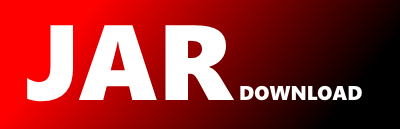
edu.uvm.ccts.arden.util.ObjectUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arden-model Show documentation
Show all versions of arden-model Show documentation
The Java model used to represent Arden objects
/*
* Copyright 2015 The University of Vermont and State
* Agricultural College, Vermont Oxford Network, and The University
* of Vermont Medical Center. All rights reserved.
*
* Written by Matthew B. Storer
*
* This file is part of Arden Model.
*
* Arden Model is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Arden Model is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Arden Model. If not, see .
*/
package edu.uvm.ccts.arden.util;
import edu.uvm.ccts.arden.model.*;
import edu.uvm.ccts.common.exceptions.UnhandledClassException;
import java.sql.Timestamp;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
/**
* Created by mstorer on 8/26/13.
*/
public class ObjectUtil {
public static ADataType and(ADataType a, ADataType b) {
ABoolean left = asBoolean(a);
ABoolean right = asBoolean(b);
if (left.isUnknown() && right.isUnknown()) {
return new ANull();
} else if (left.isUnknown()) {
return right.asBoolean() ? new ANull() : new ABoolean(false);
} else if (right.isUnknown()) {
return left.asBoolean() ? new ANull() : new ABoolean(false);
} else {
return new ABoolean(left.asBoolean() && right.asBoolean());
}
}
public static ADataType or(ADataType a, ADataType b) {
ABoolean left = asBoolean(a);
ABoolean right = asBoolean(b);
if (left.isUnknown() && right.isUnknown()) {
return new ANull();
} else if (left.isUnknown()) {
return right.asBoolean() ? new ABoolean(true) : new ANull();
} else if (right.isUnknown()) {
return left.asBoolean() ? new ABoolean(true) : new ANull();
} else {
return new ABoolean(left.asBoolean() || right.asBoolean());
}
}
@SuppressWarnings("unchecked")
public static ADataType isLessThan(ADataType a, ADataType b) {
if (isNumber(a) && isNumber(b)) {
return asNumber(a).isLessThan(asNumber(b));
} else if ((isDuration(a) && isDuration(b)) || (a.isComparable() && isLike(a, b))) {
int val = ((Comparable) a).compareTo(b);
return new ABoolean(val < 0);
} else {
return new ANull();
}
}
@SuppressWarnings("unchecked")
public static ADataType isLessThanOrEqualTo(ADataType a, ADataType b) {
if (isNumber(a) && isNumber(b)) {
return asNumber(a).isLessThanOrEqualTo(asNumber(b));
} else if ((isDuration(a) && isDuration(b)) || (a.isComparable() && isLike(a, b))) {
int val = ((Comparable) a).compareTo(b);
return new ABoolean(val <= 0);
} else {
return new ANull();
}
}
public static ADataType isEqualTo(ADataType a, ADataType b) {
return isNull(a) || isNull(b) ?
new ANull() :
isEqualToHelper(a, b);
}
public static ADataType isNotEqualTo(ADataType a, ADataType b) {
return isNull(a) || isNull(b) ?
new ANull() :
isEqualToHelper(a, b).not();
}
private static ABoolean isEqualToHelper(ADataType a, ADataType b) {
// todo : implement this properly - see spec 9.5.1
if (a == b) { // identity
return ABoolean.TRUE();
} else if (a.getClass() == b.getClass()) {
return a.hasValue(b) ? // primary times are not used in determining
ABoolean.TRUE() : // equality - spec 9.5.1
ABoolean.FALSE();
} else {
return ABoolean.FALSE();
}
}
@SuppressWarnings("unchecked")
public static ADataType isGreaterThan(ADataType a, ADataType b) {
if (isNumber(a) && isNumber(b)) {
return asNumber(a).isGreaterThan(asNumber(b));
} else if ((isDuration(a) && isDuration(b)) || (a.isComparable() && isLike(a, b))) {
int val = ((Comparable) a).compareTo(b);
return new ABoolean(val > 0);
} else {
return new ANull();
}
}
@SuppressWarnings("unchecked")
public static ADataType isGreaterThanOrEqualTo(ADataType a, ADataType b) {
if (isNumber(a) && isNumber(b)) {
return asNumber(a).isGreaterThanOrEqualTo(asNumber(b));
} else if ((isDuration(a) && isDuration(b)) || (a.isComparable() && isLike(a, b))) {
int val = ((Comparable) a).compareTo(b);
return new ABoolean(val >= 0);
} else {
return new ANull();
}
}
public static ADataType toArdenDataType(Object obj) {
if (obj == null) return new ANull();
else if (obj instanceof ADataType) return (ADataType) obj;
else if (obj instanceof String) return new AString(obj.toString());
else if (obj instanceof Boolean) return new ABoolean((Boolean) obj);
else if (obj instanceof Number) return new ANumber((Number) obj);
else if (obj instanceof java.sql.Time) return new TimeOfDay(new Date(((java.sql.Time) obj).getTime()));
else if (obj instanceof Timestamp) return new Time(new Date(((Timestamp) obj).getTime()));
else if (obj instanceof Date) return new Time((Date) obj);
else throw new UnhandledClassException("cannot convert " + obj.getClass().getCanonicalName() +
" to Arden data type");
}
public static boolean isOrdered(ADataType obj) {
return obj.isComparable();
}
public static boolean isNull(ADataType obj) {
return obj instanceof ANull;
}
public static boolean isString(ADataType obj) {
return obj instanceof AString;
}
public static AString asString(ADataType obj) {
if (obj instanceof AString) return (AString) obj;
else if (obj == null) return new AString("null");
else return new AString(obj.toString());
}
public static boolean isBoolean(ADataType obj) {
return obj instanceof ABoolean;
}
public static ABoolean asBoolean(ADataType obj) {
if (obj instanceof ABoolean) return (ABoolean) obj;
else return ABoolean.UNKNOWN();
}
public static boolean isNumber(ADataType obj) {
return obj instanceof ANumber;
}
public static ANumber asNumber(ADataType obj) {
if (obj instanceof ANumber) return (ANumber) obj;
else return null;
}
public static boolean isInteger(ADataType obj) {
if (obj instanceof ANumber) return ((ANumber) obj).isWholeNumber();
else return false;
}
public static int asInteger(ADataType obj) {
if (obj instanceof ANumber) return ((ANumber) obj).intValue();
else return 0;
}
public static boolean isTime(ADataType obj) {
return obj instanceof Time;
}
public static Time asTime(ADataType obj) {
if (obj instanceof Time) return (Time) obj;
else return null;
}
public static boolean isTimeOfDay(ADataType obj) {
return obj instanceof TimeOfDay;
}
public static TimeOfDay asTimeOfDay(ADataType obj) {
if (obj instanceof TimeOfDay) return (TimeOfDay) obj;
else return null;
}
public static boolean isDuration(ADataType obj) {
return obj instanceof Duration;
}
public static Duration asDuration(ADataType obj) {
if (obj instanceof Duration) return (Duration) obj;
else return null;
}
public static boolean isObject(ADataType obj) {
return obj instanceof AObject;
}
public static AObject asObject(ADataType obj) {
if (obj instanceof AObject) return (AObject) obj;
else return null;
}
public static boolean isObjectType(ADataType obj) {
return obj instanceof AObjectType;
}
public static AObjectType asObjectType(ADataType obj) {
if (obj instanceof AObjectType) return (AObjectType) obj;
else return null;
}
public static boolean isLike(ADataType a, ADataType b) {
return a.getClass().isInstance(b);
}
public static boolean isArgumentList(ADataType obj) {
return obj instanceof ArgumentList;
}
public static ArgumentList asArgumentList(ADataType obj) {
if (obj instanceof ArgumentList) return (ArgumentList) obj;
else return null;
}
public static boolean isList(ADataType obj) {
return obj instanceof AList;
}
public static boolean isListOfLists(ADataType obj) {
return obj instanceof AListOfLists;
}
public static AList asList(ADataType obj) {
if (obj instanceof AList) {
return (AList) obj;
} else {
AList list = new AList();
list.add(obj);
return list;
}
}
public static AListOfLists asListOfLists(ADataType obj) {
if (obj instanceof AListOfLists) {
return (AListOfLists) obj;
} else if (obj instanceof AList || obj instanceof ANull) {
AListOfLists listOfLists = new AListOfLists();
listOfLists.add(obj);
return listOfLists;
} else {
AListOfLists listOfLists = new AListOfLists(1);
listOfLists.getList(0).add(obj);
return listOfLists;
}
}
public static ListType getListType(ADataType obj) {
return asList(obj).getListType();
}
public static List asBooleanList(ADataType obj) {
List list = new ArrayList();
for (ADataType item : asList(obj)) {
list.add(asBoolean(item));
}
return list;
}
public static List asStringList(ADataType obj) {
List list = new ArrayList();
for (ADataType item : asList(obj)) {
list.add(asString(item));
}
return list;
}
public static List asNumberList(ADataType obj) {
List list = new ArrayList();
for (ADataType item : asList(obj)) {
list.add(asNumber(item));
}
return list;
}
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy