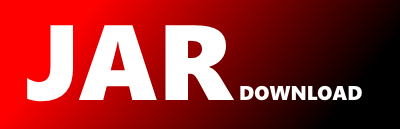
edu.uvm.ccts.arden.action.antlr.ActionVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arden-parser Show documentation
Show all versions of arden-parser Show documentation
ANTLR grammar and Java logic used to traverse the generated abstract syntax trees related to Arden-syntax Medical Logic Modules (MLMs)
// Generated from Action.g4 by ANTLR 4.1
package edu.uvm.ccts.arden.action.antlr;
import org.antlr.v4.runtime.misc.NotNull;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link ActionParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface ActionVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link ActionParser#durationExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDurationExpr(@NotNull ActionParser.DurationExprContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#arccos}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArccos(@NotNull ActionParser.ArccosContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#no}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNo(@NotNull ActionParser.NoContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#asString}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAsString(@NotNull ActionParser.AsStringContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#firstFrom}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFirstFrom(@NotNull ActionParser.FirstFromContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#dot}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDot(@NotNull ActionParser.DotContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#temporalUnit}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTemporalUnit(@NotNull ActionParser.TemporalUnitContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#minimumFrom}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMinimumFrom(@NotNull ActionParser.MinimumFromContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#indexType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIndexType(@NotNull ActionParser.IndexTypeContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#matches}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMatches(@NotNull ActionParser.MatchesContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#durationUnit}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDurationUnit(@NotNull ActionParser.DurationUnitContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#abs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAbs(@NotNull ActionParser.AbsContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isIn}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsIn(@NotNull ActionParser.IsInContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#indexOfFrom}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIndexOfFrom(@NotNull ActionParser.IndexOfFromContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#substring}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSubstring(@NotNull ActionParser.SubstringContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#forLoop}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitForLoop(@NotNull ActionParser.ForLoopContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#element}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitElement(@NotNull ActionParser.ElementContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#clone}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitClone(@NotNull ActionParser.CloneContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#occurEqual}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOccurEqual(@NotNull ActionParser.OccurEqualContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isWithinFollowing}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsWithinFollowing(@NotNull ActionParser.IsWithinFollowingContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#unaryMinus}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnaryMinus(@NotNull ActionParser.UnaryMinusContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#or}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOr(@NotNull ActionParser.OrContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#unaryList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnaryList(@NotNull ActionParser.UnaryListContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#parens}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParens(@NotNull ActionParser.ParensContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#log}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLog(@NotNull ActionParser.LogContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#atMostOrLeast}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAtMostOrLeast(@NotNull ActionParser.AtMostOrLeastContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#extract}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExtract(@NotNull ActionParser.ExtractContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#timeOfDayVal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimeOfDayVal(@NotNull ActionParser.TimeOfDayValContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#writeStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWriteStatement(@NotNull ActionParser.WriteStatementContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#not}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNot(@NotNull ActionParser.NotContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#median}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMedian(@NotNull ActionParser.MedianContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#floor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFloor(@NotNull ActionParser.FloorContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#now}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNow(@NotNull ActionParser.NowContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#truncate}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTruncate(@NotNull ActionParser.TruncateContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isLessThanOrEqual}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsLessThanOrEqual(@NotNull ActionParser.IsLessThanOrEqualContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#nullVal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNullVal(@NotNull ActionParser.NullValContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#occurBefore}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOccurBefore(@NotNull ActionParser.OccurBeforeContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#objOrIndexRule}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitObjOrIndexRule(@NotNull ActionParser.ObjOrIndexRuleContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#whereTimeIsPresent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhereTimeIsPresent(@NotNull ActionParser.WhereTimeIsPresentContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#sort}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSort(@NotNull ActionParser.SortContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#interval}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInterval(@NotNull ActionParser.IntervalContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#minimum}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMinimum(@NotNull ActionParser.MinimumContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#before}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBefore(@NotNull ActionParser.BeforeContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#merge}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMerge(@NotNull ActionParser.MergeContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#block}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBlock(@NotNull ActionParser.BlockContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isLessThan}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsLessThan(@NotNull ActionParser.IsLessThanContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isBefore}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsBefore(@NotNull ActionParser.IsBeforeContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#unaryPlus}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnaryPlus(@NotNull ActionParser.UnaryPlusContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#length}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLength(@NotNull ActionParser.LengthContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#addToList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAddToList(@NotNull ActionParser.AddToListContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#atTime}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAtTime(@NotNull ActionParser.AtTimeContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#occurWithinFollowing}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOccurWithinFollowing(@NotNull ActionParser.OccurWithinFollowingContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#earliestFrom}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEarliestFrom(@NotNull ActionParser.EarliestFromContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#occurWithinPreceding}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOccurWithinPreceding(@NotNull ActionParser.OccurWithinPrecedingContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#multipleAssignment}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMultipleAssignment(@NotNull ActionParser.MultipleAssignmentContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#reverse}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReverse(@NotNull ActionParser.ReverseContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isDataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsDataType(@NotNull ActionParser.IsDataTypeContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#arctan}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArctan(@NotNull ActionParser.ArctanContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#sqrt}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSqrt(@NotNull ActionParser.SqrtContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#occurWithinPast}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOccurWithinPast(@NotNull ActionParser.OccurWithinPastContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#asNumber}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAsNumber(@NotNull ActionParser.AsNumberContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#divide}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDivide(@NotNull ActionParser.DivideContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#stringVal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringVal(@NotNull ActionParser.StringValContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#replace}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReplace(@NotNull ActionParser.ReplaceContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#arcsin}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArcsin(@NotNull ActionParser.ArcsinContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isWithinTo}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsWithinTo(@NotNull ActionParser.IsWithinToContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#assignment}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAssignment(@NotNull ActionParser.AssignmentContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#switchStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSwitchStatement(@NotNull ActionParser.SwitchStatementContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#maximumFrom}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMaximumFrom(@NotNull ActionParser.MaximumFromContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isWithinSurrounding}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsWithinSurrounding(@NotNull ActionParser.IsWithinSurroundingContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isObjectType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsObjectType(@NotNull ActionParser.IsObjectTypeContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#whileLoop}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhileLoop(@NotNull ActionParser.WhileLoopContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#ago}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAgo(@NotNull ActionParser.AgoContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#decrease}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDecrease(@NotNull ActionParser.DecreaseContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#stdDev}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStdDev(@NotNull ActionParser.StdDevContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#extractChars}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExtractChars(@NotNull ActionParser.ExtractCharsContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#last}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLast(@NotNull ActionParser.LastContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#count}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCount(@NotNull ActionParser.CountContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#numberVal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNumberVal(@NotNull ActionParser.NumberValContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#booleanVal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBooleanVal(@NotNull ActionParser.BooleanValContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#returnStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReturnStatement(@NotNull ActionParser.ReturnStatementContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#round}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRound(@NotNull ActionParser.RoundContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#where}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhere(@NotNull ActionParser.WhereContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isWithinPreceding}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsWithinPreceding(@NotNull ActionParser.IsWithinPrecedingContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#lastFrom}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLastFrom(@NotNull ActionParser.LastFromContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#binaryList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBinaryList(@NotNull ActionParser.BinaryListContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isAfter}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsAfter(@NotNull ActionParser.IsAfterContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#after}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAfter(@NotNull ActionParser.AfterContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#buildString}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBuildString(@NotNull ActionParser.BuildStringContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#uppercase}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUppercase(@NotNull ActionParser.UppercaseContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#occurWithinTo}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOccurWithinTo(@NotNull ActionParser.OccurWithinToContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#earliest}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEarliest(@NotNull ActionParser.EarliestContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#occurWithinSameDay}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOccurWithinSameDay(@NotNull ActionParser.OccurWithinSameDayContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#dayOfWeekFunc}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDayOfWeekFunc(@NotNull ActionParser.DayOfWeekFuncContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#any}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAny(@NotNull ActionParser.AnyContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isWithinSameDay}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsWithinSameDay(@NotNull ActionParser.IsWithinSameDayContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#subList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSubList(@NotNull ActionParser.SubListContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#objNamedWith}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitObjNamedWith(@NotNull ActionParser.ObjNamedWithContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#index}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIndex(@NotNull ActionParser.IndexContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#assignable}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAssignable(@NotNull ActionParser.AssignableContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#continueLoop}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitContinueLoop(@NotNull ActionParser.ContinueLoopContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#init}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInit(@NotNull ActionParser.InitContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#latestFrom}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLatestFrom(@NotNull ActionParser.LatestFromContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#indexFrom}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIndexFrom(@NotNull ActionParser.IndexFromContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#and}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAnd(@NotNull ActionParser.AndContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isGreaterThanOrEqual}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsGreaterThanOrEqual(@NotNull ActionParser.IsGreaterThanOrEqualContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#extractAttrNames}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExtractAttrNames(@NotNull ActionParser.ExtractAttrNamesContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#exp}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExp(@NotNull ActionParser.ExpContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#breakLoop}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBreakLoop(@NotNull ActionParser.BreakLoopContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#sine}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSine(@NotNull ActionParser.SineContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#ceiling}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCeiling(@NotNull ActionParser.CeilingContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isEqual}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsEqual(@NotNull ActionParser.IsEqualContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#maximum}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMaximum(@NotNull ActionParser.MaximumContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#asTime}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAsTime(@NotNull ActionParser.AsTimeContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#tangent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTangent(@NotNull ActionParser.TangentContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#enhancedAssignment}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEnhancedAssignment(@NotNull ActionParser.EnhancedAssignmentContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isGreaterThan}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsGreaterThan(@NotNull ActionParser.IsGreaterThanContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#call}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCall(@NotNull ActionParser.CallContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#occurAfter}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOccurAfter(@NotNull ActionParser.OccurAfterContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#sum}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSum(@NotNull ActionParser.SumContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#add}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAdd(@NotNull ActionParser.AddContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#seqto}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSeqto(@NotNull ActionParser.SeqtoContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#timeOfDayFunc}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimeOfDayFunc(@NotNull ActionParser.TimeOfDayFuncContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#occurWithinSurrounding}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOccurWithinSurrounding(@NotNull ActionParser.OccurWithinSurroundingContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#id}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitId(@NotNull ActionParser.IdContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#newObject}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNewObject(@NotNull ActionParser.NewObjectContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#attributeFrom}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAttributeFrom(@NotNull ActionParser.AttributeFromContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#lowercase}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLowercase(@NotNull ActionParser.LowercaseContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#objOrderedWith}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitObjOrderedWith(@NotNull ActionParser.ObjOrderedWithContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#timeVal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimeVal(@NotNull ActionParser.TimeValContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#trim}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrim(@NotNull ActionParser.TrimContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#exist}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExist(@NotNull ActionParser.ExistContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#dayOfWeek}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDayOfWeek(@NotNull ActionParser.DayOfWeekContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#all}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAll(@NotNull ActionParser.AllContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#cosine}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCosine(@NotNull ActionParser.CosineContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#stmt}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStmt(@NotNull ActionParser.StmtContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#removeFromList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRemoveFromList(@NotNull ActionParser.RemoveFromListContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#multiply}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMultiply(@NotNull ActionParser.MultiplyContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#variance}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVariance(@NotNull ActionParser.VarianceContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#concat}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConcat(@NotNull ActionParser.ConcatContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#nearest}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNearest(@NotNull ActionParser.NearestContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#emptyList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEmptyList(@NotNull ActionParser.EmptyListContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#print}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPrint(@NotNull ActionParser.PrintContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#log10}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLog10(@NotNull ActionParser.Log10Context ctx);
/**
* Visit a parse tree produced by {@link ActionParser#ifStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIfStatement(@NotNull ActionParser.IfStatementContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#currentTime}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCurrentTime(@NotNull ActionParser.CurrentTimeContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#subtract}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSubtract(@NotNull ActionParser.SubtractContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDataType(@NotNull ActionParser.DataTypeContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#duration}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDuration(@NotNull ActionParser.DurationContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#findInString}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFindInString(@NotNull ActionParser.FindInStringContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isNotEqual}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsNotEqual(@NotNull ActionParser.IsNotEqualContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#increase}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIncrease(@NotNull ActionParser.IncreaseContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#raiseToPower}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRaiseToPower(@NotNull ActionParser.RaiseToPowerContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#timeFunc}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTimeFunc(@NotNull ActionParser.TimeFuncContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#latest}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLatest(@NotNull ActionParser.LatestContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#isWithinPast}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsWithinPast(@NotNull ActionParser.IsWithinPastContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#first}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFirst(@NotNull ActionParser.FirstContext ctx);
/**
* Visit a parse tree produced by {@link ActionParser#average}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAverage(@NotNull ActionParser.AverageContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy