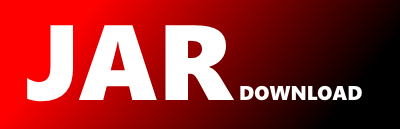
ucar.nc2.stream.NcStreamProto Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ucar/nc2/stream/ncStream.proto
package ucar.nc2.stream;
public final class NcStreamProto {
private NcStreamProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public enum DataType
implements com.google.protobuf.ProtocolMessageEnum {
CHAR(0, 0),
BYTE(1, 1),
SHORT(2, 2),
INT(3, 3),
LONG(4, 4),
FLOAT(5, 5),
DOUBLE(6, 6),
STRING(7, 7),
STRUCTURE(8, 8),
SEQUENCE(9, 9),
ENUM1(10, 10),
ENUM2(11, 11),
ENUM4(12, 12),
OPAQUE(13, 13),
;
public final int getNumber() { return value; }
public static DataType valueOf(int value) {
switch (value) {
case 0: return CHAR;
case 1: return BYTE;
case 2: return SHORT;
case 3: return INT;
case 4: return LONG;
case 5: return FLOAT;
case 6: return DOUBLE;
case 7: return STRING;
case 8: return STRUCTURE;
case 9: return SEQUENCE;
case 10: return ENUM1;
case 11: return ENUM2;
case 12: return ENUM4;
case 13: return OPAQUE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DataType findValueByNumber(int number) {
return DataType.valueOf(number)
; }
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.getDescriptor().getEnumTypes().get(0);
}
private static final DataType[] VALUES = {
CHAR, BYTE, SHORT, INT, LONG, FLOAT, DOUBLE, STRING, STRUCTURE, SEQUENCE, ENUM1, ENUM2, ENUM4, OPAQUE,
};
public static DataType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private DataType(int index, int value) {
this.index = index;
this.value = value;
}
static {
ucar.nc2.stream.NcStreamProto.getDescriptor();
}
// @@protoc_insertion_point(enum_scope:ncstream.DataType)
}
public enum Compress
implements com.google.protobuf.ProtocolMessageEnum {
NONE(0, 0),
DEFLATE(1, 1),
;
public final int getNumber() { return value; }
public static Compress valueOf(int value) {
switch (value) {
case 0: return NONE;
case 1: return DEFLATE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Compress findValueByNumber(int number) {
return Compress.valueOf(number)
; }
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.getDescriptor().getEnumTypes().get(1);
}
private static final Compress[] VALUES = {
NONE, DEFLATE,
};
public static Compress valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private Compress(int index, int value) {
this.index = index;
this.value = value;
}
static {
ucar.nc2.stream.NcStreamProto.getDescriptor();
}
// @@protoc_insertion_point(enum_scope:ncstream.Compress)
}
public static final class Attribute extends
com.google.protobuf.GeneratedMessage {
// Use Attribute.newBuilder() to construct.
private Attribute() {
initFields();
}
private Attribute(boolean noInit) {}
private static final Attribute defaultInstance;
public static Attribute getDefaultInstance() {
return defaultInstance;
}
public Attribute getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Attribute_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Attribute_fieldAccessorTable;
}
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
STRING(0, 0),
BYTE(1, 1),
SHORT(2, 2),
INT(3, 3),
LONG(4, 4),
FLOAT(5, 5),
DOUBLE(6, 6),
;
public final int getNumber() { return value; }
public static Type valueOf(int value) {
switch (value) {
case 0: return STRING;
case 1: return BYTE;
case 2: return SHORT;
case 3: return INT;
case 4: return LONG;
case 5: return FLOAT;
case 6: return DOUBLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.valueOf(number)
; }
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.Attribute.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = {
STRING, BYTE, SHORT, INT, LONG, FLOAT, DOUBLE,
};
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private Type(int index, int value) {
this.index = index;
this.value = value;
}
static {
ucar.nc2.stream.NcStreamProto.getDescriptor();
}
// @@protoc_insertion_point(enum_scope:ncstream.Attribute.Type)
}
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// required .ncstream.Attribute.Type type = 2;
public static final int TYPE_FIELD_NUMBER = 2;
private boolean hasType;
private ucar.nc2.stream.NcStreamProto.Attribute.Type type_;
public boolean hasType() { return hasType; }
public ucar.nc2.stream.NcStreamProto.Attribute.Type getType() { return type_; }
// required uint32 len = 3;
public static final int LEN_FIELD_NUMBER = 3;
private boolean hasLen;
private int len_ = 0;
public boolean hasLen() { return hasLen; }
public int getLen() { return len_; }
// optional bytes data = 4;
public static final int DATA_FIELD_NUMBER = 4;
private boolean hasData;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
public boolean hasData() { return hasData; }
public com.google.protobuf.ByteString getData() { return data_; }
// repeated string sdata = 5;
public static final int SDATA_FIELD_NUMBER = 5;
private java.util.List sdata_ =
java.util.Collections.emptyList();
public java.util.List getSdataList() {
return sdata_;
}
public int getSdataCount() { return sdata_.size(); }
public java.lang.String getSdata(int index) {
return sdata_.get(index);
}
private void initFields() {
type_ = ucar.nc2.stream.NcStreamProto.Attribute.Type.STRING;
}
public final boolean isInitialized() {
if (!hasName) return false;
if (!hasType) return false;
if (!hasLen) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasName()) {
output.writeString(1, getName());
}
if (hasType()) {
output.writeEnum(2, getType().getNumber());
}
if (hasLen()) {
output.writeUInt32(3, getLen());
}
if (hasData()) {
output.writeBytes(4, getData());
}
for (java.lang.String element : getSdataList()) {
output.writeString(5, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
if (hasType()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, getType().getNumber());
}
if (hasLen()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, getLen());
}
if (hasData()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getData());
}
{
int dataSize = 0;
for (java.lang.String element : getSdataList()) {
dataSize += com.google.protobuf.CodedOutputStream
.computeStringSizeNoTag(element);
}
size += dataSize;
size += 1 * getSdataList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Attribute parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Attribute prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.Attribute result;
// Construct using ucar.nc2.stream.NcStreamProto.Attribute.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.Attribute();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.Attribute internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.Attribute();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.Attribute.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.Attribute getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Attribute.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.Attribute build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.Attribute buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.Attribute buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.sdata_ != java.util.Collections.EMPTY_LIST) {
result.sdata_ =
java.util.Collections.unmodifiableList(result.sdata_);
}
ucar.nc2.stream.NcStreamProto.Attribute returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Attribute) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Attribute)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Attribute other) {
if (other == ucar.nc2.stream.NcStreamProto.Attribute.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasLen()) {
setLen(other.getLen());
}
if (other.hasData()) {
setData(other.getData());
}
if (!other.sdata_.isEmpty()) {
if (result.sdata_.isEmpty()) {
result.sdata_ = new java.util.ArrayList();
}
result.sdata_.addAll(other.sdata_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 16: {
int rawValue = input.readEnum();
ucar.nc2.stream.NcStreamProto.Attribute.Type value = ucar.nc2.stream.NcStreamProto.Attribute.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
setType(value);
}
break;
}
case 24: {
setLen(input.readUInt32());
break;
}
case 34: {
setData(input.readBytes());
break;
}
case 42: {
addSdata(input.readString());
break;
}
}
}
}
// required string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// required .ncstream.Attribute.Type type = 2;
public boolean hasType() {
return result.hasType();
}
public ucar.nc2.stream.NcStreamProto.Attribute.Type getType() {
return result.getType();
}
public Builder setType(ucar.nc2.stream.NcStreamProto.Attribute.Type value) {
if (value == null) {
throw new NullPointerException();
}
result.hasType = true;
result.type_ = value;
return this;
}
public Builder clearType() {
result.hasType = false;
result.type_ = ucar.nc2.stream.NcStreamProto.Attribute.Type.STRING;
return this;
}
// required uint32 len = 3;
public boolean hasLen() {
return result.hasLen();
}
public int getLen() {
return result.getLen();
}
public Builder setLen(int value) {
result.hasLen = true;
result.len_ = value;
return this;
}
public Builder clearLen() {
result.hasLen = false;
result.len_ = 0;
return this;
}
// optional bytes data = 4;
public boolean hasData() {
return result.hasData();
}
public com.google.protobuf.ByteString getData() {
return result.getData();
}
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
result.hasData = true;
result.data_ = value;
return this;
}
public Builder clearData() {
result.hasData = false;
result.data_ = getDefaultInstance().getData();
return this;
}
// repeated string sdata = 5;
public java.util.List getSdataList() {
return java.util.Collections.unmodifiableList(result.sdata_);
}
public int getSdataCount() {
return result.getSdataCount();
}
public java.lang.String getSdata(int index) {
return result.getSdata(index);
}
public Builder setSdata(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.sdata_.set(index, value);
return this;
}
public Builder addSdata(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
if (result.sdata_.isEmpty()) {
result.sdata_ = new java.util.ArrayList();
}
result.sdata_.add(value);
return this;
}
public Builder addAllSdata(
java.lang.Iterable extends java.lang.String> values) {
if (result.sdata_.isEmpty()) {
result.sdata_ = new java.util.ArrayList();
}
super.addAll(values, result.sdata_);
return this;
}
public Builder clearSdata() {
result.sdata_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Attribute)
}
static {
defaultInstance = new Attribute(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Attribute)
}
public static final class Dimension extends
com.google.protobuf.GeneratedMessage {
// Use Dimension.newBuilder() to construct.
private Dimension() {
initFields();
}
private Dimension(boolean noInit) {}
private static final Dimension defaultInstance;
public static Dimension getDefaultInstance() {
return defaultInstance;
}
public Dimension getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Dimension_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Dimension_fieldAccessorTable;
}
// optional string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// optional uint64 length = 2;
public static final int LENGTH_FIELD_NUMBER = 2;
private boolean hasLength;
private long length_ = 0L;
public boolean hasLength() { return hasLength; }
public long getLength() { return length_; }
// optional bool isUnlimited = 3 [default = false];
public static final int ISUNLIMITED_FIELD_NUMBER = 3;
private boolean hasIsUnlimited;
private boolean isUnlimited_ = false;
public boolean hasIsUnlimited() { return hasIsUnlimited; }
public boolean getIsUnlimited() { return isUnlimited_; }
// optional bool isVlen = 4 [default = false];
public static final int ISVLEN_FIELD_NUMBER = 4;
private boolean hasIsVlen;
private boolean isVlen_ = false;
public boolean hasIsVlen() { return hasIsVlen; }
public boolean getIsVlen() { return isVlen_; }
// optional bool isPrivate = 5 [default = false];
public static final int ISPRIVATE_FIELD_NUMBER = 5;
private boolean hasIsPrivate;
private boolean isPrivate_ = false;
public boolean hasIsPrivate() { return hasIsPrivate; }
public boolean getIsPrivate() { return isPrivate_; }
private void initFields() {
}
public final boolean isInitialized() {
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasName()) {
output.writeString(1, getName());
}
if (hasLength()) {
output.writeUInt64(2, getLength());
}
if (hasIsUnlimited()) {
output.writeBool(3, getIsUnlimited());
}
if (hasIsVlen()) {
output.writeBool(4, getIsVlen());
}
if (hasIsPrivate()) {
output.writeBool(5, getIsPrivate());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
if (hasLength()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, getLength());
}
if (hasIsUnlimited()) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, getIsUnlimited());
}
if (hasIsVlen()) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, getIsVlen());
}
if (hasIsPrivate()) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, getIsPrivate());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Dimension parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Dimension prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.Dimension result;
// Construct using ucar.nc2.stream.NcStreamProto.Dimension.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.Dimension();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.Dimension internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.Dimension();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.Dimension.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.Dimension getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Dimension.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.Dimension build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.Dimension buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.Dimension buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
ucar.nc2.stream.NcStreamProto.Dimension returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Dimension) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Dimension)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Dimension other) {
if (other == ucar.nc2.stream.NcStreamProto.Dimension.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasLength()) {
setLength(other.getLength());
}
if (other.hasIsUnlimited()) {
setIsUnlimited(other.getIsUnlimited());
}
if (other.hasIsVlen()) {
setIsVlen(other.getIsVlen());
}
if (other.hasIsPrivate()) {
setIsPrivate(other.getIsPrivate());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 16: {
setLength(input.readUInt64());
break;
}
case 24: {
setIsUnlimited(input.readBool());
break;
}
case 32: {
setIsVlen(input.readBool());
break;
}
case 40: {
setIsPrivate(input.readBool());
break;
}
}
}
}
// optional string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// optional uint64 length = 2;
public boolean hasLength() {
return result.hasLength();
}
public long getLength() {
return result.getLength();
}
public Builder setLength(long value) {
result.hasLength = true;
result.length_ = value;
return this;
}
public Builder clearLength() {
result.hasLength = false;
result.length_ = 0L;
return this;
}
// optional bool isUnlimited = 3 [default = false];
public boolean hasIsUnlimited() {
return result.hasIsUnlimited();
}
public boolean getIsUnlimited() {
return result.getIsUnlimited();
}
public Builder setIsUnlimited(boolean value) {
result.hasIsUnlimited = true;
result.isUnlimited_ = value;
return this;
}
public Builder clearIsUnlimited() {
result.hasIsUnlimited = false;
result.isUnlimited_ = false;
return this;
}
// optional bool isVlen = 4 [default = false];
public boolean hasIsVlen() {
return result.hasIsVlen();
}
public boolean getIsVlen() {
return result.getIsVlen();
}
public Builder setIsVlen(boolean value) {
result.hasIsVlen = true;
result.isVlen_ = value;
return this;
}
public Builder clearIsVlen() {
result.hasIsVlen = false;
result.isVlen_ = false;
return this;
}
// optional bool isPrivate = 5 [default = false];
public boolean hasIsPrivate() {
return result.hasIsPrivate();
}
public boolean getIsPrivate() {
return result.getIsPrivate();
}
public Builder setIsPrivate(boolean value) {
result.hasIsPrivate = true;
result.isPrivate_ = value;
return this;
}
public Builder clearIsPrivate() {
result.hasIsPrivate = false;
result.isPrivate_ = false;
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Dimension)
}
static {
defaultInstance = new Dimension(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Dimension)
}
public static final class Variable extends
com.google.protobuf.GeneratedMessage {
// Use Variable.newBuilder() to construct.
private Variable() {
initFields();
}
private Variable(boolean noInit) {}
private static final Variable defaultInstance;
public static Variable getDefaultInstance() {
return defaultInstance;
}
public Variable getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Variable_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Variable_fieldAccessorTable;
}
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// required .ncstream.DataType dataType = 2;
public static final int DATATYPE_FIELD_NUMBER = 2;
private boolean hasDataType;
private ucar.nc2.stream.NcStreamProto.DataType dataType_;
public boolean hasDataType() { return hasDataType; }
public ucar.nc2.stream.NcStreamProto.DataType getDataType() { return dataType_; }
// repeated .ncstream.Dimension shape = 3;
public static final int SHAPE_FIELD_NUMBER = 3;
private java.util.List shape_ =
java.util.Collections.emptyList();
public java.util.List getShapeList() {
return shape_;
}
public int getShapeCount() { return shape_.size(); }
public ucar.nc2.stream.NcStreamProto.Dimension getShape(int index) {
return shape_.get(index);
}
// repeated .ncstream.Attribute atts = 4;
public static final int ATTS_FIELD_NUMBER = 4;
private java.util.List atts_ =
java.util.Collections.emptyList();
public java.util.List getAttsList() {
return atts_;
}
public int getAttsCount() { return atts_.size(); }
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
return atts_.get(index);
}
// optional bool unsigned = 5 [default = false];
public static final int UNSIGNED_FIELD_NUMBER = 5;
private boolean hasUnsigned;
private boolean unsigned_ = false;
public boolean hasUnsigned() { return hasUnsigned; }
public boolean getUnsigned() { return unsigned_; }
// optional bytes data = 6;
public static final int DATA_FIELD_NUMBER = 6;
private boolean hasData;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
public boolean hasData() { return hasData; }
public com.google.protobuf.ByteString getData() { return data_; }
// optional string enumType = 7;
public static final int ENUMTYPE_FIELD_NUMBER = 7;
private boolean hasEnumType;
private java.lang.String enumType_ = "";
public boolean hasEnumType() { return hasEnumType; }
public java.lang.String getEnumType() { return enumType_; }
// repeated uint32 dimIndex = 8;
public static final int DIMINDEX_FIELD_NUMBER = 8;
private java.util.List dimIndex_ =
java.util.Collections.emptyList();
public java.util.List getDimIndexList() {
return dimIndex_;
}
public int getDimIndexCount() { return dimIndex_.size(); }
public int getDimIndex(int index) {
return dimIndex_.get(index);
}
private void initFields() {
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
}
public final boolean isInitialized() {
if (!hasName) return false;
if (!hasDataType) return false;
for (ucar.nc2.stream.NcStreamProto.Attribute element : getAttsList()) {
if (!element.isInitialized()) return false;
}
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasName()) {
output.writeString(1, getName());
}
if (hasDataType()) {
output.writeEnum(2, getDataType().getNumber());
}
for (ucar.nc2.stream.NcStreamProto.Dimension element : getShapeList()) {
output.writeMessage(3, element);
}
for (ucar.nc2.stream.NcStreamProto.Attribute element : getAttsList()) {
output.writeMessage(4, element);
}
if (hasUnsigned()) {
output.writeBool(5, getUnsigned());
}
if (hasData()) {
output.writeBytes(6, getData());
}
if (hasEnumType()) {
output.writeString(7, getEnumType());
}
for (int element : getDimIndexList()) {
output.writeUInt32(8, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
if (hasDataType()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, getDataType().getNumber());
}
for (ucar.nc2.stream.NcStreamProto.Dimension element : getShapeList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, element);
}
for (ucar.nc2.stream.NcStreamProto.Attribute element : getAttsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, element);
}
if (hasUnsigned()) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, getUnsigned());
}
if (hasData()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, getData());
}
if (hasEnumType()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(7, getEnumType());
}
{
int dataSize = 0;
for (int element : getDimIndexList()) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(element);
}
size += dataSize;
size += 1 * getDimIndexList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Variable parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Variable parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Variable parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Variable prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.Variable result;
// Construct using ucar.nc2.stream.NcStreamProto.Variable.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.Variable();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.Variable internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.Variable();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.Variable.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.Variable getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Variable.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.Variable build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.Variable buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.Variable buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.shape_ != java.util.Collections.EMPTY_LIST) {
result.shape_ =
java.util.Collections.unmodifiableList(result.shape_);
}
if (result.atts_ != java.util.Collections.EMPTY_LIST) {
result.atts_ =
java.util.Collections.unmodifiableList(result.atts_);
}
if (result.dimIndex_ != java.util.Collections.EMPTY_LIST) {
result.dimIndex_ =
java.util.Collections.unmodifiableList(result.dimIndex_);
}
ucar.nc2.stream.NcStreamProto.Variable returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Variable) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Variable)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Variable other) {
if (other == ucar.nc2.stream.NcStreamProto.Variable.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasDataType()) {
setDataType(other.getDataType());
}
if (!other.shape_.isEmpty()) {
if (result.shape_.isEmpty()) {
result.shape_ = new java.util.ArrayList();
}
result.shape_.addAll(other.shape_);
}
if (!other.atts_.isEmpty()) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.addAll(other.atts_);
}
if (other.hasUnsigned()) {
setUnsigned(other.getUnsigned());
}
if (other.hasData()) {
setData(other.getData());
}
if (other.hasEnumType()) {
setEnumType(other.getEnumType());
}
if (!other.dimIndex_.isEmpty()) {
if (result.dimIndex_.isEmpty()) {
result.dimIndex_ = new java.util.ArrayList();
}
result.dimIndex_.addAll(other.dimIndex_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 16: {
int rawValue = input.readEnum();
ucar.nc2.stream.NcStreamProto.DataType value = ucar.nc2.stream.NcStreamProto.DataType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
setDataType(value);
}
break;
}
case 26: {
ucar.nc2.stream.NcStreamProto.Dimension.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Dimension.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addShape(subBuilder.buildPartial());
break;
}
case 34: {
ucar.nc2.stream.NcStreamProto.Attribute.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Attribute.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addAtts(subBuilder.buildPartial());
break;
}
case 40: {
setUnsigned(input.readBool());
break;
}
case 50: {
setData(input.readBytes());
break;
}
case 58: {
setEnumType(input.readString());
break;
}
case 64: {
addDimIndex(input.readUInt32());
break;
}
case 66: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
while (input.getBytesUntilLimit() > 0) {
addDimIndex(input.readUInt32());
}
input.popLimit(limit);
break;
}
}
}
}
// required string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// required .ncstream.DataType dataType = 2;
public boolean hasDataType() {
return result.hasDataType();
}
public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
return result.getDataType();
}
public Builder setDataType(ucar.nc2.stream.NcStreamProto.DataType value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDataType = true;
result.dataType_ = value;
return this;
}
public Builder clearDataType() {
result.hasDataType = false;
result.dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
return this;
}
// repeated .ncstream.Dimension shape = 3;
public java.util.List getShapeList() {
return java.util.Collections.unmodifiableList(result.shape_);
}
public int getShapeCount() {
return result.getShapeCount();
}
public ucar.nc2.stream.NcStreamProto.Dimension getShape(int index) {
return result.getShape(index);
}
public Builder setShape(int index, ucar.nc2.stream.NcStreamProto.Dimension value) {
if (value == null) {
throw new NullPointerException();
}
result.shape_.set(index, value);
return this;
}
public Builder setShape(int index, ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
result.shape_.set(index, builderForValue.build());
return this;
}
public Builder addShape(ucar.nc2.stream.NcStreamProto.Dimension value) {
if (value == null) {
throw new NullPointerException();
}
if (result.shape_.isEmpty()) {
result.shape_ = new java.util.ArrayList();
}
result.shape_.add(value);
return this;
}
public Builder addShape(ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (result.shape_.isEmpty()) {
result.shape_ = new java.util.ArrayList();
}
result.shape_.add(builderForValue.build());
return this;
}
public Builder addAllShape(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Dimension> values) {
if (result.shape_.isEmpty()) {
result.shape_ = new java.util.ArrayList();
}
super.addAll(values, result.shape_);
return this;
}
public Builder clearShape() {
result.shape_ = java.util.Collections.emptyList();
return this;
}
// repeated .ncstream.Attribute atts = 4;
public java.util.List getAttsList() {
return java.util.Collections.unmodifiableList(result.atts_);
}
public int getAttsCount() {
return result.getAttsCount();
}
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
return result.getAtts(index);
}
public Builder setAtts(int index, ucar.nc2.stream.NcStreamProto.Attribute value) {
if (value == null) {
throw new NullPointerException();
}
result.atts_.set(index, value);
return this;
}
public Builder setAtts(int index, ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
result.atts_.set(index, builderForValue.build());
return this;
}
public Builder addAtts(ucar.nc2.stream.NcStreamProto.Attribute value) {
if (value == null) {
throw new NullPointerException();
}
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.add(value);
return this;
}
public Builder addAtts(ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.add(builderForValue.build());
return this;
}
public Builder addAllAtts(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Attribute> values) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
super.addAll(values, result.atts_);
return this;
}
public Builder clearAtts() {
result.atts_ = java.util.Collections.emptyList();
return this;
}
// optional bool unsigned = 5 [default = false];
public boolean hasUnsigned() {
return result.hasUnsigned();
}
public boolean getUnsigned() {
return result.getUnsigned();
}
public Builder setUnsigned(boolean value) {
result.hasUnsigned = true;
result.unsigned_ = value;
return this;
}
public Builder clearUnsigned() {
result.hasUnsigned = false;
result.unsigned_ = false;
return this;
}
// optional bytes data = 6;
public boolean hasData() {
return result.hasData();
}
public com.google.protobuf.ByteString getData() {
return result.getData();
}
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
result.hasData = true;
result.data_ = value;
return this;
}
public Builder clearData() {
result.hasData = false;
result.data_ = getDefaultInstance().getData();
return this;
}
// optional string enumType = 7;
public boolean hasEnumType() {
return result.hasEnumType();
}
public java.lang.String getEnumType() {
return result.getEnumType();
}
public Builder setEnumType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasEnumType = true;
result.enumType_ = value;
return this;
}
public Builder clearEnumType() {
result.hasEnumType = false;
result.enumType_ = getDefaultInstance().getEnumType();
return this;
}
// repeated uint32 dimIndex = 8;
public java.util.List getDimIndexList() {
return java.util.Collections.unmodifiableList(result.dimIndex_);
}
public int getDimIndexCount() {
return result.getDimIndexCount();
}
public int getDimIndex(int index) {
return result.getDimIndex(index);
}
public Builder setDimIndex(int index, int value) {
result.dimIndex_.set(index, value);
return this;
}
public Builder addDimIndex(int value) {
if (result.dimIndex_.isEmpty()) {
result.dimIndex_ = new java.util.ArrayList();
}
result.dimIndex_.add(value);
return this;
}
public Builder addAllDimIndex(
java.lang.Iterable extends java.lang.Integer> values) {
if (result.dimIndex_.isEmpty()) {
result.dimIndex_ = new java.util.ArrayList();
}
super.addAll(values, result.dimIndex_);
return this;
}
public Builder clearDimIndex() {
result.dimIndex_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Variable)
}
static {
defaultInstance = new Variable(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Variable)
}
public static final class Structure extends
com.google.protobuf.GeneratedMessage {
// Use Structure.newBuilder() to construct.
private Structure() {
initFields();
}
private Structure(boolean noInit) {}
private static final Structure defaultInstance;
public static Structure getDefaultInstance() {
return defaultInstance;
}
public Structure getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Structure_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Structure_fieldAccessorTable;
}
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// required .ncstream.DataType dataType = 2;
public static final int DATATYPE_FIELD_NUMBER = 2;
private boolean hasDataType;
private ucar.nc2.stream.NcStreamProto.DataType dataType_;
public boolean hasDataType() { return hasDataType; }
public ucar.nc2.stream.NcStreamProto.DataType getDataType() { return dataType_; }
// repeated .ncstream.Dimension shape = 3;
public static final int SHAPE_FIELD_NUMBER = 3;
private java.util.List shape_ =
java.util.Collections.emptyList();
public java.util.List getShapeList() {
return shape_;
}
public int getShapeCount() { return shape_.size(); }
public ucar.nc2.stream.NcStreamProto.Dimension getShape(int index) {
return shape_.get(index);
}
// repeated .ncstream.Attribute atts = 4;
public static final int ATTS_FIELD_NUMBER = 4;
private java.util.List atts_ =
java.util.Collections.emptyList();
public java.util.List getAttsList() {
return atts_;
}
public int getAttsCount() { return atts_.size(); }
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
return atts_.get(index);
}
// repeated .ncstream.Variable vars = 5;
public static final int VARS_FIELD_NUMBER = 5;
private java.util.List vars_ =
java.util.Collections.emptyList();
public java.util.List getVarsList() {
return vars_;
}
public int getVarsCount() { return vars_.size(); }
public ucar.nc2.stream.NcStreamProto.Variable getVars(int index) {
return vars_.get(index);
}
// repeated .ncstream.Structure structs = 6;
public static final int STRUCTS_FIELD_NUMBER = 6;
private java.util.List structs_ =
java.util.Collections.emptyList();
public java.util.List getStructsList() {
return structs_;
}
public int getStructsCount() { return structs_.size(); }
public ucar.nc2.stream.NcStreamProto.Structure getStructs(int index) {
return structs_.get(index);
}
private void initFields() {
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
}
public final boolean isInitialized() {
if (!hasName) return false;
if (!hasDataType) return false;
for (ucar.nc2.stream.NcStreamProto.Attribute element : getAttsList()) {
if (!element.isInitialized()) return false;
}
for (ucar.nc2.stream.NcStreamProto.Variable element : getVarsList()) {
if (!element.isInitialized()) return false;
}
for (ucar.nc2.stream.NcStreamProto.Structure element : getStructsList()) {
if (!element.isInitialized()) return false;
}
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasName()) {
output.writeString(1, getName());
}
if (hasDataType()) {
output.writeEnum(2, getDataType().getNumber());
}
for (ucar.nc2.stream.NcStreamProto.Dimension element : getShapeList()) {
output.writeMessage(3, element);
}
for (ucar.nc2.stream.NcStreamProto.Attribute element : getAttsList()) {
output.writeMessage(4, element);
}
for (ucar.nc2.stream.NcStreamProto.Variable element : getVarsList()) {
output.writeMessage(5, element);
}
for (ucar.nc2.stream.NcStreamProto.Structure element : getStructsList()) {
output.writeMessage(6, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
if (hasDataType()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, getDataType().getNumber());
}
for (ucar.nc2.stream.NcStreamProto.Dimension element : getShapeList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, element);
}
for (ucar.nc2.stream.NcStreamProto.Attribute element : getAttsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, element);
}
for (ucar.nc2.stream.NcStreamProto.Variable element : getVarsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, element);
}
for (ucar.nc2.stream.NcStreamProto.Structure element : getStructsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Structure parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Structure parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Structure parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Structure prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.Structure result;
// Construct using ucar.nc2.stream.NcStreamProto.Structure.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.Structure();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.Structure internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.Structure();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.Structure.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.Structure getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Structure.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.Structure build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.Structure buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.Structure buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.shape_ != java.util.Collections.EMPTY_LIST) {
result.shape_ =
java.util.Collections.unmodifiableList(result.shape_);
}
if (result.atts_ != java.util.Collections.EMPTY_LIST) {
result.atts_ =
java.util.Collections.unmodifiableList(result.atts_);
}
if (result.vars_ != java.util.Collections.EMPTY_LIST) {
result.vars_ =
java.util.Collections.unmodifiableList(result.vars_);
}
if (result.structs_ != java.util.Collections.EMPTY_LIST) {
result.structs_ =
java.util.Collections.unmodifiableList(result.structs_);
}
ucar.nc2.stream.NcStreamProto.Structure returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Structure) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Structure)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Structure other) {
if (other == ucar.nc2.stream.NcStreamProto.Structure.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasDataType()) {
setDataType(other.getDataType());
}
if (!other.shape_.isEmpty()) {
if (result.shape_.isEmpty()) {
result.shape_ = new java.util.ArrayList();
}
result.shape_.addAll(other.shape_);
}
if (!other.atts_.isEmpty()) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.addAll(other.atts_);
}
if (!other.vars_.isEmpty()) {
if (result.vars_.isEmpty()) {
result.vars_ = new java.util.ArrayList();
}
result.vars_.addAll(other.vars_);
}
if (!other.structs_.isEmpty()) {
if (result.structs_.isEmpty()) {
result.structs_ = new java.util.ArrayList();
}
result.structs_.addAll(other.structs_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 16: {
int rawValue = input.readEnum();
ucar.nc2.stream.NcStreamProto.DataType value = ucar.nc2.stream.NcStreamProto.DataType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
setDataType(value);
}
break;
}
case 26: {
ucar.nc2.stream.NcStreamProto.Dimension.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Dimension.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addShape(subBuilder.buildPartial());
break;
}
case 34: {
ucar.nc2.stream.NcStreamProto.Attribute.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Attribute.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addAtts(subBuilder.buildPartial());
break;
}
case 42: {
ucar.nc2.stream.NcStreamProto.Variable.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Variable.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addVars(subBuilder.buildPartial());
break;
}
case 50: {
ucar.nc2.stream.NcStreamProto.Structure.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Structure.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addStructs(subBuilder.buildPartial());
break;
}
}
}
}
// required string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// required .ncstream.DataType dataType = 2;
public boolean hasDataType() {
return result.hasDataType();
}
public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
return result.getDataType();
}
public Builder setDataType(ucar.nc2.stream.NcStreamProto.DataType value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDataType = true;
result.dataType_ = value;
return this;
}
public Builder clearDataType() {
result.hasDataType = false;
result.dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
return this;
}
// repeated .ncstream.Dimension shape = 3;
public java.util.List getShapeList() {
return java.util.Collections.unmodifiableList(result.shape_);
}
public int getShapeCount() {
return result.getShapeCount();
}
public ucar.nc2.stream.NcStreamProto.Dimension getShape(int index) {
return result.getShape(index);
}
public Builder setShape(int index, ucar.nc2.stream.NcStreamProto.Dimension value) {
if (value == null) {
throw new NullPointerException();
}
result.shape_.set(index, value);
return this;
}
public Builder setShape(int index, ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
result.shape_.set(index, builderForValue.build());
return this;
}
public Builder addShape(ucar.nc2.stream.NcStreamProto.Dimension value) {
if (value == null) {
throw new NullPointerException();
}
if (result.shape_.isEmpty()) {
result.shape_ = new java.util.ArrayList();
}
result.shape_.add(value);
return this;
}
public Builder addShape(ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (result.shape_.isEmpty()) {
result.shape_ = new java.util.ArrayList();
}
result.shape_.add(builderForValue.build());
return this;
}
public Builder addAllShape(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Dimension> values) {
if (result.shape_.isEmpty()) {
result.shape_ = new java.util.ArrayList();
}
super.addAll(values, result.shape_);
return this;
}
public Builder clearShape() {
result.shape_ = java.util.Collections.emptyList();
return this;
}
// repeated .ncstream.Attribute atts = 4;
public java.util.List getAttsList() {
return java.util.Collections.unmodifiableList(result.atts_);
}
public int getAttsCount() {
return result.getAttsCount();
}
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
return result.getAtts(index);
}
public Builder setAtts(int index, ucar.nc2.stream.NcStreamProto.Attribute value) {
if (value == null) {
throw new NullPointerException();
}
result.atts_.set(index, value);
return this;
}
public Builder setAtts(int index, ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
result.atts_.set(index, builderForValue.build());
return this;
}
public Builder addAtts(ucar.nc2.stream.NcStreamProto.Attribute value) {
if (value == null) {
throw new NullPointerException();
}
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.add(value);
return this;
}
public Builder addAtts(ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.add(builderForValue.build());
return this;
}
public Builder addAllAtts(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Attribute> values) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
super.addAll(values, result.atts_);
return this;
}
public Builder clearAtts() {
result.atts_ = java.util.Collections.emptyList();
return this;
}
// repeated .ncstream.Variable vars = 5;
public java.util.List getVarsList() {
return java.util.Collections.unmodifiableList(result.vars_);
}
public int getVarsCount() {
return result.getVarsCount();
}
public ucar.nc2.stream.NcStreamProto.Variable getVars(int index) {
return result.getVars(index);
}
public Builder setVars(int index, ucar.nc2.stream.NcStreamProto.Variable value) {
if (value == null) {
throw new NullPointerException();
}
result.vars_.set(index, value);
return this;
}
public Builder setVars(int index, ucar.nc2.stream.NcStreamProto.Variable.Builder builderForValue) {
result.vars_.set(index, builderForValue.build());
return this;
}
public Builder addVars(ucar.nc2.stream.NcStreamProto.Variable value) {
if (value == null) {
throw new NullPointerException();
}
if (result.vars_.isEmpty()) {
result.vars_ = new java.util.ArrayList();
}
result.vars_.add(value);
return this;
}
public Builder addVars(ucar.nc2.stream.NcStreamProto.Variable.Builder builderForValue) {
if (result.vars_.isEmpty()) {
result.vars_ = new java.util.ArrayList();
}
result.vars_.add(builderForValue.build());
return this;
}
public Builder addAllVars(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Variable> values) {
if (result.vars_.isEmpty()) {
result.vars_ = new java.util.ArrayList();
}
super.addAll(values, result.vars_);
return this;
}
public Builder clearVars() {
result.vars_ = java.util.Collections.emptyList();
return this;
}
// repeated .ncstream.Structure structs = 6;
public java.util.List getStructsList() {
return java.util.Collections.unmodifiableList(result.structs_);
}
public int getStructsCount() {
return result.getStructsCount();
}
public ucar.nc2.stream.NcStreamProto.Structure getStructs(int index) {
return result.getStructs(index);
}
public Builder setStructs(int index, ucar.nc2.stream.NcStreamProto.Structure value) {
if (value == null) {
throw new NullPointerException();
}
result.structs_.set(index, value);
return this;
}
public Builder setStructs(int index, ucar.nc2.stream.NcStreamProto.Structure.Builder builderForValue) {
result.structs_.set(index, builderForValue.build());
return this;
}
public Builder addStructs(ucar.nc2.stream.NcStreamProto.Structure value) {
if (value == null) {
throw new NullPointerException();
}
if (result.structs_.isEmpty()) {
result.structs_ = new java.util.ArrayList();
}
result.structs_.add(value);
return this;
}
public Builder addStructs(ucar.nc2.stream.NcStreamProto.Structure.Builder builderForValue) {
if (result.structs_.isEmpty()) {
result.structs_ = new java.util.ArrayList();
}
result.structs_.add(builderForValue.build());
return this;
}
public Builder addAllStructs(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Structure> values) {
if (result.structs_.isEmpty()) {
result.structs_ = new java.util.ArrayList();
}
super.addAll(values, result.structs_);
return this;
}
public Builder clearStructs() {
result.structs_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Structure)
}
static {
defaultInstance = new Structure(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Structure)
}
public static final class EnumTypedef extends
com.google.protobuf.GeneratedMessage {
// Use EnumTypedef.newBuilder() to construct.
private EnumTypedef() {
initFields();
}
private EnumTypedef(boolean noInit) {}
private static final EnumTypedef defaultInstance;
public static EnumTypedef getDefaultInstance() {
return defaultInstance;
}
public EnumTypedef getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_fieldAccessorTable;
}
public static final class EnumType extends
com.google.protobuf.GeneratedMessage {
// Use EnumType.newBuilder() to construct.
private EnumType() {
initFields();
}
private EnumType(boolean noInit) {}
private static final EnumType defaultInstance;
public static EnumType getDefaultInstance() {
return defaultInstance;
}
public EnumType getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_EnumType_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_EnumTypedef_EnumType_fieldAccessorTable;
}
// required uint32 code = 1;
public static final int CODE_FIELD_NUMBER = 1;
private boolean hasCode;
private int code_ = 0;
public boolean hasCode() { return hasCode; }
public int getCode() { return code_; }
// required string value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private boolean hasValue;
private java.lang.String value_ = "";
public boolean hasValue() { return hasValue; }
public java.lang.String getValue() { return value_; }
private void initFields() {
}
public final boolean isInitialized() {
if (!hasCode) return false;
if (!hasValue) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasCode()) {
output.writeUInt32(1, getCode());
}
if (hasValue()) {
output.writeString(2, getValue());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasCode()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, getCode());
}
if (hasValue()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getValue());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType result;
// Construct using ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType other) {
if (other == ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.getDefaultInstance()) return this;
if (other.hasCode()) {
setCode(other.getCode());
}
if (other.hasValue()) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 8: {
setCode(input.readUInt32());
break;
}
case 18: {
setValue(input.readString());
break;
}
}
}
}
// required uint32 code = 1;
public boolean hasCode() {
return result.hasCode();
}
public int getCode() {
return result.getCode();
}
public Builder setCode(int value) {
result.hasCode = true;
result.code_ = value;
return this;
}
public Builder clearCode() {
result.hasCode = false;
result.code_ = 0;
return this;
}
// required string value = 2;
public boolean hasValue() {
return result.hasValue();
}
public java.lang.String getValue() {
return result.getValue();
}
public Builder setValue(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasValue = true;
result.value_ = value;
return this;
}
public Builder clearValue() {
result.hasValue = false;
result.value_ = getDefaultInstance().getValue();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.EnumTypedef.EnumType)
}
static {
defaultInstance = new EnumType(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.EnumTypedef.EnumType)
}
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// repeated .ncstream.EnumTypedef.EnumType map = 2;
public static final int MAP_FIELD_NUMBER = 2;
private java.util.List map_ =
java.util.Collections.emptyList();
public java.util.List getMapList() {
return map_;
}
public int getMapCount() { return map_.size(); }
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType getMap(int index) {
return map_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
if (!hasName) return false;
for (ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType element : getMapList()) {
if (!element.isInitialized()) return false;
}
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasName()) {
output.writeString(1, getName());
}
for (ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType element : getMapList()) {
output.writeMessage(2, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
for (ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType element : getMapList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.EnumTypedef parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.EnumTypedef prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.EnumTypedef result;
// Construct using ucar.nc2.stream.NcStreamProto.EnumTypedef.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.EnumTypedef();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.EnumTypedef internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.EnumTypedef();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.EnumTypedef.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.EnumTypedef.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.EnumTypedef buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.map_ != java.util.Collections.EMPTY_LIST) {
result.map_ =
java.util.Collections.unmodifiableList(result.map_);
}
ucar.nc2.stream.NcStreamProto.EnumTypedef returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.EnumTypedef) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.EnumTypedef)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.EnumTypedef other) {
if (other == ucar.nc2.stream.NcStreamProto.EnumTypedef.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (!other.map_.isEmpty()) {
if (result.map_.isEmpty()) {
result.map_ = new java.util.ArrayList();
}
result.map_.addAll(other.map_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 18: {
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder subBuilder = ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addMap(subBuilder.buildPartial());
break;
}
}
}
}
// required string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// repeated .ncstream.EnumTypedef.EnumType map = 2;
public java.util.List getMapList() {
return java.util.Collections.unmodifiableList(result.map_);
}
public int getMapCount() {
return result.getMapCount();
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType getMap(int index) {
return result.getMap(index);
}
public Builder setMap(int index, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType value) {
if (value == null) {
throw new NullPointerException();
}
result.map_.set(index, value);
return this;
}
public Builder setMap(int index, ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder builderForValue) {
result.map_.set(index, builderForValue.build());
return this;
}
public Builder addMap(ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType value) {
if (value == null) {
throw new NullPointerException();
}
if (result.map_.isEmpty()) {
result.map_ = new java.util.ArrayList();
}
result.map_.add(value);
return this;
}
public Builder addMap(ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder builderForValue) {
if (result.map_.isEmpty()) {
result.map_ = new java.util.ArrayList();
}
result.map_.add(builderForValue.build());
return this;
}
public Builder addAllMap(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType> values) {
if (result.map_.isEmpty()) {
result.map_ = new java.util.ArrayList();
}
super.addAll(values, result.map_);
return this;
}
public Builder clearMap() {
result.map_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.EnumTypedef)
}
static {
defaultInstance = new EnumTypedef(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.EnumTypedef)
}
public static final class Group extends
com.google.protobuf.GeneratedMessage {
// Use Group.newBuilder() to construct.
private Group() {
initFields();
}
private Group(boolean noInit) {}
private static final Group defaultInstance;
public static Group getDefaultInstance() {
return defaultInstance;
}
public Group getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Group_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Group_fieldAccessorTable;
}
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// repeated .ncstream.Dimension dims = 2;
public static final int DIMS_FIELD_NUMBER = 2;
private java.util.List dims_ =
java.util.Collections.emptyList();
public java.util.List getDimsList() {
return dims_;
}
public int getDimsCount() { return dims_.size(); }
public ucar.nc2.stream.NcStreamProto.Dimension getDims(int index) {
return dims_.get(index);
}
// repeated .ncstream.Variable vars = 3;
public static final int VARS_FIELD_NUMBER = 3;
private java.util.List vars_ =
java.util.Collections.emptyList();
public java.util.List getVarsList() {
return vars_;
}
public int getVarsCount() { return vars_.size(); }
public ucar.nc2.stream.NcStreamProto.Variable getVars(int index) {
return vars_.get(index);
}
// repeated .ncstream.Structure structs = 4;
public static final int STRUCTS_FIELD_NUMBER = 4;
private java.util.List structs_ =
java.util.Collections.emptyList();
public java.util.List getStructsList() {
return structs_;
}
public int getStructsCount() { return structs_.size(); }
public ucar.nc2.stream.NcStreamProto.Structure getStructs(int index) {
return structs_.get(index);
}
// repeated .ncstream.Attribute atts = 5;
public static final int ATTS_FIELD_NUMBER = 5;
private java.util.List atts_ =
java.util.Collections.emptyList();
public java.util.List getAttsList() {
return atts_;
}
public int getAttsCount() { return atts_.size(); }
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
return atts_.get(index);
}
// repeated .ncstream.Group groups = 6;
public static final int GROUPS_FIELD_NUMBER = 6;
private java.util.List groups_ =
java.util.Collections.emptyList();
public java.util.List getGroupsList() {
return groups_;
}
public int getGroupsCount() { return groups_.size(); }
public ucar.nc2.stream.NcStreamProto.Group getGroups(int index) {
return groups_.get(index);
}
// repeated .ncstream.EnumTypedef enumTypes = 7;
public static final int ENUMTYPES_FIELD_NUMBER = 7;
private java.util.List enumTypes_ =
java.util.Collections.emptyList();
public java.util.List getEnumTypesList() {
return enumTypes_;
}
public int getEnumTypesCount() { return enumTypes_.size(); }
public ucar.nc2.stream.NcStreamProto.EnumTypedef getEnumTypes(int index) {
return enumTypes_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
if (!hasName) return false;
for (ucar.nc2.stream.NcStreamProto.Variable element : getVarsList()) {
if (!element.isInitialized()) return false;
}
for (ucar.nc2.stream.NcStreamProto.Structure element : getStructsList()) {
if (!element.isInitialized()) return false;
}
for (ucar.nc2.stream.NcStreamProto.Attribute element : getAttsList()) {
if (!element.isInitialized()) return false;
}
for (ucar.nc2.stream.NcStreamProto.Group element : getGroupsList()) {
if (!element.isInitialized()) return false;
}
for (ucar.nc2.stream.NcStreamProto.EnumTypedef element : getEnumTypesList()) {
if (!element.isInitialized()) return false;
}
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasName()) {
output.writeString(1, getName());
}
for (ucar.nc2.stream.NcStreamProto.Dimension element : getDimsList()) {
output.writeMessage(2, element);
}
for (ucar.nc2.stream.NcStreamProto.Variable element : getVarsList()) {
output.writeMessage(3, element);
}
for (ucar.nc2.stream.NcStreamProto.Structure element : getStructsList()) {
output.writeMessage(4, element);
}
for (ucar.nc2.stream.NcStreamProto.Attribute element : getAttsList()) {
output.writeMessage(5, element);
}
for (ucar.nc2.stream.NcStreamProto.Group element : getGroupsList()) {
output.writeMessage(6, element);
}
for (ucar.nc2.stream.NcStreamProto.EnumTypedef element : getEnumTypesList()) {
output.writeMessage(7, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
for (ucar.nc2.stream.NcStreamProto.Dimension element : getDimsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, element);
}
for (ucar.nc2.stream.NcStreamProto.Variable element : getVarsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, element);
}
for (ucar.nc2.stream.NcStreamProto.Structure element : getStructsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, element);
}
for (ucar.nc2.stream.NcStreamProto.Attribute element : getAttsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, element);
}
for (ucar.nc2.stream.NcStreamProto.Group element : getGroupsList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, element);
}
for (ucar.nc2.stream.NcStreamProto.EnumTypedef element : getEnumTypesList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Group parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Group parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Group parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Group prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.Group result;
// Construct using ucar.nc2.stream.NcStreamProto.Group.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.Group();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.Group internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.Group();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.Group.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.Group getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.Group build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.Group buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.Group buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.dims_ != java.util.Collections.EMPTY_LIST) {
result.dims_ =
java.util.Collections.unmodifiableList(result.dims_);
}
if (result.vars_ != java.util.Collections.EMPTY_LIST) {
result.vars_ =
java.util.Collections.unmodifiableList(result.vars_);
}
if (result.structs_ != java.util.Collections.EMPTY_LIST) {
result.structs_ =
java.util.Collections.unmodifiableList(result.structs_);
}
if (result.atts_ != java.util.Collections.EMPTY_LIST) {
result.atts_ =
java.util.Collections.unmodifiableList(result.atts_);
}
if (result.groups_ != java.util.Collections.EMPTY_LIST) {
result.groups_ =
java.util.Collections.unmodifiableList(result.groups_);
}
if (result.enumTypes_ != java.util.Collections.EMPTY_LIST) {
result.enumTypes_ =
java.util.Collections.unmodifiableList(result.enumTypes_);
}
ucar.nc2.stream.NcStreamProto.Group returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Group) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Group)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Group other) {
if (other == ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (!other.dims_.isEmpty()) {
if (result.dims_.isEmpty()) {
result.dims_ = new java.util.ArrayList();
}
result.dims_.addAll(other.dims_);
}
if (!other.vars_.isEmpty()) {
if (result.vars_.isEmpty()) {
result.vars_ = new java.util.ArrayList();
}
result.vars_.addAll(other.vars_);
}
if (!other.structs_.isEmpty()) {
if (result.structs_.isEmpty()) {
result.structs_ = new java.util.ArrayList();
}
result.structs_.addAll(other.structs_);
}
if (!other.atts_.isEmpty()) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.addAll(other.atts_);
}
if (!other.groups_.isEmpty()) {
if (result.groups_.isEmpty()) {
result.groups_ = new java.util.ArrayList();
}
result.groups_.addAll(other.groups_);
}
if (!other.enumTypes_.isEmpty()) {
if (result.enumTypes_.isEmpty()) {
result.enumTypes_ = new java.util.ArrayList();
}
result.enumTypes_.addAll(other.enumTypes_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 18: {
ucar.nc2.stream.NcStreamProto.Dimension.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Dimension.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addDims(subBuilder.buildPartial());
break;
}
case 26: {
ucar.nc2.stream.NcStreamProto.Variable.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Variable.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addVars(subBuilder.buildPartial());
break;
}
case 34: {
ucar.nc2.stream.NcStreamProto.Structure.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Structure.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addStructs(subBuilder.buildPartial());
break;
}
case 42: {
ucar.nc2.stream.NcStreamProto.Attribute.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Attribute.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addAtts(subBuilder.buildPartial());
break;
}
case 50: {
ucar.nc2.stream.NcStreamProto.Group.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Group.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addGroups(subBuilder.buildPartial());
break;
}
case 58: {
ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder subBuilder = ucar.nc2.stream.NcStreamProto.EnumTypedef.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addEnumTypes(subBuilder.buildPartial());
break;
}
}
}
}
// required string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// repeated .ncstream.Dimension dims = 2;
public java.util.List getDimsList() {
return java.util.Collections.unmodifiableList(result.dims_);
}
public int getDimsCount() {
return result.getDimsCount();
}
public ucar.nc2.stream.NcStreamProto.Dimension getDims(int index) {
return result.getDims(index);
}
public Builder setDims(int index, ucar.nc2.stream.NcStreamProto.Dimension value) {
if (value == null) {
throw new NullPointerException();
}
result.dims_.set(index, value);
return this;
}
public Builder setDims(int index, ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
result.dims_.set(index, builderForValue.build());
return this;
}
public Builder addDims(ucar.nc2.stream.NcStreamProto.Dimension value) {
if (value == null) {
throw new NullPointerException();
}
if (result.dims_.isEmpty()) {
result.dims_ = new java.util.ArrayList();
}
result.dims_.add(value);
return this;
}
public Builder addDims(ucar.nc2.stream.NcStreamProto.Dimension.Builder builderForValue) {
if (result.dims_.isEmpty()) {
result.dims_ = new java.util.ArrayList();
}
result.dims_.add(builderForValue.build());
return this;
}
public Builder addAllDims(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Dimension> values) {
if (result.dims_.isEmpty()) {
result.dims_ = new java.util.ArrayList();
}
super.addAll(values, result.dims_);
return this;
}
public Builder clearDims() {
result.dims_ = java.util.Collections.emptyList();
return this;
}
// repeated .ncstream.Variable vars = 3;
public java.util.List getVarsList() {
return java.util.Collections.unmodifiableList(result.vars_);
}
public int getVarsCount() {
return result.getVarsCount();
}
public ucar.nc2.stream.NcStreamProto.Variable getVars(int index) {
return result.getVars(index);
}
public Builder setVars(int index, ucar.nc2.stream.NcStreamProto.Variable value) {
if (value == null) {
throw new NullPointerException();
}
result.vars_.set(index, value);
return this;
}
public Builder setVars(int index, ucar.nc2.stream.NcStreamProto.Variable.Builder builderForValue) {
result.vars_.set(index, builderForValue.build());
return this;
}
public Builder addVars(ucar.nc2.stream.NcStreamProto.Variable value) {
if (value == null) {
throw new NullPointerException();
}
if (result.vars_.isEmpty()) {
result.vars_ = new java.util.ArrayList();
}
result.vars_.add(value);
return this;
}
public Builder addVars(ucar.nc2.stream.NcStreamProto.Variable.Builder builderForValue) {
if (result.vars_.isEmpty()) {
result.vars_ = new java.util.ArrayList();
}
result.vars_.add(builderForValue.build());
return this;
}
public Builder addAllVars(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Variable> values) {
if (result.vars_.isEmpty()) {
result.vars_ = new java.util.ArrayList();
}
super.addAll(values, result.vars_);
return this;
}
public Builder clearVars() {
result.vars_ = java.util.Collections.emptyList();
return this;
}
// repeated .ncstream.Structure structs = 4;
public java.util.List getStructsList() {
return java.util.Collections.unmodifiableList(result.structs_);
}
public int getStructsCount() {
return result.getStructsCount();
}
public ucar.nc2.stream.NcStreamProto.Structure getStructs(int index) {
return result.getStructs(index);
}
public Builder setStructs(int index, ucar.nc2.stream.NcStreamProto.Structure value) {
if (value == null) {
throw new NullPointerException();
}
result.structs_.set(index, value);
return this;
}
public Builder setStructs(int index, ucar.nc2.stream.NcStreamProto.Structure.Builder builderForValue) {
result.structs_.set(index, builderForValue.build());
return this;
}
public Builder addStructs(ucar.nc2.stream.NcStreamProto.Structure value) {
if (value == null) {
throw new NullPointerException();
}
if (result.structs_.isEmpty()) {
result.structs_ = new java.util.ArrayList();
}
result.structs_.add(value);
return this;
}
public Builder addStructs(ucar.nc2.stream.NcStreamProto.Structure.Builder builderForValue) {
if (result.structs_.isEmpty()) {
result.structs_ = new java.util.ArrayList();
}
result.structs_.add(builderForValue.build());
return this;
}
public Builder addAllStructs(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Structure> values) {
if (result.structs_.isEmpty()) {
result.structs_ = new java.util.ArrayList();
}
super.addAll(values, result.structs_);
return this;
}
public Builder clearStructs() {
result.structs_ = java.util.Collections.emptyList();
return this;
}
// repeated .ncstream.Attribute atts = 5;
public java.util.List getAttsList() {
return java.util.Collections.unmodifiableList(result.atts_);
}
public int getAttsCount() {
return result.getAttsCount();
}
public ucar.nc2.stream.NcStreamProto.Attribute getAtts(int index) {
return result.getAtts(index);
}
public Builder setAtts(int index, ucar.nc2.stream.NcStreamProto.Attribute value) {
if (value == null) {
throw new NullPointerException();
}
result.atts_.set(index, value);
return this;
}
public Builder setAtts(int index, ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
result.atts_.set(index, builderForValue.build());
return this;
}
public Builder addAtts(ucar.nc2.stream.NcStreamProto.Attribute value) {
if (value == null) {
throw new NullPointerException();
}
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.add(value);
return this;
}
public Builder addAtts(ucar.nc2.stream.NcStreamProto.Attribute.Builder builderForValue) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
result.atts_.add(builderForValue.build());
return this;
}
public Builder addAllAtts(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Attribute> values) {
if (result.atts_.isEmpty()) {
result.atts_ = new java.util.ArrayList();
}
super.addAll(values, result.atts_);
return this;
}
public Builder clearAtts() {
result.atts_ = java.util.Collections.emptyList();
return this;
}
// repeated .ncstream.Group groups = 6;
public java.util.List getGroupsList() {
return java.util.Collections.unmodifiableList(result.groups_);
}
public int getGroupsCount() {
return result.getGroupsCount();
}
public ucar.nc2.stream.NcStreamProto.Group getGroups(int index) {
return result.getGroups(index);
}
public Builder setGroups(int index, ucar.nc2.stream.NcStreamProto.Group value) {
if (value == null) {
throw new NullPointerException();
}
result.groups_.set(index, value);
return this;
}
public Builder setGroups(int index, ucar.nc2.stream.NcStreamProto.Group.Builder builderForValue) {
result.groups_.set(index, builderForValue.build());
return this;
}
public Builder addGroups(ucar.nc2.stream.NcStreamProto.Group value) {
if (value == null) {
throw new NullPointerException();
}
if (result.groups_.isEmpty()) {
result.groups_ = new java.util.ArrayList();
}
result.groups_.add(value);
return this;
}
public Builder addGroups(ucar.nc2.stream.NcStreamProto.Group.Builder builderForValue) {
if (result.groups_.isEmpty()) {
result.groups_ = new java.util.ArrayList();
}
result.groups_.add(builderForValue.build());
return this;
}
public Builder addAllGroups(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Group> values) {
if (result.groups_.isEmpty()) {
result.groups_ = new java.util.ArrayList();
}
super.addAll(values, result.groups_);
return this;
}
public Builder clearGroups() {
result.groups_ = java.util.Collections.emptyList();
return this;
}
// repeated .ncstream.EnumTypedef enumTypes = 7;
public java.util.List getEnumTypesList() {
return java.util.Collections.unmodifiableList(result.enumTypes_);
}
public int getEnumTypesCount() {
return result.getEnumTypesCount();
}
public ucar.nc2.stream.NcStreamProto.EnumTypedef getEnumTypes(int index) {
return result.getEnumTypes(index);
}
public Builder setEnumTypes(int index, ucar.nc2.stream.NcStreamProto.EnumTypedef value) {
if (value == null) {
throw new NullPointerException();
}
result.enumTypes_.set(index, value);
return this;
}
public Builder setEnumTypes(int index, ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder builderForValue) {
result.enumTypes_.set(index, builderForValue.build());
return this;
}
public Builder addEnumTypes(ucar.nc2.stream.NcStreamProto.EnumTypedef value) {
if (value == null) {
throw new NullPointerException();
}
if (result.enumTypes_.isEmpty()) {
result.enumTypes_ = new java.util.ArrayList();
}
result.enumTypes_.add(value);
return this;
}
public Builder addEnumTypes(ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder builderForValue) {
if (result.enumTypes_.isEmpty()) {
result.enumTypes_ = new java.util.ArrayList();
}
result.enumTypes_.add(builderForValue.build());
return this;
}
public Builder addAllEnumTypes(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.EnumTypedef> values) {
if (result.enumTypes_.isEmpty()) {
result.enumTypes_ = new java.util.ArrayList();
}
super.addAll(values, result.enumTypes_);
return this;
}
public Builder clearEnumTypes() {
result.enumTypes_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Group)
}
static {
defaultInstance = new Group(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Group)
}
public static final class Header extends
com.google.protobuf.GeneratedMessage {
// Use Header.newBuilder() to construct.
private Header() {
initFields();
}
private Header(boolean noInit) {}
private static final Header defaultInstance;
public static Header getDefaultInstance() {
return defaultInstance;
}
public Header getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Header_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Header_fieldAccessorTable;
}
// optional string location = 1;
public static final int LOCATION_FIELD_NUMBER = 1;
private boolean hasLocation;
private java.lang.String location_ = "";
public boolean hasLocation() { return hasLocation; }
public java.lang.String getLocation() { return location_; }
// optional string title = 2;
public static final int TITLE_FIELD_NUMBER = 2;
private boolean hasTitle;
private java.lang.String title_ = "";
public boolean hasTitle() { return hasTitle; }
public java.lang.String getTitle() { return title_; }
// optional string id = 3;
public static final int ID_FIELD_NUMBER = 3;
private boolean hasId;
private java.lang.String id_ = "";
public boolean hasId() { return hasId; }
public java.lang.String getId() { return id_; }
// required .ncstream.Group root = 4;
public static final int ROOT_FIELD_NUMBER = 4;
private boolean hasRoot;
private ucar.nc2.stream.NcStreamProto.Group root_;
public boolean hasRoot() { return hasRoot; }
public ucar.nc2.stream.NcStreamProto.Group getRoot() { return root_; }
// optional uint32 version = 5 [default = 0];
public static final int VERSION_FIELD_NUMBER = 5;
private boolean hasVersion;
private int version_ = 0;
public boolean hasVersion() { return hasVersion; }
public int getVersion() { return version_; }
private void initFields() {
root_ = ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance();
}
public final boolean isInitialized() {
if (!hasRoot) return false;
if (!getRoot().isInitialized()) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasLocation()) {
output.writeString(1, getLocation());
}
if (hasTitle()) {
output.writeString(2, getTitle());
}
if (hasId()) {
output.writeString(3, getId());
}
if (hasRoot()) {
output.writeMessage(4, getRoot());
}
if (hasVersion()) {
output.writeUInt32(5, getVersion());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasLocation()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getLocation());
}
if (hasTitle()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getTitle());
}
if (hasId()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(3, getId());
}
if (hasRoot()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getRoot());
}
if (hasVersion()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, getVersion());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Header parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Header parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Header parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Header prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.Header result;
// Construct using ucar.nc2.stream.NcStreamProto.Header.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.Header();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.Header internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.Header();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.Header.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.Header getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Header.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.Header build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.Header buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.Header buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
ucar.nc2.stream.NcStreamProto.Header returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Header) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Header)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Header other) {
if (other == ucar.nc2.stream.NcStreamProto.Header.getDefaultInstance()) return this;
if (other.hasLocation()) {
setLocation(other.getLocation());
}
if (other.hasTitle()) {
setTitle(other.getTitle());
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasRoot()) {
mergeRoot(other.getRoot());
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setLocation(input.readString());
break;
}
case 18: {
setTitle(input.readString());
break;
}
case 26: {
setId(input.readString());
break;
}
case 34: {
ucar.nc2.stream.NcStreamProto.Group.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Group.newBuilder();
if (hasRoot()) {
subBuilder.mergeFrom(getRoot());
}
input.readMessage(subBuilder, extensionRegistry);
setRoot(subBuilder.buildPartial());
break;
}
case 40: {
setVersion(input.readUInt32());
break;
}
}
}
}
// optional string location = 1;
public boolean hasLocation() {
return result.hasLocation();
}
public java.lang.String getLocation() {
return result.getLocation();
}
public Builder setLocation(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasLocation = true;
result.location_ = value;
return this;
}
public Builder clearLocation() {
result.hasLocation = false;
result.location_ = getDefaultInstance().getLocation();
return this;
}
// optional string title = 2;
public boolean hasTitle() {
return result.hasTitle();
}
public java.lang.String getTitle() {
return result.getTitle();
}
public Builder setTitle(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasTitle = true;
result.title_ = value;
return this;
}
public Builder clearTitle() {
result.hasTitle = false;
result.title_ = getDefaultInstance().getTitle();
return this;
}
// optional string id = 3;
public boolean hasId() {
return result.hasId();
}
public java.lang.String getId() {
return result.getId();
}
public Builder setId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasId = true;
result.id_ = value;
return this;
}
public Builder clearId() {
result.hasId = false;
result.id_ = getDefaultInstance().getId();
return this;
}
// required .ncstream.Group root = 4;
public boolean hasRoot() {
return result.hasRoot();
}
public ucar.nc2.stream.NcStreamProto.Group getRoot() {
return result.getRoot();
}
public Builder setRoot(ucar.nc2.stream.NcStreamProto.Group value) {
if (value == null) {
throw new NullPointerException();
}
result.hasRoot = true;
result.root_ = value;
return this;
}
public Builder setRoot(ucar.nc2.stream.NcStreamProto.Group.Builder builderForValue) {
result.hasRoot = true;
result.root_ = builderForValue.build();
return this;
}
public Builder mergeRoot(ucar.nc2.stream.NcStreamProto.Group value) {
if (result.hasRoot() &&
result.root_ != ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance()) {
result.root_ =
ucar.nc2.stream.NcStreamProto.Group.newBuilder(result.root_).mergeFrom(value).buildPartial();
} else {
result.root_ = value;
}
result.hasRoot = true;
return this;
}
public Builder clearRoot() {
result.hasRoot = false;
result.root_ = ucar.nc2.stream.NcStreamProto.Group.getDefaultInstance();
return this;
}
// optional uint32 version = 5 [default = 0];
public boolean hasVersion() {
return result.hasVersion();
}
public int getVersion() {
return result.getVersion();
}
public Builder setVersion(int value) {
result.hasVersion = true;
result.version_ = value;
return this;
}
public Builder clearVersion() {
result.hasVersion = false;
result.version_ = 0;
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Header)
}
static {
defaultInstance = new Header(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Header)
}
public static final class Data extends
com.google.protobuf.GeneratedMessage {
// Use Data.newBuilder() to construct.
private Data() {
initFields();
}
private Data(boolean noInit) {}
private static final Data defaultInstance;
public static Data getDefaultInstance() {
return defaultInstance;
}
public Data getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Data_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Data_fieldAccessorTable;
}
// required string varName = 1;
public static final int VARNAME_FIELD_NUMBER = 1;
private boolean hasVarName;
private java.lang.String varName_ = "";
public boolean hasVarName() { return hasVarName; }
public java.lang.String getVarName() { return varName_; }
// required .ncstream.DataType dataType = 2;
public static final int DATATYPE_FIELD_NUMBER = 2;
private boolean hasDataType;
private ucar.nc2.stream.NcStreamProto.DataType dataType_;
public boolean hasDataType() { return hasDataType; }
public ucar.nc2.stream.NcStreamProto.DataType getDataType() { return dataType_; }
// required .ncstream.Section section = 3;
public static final int SECTION_FIELD_NUMBER = 3;
private boolean hasSection;
private ucar.nc2.stream.NcStreamProto.Section section_;
public boolean hasSection() { return hasSection; }
public ucar.nc2.stream.NcStreamProto.Section getSection() { return section_; }
// optional bool bigend = 4 [default = true];
public static final int BIGEND_FIELD_NUMBER = 4;
private boolean hasBigend;
private boolean bigend_ = true;
public boolean hasBigend() { return hasBigend; }
public boolean getBigend() { return bigend_; }
// optional uint32 version = 5 [default = 0];
public static final int VERSION_FIELD_NUMBER = 5;
private boolean hasVersion;
private int version_ = 0;
public boolean hasVersion() { return hasVersion; }
public int getVersion() { return version_; }
// optional .ncstream.Compress compress = 6 [default = NONE];
public static final int COMPRESS_FIELD_NUMBER = 6;
private boolean hasCompress;
private ucar.nc2.stream.NcStreamProto.Compress compress_;
public boolean hasCompress() { return hasCompress; }
public ucar.nc2.stream.NcStreamProto.Compress getCompress() { return compress_; }
// optional fixed32 crc32 = 7;
public static final int CRC32_FIELD_NUMBER = 7;
private boolean hasCrc32;
private int crc32_ = 0;
public boolean hasCrc32() { return hasCrc32; }
public int getCrc32() { return crc32_; }
private void initFields() {
dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
section_ = ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance();
compress_ = ucar.nc2.stream.NcStreamProto.Compress.NONE;
}
public final boolean isInitialized() {
if (!hasVarName) return false;
if (!hasDataType) return false;
if (!hasSection) return false;
if (!getSection().isInitialized()) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasVarName()) {
output.writeString(1, getVarName());
}
if (hasDataType()) {
output.writeEnum(2, getDataType().getNumber());
}
if (hasSection()) {
output.writeMessage(3, getSection());
}
if (hasBigend()) {
output.writeBool(4, getBigend());
}
if (hasVersion()) {
output.writeUInt32(5, getVersion());
}
if (hasCompress()) {
output.writeEnum(6, getCompress().getNumber());
}
if (hasCrc32()) {
output.writeFixed32(7, getCrc32());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasVarName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getVarName());
}
if (hasDataType()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, getDataType().getNumber());
}
if (hasSection()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getSection());
}
if (hasBigend()) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, getBigend());
}
if (hasVersion()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, getVersion());
}
if (hasCompress()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, getCompress().getNumber());
}
if (hasCrc32()) {
size += com.google.protobuf.CodedOutputStream
.computeFixed32Size(7, getCrc32());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Data parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Data parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Data parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Data prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.Data result;
// Construct using ucar.nc2.stream.NcStreamProto.Data.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.Data();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.Data internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.Data();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.Data.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.Data getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Data.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.Data build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.Data buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.Data buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
ucar.nc2.stream.NcStreamProto.Data returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Data) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Data)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Data other) {
if (other == ucar.nc2.stream.NcStreamProto.Data.getDefaultInstance()) return this;
if (other.hasVarName()) {
setVarName(other.getVarName());
}
if (other.hasDataType()) {
setDataType(other.getDataType());
}
if (other.hasSection()) {
mergeSection(other.getSection());
}
if (other.hasBigend()) {
setBigend(other.getBigend());
}
if (other.hasVersion()) {
setVersion(other.getVersion());
}
if (other.hasCompress()) {
setCompress(other.getCompress());
}
if (other.hasCrc32()) {
setCrc32(other.getCrc32());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setVarName(input.readString());
break;
}
case 16: {
int rawValue = input.readEnum();
ucar.nc2.stream.NcStreamProto.DataType value = ucar.nc2.stream.NcStreamProto.DataType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
setDataType(value);
}
break;
}
case 26: {
ucar.nc2.stream.NcStreamProto.Section.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Section.newBuilder();
if (hasSection()) {
subBuilder.mergeFrom(getSection());
}
input.readMessage(subBuilder, extensionRegistry);
setSection(subBuilder.buildPartial());
break;
}
case 32: {
setBigend(input.readBool());
break;
}
case 40: {
setVersion(input.readUInt32());
break;
}
case 48: {
int rawValue = input.readEnum();
ucar.nc2.stream.NcStreamProto.Compress value = ucar.nc2.stream.NcStreamProto.Compress.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(6, rawValue);
} else {
setCompress(value);
}
break;
}
case 61: {
setCrc32(input.readFixed32());
break;
}
}
}
}
// required string varName = 1;
public boolean hasVarName() {
return result.hasVarName();
}
public java.lang.String getVarName() {
return result.getVarName();
}
public Builder setVarName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasVarName = true;
result.varName_ = value;
return this;
}
public Builder clearVarName() {
result.hasVarName = false;
result.varName_ = getDefaultInstance().getVarName();
return this;
}
// required .ncstream.DataType dataType = 2;
public boolean hasDataType() {
return result.hasDataType();
}
public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
return result.getDataType();
}
public Builder setDataType(ucar.nc2.stream.NcStreamProto.DataType value) {
if (value == null) {
throw new NullPointerException();
}
result.hasDataType = true;
result.dataType_ = value;
return this;
}
public Builder clearDataType() {
result.hasDataType = false;
result.dataType_ = ucar.nc2.stream.NcStreamProto.DataType.CHAR;
return this;
}
// required .ncstream.Section section = 3;
public boolean hasSection() {
return result.hasSection();
}
public ucar.nc2.stream.NcStreamProto.Section getSection() {
return result.getSection();
}
public Builder setSection(ucar.nc2.stream.NcStreamProto.Section value) {
if (value == null) {
throw new NullPointerException();
}
result.hasSection = true;
result.section_ = value;
return this;
}
public Builder setSection(ucar.nc2.stream.NcStreamProto.Section.Builder builderForValue) {
result.hasSection = true;
result.section_ = builderForValue.build();
return this;
}
public Builder mergeSection(ucar.nc2.stream.NcStreamProto.Section value) {
if (result.hasSection() &&
result.section_ != ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance()) {
result.section_ =
ucar.nc2.stream.NcStreamProto.Section.newBuilder(result.section_).mergeFrom(value).buildPartial();
} else {
result.section_ = value;
}
result.hasSection = true;
return this;
}
public Builder clearSection() {
result.hasSection = false;
result.section_ = ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance();
return this;
}
// optional bool bigend = 4 [default = true];
public boolean hasBigend() {
return result.hasBigend();
}
public boolean getBigend() {
return result.getBigend();
}
public Builder setBigend(boolean value) {
result.hasBigend = true;
result.bigend_ = value;
return this;
}
public Builder clearBigend() {
result.hasBigend = false;
result.bigend_ = true;
return this;
}
// optional uint32 version = 5 [default = 0];
public boolean hasVersion() {
return result.hasVersion();
}
public int getVersion() {
return result.getVersion();
}
public Builder setVersion(int value) {
result.hasVersion = true;
result.version_ = value;
return this;
}
public Builder clearVersion() {
result.hasVersion = false;
result.version_ = 0;
return this;
}
// optional .ncstream.Compress compress = 6 [default = NONE];
public boolean hasCompress() {
return result.hasCompress();
}
public ucar.nc2.stream.NcStreamProto.Compress getCompress() {
return result.getCompress();
}
public Builder setCompress(ucar.nc2.stream.NcStreamProto.Compress value) {
if (value == null) {
throw new NullPointerException();
}
result.hasCompress = true;
result.compress_ = value;
return this;
}
public Builder clearCompress() {
result.hasCompress = false;
result.compress_ = ucar.nc2.stream.NcStreamProto.Compress.NONE;
return this;
}
// optional fixed32 crc32 = 7;
public boolean hasCrc32() {
return result.hasCrc32();
}
public int getCrc32() {
return result.getCrc32();
}
public Builder setCrc32(int value) {
result.hasCrc32 = true;
result.crc32_ = value;
return this;
}
public Builder clearCrc32() {
result.hasCrc32 = false;
result.crc32_ = 0;
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Data)
}
static {
defaultInstance = new Data(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Data)
}
public static final class Range extends
com.google.protobuf.GeneratedMessage {
// Use Range.newBuilder() to construct.
private Range() {
initFields();
}
private Range(boolean noInit) {}
private static final Range defaultInstance;
public static Range getDefaultInstance() {
return defaultInstance;
}
public Range getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Range_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Range_fieldAccessorTable;
}
// optional uint64 start = 1 [default = 0];
public static final int START_FIELD_NUMBER = 1;
private boolean hasStart;
private long start_ = 0L;
public boolean hasStart() { return hasStart; }
public long getStart() { return start_; }
// required uint64 size = 2;
public static final int SIZE_FIELD_NUMBER = 2;
private boolean hasSize;
private long size_ = 0L;
public boolean hasSize() { return hasSize; }
public long getSize() { return size_; }
// optional uint64 stride = 3 [default = 1];
public static final int STRIDE_FIELD_NUMBER = 3;
private boolean hasStride;
private long stride_ = 1L;
public boolean hasStride() { return hasStride; }
public long getStride() { return stride_; }
private void initFields() {
}
public final boolean isInitialized() {
if (!hasSize) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasStart()) {
output.writeUInt64(1, getStart());
}
if (hasSize()) {
output.writeUInt64(2, getSize());
}
if (hasStride()) {
output.writeUInt64(3, getStride());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasStart()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, getStart());
}
if (hasSize()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, getSize());
}
if (hasStride()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, getStride());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Range parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Range parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Range parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Range prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.Range result;
// Construct using ucar.nc2.stream.NcStreamProto.Range.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.Range();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.Range internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.Range();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.Range.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.Range getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Range.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.Range build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.Range buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.Range buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
ucar.nc2.stream.NcStreamProto.Range returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Range) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Range)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Range other) {
if (other == ucar.nc2.stream.NcStreamProto.Range.getDefaultInstance()) return this;
if (other.hasStart()) {
setStart(other.getStart());
}
if (other.hasSize()) {
setSize(other.getSize());
}
if (other.hasStride()) {
setStride(other.getStride());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 8: {
setStart(input.readUInt64());
break;
}
case 16: {
setSize(input.readUInt64());
break;
}
case 24: {
setStride(input.readUInt64());
break;
}
}
}
}
// optional uint64 start = 1 [default = 0];
public boolean hasStart() {
return result.hasStart();
}
public long getStart() {
return result.getStart();
}
public Builder setStart(long value) {
result.hasStart = true;
result.start_ = value;
return this;
}
public Builder clearStart() {
result.hasStart = false;
result.start_ = 0L;
return this;
}
// required uint64 size = 2;
public boolean hasSize() {
return result.hasSize();
}
public long getSize() {
return result.getSize();
}
public Builder setSize(long value) {
result.hasSize = true;
result.size_ = value;
return this;
}
public Builder clearSize() {
result.hasSize = false;
result.size_ = 0L;
return this;
}
// optional uint64 stride = 3 [default = 1];
public boolean hasStride() {
return result.hasStride();
}
public long getStride() {
return result.getStride();
}
public Builder setStride(long value) {
result.hasStride = true;
result.stride_ = value;
return this;
}
public Builder clearStride() {
result.hasStride = false;
result.stride_ = 1L;
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Range)
}
static {
defaultInstance = new Range(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Range)
}
public static final class Section extends
com.google.protobuf.GeneratedMessage {
// Use Section.newBuilder() to construct.
private Section() {
initFields();
}
private Section(boolean noInit) {}
private static final Section defaultInstance;
public static Section getDefaultInstance() {
return defaultInstance;
}
public Section getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Section_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Section_fieldAccessorTable;
}
// repeated .ncstream.Range range = 1;
public static final int RANGE_FIELD_NUMBER = 1;
private java.util.List range_ =
java.util.Collections.emptyList();
public java.util.List getRangeList() {
return range_;
}
public int getRangeCount() { return range_.size(); }
public ucar.nc2.stream.NcStreamProto.Range getRange(int index) {
return range_.get(index);
}
private void initFields() {
}
public final boolean isInitialized() {
for (ucar.nc2.stream.NcStreamProto.Range element : getRangeList()) {
if (!element.isInitialized()) return false;
}
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (ucar.nc2.stream.NcStreamProto.Range element : getRangeList()) {
output.writeMessage(1, element);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (ucar.nc2.stream.NcStreamProto.Range element : getRangeList()) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, element);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Section parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Section parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Section parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Section prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.Section result;
// Construct using ucar.nc2.stream.NcStreamProto.Section.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.Section();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.Section internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.Section();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.Section.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.Section getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.Section build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.Section buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.Section buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.range_ != java.util.Collections.EMPTY_LIST) {
result.range_ =
java.util.Collections.unmodifiableList(result.range_);
}
ucar.nc2.stream.NcStreamProto.Section returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Section) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Section)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Section other) {
if (other == ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance()) return this;
if (!other.range_.isEmpty()) {
if (result.range_.isEmpty()) {
result.range_ = new java.util.ArrayList();
}
result.range_.addAll(other.range_);
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
ucar.nc2.stream.NcStreamProto.Range.Builder subBuilder = ucar.nc2.stream.NcStreamProto.Range.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addRange(subBuilder.buildPartial());
break;
}
}
}
}
// repeated .ncstream.Range range = 1;
public java.util.List getRangeList() {
return java.util.Collections.unmodifiableList(result.range_);
}
public int getRangeCount() {
return result.getRangeCount();
}
public ucar.nc2.stream.NcStreamProto.Range getRange(int index) {
return result.getRange(index);
}
public Builder setRange(int index, ucar.nc2.stream.NcStreamProto.Range value) {
if (value == null) {
throw new NullPointerException();
}
result.range_.set(index, value);
return this;
}
public Builder setRange(int index, ucar.nc2.stream.NcStreamProto.Range.Builder builderForValue) {
result.range_.set(index, builderForValue.build());
return this;
}
public Builder addRange(ucar.nc2.stream.NcStreamProto.Range value) {
if (value == null) {
throw new NullPointerException();
}
if (result.range_.isEmpty()) {
result.range_ = new java.util.ArrayList();
}
result.range_.add(value);
return this;
}
public Builder addRange(ucar.nc2.stream.NcStreamProto.Range.Builder builderForValue) {
if (result.range_.isEmpty()) {
result.range_ = new java.util.ArrayList();
}
result.range_.add(builderForValue.build());
return this;
}
public Builder addAllRange(
java.lang.Iterable extends ucar.nc2.stream.NcStreamProto.Range> values) {
if (result.range_.isEmpty()) {
result.range_ = new java.util.ArrayList();
}
super.addAll(values, result.range_);
return this;
}
public Builder clearRange() {
result.range_ = java.util.Collections.emptyList();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Section)
}
static {
defaultInstance = new Section(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Section)
}
public static final class StructureData extends
com.google.protobuf.GeneratedMessage {
// Use StructureData.newBuilder() to construct.
private StructureData() {
initFields();
}
private StructureData(boolean noInit) {}
private static final StructureData defaultInstance;
public static StructureData getDefaultInstance() {
return defaultInstance;
}
public StructureData getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_StructureData_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_StructureData_fieldAccessorTable;
}
// repeated uint32 member = 1;
public static final int MEMBER_FIELD_NUMBER = 1;
private java.util.List member_ =
java.util.Collections.emptyList();
public java.util.List getMemberList() {
return member_;
}
public int getMemberCount() { return member_.size(); }
public int getMember(int index) {
return member_.get(index);
}
// required bytes data = 2;
public static final int DATA_FIELD_NUMBER = 2;
private boolean hasData;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
public boolean hasData() { return hasData; }
public com.google.protobuf.ByteString getData() { return data_; }
// repeated uint32 heapCount = 3;
public static final int HEAPCOUNT_FIELD_NUMBER = 3;
private java.util.List heapCount_ =
java.util.Collections.emptyList();
public java.util.List getHeapCountList() {
return heapCount_;
}
public int getHeapCountCount() { return heapCount_.size(); }
public int getHeapCount(int index) {
return heapCount_.get(index);
}
// repeated string sdata = 4;
public static final int SDATA_FIELD_NUMBER = 4;
private java.util.List sdata_ =
java.util.Collections.emptyList();
public java.util.List getSdataList() {
return sdata_;
}
public int getSdataCount() { return sdata_.size(); }
public java.lang.String getSdata(int index) {
return sdata_.get(index);
}
// optional uint64 nrows = 5 [default = 1];
public static final int NROWS_FIELD_NUMBER = 5;
private boolean hasNrows;
private long nrows_ = 1L;
public boolean hasNrows() { return hasNrows; }
public long getNrows() { return nrows_; }
private void initFields() {
}
public final boolean isInitialized() {
if (!hasData) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int element : getMemberList()) {
output.writeUInt32(1, element);
}
if (hasData()) {
output.writeBytes(2, getData());
}
for (int element : getHeapCountList()) {
output.writeUInt32(3, element);
}
for (java.lang.String element : getSdataList()) {
output.writeString(4, element);
}
if (hasNrows()) {
output.writeUInt64(5, getNrows());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int element : getMemberList()) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(element);
}
size += dataSize;
size += 1 * getMemberList().size();
}
if (hasData()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getData());
}
{
int dataSize = 0;
for (int element : getHeapCountList()) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(element);
}
size += dataSize;
size += 1 * getHeapCountList().size();
}
{
int dataSize = 0;
for (java.lang.String element : getSdataList()) {
dataSize += com.google.protobuf.CodedOutputStream
.computeStringSizeNoTag(element);
}
size += dataSize;
size += 1 * getSdataList().size();
}
if (hasNrows()) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, getNrows());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.StructureData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.StructureData prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.StructureData result;
// Construct using ucar.nc2.stream.NcStreamProto.StructureData.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.StructureData();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.StructureData internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.StructureData();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.StructureData.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.StructureData getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.StructureData.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.StructureData build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.StructureData buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.StructureData buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
if (result.member_ != java.util.Collections.EMPTY_LIST) {
result.member_ =
java.util.Collections.unmodifiableList(result.member_);
}
if (result.heapCount_ != java.util.Collections.EMPTY_LIST) {
result.heapCount_ =
java.util.Collections.unmodifiableList(result.heapCount_);
}
if (result.sdata_ != java.util.Collections.EMPTY_LIST) {
result.sdata_ =
java.util.Collections.unmodifiableList(result.sdata_);
}
ucar.nc2.stream.NcStreamProto.StructureData returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.StructureData) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.StructureData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.StructureData other) {
if (other == ucar.nc2.stream.NcStreamProto.StructureData.getDefaultInstance()) return this;
if (!other.member_.isEmpty()) {
if (result.member_.isEmpty()) {
result.member_ = new java.util.ArrayList();
}
result.member_.addAll(other.member_);
}
if (other.hasData()) {
setData(other.getData());
}
if (!other.heapCount_.isEmpty()) {
if (result.heapCount_.isEmpty()) {
result.heapCount_ = new java.util.ArrayList();
}
result.heapCount_.addAll(other.heapCount_);
}
if (!other.sdata_.isEmpty()) {
if (result.sdata_.isEmpty()) {
result.sdata_ = new java.util.ArrayList();
}
result.sdata_.addAll(other.sdata_);
}
if (other.hasNrows()) {
setNrows(other.getNrows());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 8: {
addMember(input.readUInt32());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
while (input.getBytesUntilLimit() > 0) {
addMember(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 18: {
setData(input.readBytes());
break;
}
case 24: {
addHeapCount(input.readUInt32());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
while (input.getBytesUntilLimit() > 0) {
addHeapCount(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 34: {
addSdata(input.readString());
break;
}
case 40: {
setNrows(input.readUInt64());
break;
}
}
}
}
// repeated uint32 member = 1;
public java.util.List getMemberList() {
return java.util.Collections.unmodifiableList(result.member_);
}
public int getMemberCount() {
return result.getMemberCount();
}
public int getMember(int index) {
return result.getMember(index);
}
public Builder setMember(int index, int value) {
result.member_.set(index, value);
return this;
}
public Builder addMember(int value) {
if (result.member_.isEmpty()) {
result.member_ = new java.util.ArrayList();
}
result.member_.add(value);
return this;
}
public Builder addAllMember(
java.lang.Iterable extends java.lang.Integer> values) {
if (result.member_.isEmpty()) {
result.member_ = new java.util.ArrayList();
}
super.addAll(values, result.member_);
return this;
}
public Builder clearMember() {
result.member_ = java.util.Collections.emptyList();
return this;
}
// required bytes data = 2;
public boolean hasData() {
return result.hasData();
}
public com.google.protobuf.ByteString getData() {
return result.getData();
}
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
result.hasData = true;
result.data_ = value;
return this;
}
public Builder clearData() {
result.hasData = false;
result.data_ = getDefaultInstance().getData();
return this;
}
// repeated uint32 heapCount = 3;
public java.util.List getHeapCountList() {
return java.util.Collections.unmodifiableList(result.heapCount_);
}
public int getHeapCountCount() {
return result.getHeapCountCount();
}
public int getHeapCount(int index) {
return result.getHeapCount(index);
}
public Builder setHeapCount(int index, int value) {
result.heapCount_.set(index, value);
return this;
}
public Builder addHeapCount(int value) {
if (result.heapCount_.isEmpty()) {
result.heapCount_ = new java.util.ArrayList();
}
result.heapCount_.add(value);
return this;
}
public Builder addAllHeapCount(
java.lang.Iterable extends java.lang.Integer> values) {
if (result.heapCount_.isEmpty()) {
result.heapCount_ = new java.util.ArrayList();
}
super.addAll(values, result.heapCount_);
return this;
}
public Builder clearHeapCount() {
result.heapCount_ = java.util.Collections.emptyList();
return this;
}
// repeated string sdata = 4;
public java.util.List getSdataList() {
return java.util.Collections.unmodifiableList(result.sdata_);
}
public int getSdataCount() {
return result.getSdataCount();
}
public java.lang.String getSdata(int index) {
return result.getSdata(index);
}
public Builder setSdata(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.sdata_.set(index, value);
return this;
}
public Builder addSdata(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
if (result.sdata_.isEmpty()) {
result.sdata_ = new java.util.ArrayList();
}
result.sdata_.add(value);
return this;
}
public Builder addAllSdata(
java.lang.Iterable extends java.lang.String> values) {
if (result.sdata_.isEmpty()) {
result.sdata_ = new java.util.ArrayList();
}
super.addAll(values, result.sdata_);
return this;
}
public Builder clearSdata() {
result.sdata_ = java.util.Collections.emptyList();
return this;
}
// optional uint64 nrows = 5 [default = 1];
public boolean hasNrows() {
return result.hasNrows();
}
public long getNrows() {
return result.getNrows();
}
public Builder setNrows(long value) {
result.hasNrows = true;
result.nrows_ = value;
return this;
}
public Builder clearNrows() {
result.hasNrows = false;
result.nrows_ = 1L;
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.StructureData)
}
static {
defaultInstance = new StructureData(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.StructureData)
}
public static final class Error extends
com.google.protobuf.GeneratedMessage {
// Use Error.newBuilder() to construct.
private Error() {
initFields();
}
private Error(boolean noInit) {}
private static final Error defaultInstance;
public static Error getDefaultInstance() {
return defaultInstance;
}
public Error getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Error_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.stream.NcStreamProto.internal_static_ncstream_Error_fieldAccessorTable;
}
// required string message = 1;
public static final int MESSAGE_FIELD_NUMBER = 1;
private boolean hasMessage;
private java.lang.String message_ = "";
public boolean hasMessage() { return hasMessage; }
public java.lang.String getMessage() { return message_; }
private void initFields() {
}
public final boolean isInitialized() {
if (!hasMessage) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (hasMessage()) {
output.writeString(1, getMessage());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasMessage()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getMessage());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Error parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Error parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static ucar.nc2.stream.NcStreamProto.Error parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(ucar.nc2.stream.NcStreamProto.Error prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private ucar.nc2.stream.NcStreamProto.Error result;
// Construct using ucar.nc2.stream.NcStreamProto.Error.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new ucar.nc2.stream.NcStreamProto.Error();
return builder;
}
protected ucar.nc2.stream.NcStreamProto.Error internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new ucar.nc2.stream.NcStreamProto.Error();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.stream.NcStreamProto.Error.getDescriptor();
}
public ucar.nc2.stream.NcStreamProto.Error getDefaultInstanceForType() {
return ucar.nc2.stream.NcStreamProto.Error.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public ucar.nc2.stream.NcStreamProto.Error build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private ucar.nc2.stream.NcStreamProto.Error buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public ucar.nc2.stream.NcStreamProto.Error buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
ucar.nc2.stream.NcStreamProto.Error returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.stream.NcStreamProto.Error) {
return mergeFrom((ucar.nc2.stream.NcStreamProto.Error)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.stream.NcStreamProto.Error other) {
if (other == ucar.nc2.stream.NcStreamProto.Error.getDefaultInstance()) return this;
if (other.hasMessage()) {
setMessage(other.getMessage());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setMessage(input.readString());
break;
}
}
}
}
// required string message = 1;
public boolean hasMessage() {
return result.hasMessage();
}
public java.lang.String getMessage() {
return result.getMessage();
}
public Builder setMessage(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasMessage = true;
result.message_ = value;
return this;
}
public Builder clearMessage() {
result.hasMessage = false;
result.message_ = getDefaultInstance().getMessage();
return this;
}
// @@protoc_insertion_point(builder_scope:ncstream.Error)
}
static {
defaultInstance = new Error(true);
ucar.nc2.stream.NcStreamProto.internalForceInit();
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ncstream.Error)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Attribute_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Attribute_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Dimension_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Dimension_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Variable_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Variable_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Structure_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Structure_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_EnumTypedef_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_EnumTypedef_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_EnumTypedef_EnumType_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_EnumTypedef_EnumType_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Group_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Group_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Header_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Header_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Data_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Data_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Range_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Range_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Section_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Section_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_StructureData_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_StructureData_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ncstream_Error_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ncstream_Error_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\036ucar/nc2/stream/ncStream.proto\022\010ncstre" +
"am\"\276\001\n\tAttribute\022\014\n\004name\030\001 \002(\t\022&\n\004type\030\002" +
" \002(\0162\030.ncstream.Attribute.Type\022\013\n\003len\030\003 " +
"\002(\r\022\014\n\004data\030\004 \001(\014\022\r\n\005sdata\030\005 \003(\t\"Q\n\004Type" +
"\022\n\n\006STRING\020\000\022\010\n\004BYTE\020\001\022\t\n\005SHORT\020\002\022\007\n\003INT" +
"\020\003\022\010\n\004LONG\020\004\022\t\n\005FLOAT\020\005\022\n\n\006DOUBLE\020\006\"v\n\tD" +
"imension\022\014\n\004name\030\001 \001(\t\022\016\n\006length\030\002 \001(\004\022\032" +
"\n\013isUnlimited\030\003 \001(\010:\005false\022\025\n\006isVlen\030\004 \001" +
"(\010:\005false\022\030\n\tisPrivate\030\005 \001(\010:\005false\"\320\001\n\010" +
"Variable\022\014\n\004name\030\001 \002(\t\022$\n\010dataType\030\002 \002(\016",
"2\022.ncstream.DataType\022\"\n\005shape\030\003 \003(\0132\023.nc" +
"stream.Dimension\022!\n\004atts\030\004 \003(\0132\023.ncstrea" +
"m.Attribute\022\027\n\010unsigned\030\005 \001(\010:\005false\022\014\n\004" +
"data\030\006 \001(\014\022\020\n\010enumType\030\007 \001(\t\022\020\n\010dimIndex" +
"\030\010 \003(\r\"\316\001\n\tStructure\022\014\n\004name\030\001 \002(\t\022$\n\010da" +
"taType\030\002 \002(\0162\022.ncstream.DataType\022\"\n\005shap" +
"e\030\003 \003(\0132\023.ncstream.Dimension\022!\n\004atts\030\004 \003" +
"(\0132\023.ncstream.Attribute\022 \n\004vars\030\005 \003(\0132\022." +
"ncstream.Variable\022$\n\007structs\030\006 \003(\0132\023.ncs" +
"tream.Structure\"q\n\013EnumTypedef\022\014\n\004name\030\001",
" \002(\t\022+\n\003map\030\002 \003(\0132\036.ncstream.EnumTypedef" +
".EnumType\032\'\n\010EnumType\022\014\n\004code\030\001 \002(\r\022\r\n\005v" +
"alue\030\002 \002(\t\"\356\001\n\005Group\022\014\n\004name\030\001 \002(\t\022!\n\004di" +
"ms\030\002 \003(\0132\023.ncstream.Dimension\022 \n\004vars\030\003 " +
"\003(\0132\022.ncstream.Variable\022$\n\007structs\030\004 \003(\013" +
"2\023.ncstream.Structure\022!\n\004atts\030\005 \003(\0132\023.nc" +
"stream.Attribute\022\037\n\006groups\030\006 \003(\0132\017.ncstr" +
"eam.Group\022(\n\tenumTypes\030\007 \003(\0132\025.ncstream." +
"EnumTypedef\"h\n\006Header\022\020\n\010location\030\001 \001(\t\022" +
"\r\n\005title\030\002 \001(\t\022\n\n\002id\030\003 \001(\t\022\035\n\004root\030\004 \002(\013",
"2\017.ncstream.Group\022\022\n\007version\030\005 \001(\r:\0010\"\306\001" +
"\n\004Data\022\017\n\007varName\030\001 \002(\t\022$\n\010dataType\030\002 \002(" +
"\0162\022.ncstream.DataType\022\"\n\007section\030\003 \002(\0132\021" +
".ncstream.Section\022\024\n\006bigend\030\004 \001(\010:\004true\022" +
"\022\n\007version\030\005 \001(\r:\0010\022*\n\010compress\030\006 \001(\0162\022." +
"ncstream.Compress:\004NONE\022\r\n\005crc32\030\007 \001(\007\":" +
"\n\005Range\022\020\n\005start\030\001 \001(\004:\0010\022\014\n\004size\030\002 \002(\004\022" +
"\021\n\006stride\030\003 \001(\004:\0011\")\n\007Section\022\036\n\005range\030\001" +
" \003(\0132\017.ncstream.Range\"a\n\rStructureData\022\016" +
"\n\006member\030\001 \003(\r\022\014\n\004data\030\002 \002(\014\022\021\n\theapCoun",
"t\030\003 \003(\r\022\r\n\005sdata\030\004 \003(\t\022\020\n\005nrows\030\005 \001(\004:\0011" +
"\"\030\n\005Error\022\017\n\007message\030\001 \002(\t*\251\001\n\010DataType\022" +
"\010\n\004CHAR\020\000\022\010\n\004BYTE\020\001\022\t\n\005SHORT\020\002\022\007\n\003INT\020\003\022" +
"\010\n\004LONG\020\004\022\t\n\005FLOAT\020\005\022\n\n\006DOUBLE\020\006\022\n\n\006STRI" +
"NG\020\007\022\r\n\tSTRUCTURE\020\010\022\014\n\010SEQUENCE\020\t\022\t\n\005ENU" +
"M1\020\n\022\t\n\005ENUM2\020\013\022\t\n\005ENUM4\020\014\022\n\n\006OPAQUE\020\r*!" +
"\n\010Compress\022\010\n\004NONE\020\000\022\013\n\007DEFLATE\020\001B \n\017uca" +
"r.nc2.streamB\rNcStreamProto"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_ncstream_Attribute_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_ncstream_Attribute_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Attribute_descriptor,
new java.lang.String[] { "Name", "Type", "Len", "Data", "Sdata", },
ucar.nc2.stream.NcStreamProto.Attribute.class,
ucar.nc2.stream.NcStreamProto.Attribute.Builder.class);
internal_static_ncstream_Dimension_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_ncstream_Dimension_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Dimension_descriptor,
new java.lang.String[] { "Name", "Length", "IsUnlimited", "IsVlen", "IsPrivate", },
ucar.nc2.stream.NcStreamProto.Dimension.class,
ucar.nc2.stream.NcStreamProto.Dimension.Builder.class);
internal_static_ncstream_Variable_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_ncstream_Variable_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Variable_descriptor,
new java.lang.String[] { "Name", "DataType", "Shape", "Atts", "Unsigned", "Data", "EnumType", "DimIndex", },
ucar.nc2.stream.NcStreamProto.Variable.class,
ucar.nc2.stream.NcStreamProto.Variable.Builder.class);
internal_static_ncstream_Structure_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_ncstream_Structure_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Structure_descriptor,
new java.lang.String[] { "Name", "DataType", "Shape", "Atts", "Vars", "Structs", },
ucar.nc2.stream.NcStreamProto.Structure.class,
ucar.nc2.stream.NcStreamProto.Structure.Builder.class);
internal_static_ncstream_EnumTypedef_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_ncstream_EnumTypedef_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_EnumTypedef_descriptor,
new java.lang.String[] { "Name", "Map", },
ucar.nc2.stream.NcStreamProto.EnumTypedef.class,
ucar.nc2.stream.NcStreamProto.EnumTypedef.Builder.class);
internal_static_ncstream_EnumTypedef_EnumType_descriptor =
internal_static_ncstream_EnumTypedef_descriptor.getNestedTypes().get(0);
internal_static_ncstream_EnumTypedef_EnumType_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_EnumTypedef_EnumType_descriptor,
new java.lang.String[] { "Code", "Value", },
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.class,
ucar.nc2.stream.NcStreamProto.EnumTypedef.EnumType.Builder.class);
internal_static_ncstream_Group_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_ncstream_Group_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Group_descriptor,
new java.lang.String[] { "Name", "Dims", "Vars", "Structs", "Atts", "Groups", "EnumTypes", },
ucar.nc2.stream.NcStreamProto.Group.class,
ucar.nc2.stream.NcStreamProto.Group.Builder.class);
internal_static_ncstream_Header_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_ncstream_Header_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Header_descriptor,
new java.lang.String[] { "Location", "Title", "Id", "Root", "Version", },
ucar.nc2.stream.NcStreamProto.Header.class,
ucar.nc2.stream.NcStreamProto.Header.Builder.class);
internal_static_ncstream_Data_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_ncstream_Data_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Data_descriptor,
new java.lang.String[] { "VarName", "DataType", "Section", "Bigend", "Version", "Compress", "Crc32", },
ucar.nc2.stream.NcStreamProto.Data.class,
ucar.nc2.stream.NcStreamProto.Data.Builder.class);
internal_static_ncstream_Range_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_ncstream_Range_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Range_descriptor,
new java.lang.String[] { "Start", "Size", "Stride", },
ucar.nc2.stream.NcStreamProto.Range.class,
ucar.nc2.stream.NcStreamProto.Range.Builder.class);
internal_static_ncstream_Section_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_ncstream_Section_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Section_descriptor,
new java.lang.String[] { "Range", },
ucar.nc2.stream.NcStreamProto.Section.class,
ucar.nc2.stream.NcStreamProto.Section.Builder.class);
internal_static_ncstream_StructureData_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_ncstream_StructureData_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_StructureData_descriptor,
new java.lang.String[] { "Member", "Data", "HeapCount", "Sdata", "Nrows", },
ucar.nc2.stream.NcStreamProto.StructureData.class,
ucar.nc2.stream.NcStreamProto.StructureData.Builder.class);
internal_static_ncstream_Error_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_ncstream_Error_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ncstream_Error_descriptor,
new java.lang.String[] { "Message", },
ucar.nc2.stream.NcStreamProto.Error.class,
ucar.nc2.stream.NcStreamProto.Error.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
public static void internalForceInit() {}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy