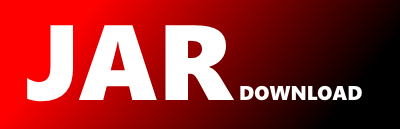
edu.uvm.ccts.jmethodsig.antlr.JMethodSigLexer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arden-parser Show documentation
Show all versions of arden-parser Show documentation
ANTLR grammar and Java logic used to traverse the generated abstract syntax trees related to Arden-syntax Medical Logic Modules (MLMs)
// Generated from JMethodSig.g4 by ANTLR 4.1
package edu.uvm.ccts.jmethodsig.antlr;
import org.antlr.v4.runtime.Lexer;
import org.antlr.v4.runtime.CharStream;
import org.antlr.v4.runtime.Token;
import org.antlr.v4.runtime.TokenStream;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.misc.*;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class JMethodSigLexer extends Lexer {
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
T__6=1, T__5=2, T__4=3, T__3=4, T__2=5, T__1=6, T__0=7, ListType=8, MapType=9,
PrimitiveType=10, ID=11, WS=12;
public static String[] modeNames = {
"DEFAULT_MODE"
};
public static final String[] tokenNames = {
"",
"'>'", "'.'", "')'", "','", "':'", "'('", "'<'", "ListType", "'Map'",
"PrimitiveType", "ID", "WS"
};
public static final String[] ruleNames = {
"T__6", "T__5", "T__4", "T__3", "T__2", "T__1", "T__0", "ListType", "MapType",
"PrimitiveType", "ID", "WS"
};
public JMethodSigLexer(CharStream input) {
super(input);
_interp = new LexerATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
@Override
public String getGrammarFileName() { return "JMethodSig.g4"; }
@Override
public String[] getTokenNames() { return tokenNames; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String[] getModeNames() { return modeNames; }
@Override
public ATN getATN() { return _ATN; }
@Override
public void action(RuleContext _localctx, int ruleIndex, int actionIndex) {
switch (ruleIndex) {
case 11: WS_action((RuleContext)_localctx, actionIndex); break;
}
}
private void WS_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 0: skip(); break;
}
}
public static final String _serializedATN =
"\3\uacf5\uee8c\u4f5d\u8b0d\u4a45\u78bd\u1b2f\u3378\2\16\u0091\b\1\4\2"+
"\t\2\4\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4"+
"\13\t\13\4\f\t\f\4\r\t\r\3\2\3\2\3\3\3\3\3\4\3\4\3\5\3\5\3\6\3\6\3\7\3"+
"\7\3\b\3\b\3\t\3\t\3\t\3\t\3\t\3\t\3\t\3\t\3\t\3\t\3\t\3\t\3\t\3\t\5\t"+
"8\n\t\3\n\3\n\3\n\3\n\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13"+
"\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\5\13Q\n\13\3\13\3\13\3\13"+
"\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13"+
"\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13"+
"\3\13\3\13\3\13\3\13\5\13v\n\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13"+
"\3\13\3\13\5\13\u0082\n\13\3\f\3\f\7\f\u0086\n\f\f\f\16\f\u0089\13\f\3"+
"\r\6\r\u008c\n\r\r\r\16\r\u008d\3\r\3\r\2\16\3\3\1\5\4\1\7\5\1\t\6\1\13"+
"\7\1\r\b\1\17\t\1\21\n\1\23\13\1\25\f\1\27\r\1\31\16\2\3\2\n\4\2DDdd\4"+
"\2UUuu\4\2NNnn\4\2HHhh\4\2FFff\5\2C\\aac|\6\2\62;C\\aac|\5\2\13\f\17\17"+
"\"\"\u009e\2\3\3\2\2\2\2\5\3\2\2\2\2\7\3\2\2\2\2\t\3\2\2\2\2\13\3\2\2"+
"\2\2\r\3\2\2\2\2\17\3\2\2\2\2\21\3\2\2\2\2\23\3\2\2\2\2\25\3\2\2\2\2\27"+
"\3\2\2\2\2\31\3\2\2\2\3\33\3\2\2\2\5\35\3\2\2\2\7\37\3\2\2\2\t!\3\2\2"+
"\2\13#\3\2\2\2\r%\3\2\2\2\17\'\3\2\2\2\21\67\3\2\2\2\239\3\2\2\2\25\u0081"+
"\3\2\2\2\27\u0083\3\2\2\2\31\u008b\3\2\2\2\33\34\7@\2\2\34\4\3\2\2\2\35"+
"\36\7\60\2\2\36\6\3\2\2\2\37 \7+\2\2 \b\3\2\2\2!\"\7.\2\2\"\n\3\2\2\2"+
"#$\7<\2\2$\f\3\2\2\2%&\7*\2\2&\16\3\2\2\2\'(\7>\2\2(\20\3\2\2\2)*\7E\2"+
"\2*+\7q\2\2+,\7n\2\2,-\7n\2\2-.\7g\2\2./\7e\2\2/\60\7v\2\2\60\61\7k\2"+
"\2\61\62\7q\2\2\628\7p\2\2\63\64\7N\2\2\64\65\7k\2\2\65\66\7u\2\2\668"+
"\7v\2\2\67)\3\2\2\2\67\63\3\2\2\28\22\3\2\2\29:\7O\2\2:;\7c\2\2;<\7r\2"+
"\2<\24\3\2\2\2=>\t\2\2\2>?\7{\2\2?@\7v\2\2@\u0082\7g\2\2AB\t\3\2\2BC\7"+
"j\2\2CD\7q\2\2DE\7t\2\2E\u0082\7v\2\2FG\7k\2\2GH\7p\2\2HQ\7v\2\2IJ\7K"+
"\2\2JK\7p\2\2KL\7v\2\2LM\7g\2\2MN\7i\2\2NO\7g\2\2OQ\7t\2\2PF\3\2\2\2P"+
"I\3\2\2\2Q\u0082\3\2\2\2RS\t\4\2\2ST\7q\2\2TU\7p\2\2U\u0082\7i\2\2VW\t"+
"\5\2\2WX\7n\2\2XY\7q\2\2YZ\7c\2\2Z\u0082\7v\2\2[\\\t\6\2\2\\]\7q\2\2]"+
"^\7w\2\2^_\7d\2\2_`\7n\2\2`\u0082\7g\2\2ab\t\2\2\2bc\7q\2\2cd\7q\2\2d"+
"e\7n\2\2ef\7g\2\2fg\7c\2\2g\u0082\7p\2\2hi\7e\2\2ij\7j\2\2jk\7c\2\2kv"+
"\7t\2\2lm\7E\2\2mn\7j\2\2no\7c\2\2op\7t\2\2pq\7c\2\2qr\7e\2\2rs\7v\2\2"+
"st\7g\2\2tv\7t\2\2uh\3\2\2\2ul\3\2\2\2v\u0082\3\2\2\2wx\7U\2\2xy\7v\2"+
"\2yz\7t\2\2z{\7k\2\2{|\7p\2\2|\u0082\7i\2\2}~\7F\2\2~\177\7c\2\2\177\u0080"+
"\7v\2\2\u0080\u0082\7g\2\2\u0081=\3\2\2\2\u0081A\3\2\2\2\u0081P\3\2\2"+
"\2\u0081R\3\2\2\2\u0081V\3\2\2\2\u0081[\3\2\2\2\u0081a\3\2\2\2\u0081u"+
"\3\2\2\2\u0081w\3\2\2\2\u0081}\3\2\2\2\u0082\26\3\2\2\2\u0083\u0087\t"+
"\7\2\2\u0084\u0086\t\b\2\2\u0085\u0084\3\2\2\2\u0086\u0089\3\2\2\2\u0087"+
"\u0085\3\2\2\2\u0087\u0088\3\2\2\2\u0088\30\3\2\2\2\u0089\u0087\3\2\2"+
"\2\u008a\u008c\t\t\2\2\u008b\u008a\3\2\2\2\u008c\u008d\3\2\2\2\u008d\u008b"+
"\3\2\2\2\u008d\u008e\3\2\2\2\u008e\u008f\3\2\2\2\u008f\u0090\b\r\2\2\u0090"+
"\32\3\2\2\2\t\2\67Pu\u0081\u0087\u008d";
public static final ATN _ATN =
ATNSimulator.deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy