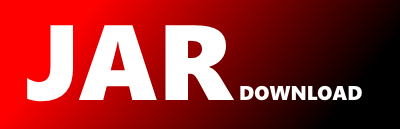
edu.uvm.ccts.arden.util.DurationUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arden-model Show documentation
Show all versions of arden-model Show documentation
The Java model used to represent Arden objects
/*
* Copyright 2015 The University of Vermont and State
* Agricultural College, Vermont Oxford Network, and The University
* of Vermont Medical Center. All rights reserved.
*
* Written by Matthew B. Storer
*
* This file is part of Arden Model.
*
* Arden Model is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Arden Model is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Arden Model. If not, see .
*/
package edu.uvm.ccts.arden.util;
import edu.uvm.ccts.arden.model.*;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* Created by mstorer on 8/13/13.
*/
public class DurationUtil {
private static final Log log = LogFactory.getLog(DurationUtil.class);
public static Duration buildDuration(ANumber n, String s) throws ParseException {
return buildDuration(n, parseUnit(s));
}
public static Duration buildDuration(ANumber n, DurationUnit unit) { // spec 8.5.1
switch (unit) {
case YEARS: return new MonthsDuration(n.multiply(12));
case MONTHS: return new MonthsDuration(n);
case WEEKS: return new SecondsDuration(n.multiply(Duration.SEC_IN_WEEK).longValue());
case DAYS: return new SecondsDuration(n.multiply(Duration.SEC_IN_DAY).longValue());
case HOURS: return new SecondsDuration(n.multiply(Duration.SEC_IN_HOUR).longValue());
case MINUTES: return new SecondsDuration(n.multiply(Duration.SEC_IN_MINUTE).longValue());
default: return new SecondsDuration(n.longValue());
}
}
public static long convertToSeconds(ANumber n, DurationUnit unit) {
return convertToSeconds(n.doubleValue(), unit);
}
public static long convertToSeconds(double d, DurationUnit unit) {
switch (unit) {
case YEARS: return (long) (Duration.SEC_IN_YEAR * d);
case MONTHS: return (long) (Duration.SEC_IN_MONTH * d);
case WEEKS: return (long) (Duration.SEC_IN_WEEK * d);
case DAYS: return (long) (Duration.SEC_IN_DAY * d);
case HOURS: return (long) (Duration.SEC_IN_HOUR * d);
case MINUTES: return (long) (Duration.SEC_IN_MINUTE * d);
default: return (long) (Duration.SECOND * d);
}
}
public static DurationUnit parseUnit(String s) throws ParseException {
s = s.toLowerCase();
if (s.equals("year") || s.equals("years")) {
return DurationUnit.YEARS;
} else if (s.equals("month") || s.equals("months")) {
return DurationUnit.MONTHS;
} else if (s.equals("week") || s.equals("weeks")) {
return DurationUnit.WEEKS;
} else if (s.equals("day") || s.equals("days")) {
return DurationUnit.DAYS;
} else if (s.equals("hour") || s.equals("hours")) {
return DurationUnit.HOURS;
} else if (s.equals("minute") || s.equals("minutes")) {
return DurationUnit.MINUTES;
} else if (s.equals("second") || s.equals("seconds")) {
return DurationUnit.SECONDS;
} else {
throw new ParseException("couldn't parse duration unit '" + s + "'", 0);
}
}
public static Duration mean(List list) {
if (list == null || list.isEmpty()) return null;
long total = 0L;
for (Duration d : list) {
total += d.getSeconds();
}
SecondsDuration mean = new SecondsDuration(total / list.size());
mean.setPrimaryTime(ListUtil.getPrimaryTime(list)); // maintain primary time
return mean;
}
public static Duration median(List list) {
if (list == null || list.isEmpty()) return null;
list = shallowCopy(list);
Collections.sort(list);
if (list.size() % 2 == 0) { // even
int index = list.size() / 2 - 1;
Duration d = list.get(index).add(list.get(index + 1));
Duration median = new SecondsDuration(d.getSeconds() / 2);
median.setPrimaryTime(ListUtil.getPrimaryTime(list.subList(index, index + 1)));
return median;
} else {
int index = ((list.size() - 1) / 2);
return list.get(index);
}
}
public static Duration sum(List list) {
if (list == null) return null;
Duration sum = new SecondsDuration(0);
for (Duration d : list) {
sum = sum.add(d);
}
sum.setPrimaryTime(ListUtil.getPrimaryTime(list));
return sum;
}
/**
* @deprecated use {@link AList#minimum} instead
* @param list
* @return
*/
@Deprecated
public static Duration min(List list) {
if (list == null || list.isEmpty()) return null;
Duration min = null;
for (Duration d : list) {
if (min == null) min = d;
else if (d.compareTo(min) < 0) min = d;
}
return min;
}
/**
* @deprecated use {@link AList#maximum} instead
* @param list
* @return
*/
@Deprecated
public static Duration max(List list) {
if (list == null || list.isEmpty()) return null;
Duration max = null;
for (Duration d : list) {
if (max == null) max = d;
else if (d.compareTo(max) > 0) max = d;
}
return max;
}
private static List shallowCopy(List list) {
if (list == null) return null;
List copied = new ArrayList();
copied.addAll(list);
return copied;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy