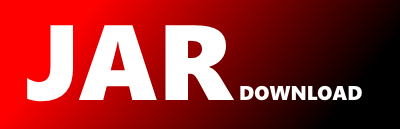
edu.vt.middleware.crypt.io.AbstractEncodedCredentialReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-crypt Show documentation
Show all versions of vt-crypt Show documentation
Library for performing common cryptographic operations
/*
$Id: AbstractEncodedCredentialReader.java 1818 2011-02-08 19:19:09Z dfisher $
Copyright (C) 2007-2011 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1818 $
Updated: $Date: 2011-02-08 14:19:09 -0500 (Tue, 08 Feb 2011) $
*/
package edu.vt.middleware.crypt.io;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import edu.vt.middleware.crypt.CryptException;
import edu.vt.middleware.crypt.util.PemHelper;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.bouncycastle.asn1.DERObjectIdentifier;
/**
* Base class for credential readers that handle credentials that can be
* described as a byte array.
*
* @param Type of credential handled by this class.
*
* @author Middleware Services
* @version $Revision: 1818 $
*/
public abstract class AbstractEncodedCredentialReader
implements CredentialReader
{
/** DSA algorithm OID. */
protected static final DERObjectIdentifier DSA_ID = new DERObjectIdentifier(
"1.2.840.10040.4.1");
/** RSA algorithm OID. */
protected static final DERObjectIdentifier RSA_ID = new DERObjectIdentifier(
"1.2.840.113549.1.1.1");
/** Logger instance. */
protected final Log logger = LogFactory.getLog(getClass());
/** {@inheritDoc} */
public T read(final File file)
throws IOException, CryptException
{
byte[] data = IOHelper.read(new FileInputStream(file).getChannel());
if (PemHelper.isPem(data)) {
data = PemHelper.decode(data);
}
return decode(data);
}
/** {@inheritDoc} */
public T read(final InputStream in)
throws IOException, CryptException
{
byte[] data = IOHelper.read(in);
if (PemHelper.isPem(data)) {
data = PemHelper.decode(data);
}
return decode(data);
}
/**
* Decodes an encoded representation of a credential into a corresponding
* object.
*
* @param encoded Encoded representation of credential.
*
* @return Decoded credential.
*
* @throws CryptException On decoding errors.
*/
protected abstract T decode(final byte[] encoded)
throws CryptException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy