_FIELD_NUMBER = 3;
private boolean hasLon;
private double lon_ = 0D;
public boolean hasLon() { return hasLon; }
public double getLon() { return lon_; }
// optional double alt = 4;
public static final int ALT_FIELD_NUMBER = 4;
private boolean hasAlt;
private double alt_ = 0D;
public boolean hasAlt() { return hasAlt; }
public double getAlt() { return alt_; }
// optional double nomTime = 5;
public static final int NOMTIME_FIELD_NUMBER = 5;
private boolean hasNomTime;
private double nomTime_ = 0D;
public boolean hasNomTime() { return hasNomTime; }
public double getNomTime() { return nomTime_; }
private void initFields() {
}
public final boolean isInitialized() {
if (!hasTime) return false;
if (!hasLat) return false;
if (!hasLon) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasTime()) {
output.writeDouble(1, getTime());
}
if (hasLat()) {
output.writeDouble(2, getLat());
}
if (hasLon()) {
output.writeDouble(3, getLon());
}
if (hasAlt()) {
output.writeDouble(4, getAlt());
}
if (hasNomTime()) {
output.writeDouble(5, getNomTime());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasTime()) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(1, getTime());
}
if (hasLat()) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(2, getLat());
}
if (hasLon()) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(3, getLon());
}
if (hasAlt()) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, getAlt());
}
if (hasNomTime()) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(5, getNomTime());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.Location prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.ft.point.remote.PointStreamProto.Location result;
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.Location.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.ft.point.remote.PointStreamProto.Location();
return builder;
}
protected ucar.nc2.ft.point.remote.PointStreamProto.Location internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.ft.point.remote.PointStreamProto.Location();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.Location.getDescriptor();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Location getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Location build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.ft.point.remote.PointStreamProto.Location buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Location buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
ucar.nc2.ft.point.remote.PointStreamProto.Location returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.Location) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.Location)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.Location other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance()) return this;
if (other.hasTime()) {
setTime(other.getTime());
}
if (other.hasLat()) {
setLat(other.getLat());
}
if (other.hasLon()) {
setLon(other.getLon());
}
if (other.hasAlt()) {
setAlt(other.getAlt());
}
if (other.hasNomTime()) {
setNomTime(other.getNomTime());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 9: {
setTime(input.readDouble());
break;
}
case 17: {
setLat(input.readDouble());
break;
}
case 25: {
setLon(input.readDouble());
break;
}
case 33: {
setAlt(input.readDouble());
break;
}
case 41: {
setNomTime(input.readDouble());
break;
}
}
}
}
// required double time = 1;
public boolean hasTime() {
return result.hasTime();
}
public double getTime() {
return result.getTime();
}
public Builder setTime(double value) {
result.hasTime = true;
result.time_ = value;
return this;
}
public Builder clearTime() {
result.hasTime = false;
result.time_ = 0D;
return this;
}
// required double lat = 2;
public boolean hasLat() {
return result.hasLat();
}
public double getLat() {
return result.getLat();
}
public Builder setLat(double value) {
result.hasLat = true;
result.lat_ = value;
return this;
}
public Builder clearLat() {
result.hasLat = false;
result.lat_ = 0D;
return this;
}
// required double lon = 3;
public boolean hasLon() {
return result.hasLon();
}
public double getLon() {
return result.getLon();
}
public Builder setLon(double value) {
result.hasLon = true;
result.lon_ = value;
return this;
}
public Builder clearLon() {
result.hasLon = false;
result.lon_ = 0D;
return this;
}
// optional double alt = 4;
public boolean hasAlt() {
return result.hasAlt();
}
public double getAlt() {
return result.getAlt();
}
public Builder setAlt(double value) {
result.hasAlt = true;
result.alt_ = value;
return this;
}
public Builder clearAlt() {
result.hasAlt = false;
result.alt_ = 0D;
return this;
}
// optional double nomTime = 5;
public boolean hasNomTime() {
return result.hasNomTime();
}
public double getNomTime() {
return result.getNomTime();
}
public Builder setNomTime(double value) {
result.hasNomTime = true;
result.nomTime_ = value;
return this;
}
public Builder clearNomTime() {
result.hasNomTime = false;
result.nomTime_ = 0D;
return this;
}
// @@protoc_insertion_point(builder_scope:pointStream.Location)
}
static {
defaultInstance = new Location(true);
ucar.nc2.ft.point.remote.PointStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:pointStream.Location)
}
public static final class PointFeature extends
com.google.protobuf.GeneratedMessage {
// Use PointFeature.newBuilder() to construct.
private PointFeature() {
initFields();
}
private PointFeature(boolean noInit) {}
private static final PointFeature defaultInstance;
public static PointFeature getDefaultInstance() {
return defaultInstance;
}
public PointFeature getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_PointFeature_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_PointFeature_fieldAccessorTable;
}
// required .pointStream.Location loc = 1;
public static final int LOC_FIELD_NUMBER = 1;
private boolean hasLoc;
private ucar.nc2.ft.point.remote.PointStreamProto.Location loc_;
public boolean hasLoc() { return hasLoc; }
public ucar.nc2.ft.point.remote.PointStreamProto.Location getLoc() { return loc_; }
// required bytes data = 3;
public static final int DATA_FIELD_NUMBER = 3;
private boolean hasData;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
public boolean hasData() { return hasData; }
public com.google.protobuf.ByteString getData() { return data_; }
// repeated string sdata = 4;
public static final int SDATA_FIELD_NUMBER = 4;
private java.util.List sdata_ =
java.util.Collections.emptyList();
public java.util.List getSdataList() {
return sdata_;
}
public int getSdataCount() { return sdata_.size(); }
public java.lang.String getSdata(int index) {
return sdata_.get(index);
}
private void initFields() {
loc_ = ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance();
}
public final boolean isInitialized() {
if (!hasLoc) return false;
if (!hasData) return false;
if (!getLoc().isInitialized()) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasLoc()) {
output.writeMessage(1, getLoc());
}
if (hasData()) {
output.writeBytes(3, getData());
}
for (java.lang.String element : getSdataList()) {
output.writeString(4, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasLoc()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getLoc());
}
if (hasData()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getData());
}
{
int dataSize = 0;
for (java.lang.String element : getSdataList()) {
dataSize += com.google.protobuf.CodedOutputStream
.computeStringSizeNoTag(element);
}
size += dataSize;
size += 1 * getSdataList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.PointFeature prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.ft.point.remote.PointStreamProto.PointFeature result;
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.ft.point.remote.PointStreamProto.PointFeature();
return builder;
}
protected ucar.nc2.ft.point.remote.PointStreamProto.PointFeature internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.ft.point.remote.PointStreamProto.PointFeature();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.getDescriptor();
}
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeature getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeature build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.ft.point.remote.PointStreamProto.PointFeature buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeature buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.sdata_ != java.util.Collections.EMPTY_LIST) {
result.sdata_ =
java.util.Collections.unmodifiableList(result.sdata_);
}
ucar.nc2.ft.point.remote.PointStreamProto.PointFeature returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.PointFeature) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.PointFeature)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.PointFeature other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.getDefaultInstance()) return this;
if (other.hasLoc()) {
mergeLoc(other.getLoc());
}
if (other.hasData()) {
setData(other.getData());
}
if (!other.sdata_.isEmpty()) {
if (result.sdata_.isEmpty()) {
result.sdata_ = new java.util.ArrayList();
}
result.sdata_.addAll(other.sdata_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
ucar.nc2.ft.point.remote.PointStreamProto.Location.Builder subBuilder = ucar.nc2.ft.point.remote.PointStreamProto.Location.newBuilder();
if (hasLoc()) {
subBuilder.mergeFrom(getLoc());
}
input.readMessage(subBuilder, extensionRegistry);
setLoc(subBuilder.buildPartial());
break;
}
case 26: {
setData(input.readBytes());
break;
}
case 34: {
addSdata(input.readString());
break;
}
}
}
}
// required .pointStream.Location loc = 1;
public boolean hasLoc() {
return result.hasLoc();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Location getLoc() {
return result.getLoc();
}
public Builder setLoc(ucar.nc2.ft.point.remote.PointStreamProto.Location value) {
if (value == null) {
throw new NullPointerException();
}
result.hasLoc = true;
result.loc_ = value;
return this;
}
public Builder setLoc(ucar.nc2.ft.point.remote.PointStreamProto.Location.Builder builderForValue) {
result.hasLoc = true;
result.loc_ = builderForValue.build();
return this;
}
public Builder mergeLoc(ucar.nc2.ft.point.remote.PointStreamProto.Location value) {
if (result.hasLoc() &&
result.loc_ != ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance()) {
result.loc_ =
ucar.nc2.ft.point.remote.PointStreamProto.Location.newBuilder(result.loc_).mergeFrom(value).buildPartial();
} else {
result.loc_ = value;
}
result.hasLoc = true;
return this;
}
public Builder clearLoc() {
result.hasLoc = false;
result.loc_ = ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance();
return this;
}
// required bytes data = 3;
public boolean hasData() {
return result.hasData();
}
public com.google.protobuf.ByteString getData() {
return result.getData();
}
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
result.hasData = true;
result.data_ = value;
return this;
}
public Builder clearData() {
result.hasData = false;
result.data_ = getDefaultInstance().getData();
return this;
}
// repeated string sdata = 4;
public java.util.List getSdataList() {
return java.util.Collections.unmodifiableList(result.sdata_);
}
public int getSdataCount() {
return result.getSdataCount();
}
public java.lang.String getSdata(int index) {
return result.getSdata(index);
}
public Builder setSdata(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.sdata_.set(index, value);
return this;
}
public Builder addSdata(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
if (result.sdata_.isEmpty()) {
result.sdata_ = new java.util.ArrayList();
}
result.sdata_.add(value);
return this;
}
public Builder addAllSdata(
java.lang.Iterable extends java.lang.String> values) {
if (result.sdata_.isEmpty()) {
result.sdata_ = new java.util.ArrayList();
}
super.addAll(values, result.sdata_);
return this;
}
public Builder clearSdata() {
result.sdata_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:pointStream.PointFeature)
}
static {
defaultInstance = new PointFeature(true);
ucar.nc2.ft.point.remote.PointStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:pointStream.PointFeature)
}
public static final class Member extends
com.google.protobuf.GeneratedMessage {
// Use Member.newBuilder() to construct.
private Member() {
initFields();
}
private Member(boolean noInit) {}
private static final Member defaultInstance;
public static Member getDefaultInstance() {
return defaultInstance;
}
public Member getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_Member_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_Member_fieldAccessorTable;
}
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// optional string desc = 2;
public static final int DESC_FIELD_NUMBER = 2;
private boolean hasDesc;
private java.lang.String desc_ = "";
public boolean hasDesc() { return hasDesc; }
public java.lang.String getDesc() { return desc_; }
// optional string units = 3;
public static final int UNITS_FIELD_NUMBER = 3;
private boolean hasUnits;
private java.lang.String units_ = "";
public boolean hasUnits() { return hasUnits; }
public java.lang.String getUnits() { return units_; }
// required .ncstream.DataType dataType = 4;
public static final int DATATYPE_FIELD_NUMBER = 4;
private boolean hasDataType;
private ucar.nc2.stream.NcStreamProto.DataType dataType_;
public boolean hasDataType() { return hasDataType; }
public ucar.nc2.stream.NcStreamProto.DataType getDataType() { return dataType_; }
// required .ncstream.Section section = 5;
public static final int SECTION_FIELD_NUMBER = 5;
private boolean hasSection;
private ucar.nc2.stream.NcStreamProto.Section section_;
public boolean hasSection() { return hasSection; }
public ucar.nc2.stream.NcStreamProto.Section getSection() { return section_; }
private void initFields() {
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
section_ = ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance();
}
public final boolean isInitialized() {
if (!hasName) return false;
if (!hasDataType) return false;
if (!hasSection) return false;
if (!getSection().isInitialized()) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasName()) {
output.writeString(1, getName());
}
if (hasDesc()) {
output.writeString(2, getDesc());
}
if (hasUnits()) {
output.writeString(3, getUnits());
}
if (hasDataType()) {
output.writeEnum(4, getDataType().getNumber());
}
if (hasSection()) {
output.writeMessage(5, getSection());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
if (hasDesc()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getDesc());
}
if (hasUnits()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(3, getUnits());
}
if (hasDataType()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, getDataType().getNumber());
}
if (hasSection()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getSection());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Member parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Member parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Member parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Member parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Member parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Member parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Member parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Member parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Member parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Member parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.Member prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.ft.point.remote.PointStreamProto.Member result;
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.Member.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.ft.point.remote.PointStreamProto.Member();
return builder;
}
protected ucar.nc2.ft.point.remote.PointStreamProto.Member internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.ft.point.remote.PointStreamProto.Member();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.Member.getDescriptor();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Member getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.Member.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Member build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.ft.point.remote.PointStreamProto.Member buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Member buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
ucar.nc2.ft.point.remote.PointStreamProto.Member returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.Member) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.Member)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.Member other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.Member.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasDesc()) {
setDesc(other.getDesc());
}
if (other.hasUnits()) {
setUnits(other.getUnits());
}
if (other.hasDataType()) {
setDataType(other.getDataType());
}
if (other.hasSection()) {
mergeSection(other.getSection());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 18: {
setDesc(input.readString());
break;
}
case 26: {
setUnits(input.readString());
break;
}
case 32: {
int rawValue = input.readEnum();
ucar.nc2.stream.NcStreamProto.DataType value = ucar.nc2.stream.NcStreamProto.DataType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(4, rawValue);
} else {
setDataType(value);
}
break;
}
case 42: {
ucar.nc2.stream.NcStreamProto.Section.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Section.newBuilder();
if (hasSection()) {
subBuilder.mergeFrom(getSection());
}
input.readMessage(subBuilder, extensionRegistry);
setSection(subBuilder.buildPartial());
break;
}
}
}
}
// required string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// optional string desc = 2;
public boolean hasDesc() {
return result.hasDesc();
}
public java.lang.String getDesc() {
return result.getDesc();
}
public Builder setDesc(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDesc = true;
result.desc_ = value;
return this;
}
public Builder clearDesc() {
result.hasDesc = false;
result.desc_ = getDefaultInstance().getDesc();
return this;
}
// optional string units = 3;
public boolean hasUnits() {
return result.hasUnits();
}
public java.lang.String getUnits() {
return result.getUnits();
}
public Builder setUnits(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasUnits = true;
result.units_ = value;
return this;
}
public Builder clearUnits() {
result.hasUnits = false;
result.units_ = getDefaultInstance().getUnits();
return this;
}
// required .ncstream.DataType dataType = 4;
public boolean hasDataType() {
return result.hasDataType();
}
public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
return result.getDataType();
}
public Builder setDataType(ucar.nc2.stream.NcStreamProto.DataType value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDataType = true;
result.dataType_ = value;
return this;
}
public Builder clearDataType() {
result.hasDataType = false;
result.dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
return this;
}
// required .ncstream.Section section = 5;
public boolean hasSection() {
return result.hasSection();
}
public ucar.nc2.stream.NcStreamProto.Section getSection() {
return result.getSection();
}
public Builder setSection(ucar.nc2.stream.NcStreamProto.Section value) {
if (value == null) {
throw new NullPointerException();
}
result.hasSection = true;
result.section_ = value;
return this;
}
public Builder setSection(ucar.nc2.stream.NcStreamProto.Section.Builder builderForValue) {
result.hasSection = true;
result.section_ = builderForValue.build();
return this;
}
public Builder mergeSection(ucar.nc2.stream.NcStreamProto.Section value) {
if (result.hasSection() &&
result.section_ != ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance()) {
result.section_ =
ucar.nc2.stream.NcStreamProto.Section.newBuilder(result.section_).mergeFrom(value).buildPartial();
} else {
result.section_ = value;
}
result.hasSection = true;
return this;
}
public Builder clearSection() {
result.hasSection = false;
result.section_ = ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance();
return this;
}
// @@protoc_insertion_point(builder_scope:pointStream.Member)
}
static {
defaultInstance = new Member(true);
ucar.nc2.ft.point.remote.PointStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:pointStream.Member)
}
public static final class PointFeatureCollection extends
com.google.protobuf.GeneratedMessage {
// Use PointFeatureCollection.newBuilder() to construct.
private PointFeatureCollection() {
initFields();
}
private PointFeatureCollection(boolean noInit) {}
private static final PointFeatureCollection defaultInstance;
public static PointFeatureCollection getDefaultInstance() {
return defaultInstance;
}
public PointFeatureCollection getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_PointFeatureCollection_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_PointFeatureCollection_fieldAccessorTable;
}
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// required string timeUnit = 2;
public static final int TIMEUNIT_FIELD_NUMBER = 2;
private boolean hasTimeUnit;
private java.lang.String timeUnit_ = "";
public boolean hasTimeUnit() { return hasTimeUnit; }
public java.lang.String getTimeUnit() { return timeUnit_; }
// repeated .pointStream.Member members = 3;
public static final int MEMBERS_FIELD_NUMBER = 3;
private java.util.List members_ =
java.util.Collections.emptyList();
public java.util.List getMembersList() {
return members_;
}
public int getMembersCount() { return members_.size(); }
public ucar.nc2.ft.point.remote.PointStreamProto.Member getMembers(int index) {
return members_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
if (!hasName) return false;
if (!hasTimeUnit) return false;
for (ucar.nc2.ft.point.remote.PointStreamProto.Member element : getMembersList()) {
if (!element.isInitialized()) return false;
}
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasName()) {
output.writeString(1, getName());
}
if (hasTimeUnit()) {
output.writeString(2, getTimeUnit());
}
for (ucar.nc2.ft.point.remote.PointStreamProto.Member element : getMembersList()) {
output.writeMessage(3, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
if (hasTimeUnit()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getTimeUnit());
}
for (ucar.nc2.ft.point.remote.PointStreamProto.Member element : getMembersList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection result;
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection();
return builder;
}
protected ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.getDescriptor();
}
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.members_ != java.util.Collections.EMPTY_LIST) {
result.members_ =
java.util.Collections.unmodifiableList(result.members_);
}
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasTimeUnit()) {
setTimeUnit(other.getTimeUnit());
}
if (!other.members_.isEmpty()) {
if (result.members_.isEmpty()) {
result.members_ = new java.util.ArrayList();
}
result.members_.addAll(other.members_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 18: {
setTimeUnit(input.readString());
break;
}
case 26: {
ucar.nc2.ft.point.remote.PointStreamProto.Member.Builder subBuilder = ucar.nc2.ft.point.remote.PointStreamProto.Member.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addMembers(subBuilder.buildPartial());
break;
}
}
}
}
// required string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// required string timeUnit = 2;
public boolean hasTimeUnit() {
return result.hasTimeUnit();
}
public java.lang.String getTimeUnit() {
return result.getTimeUnit();
}
public Builder setTimeUnit(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasTimeUnit = true;
result.timeUnit_ = value;
return this;
}
public Builder clearTimeUnit() {
result.hasTimeUnit = false;
result.timeUnit_ = getDefaultInstance().getTimeUnit();
return this;
}
// repeated .pointStream.Member members = 3;
public java.util.List getMembersList() {
return java.util.Collections.unmodifiableList(result.members_);
}
public int getMembersCount() {
return result.getMembersCount();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Member getMembers(int index) {
return result.getMembers(index);
}
public Builder setMembers(int index, ucar.nc2.ft.point.remote.PointStreamProto.Member value) {
if (value == null) {
throw new NullPointerException();
}
result.members_.set(index, value);
return this;
}
public Builder setMembers(int index, ucar.nc2.ft.point.remote.PointStreamProto.Member.Builder builderForValue) {
result.members_.set(index, builderForValue.build());
return this;
}
public Builder addMembers(ucar.nc2.ft.point.remote.PointStreamProto.Member value) {
if (value == null) {
throw new NullPointerException();
}
if (result.members_.isEmpty()) {
result.members_ = new java.util.ArrayList();
}
result.members_.add(value);
return this;
}
public Builder addMembers(ucar.nc2.ft.point.remote.PointStreamProto.Member.Builder builderForValue) {
if (result.members_.isEmpty()) {
result.members_ = new java.util.ArrayList();
}
result.members_.add(builderForValue.build());
return this;
}
public Builder addAllMembers(
java.lang.Iterable extends ucar.nc2.ft.point.remote.PointStreamProto.Member> values) {
if (result.members_.isEmpty()) {
result.members_ = new java.util.ArrayList();
}
super.addAll(values, result.members_);
return this;
}
public Builder clearMembers() {
result.members_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:pointStream.PointFeatureCollection)
}
static {
defaultInstance = new PointFeatureCollection(true);
ucar.nc2.ft.point.remote.PointStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:pointStream.PointFeatureCollection)
}
public static final class Station extends
com.google.protobuf.GeneratedMessage {
// Use Station.newBuilder() to construct.
private Station() {
initFields();
}
private Station(boolean noInit) {}
private static final Station defaultInstance;
public static Station getDefaultInstance() {
return defaultInstance;
}
public Station getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_Station_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_Station_fieldAccessorTable;
}
// required string id = 1;
public static final int ID_FIELD_NUMBER = 1;
private boolean hasId;
private java.lang.String id_ = "";
public boolean hasId() { return hasId; }
public java.lang.String getId() { return id_; }
// required double lat = 2;
public static final int LAT_FIELD_NUMBER = 2;
private boolean hasLat;
private double lat_ = 0D;
public boolean hasLat() { return hasLat; }
public double getLat() { return lat_; }
// required double lon = 3;
public static final int LON_FIELD_NUMBER = 3;
private boolean hasLon;
private double lon_ = 0D;
public boolean hasLon() { return hasLon; }
public double getLon() { return lon_; }
// optional double alt = 4;
public static final int ALT_FIELD_NUMBER = 4;
private boolean hasAlt;
private double alt_ = 0D;
public boolean hasAlt() { return hasAlt; }
public double getAlt() { return alt_; }
// optional string desc = 5;
public static final int DESC_FIELD_NUMBER = 5;
private boolean hasDesc;
private java.lang.String desc_ = "";
public boolean hasDesc() { return hasDesc; }
public java.lang.String getDesc() { return desc_; }
// optional string wmoId = 6;
public static final int WMOID_FIELD_NUMBER = 6;
private boolean hasWmoId;
private java.lang.String wmoId_ = "";
public boolean hasWmoId() { return hasWmoId; }
public java.lang.String getWmoId() { return wmoId_; }
private void initFields() {
}
public final boolean isInitialized() {
if (!hasId) return false;
if (!hasLat) return false;
if (!hasLon) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasId()) {
output.writeString(1, getId());
}
if (hasLat()) {
output.writeDouble(2, getLat());
}
if (hasLon()) {
output.writeDouble(3, getLon());
}
if (hasAlt()) {
output.writeDouble(4, getAlt());
}
if (hasDesc()) {
output.writeString(5, getDesc());
}
if (hasWmoId()) {
output.writeString(6, getWmoId());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasId()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getId());
}
if (hasLat()) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(2, getLat());
}
if (hasLon()) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(3, getLon());
}
if (hasAlt()) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, getAlt());
}
if (hasDesc()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(5, getDesc());
}
if (hasWmoId()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(6, getWmoId());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.Station prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.ft.point.remote.PointStreamProto.Station result;
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.Station.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.ft.point.remote.PointStreamProto.Station();
return builder;
}
protected ucar.nc2.ft.point.remote.PointStreamProto.Station internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.ft.point.remote.PointStreamProto.Station();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.Station.getDescriptor();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Station getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.Station.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Station build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.ft.point.remote.PointStreamProto.Station buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Station buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
ucar.nc2.ft.point.remote.PointStreamProto.Station returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.Station) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.Station)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.Station other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.Station.getDefaultInstance()) return this;
if (other.hasId()) {
setId(other.getId());
}
if (other.hasLat()) {
setLat(other.getLat());
}
if (other.hasLon()) {
setLon(other.getLon());
}
if (other.hasAlt()) {
setAlt(other.getAlt());
}
if (other.hasDesc()) {
setDesc(other.getDesc());
}
if (other.hasWmoId()) {
setWmoId(other.getWmoId());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setId(input.readString());
break;
}
case 17: {
setLat(input.readDouble());
break;
}
case 25: {
setLon(input.readDouble());
break;
}
case 33: {
setAlt(input.readDouble());
break;
}
case 42: {
setDesc(input.readString());
break;
}
case 50: {
setWmoId(input.readString());
break;
}
}
}
}
// required string id = 1;
public boolean hasId() {
return result.hasId();
}
public java.lang.String getId() {
return result.getId();
}
public Builder setId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasId = true;
result.id_ = value;
return this;
}
public Builder clearId() {
result.hasId = false;
result.id_ = getDefaultInstance().getId();
return this;
}
// required double lat = 2;
public boolean hasLat() {
return result.hasLat();
}
public double getLat() {
return result.getLat();
}
public Builder setLat(double value) {
result.hasLat = true;
result.lat_ = value;
return this;
}
public Builder clearLat() {
result.hasLat = false;
result.lat_ = 0D;
return this;
}
// required double lon = 3;
public boolean hasLon() {
return result.hasLon();
}
public double getLon() {
return result.getLon();
}
public Builder setLon(double value) {
result.hasLon = true;
result.lon_ = value;
return this;
}
public Builder clearLon() {
result.hasLon = false;
result.lon_ = 0D;
return this;
}
// optional double alt = 4;
public boolean hasAlt() {
return result.hasAlt();
}
public double getAlt() {
return result.getAlt();
}
public Builder setAlt(double value) {
result.hasAlt = true;
result.alt_ = value;
return this;
}
public Builder clearAlt() {
result.hasAlt = false;
result.alt_ = 0D;
return this;
}
// optional string desc = 5;
public boolean hasDesc() {
return result.hasDesc();
}
public java.lang.String getDesc() {
return result.getDesc();
}
public Builder setDesc(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDesc = true;
result.desc_ = value;
return this;
}
public Builder clearDesc() {
result.hasDesc = false;
result.desc_ = getDefaultInstance().getDesc();
return this;
}
// optional string wmoId = 6;
public boolean hasWmoId() {
return result.hasWmoId();
}
public java.lang.String getWmoId() {
return result.getWmoId();
}
public Builder setWmoId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasWmoId = true;
result.wmoId_ = value;
return this;
}
public Builder clearWmoId() {
result.hasWmoId = false;
result.wmoId_ = getDefaultInstance().getWmoId();
return this;
}
// @@protoc_insertion_point(builder_scope:pointStream.Station)
}
static {
defaultInstance = new Station(true);
ucar.nc2.ft.point.remote.PointStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:pointStream.Station)
}
public static final class StationList extends
com.google.protobuf.GeneratedMessage {
// Use StationList.newBuilder() to construct.
private StationList() {
initFields();
}
private StationList(boolean noInit) {}
private static final StationList defaultInstance;
public static StationList getDefaultInstance() {
return defaultInstance;
}
public StationList getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_StationList_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_pointStream_StationList_fieldAccessorTable;
}
// repeated .pointStream.Station stations = 1;
public static final int STATIONS_FIELD_NUMBER = 1;
private java.util.List stations_ =
java.util.Collections.emptyList();
public java.util.List getStationsList() {
return stations_;
}
public int getStationsCount() { return stations_.size(); }
public ucar.nc2.ft.point.remote.PointStreamProto.Station getStations(int index) {
return stations_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
for (ucar.nc2.ft.point.remote.PointStreamProto.Station element : getStationsList()) {
if (!element.isInitialized()) return false;
}
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (ucar.nc2.ft.point.remote.PointStreamProto.Station element : getStationsList()) {
output.writeMessage(1, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (ucar.nc2.ft.point.remote.PointStreamProto.Station element : getStationsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.StationList prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.ft.point.remote.PointStreamProto.StationList result;
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.StationList.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.ft.point.remote.PointStreamProto.StationList();
return builder;
}
protected ucar.nc2.ft.point.remote.PointStreamProto.StationList internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.ft.point.remote.PointStreamProto.StationList();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.StationList.getDescriptor();
}
public ucar.nc2.ft.point.remote.PointStreamProto.StationList getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.StationList.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.ft.point.remote.PointStreamProto.StationList build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.ft.point.remote.PointStreamProto.StationList buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.ft.point.remote.PointStreamProto.StationList buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.stations_ != java.util.Collections.EMPTY_LIST) {
result.stations_ =
java.util.Collections.unmodifiableList(result.stations_);
}
ucar.nc2.ft.point.remote.PointStreamProto.StationList returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.StationList) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.StationList)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.StationList other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.StationList.getDefaultInstance()) return this;
if (!other.stations_.isEmpty()) {
if (result.stations_.isEmpty()) {
result.stations_ = new java.util.ArrayList();
}
result.stations_.addAll(other.stations_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder subBuilder = ucar.nc2.ft.point.remote.PointStreamProto.Station.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addStations(subBuilder.buildPartial());
break;
}
}
}
}
// repeated .pointStream.Station stations = 1;
public java.util.List getStationsList() {
return java.util.Collections.unmodifiableList(result.stations_);
}
public int getStationsCount() {
return result.getStationsCount();
}
public ucar.nc2.ft.point.remote.PointStreamProto.Station getStations(int index) {
return result.getStations(index);
}
public Builder setStations(int index, ucar.nc2.ft.point.remote.PointStreamProto.Station value) {
if (value == null) {
throw new NullPointerException();
}
result.stations_.set(index, value);
return this;
}
public Builder setStations(int index, ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder builderForValue) {
result.stations_.set(index, builderForValue.build());
return this;
}
public Builder addStations(ucar.nc2.ft.point.remote.PointStreamProto.Station value) {
if (value == null) {
throw new NullPointerException();
}
if (result.stations_.isEmpty()) {
result.stations_ = new java.util.ArrayList();
}
result.stations_.add(value);
return this;
}
public Builder addStations(ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder builderForValue) {
if (result.stations_.isEmpty()) {
result.stations_ = new java.util.ArrayList();
}
result.stations_.add(builderForValue.build());
return this;
}
public Builder addAllStations(
java.lang.Iterable extends ucar.nc2.ft.point.remote.PointStreamProto.Station> values) {
if (result.stations_.isEmpty()) {
result.stations_ = new java.util.ArrayList();
}
super.addAll(values, result.stations_);
return this;
}
public Builder clearStations() {
result.stations_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:pointStream.StationList)
}
static {
defaultInstance = new StationList(true);
ucar.nc2.ft.point.remote.PointStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:pointStream.StationList)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_pointStream_Location_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_pointStream_Location_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_pointStream_PointFeature_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_pointStream_PointFeature_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_pointStream_Member_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_pointStream_Member_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_pointStream_PointFeatureCollection_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_pointStream_PointFeatureCollection_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_pointStream_Station_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_pointStream_Station_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_pointStream_StationList_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_pointStream_StationList_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n*ucar/nc2/ft/point/remote/pointStream.p" +
"roto\022\013pointStream\032\036ucar/nc2/stream/ncStr" +
"eam.proto\"P\n\010Location\022\014\n\004time\030\001 \002(\001\022\013\n\003l" +
"at\030\002 \002(\001\022\013\n\003lon\030\003 \002(\001\022\013\n\003alt\030\004 \001(\001\022\017\n\007no" +
"mTime\030\005 \001(\001\"O\n\014PointFeature\022\"\n\003loc\030\001 \002(\013" +
"2\025.pointStream.Location\022\014\n\004data\030\003 \002(\014\022\r\n" +
"\005sdata\030\004 \003(\t\"}\n\006Member\022\014\n\004name\030\001 \002(\t\022\014\n\004" +
"desc\030\002 \001(\t\022\r\n\005units\030\003 \001(\t\022$\n\010dataType\030\004 " +
"\002(\0162\022.ncstream.DataType\022\"\n\007section\030\005 \002(\013" +
"2\021.ncstream.Section\"^\n\026PointFeatureColle",
"ction\022\014\n\004name\030\001 \002(\t\022\020\n\010timeUnit\030\002 \002(\t\022$\n" +
"\007members\030\003 \003(\0132\023.pointStream.Member\"Y\n\007S" +
"tation\022\n\n\002id\030\001 \002(\t\022\013\n\003lat\030\002 \002(\001\022\013\n\003lon\030\003" +
" \002(\001\022\013\n\003alt\030\004 \001(\001\022\014\n\004desc\030\005 \001(\t\022\r\n\005wmoId" +
"\030\006 \001(\t\"5\n\013StationList\022&\n\010stations\030\001 \003(\0132" +
"\024.pointStream.StationB,\n\030ucar.nc2.ft.poi" +
"nt.remoteB\020PointStreamProto"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_pointStream_Location_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_pointStream_Location_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_pointStream_Location_descriptor,
new java.lang.String[] { "Time", "Lat", "Lon", "Alt", "NomTime", },
ucar.nc2.ft.point.remote.PointStreamProto.Location.class,
ucar.nc2.ft.point.remote.PointStreamProto.Location.Builder.class);
internal_static_pointStream_PointFeature_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_pointStream_PointFeature_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_pointStream_PointFeature_descriptor,
new java.lang.String[] { "Loc", "Data", "Sdata", },
ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.class,
ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.Builder.class);
internal_static_pointStream_Member_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_pointStream_Member_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_pointStream_Member_descriptor,
new java.lang.String[] { "Name", "Desc", "Units", "DataType", "Section", },
ucar.nc2.ft.point.remote.PointStreamProto.Member.class,
ucar.nc2.ft.point.remote.PointStreamProto.Member.Builder.class);
internal_static_pointStream_PointFeatureCollection_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_pointStream_PointFeatureCollection_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_pointStream_PointFeatureCollection_descriptor,
new java.lang.String[] { "Name", "TimeUnit", "Members", },
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.class,
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.Builder.class);
internal_static_pointStream_Station_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_pointStream_Station_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_pointStream_Station_descriptor,
new java.lang.String[] { "Id", "Lat", "Lon", "Alt", "Desc", "WmoId", },
ucar.nc2.ft.point.remote.PointStreamProto.Station.class,
ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder.class);
internal_static_pointStream_StationList_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_pointStream_StationList_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_pointStream_StationList_descriptor,
new java.lang.String[] { "Stations", },
ucar.nc2.ft.point.remote.PointStreamProto.StationList.class,
ucar.nc2.ft.point.remote.PointStreamProto.StationList.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
ucar.nc2.stream.NcStreamProto.getDescriptor(),
}, assigner);
}
public static void internalForceInit() {}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy