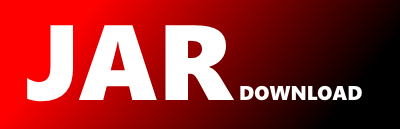
edu.uvm.ccts.common.ui.GetCredentials Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ccts-common Show documentation
Show all versions of ccts-common Show documentation
A library of useful generic objects and tools consolidated here to simplify all UVM CCTS projects
/*
* Copyright 2015 The University of Vermont and State
* Agricultural College. All rights reserved.
*
* Written by Matthew B. Storer
*
* This file is part of CCTS Common.
*
* CCTS Common is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* CCTS Common is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with CCTS Common. If not, see .
*/
/*
* Created by JFormDesigner on Thu Apr 10 09:55:37 EDT 2014
*/
package edu.uvm.ccts.common.ui;
import edu.uvm.ccts.common.util.DialogUtil;
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
/**
* @author Matthew Storer
*/
public class GetCredentials extends JDialog {
private CloseAction closeAction = null;
public GetCredentials(Frame owner, String prompt, String note, String user, String message) {
super(owner);
initComponents();
setup(prompt, note, user, message);
}
public GetCredentials(Dialog owner, String prompt, String note, String user, String message) {
super(owner);
initComponents();
setup(prompt, note, user, message);
}
private void setup(String prompt, String note, String user, String message) {
if (message != null) lblMessage.setText("" + message + "");
else lblMessage.setVisible(false);
lblPrompt.setText("" + prompt + "");
if (note != null) {
lblNoteText.setText("" + note + "");
} else {
lblNote.setVisible(false);
lblNoteText.setVisible(false);
}
if (user != null && ! user.trim().equals("")) {
txtUser.setText(user);
txtUser.setEditable(false);
txtUser.setFocusable(false);
}
}
public String getUser() {
return txtUser.getText();
}
public String getPassword() {
return new String(txtPass.getPassword());
}
public CloseAction getCloseAction() {
return closeAction;
}
private void okButtonActionPerformed() {
String user = txtUser.getText().trim();
if (user.isEmpty()) {
DialogUtil.showErrorDialog(this, "Username cannot be blank.");
txtUser.requestFocus();
} else {
closeAction = CloseAction.OK;
this.setVisible(false);
}
}
private void cancelButtonActionPerformed() {
closeAction = CloseAction.CANCEL;
this.setVisible(false);
}
private void txtUserKeyPressed(KeyEvent e) {
if (e.getKeyCode() == 10) {
okButtonActionPerformed();
} else if (e.getKeyCode() == 27) {
cancelButtonActionPerformed();
}
}
private void txtPassKeyPressed(KeyEvent e) {
if (e.getKeyCode() == 10) {
okButtonActionPerformed();
} else if (e.getKeyCode() == 27) {
cancelButtonActionPerformed();
}
}
public enum CloseAction {
OK,
CANCEL
}
private void initComponents() {
// JFormDesigner - Component initialization - DO NOT MODIFY //GEN-BEGIN:initComponents
// Generated using JFormDesigner non-commercial license
dialogPane = new JPanel();
contentPanel = new JPanel();
lblPrompt = new JLabel();
lblUser = new JLabel();
txtUser = new JTextField();
lblPass = new JLabel();
txtPass = new JPasswordField();
lblMessage = new JLabel();
lblNote = new JLabel();
lblNoteText = new JLabel();
buttonBar = new JPanel();
okButton = new JButton();
cancelButton = new JButton();
//======== this ========
setModal(true);
setTitle("Provide Credentials");
setResizable(false);
setDefaultCloseOperation(WindowConstants.DO_NOTHING_ON_CLOSE);
setAlwaysOnTop(true);
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
//======== dialogPane ========
{
dialogPane.setBorder(new EmptyBorder(12, 12, 12, 12));
dialogPane.setLayout(new BorderLayout());
//======== contentPanel ========
{
//---- lblPrompt ----
lblPrompt.setText("Please provide login credentials for 'x':");
//---- lblUser ----
lblUser.setText("Username:");
//---- txtUser ----
txtUser.addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
txtUserKeyPressed(e);
}
});
//---- lblPass ----
lblPass.setText("Password:");
//---- txtPass ----
txtPass.addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
txtPassKeyPressed(e);
}
});
//---- lblMessage ----
lblMessage.setText("Error message");
lblMessage.setForeground(Color.red);
//---- lblNote ----
lblNote.setText("Note:");
lblNote.setFont(new Font("Lucida Grande", Font.ITALIC, 12));
lblNote.setForeground(Color.darkGray);
//---- lblNoteText ----
lblNoteText.setText("text");
lblNoteText.setForeground(Color.darkGray);
lblNoteText.setFont(new Font("Lucida Grande", Font.ITALIC, 12));
lblNoteText.setVerticalAlignment(SwingConstants.TOP);
GroupLayout contentPanelLayout = new GroupLayout(contentPanel);
contentPanel.setLayout(contentPanelLayout);
contentPanelLayout.setHorizontalGroup(
contentPanelLayout.createParallelGroup()
.addGroup(contentPanelLayout.createSequentialGroup()
.addContainerGap()
.addGroup(contentPanelLayout.createParallelGroup()
.addComponent(lblMessage, GroupLayout.DEFAULT_SIZE, 334, Short.MAX_VALUE)
.addGroup(GroupLayout.Alignment.TRAILING, contentPanelLayout.createSequentialGroup()
.addGroup(contentPanelLayout.createParallelGroup()
.addComponent(lblUser)
.addComponent(lblPass))
.addPreferredGap(LayoutStyle.ComponentPlacement.RELATED)
.addGroup(contentPanelLayout.createParallelGroup()
.addComponent(txtPass, GroupLayout.DEFAULT_SIZE, 258, Short.MAX_VALUE)
.addComponent(txtUser, GroupLayout.DEFAULT_SIZE, 258, Short.MAX_VALUE)))
.addComponent(lblPrompt, GroupLayout.DEFAULT_SIZE, 334, Short.MAX_VALUE)
.addGroup(contentPanelLayout.createSequentialGroup()
.addComponent(lblNote)
.addPreferredGap(LayoutStyle.ComponentPlacement.RELATED)
.addComponent(lblNoteText, GroupLayout.DEFAULT_SIZE, 295, Short.MAX_VALUE)))
.addContainerGap())
);
contentPanelLayout.setVerticalGroup(
contentPanelLayout.createParallelGroup()
.addGroup(contentPanelLayout.createSequentialGroup()
.addContainerGap()
.addComponent(lblMessage)
.addPreferredGap(LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(lblPrompt)
.addPreferredGap(LayoutStyle.ComponentPlacement.RELATED)
.addGroup(contentPanelLayout.createParallelGroup(GroupLayout.Alignment.BASELINE)
.addComponent(lblNote)
.addComponent(lblNoteText))
.addPreferredGap(LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(contentPanelLayout.createParallelGroup(GroupLayout.Alignment.BASELINE)
.addComponent(lblUser)
.addComponent(txtUser, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
.addPreferredGap(LayoutStyle.ComponentPlacement.RELATED)
.addGroup(contentPanelLayout.createParallelGroup(GroupLayout.Alignment.BASELINE)
.addComponent(lblPass)
.addComponent(txtPass, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
.addContainerGap(11, Short.MAX_VALUE))
);
}
dialogPane.add(contentPanel, BorderLayout.CENTER);
//======== buttonBar ========
{
buttonBar.setBorder(new EmptyBorder(12, 0, 0, 0));
buttonBar.setLayout(new GridBagLayout());
((GridBagLayout)buttonBar.getLayout()).columnWidths = new int[] {0, 85, 80};
((GridBagLayout)buttonBar.getLayout()).columnWeights = new double[] {1.0, 0.0, 0.0};
//---- okButton ----
okButton.setText("OK");
okButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
okButtonActionPerformed();
}
});
buttonBar.add(okButton, new GridBagConstraints(1, 0, 1, 1, 0.0, 0.0,
GridBagConstraints.CENTER, GridBagConstraints.BOTH,
new Insets(0, 0, 0, 5), 0, 0));
//---- cancelButton ----
cancelButton.setText("Cancel");
cancelButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
cancelButtonActionPerformed();
}
});
buttonBar.add(cancelButton, new GridBagConstraints(2, 0, 1, 1, 0.0, 0.0,
GridBagConstraints.CENTER, GridBagConstraints.BOTH,
new Insets(0, 0, 0, 0), 0, 0));
}
dialogPane.add(buttonBar, BorderLayout.SOUTH);
}
contentPane.add(dialogPane, BorderLayout.CENTER);
setSize(400, 265);
setLocationRelativeTo(getOwner());
// JFormDesigner - End of component initialization //GEN-END:initComponents
}
// JFormDesigner - Variables declaration - DO NOT MODIFY //GEN-BEGIN:variables
// Generated using JFormDesigner non-commercial license
private JPanel dialogPane;
private JPanel contentPanel;
private JLabel lblPrompt;
private JLabel lblUser;
private JTextField txtUser;
private JLabel lblPass;
private JPasswordField txtPass;
private JLabel lblMessage;
private JLabel lblNote;
private JLabel lblNoteText;
private JPanel buttonBar;
private JButton okButton;
private JButton cancelButton;
// JFormDesigner - End of variables declaration //GEN-END:variables
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy