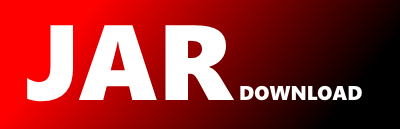
edu.vt.middleware.crypt.CryptProvider Maven / Gradle / Ivy
Show all versions of vt-crypt Show documentation
/*
$Id$
Copyright (C) 2007-2011 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision$
Updated: $Date$
*/
package edu.vt.middleware.crypt;
import java.security.KeyFactory;
import java.security.KeyPairGenerator;
import java.security.KeyStore;
import java.security.KeyStoreException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.security.NoSuchProviderException;
import java.security.Provider;
import java.security.Signature;
import java.security.cert.CertificateException;
import java.security.cert.CertificateFactory;
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.SecretKeyFactory;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* CryptProvider
contains methods for finding cryptographic
* objects using a set of providers.
*
* @author Middleware Services
* @version $Revision: 3 $
*/
public final class CryptProvider
{
/** Default size of random byte array. */
public static final int RANDOM_BYTE_ARRAY_SIZE = 256;
/** List of providers to use. */
private static String[] providers = new String[0];
/**
* Dynamically register the Bouncy Castle provider.
*/
static {
// Bouncy Castle provider
addProvider(new org.bouncycastle.jce.provider.BouncyCastleProvider(), "BC");
}
/** Default constructor.
*/
private CryptProvider() {}
/**
* This will add an additional security provider.
*
* @param provider Provider
* @param name String
*/
public static void addProvider(final Provider provider, final String name)
{
java.security.Security.addProvider(provider);
final String[] tmp = new String[providers.length + 1];
for (int i = 0; i < providers.length; i++) {
tmp[i] = providers[i];
}
tmp[providers.length] = name;
providers = tmp;
final Log logger = LogFactory.getLog(CryptProvider.class);
if (logger.isDebugEnabled()) {
logger.debug("Added new security provider " + name);
}
}
/**
* This finds a Cipher
using the known providers and the
* supplied parameters.
*
* @param algorithm String
name
* @param mode String
name
* @param padding String
name
*
* @return Cipher
*
* @throws CryptException if the algorithm is not available from any
* provider or if the provider is not available in the environment
*/
public static Cipher getCipher(
final String algorithm,
final String mode,
final String padding)
throws CryptException
{
final Log logger = LogFactory.getLog(CryptProvider.class);
Cipher cipher = null;
String transformation = null;
if (mode != null && padding != null) {
transformation = algorithm + "/" + mode + "/" + padding;
} else if (mode != null) {
transformation = algorithm + "/" + mode;
} else {
transformation = algorithm;
}
for (int i = 0; i < providers.length; i++) {
try {
cipher = Cipher.getInstance(transformation, providers[i]);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug(
"Could not find algorithm " + algorithm + " in " + providers[i]);
}
} catch (NoSuchProviderException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find provider " + providers[i]);
}
} catch (NoSuchPaddingException e) {
if (logger.isDebugEnabled()) {
logger.debug(
"Could not find padding " + padding + " in " + providers[i]);
}
} finally {
if (cipher != null) {
break;
}
}
}
if (cipher == null) {
try {
cipher = Cipher.getInstance(transformation);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find algorithm " + algorithm);
}
throw new CryptException(e.getMessage());
} catch (NoSuchPaddingException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find padding " + padding);
}
throw new CryptException(e.getMessage());
}
}
return cipher;
}
/**
* This finds a SecretKeyFactory
using the known providers and
* the supplied algorithm parameter.
*
* @param algorithm String
name
*
* @return SecretKeyFactory
*
* @throws CryptException if the algorithm is not available from any
* provider or if the provider is not available in the environment
*/
public static SecretKeyFactory getSecretKeyFactory(final String algorithm)
throws CryptException
{
final Log logger = LogFactory.getLog(CryptProvider.class);
SecretKeyFactory kf = null;
for (int i = 0; i < providers.length; i++) {
try {
kf = SecretKeyFactory.getInstance(algorithm, providers[i]);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug(
"Could not find algorithm " + algorithm + " in " + providers[i]);
}
} catch (NoSuchProviderException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find provider " + providers[i]);
}
} finally {
if (kf != null) {
break;
}
}
}
if (kf == null) {
try {
kf = SecretKeyFactory.getInstance(algorithm);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find algorithm " + algorithm);
}
throw new CryptException(e.getMessage());
}
}
return kf;
}
/**
* This finds a KeyFactory
using the known providers and the
* supplied algorithm parameter.
*
* @param algorithm String
name
*
* @return KeyFactory
*
* @throws CryptException if the algorithm is not available from any
* provider or if the provider is not available in the environment
*/
public static KeyFactory getKeyFactory(final String algorithm)
throws CryptException
{
final Log logger = LogFactory.getLog(CryptProvider.class);
KeyFactory kf = null;
for (int i = 0; i < providers.length; i++) {
try {
kf = KeyFactory.getInstance(algorithm, providers[i]);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug(
"Could not find algorithm " + algorithm + " in " + providers[i]);
}
} catch (NoSuchProviderException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find provider " + providers[i]);
}
} finally {
if (kf != null) {
break;
}
}
}
if (kf == null) {
try {
kf = KeyFactory.getInstance(algorithm);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find algorithm " + algorithm);
}
throw new CryptException(e.getMessage());
}
}
return kf;
}
/**
* This finds a KeyGenerator
using the known providers and the
* supplied algorithm parameter.
*
* @param algorithm String
name
*
* @return KeyGenerator
*
* @throws CryptException if the algorithm is not available from any
* provider or if the provider is not available in the environment
*/
public static KeyGenerator getKeyGenerator(final String algorithm)
throws CryptException
{
final Log logger = LogFactory.getLog(CryptProvider.class);
KeyGenerator generator = null;
for (int i = 0; i < providers.length; i++) {
try {
generator = KeyGenerator.getInstance(algorithm, providers[i]);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug(
"Could not find algorithm " + algorithm + " in " + providers[i]);
}
} catch (NoSuchProviderException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find provider " + providers[i]);
}
} finally {
if (generator != null) {
break;
}
}
}
if (generator == null) {
try {
generator = KeyGenerator.getInstance(algorithm);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find algorithm " + algorithm);
}
throw new CryptException(e.getMessage());
}
}
return generator;
}
/**
* This finds a KeyPairGenerator
using the known providers and
* the supplied algorithm parameter.
*
* @param algorithm String
name
*
* @return KeyPairGenerator
*
* @throws CryptException if the algorithm is not available from any
* provider or if the provider is not available in the environment
*/
public static KeyPairGenerator getKeyPairGenerator(final String algorithm)
throws CryptException
{
final Log logger = LogFactory.getLog(CryptProvider.class);
KeyPairGenerator generator = null;
for (int i = 0; i < providers.length; i++) {
try {
generator = KeyPairGenerator.getInstance(algorithm, providers[i]);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug(
"Could not find algorithm " + algorithm + " in " + providers[i]);
}
} catch (NoSuchProviderException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find provider " + providers[i]);
}
} finally {
if (generator != null) {
break;
}
}
}
if (generator == null) {
try {
generator = KeyPairGenerator.getInstance(algorithm);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find algorithm " + algorithm);
}
throw new CryptException(e.getMessage());
}
}
return generator;
}
/**
* This finds a Signature
using the known providers and the
* supplied parameters.
*
* @param digestAlgorithm String
name
* @param algorithm String
name
* @param padding String
name
*
* @return Signature
*
* @throws CryptException if the algorithm is not available from any
* provider or if the provider is not available in the environment
*/
public static Signature getSignature(
final String digestAlgorithm,
final String algorithm,
final String padding)
throws CryptException
{
final Log logger = LogFactory.getLog(CryptProvider.class);
Signature sig = null;
String transformation = null;
if (digestAlgorithm != null && padding != null) {
transformation = digestAlgorithm + "/" + algorithm + "/" + padding;
} else if (digestAlgorithm != null) {
transformation = digestAlgorithm + "/" + algorithm;
} else {
transformation = algorithm;
}
for (int i = 0; i < providers.length; i++) {
try {
sig = Signature.getInstance(transformation, providers[i]);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug(
"Could not find algorithm " + algorithm + " in " + providers[i]);
}
} catch (NoSuchProviderException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find provider " + providers[i]);
}
} finally {
if (sig != null) {
break;
}
}
}
if (sig == null) {
try {
sig = Signature.getInstance(transformation);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find algorithm " + algorithm);
}
throw new CryptException(e.getMessage());
}
}
return sig;
}
/**
* This creates a MessageDigest
using the supplied algorithm
* name.
*
* @param algorithm String
name
*
* @return MessageDigest
*
* @throws CryptException if the algorithm is not available from any
* provider or the provider is not available in the environment
*/
public static MessageDigest getMessageDigest(final String algorithm)
throws CryptException
{
final Log logger = LogFactory.getLog(CryptProvider.class);
MessageDigest digest = null;
for (int i = 0; i < providers.length; i++) {
try {
digest = MessageDigest.getInstance(algorithm, providers[i]);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug(
"Could not find algorithm " + algorithm + " in " + providers[i]);
}
} catch (NoSuchProviderException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find provider " + providers[i]);
}
} finally {
if (digest != null) {
break;
}
}
}
if (digest == null) {
try {
digest = MessageDigest.getInstance(algorithm);
} catch (NoSuchAlgorithmException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find algorithm " + algorithm);
}
throw new CryptException(e.getMessage());
}
}
return digest;
}
/**
* This creates a KeyStore
using the supplied type name.
*
* @param type String
*
* @return KeyStore
*
* @throws CryptException if the type is not available from any provider or
* the provider is not available in the environment
*/
public static KeyStore getKeyStore(final String type)
throws CryptException
{
final Log logger = LogFactory.getLog(CryptProvider.class);
KeyStore store = null;
String keyStoreType = type;
if (keyStoreType == null) {
keyStoreType = KeyStore.getDefaultType();
}
for (int i = 0; i < providers.length; i++) {
try {
store = KeyStore.getInstance(keyStoreType, providers[i]);
} catch (KeyStoreException e) {
if (logger.isDebugEnabled()) {
logger.debug(
"Could not get instance of keystore type " + type + " from " +
providers[i]);
}
} catch (NoSuchProviderException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find provider " + providers[i]);
}
} finally {
if (store != null) {
break;
}
}
}
if (store == null) {
try {
store = KeyStore.getInstance(keyStoreType);
} catch (KeyStoreException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not get instance of keystore type " + type);
}
throw new CryptException(e.getMessage());
}
}
return store;
}
/**
* This creates a KeyStore
using the default keystore type.
*
*
* @return KeyStore
*
* @throws CryptException if the default type is not available from any
* provider or the provider is not available in the environment
*/
public static KeyStore getKeyStore()
throws CryptException
{
return getKeyStore(null);
}
/**
* This creates a CertificateFactory
using the supplied type
* name.
*
* @param type String
*
* @return CertificateFactory
*
* @throws CryptException if the type is not available from any provider or
* the provider is not available in the environment
*/
public static CertificateFactory getCertificateFactory(final String type)
throws CryptException
{
final Log logger = LogFactory.getLog(CryptProvider.class);
CertificateFactory cf = null;
for (int i = 0; i < providers.length; i++) {
try {
cf = CertificateFactory.getInstance(type, providers[i]);
} catch (CertificateException e) {
if (logger.isDebugEnabled()) {
logger.debug(
"Could not get instance of certificate factory type " + type +
" from " + providers[i]);
}
} catch (NoSuchProviderException e) {
if (logger.isDebugEnabled()) {
logger.debug("Could not find provider " + providers[i]);
}
} finally {
if (cf != null) {
break;
}
}
}
if (cf == null) {
try {
cf = CertificateFactory.getInstance(type);
} catch (CertificateException e) {
if (logger.isDebugEnabled()) {
logger.debug(
"Could not get instance of certificate factory type " + type);
}
throw new CryptException(e.getMessage());
}
}
return cf;
}
}