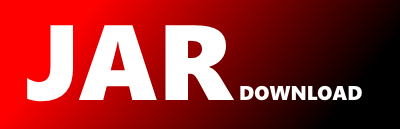
edu.vt.middleware.crypt.io.SecretKeyCredentialReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-crypt Show documentation
Show all versions of vt-crypt Show documentation
Library for performing common cryptographic operations
/*
$Id: SecretKeyCredentialReader.java 1818 2011-02-08 19:19:09Z dfisher $
Copyright (C) 2007-2011 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1818 $
Updated: $Date: 2011-02-08 14:19:09 -0500 (Tue, 08 Feb 2011) $
*/
package edu.vt.middleware.crypt.io;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import edu.vt.middleware.crypt.CryptException;
/**
* Reads symmetric algorithm secret keys.
*
* @author Middleware Services
* @version $Revision: 1818 $
*/
public class SecretKeyCredentialReader implements CredentialReader
{
/** Secret key algorithm. */
private final String algorithm;
/**
* Creates a new instance that can read keys for the given symmetric cipher
* algorithm.
*
* @param cipherAlgorithm Cipher algorithm name, e.g. AES.
*/
public SecretKeyCredentialReader(final String cipherAlgorithm)
{
this.algorithm = cipherAlgorithm;
}
/** {@inheritDoc} */
public SecretKey read(final File file)
throws IOException, CryptException
{
final byte[] data = IOHelper.read(new FileInputStream(file).getChannel());
try {
return new SecretKeySpec(data, algorithm);
} catch (Exception e) {
throw new CryptException("Invalid key format.", e);
}
}
/** {@inheritDoc} */
public SecretKey read(final InputStream in)
throws IOException, CryptException
{
final byte[] data = IOHelper.read(in);
try {
return new SecretKeySpec(data, algorithm);
} catch (Exception e) {
throw new CryptException("Invalid key format.", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy