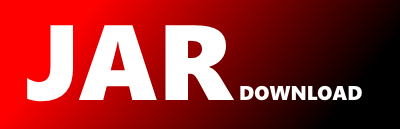
edu.uvm.ccts.common.ui.DisplayProgress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ccts-common Show documentation
Show all versions of ccts-common Show documentation
A library of useful generic objects and tools consolidated here to simplify all UVM CCTS projects
/*
* Copyright 2015 The University of Vermont and State
* Agricultural College. All rights reserved.
*
* Written by Matthew B. Storer
*
* This file is part of CCTS Common.
*
* CCTS Common is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* CCTS Common is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with CCTS Common. If not, see .
*/
/*
* Created by JFormDesigner on Tue Oct 14 14:18:20 EDT 2014
*/
package edu.uvm.ccts.common.ui;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.List;
/**
* @author Matthew Storer
*/
public class DisplayProgress extends JDialog {
private static final Log log = LogFactory.getLog(DisplayProgress.class);
private boolean running = false;
private boolean stopRequested = false;
private String stopMessage = null;
private List statusQueue = new ArrayList();
private Thread statusMonitor;
public DisplayProgress(String title, String desc, int size) {
super((Frame) null);
initComponents();
setTitle(title);
lblDesc.setText("" + desc + "");
progressBar.setMinimum(0);
progressBar.setMaximum(size);
progressBar.setValue(0);
lblMessage.setText("Preparing");
statusMonitor = new Thread(new Runnable() {
@Override
public void run() {
try {
running = true;
while (true) {
Thread.sleep(500);
if (stopRequested) {
progressBar.setValue(progressBar.getMaximum());
if (stopMessage != null) setMessage(stopMessage);
repaint();
break;
} else if (statusQueue.size() > 0) {
if (progressBar.getValue() < progressBar.getMaximum()) {
progressBar.setValue(progressBar.getValue() + 1);
}
setMessage(statusQueue.remove(0));
repaint();
}
}
} catch (Exception e) {
log.error("caught " + e.getClass().getName() + " - " + e.getMessage(), e);
}
startCloseTimer();
}
});
}
public void start() {
if (running) throw new RuntimeException("DisplayProgress has already been started.");
statusMonitor.start();
setVisible(true);
}
public void advance(String message) {
statusQueue.add(message);
}
public void stop(String message) {
stopMessage = message;
stop();
}
public void stop() {
stopRequested = true;
}
public boolean isRunning() {
return running;
}
public boolean isStopped() {
return ! running;
}
public int getProgressBarMin() {
return progressBar.getMinimum();
}
public int getProgressBarMax() {
return progressBar.getMaximum();
}
public int getProgressBarValue() {
return progressBar.getValue();
}
///////////////////////////////////////////////////////////////////////////////////////////////
private void setMessage(String message) {
lblMessage.setText("" + message + "");
}
private void startCloseTimer() {
final Component comp = this;
Timer t = new Timer(1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
comp.setVisible(false);
running = false;
}
});
t.setRepeats(false);
t.start();
}
private void initComponents() {
// JFormDesigner - Component initialization - DO NOT MODIFY //GEN-BEGIN:initComponents
// Generated using JFormDesigner non-commercial license
contentPanel = new JPanel();
lblDesc = new JLabel();
progressBar = new JProgressBar();
lblMessage = new JLabel();
//======== this ========
setDefaultCloseOperation(WindowConstants.DO_NOTHING_ON_CLOSE);
setTitle("");
setAlwaysOnTop(true);
setResizable(false);
Container contentPane = getContentPane();
//======== contentPanel ========
{
//---- lblDesc ----
lblDesc.setText("");
//---- lblMessage ----
lblMessage.setText("");
lblMessage.setFont(new Font("Lucida Grande", Font.ITALIC, 12));
GroupLayout contentPanelLayout = new GroupLayout(contentPanel);
contentPanel.setLayout(contentPanelLayout);
contentPanelLayout.setHorizontalGroup(
contentPanelLayout.createParallelGroup()
.addGroup(contentPanelLayout.createSequentialGroup()
.addContainerGap()
.addGroup(contentPanelLayout.createParallelGroup()
.addComponent(progressBar, GroupLayout.Alignment.TRAILING, GroupLayout.DEFAULT_SIZE, 364, Short.MAX_VALUE)
.addComponent(lblMessage, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(lblDesc, GroupLayout.DEFAULT_SIZE, 364, Short.MAX_VALUE))
.addContainerGap())
);
contentPanelLayout.setVerticalGroup(
contentPanelLayout.createParallelGroup()
.addGroup(contentPanelLayout.createSequentialGroup()
.addContainerGap()
.addComponent(lblDesc)
.addGap(18, 18, 18)
.addComponent(progressBar, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addPreferredGap(LayoutStyle.ComponentPlacement.RELATED)
.addComponent(lblMessage)
.addContainerGap(28, Short.MAX_VALUE))
);
}
GroupLayout contentPaneLayout = new GroupLayout(contentPane);
contentPane.setLayout(contentPaneLayout);
contentPaneLayout.setHorizontalGroup(
contentPaneLayout.createParallelGroup()
.addGroup(contentPaneLayout.createSequentialGroup()
.addGap(24, 24, 24)
.addComponent(contentPanel, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGap(24, 24, 24))
);
contentPaneLayout.setVerticalGroup(
contentPaneLayout.createParallelGroup()
.addGroup(GroupLayout.Alignment.TRAILING, contentPaneLayout.createSequentialGroup()
.addGap(24, 24, 24)
.addComponent(contentPanel, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGap(24, 24, 24))
);
pack();
setLocationRelativeTo(getOwner());
// JFormDesigner - End of component initialization //GEN-END:initComponents
}
// JFormDesigner - Variables declaration - DO NOT MODIFY //GEN-BEGIN:variables
// Generated using JFormDesigner non-commercial license
private JPanel contentPanel;
private JLabel lblDesc;
private JProgressBar progressBar;
private JLabel lblMessage;
// JFormDesigner - End of variables declaration //GEN-END:variables
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy