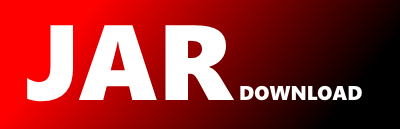
edu.uvm.ccts.common.mail.MailUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ccts-common Show documentation
Show all versions of ccts-common Show documentation
A library of useful generic objects and tools consolidated here to simplify all UVM CCTS projects
/*
* Copyright 2015 The University of Vermont and State
* Agricultural College. All rights reserved.
*
* Written by Matthew B. Storer
*
* This file is part of CCTS Common.
*
* CCTS Common is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* CCTS Common is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with CCTS Common. If not, see .
*/
package edu.uvm.ccts.common.mail;
import org.apache.commons.mail.Email;
import org.apache.commons.mail.EmailException;
import org.apache.commons.mail.SimpleEmail;
import javax.mail.MessagingException;
import javax.mail.Session;
import javax.mail.Transport;
import java.util.Arrays;
import java.util.List;
import java.util.Properties;
/**
* Created by mstorer on 1/16/14.
*/
public class MailUtil {
public static void sendMail(MailConfig mailConfig, String subject, String toAddress,
String message) throws EmailException {
sendMail(mailConfig, subject, Arrays.asList(toAddress), message);
}
public static void sendMail(MailConfig mc, String subject, List toAddressList,
String message) throws EmailException {
Email email = new SimpleEmail();
email.setHostName(mc.getSmtpHost());
email.setSmtpPort(mc.getSmtpPort());
email.setAuthentication(mc.getUser(), mc.getPassword());
if (mc.isUseTLS()) {
email.setStartTLSEnabled(true);
email.setStartTLSRequired(true);
}
email.setSubject(subject);
email.setFrom(mc.getFromAddress());
for (String toAddress : toAddressList) {
email.addTo(toAddress);
}
email.setMsg(message);
email.send();
}
public static void testConnection(MailConfig mc) throws MessagingException {
Properties props = new Properties();
props.put("mail.smtp.host", mc.getSmtpHost());
props.put("mail.smtp.port", mc.getSmtpPort());
props.put("mail.debug", "false");
if (mc.isUseTLS()) {
props.put("mail.smtp.starttls.enable", true);
props.put("mail.smtp.starttls.required", true);
}
Session session = Session.getInstance(props);
Transport tr = session.getTransport("smtp");
try {
tr.connect(mc.getSmtpHost(), mc.getSmtpPort(), mc.getUser(), mc.getPassword());
} finally {
if (tr != null && tr.isConnected()) {
tr.close();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy