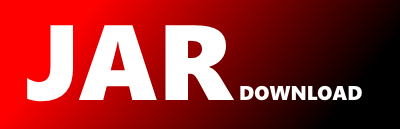
edu.vt.middleware.crypt.tasks.AbstractCryptTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-crypt Show documentation
Show all versions of vt-crypt Show documentation
Library for performing common cryptographic operations
/*
$Id$
Copyright (C) 2007-2011 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision$
Updated: $Date$
*/
package edu.vt.middleware.crypt.tasks;
import java.io.File;
import java.io.IOException;
import edu.vt.middleware.crypt.CryptException;
import edu.vt.middleware.crypt.symmetric.SymmetricAlgorithm;
import edu.vt.middleware.crypt.util.CryptReader;
import edu.vt.middleware.crypt.util.HexConverter;
import org.apache.tools.ant.Task;
/**
* AbstractCryptTask
provides common methods for crypt ant
* tasks.
*
* @author Middleware Services
* @version $Revision: 21 $
*/
public abstract class AbstractCryptTask extends Task
{
/** Property name to set. */
protected String propertyName;
/** Algorithm name. */
protected String algorithm = "AES";
/** Mode used for encryption and decryption. */
protected String mode = "CBC";
/** Padding used for encryption and decryption. */
protected String padding = "PKCS5Padding";
/** Initialization vector for encryption/decryption. */
protected String iv;
/** Private key to read. */
protected File privateKey;
/**
* This sets the name of the property to set with the decrypted value.
*
* @param s String
*/
public void setName(final String s)
{
this.propertyName = s;
}
/**
* This sets the algorithm used for decryption.
*
* @param s String
*/
public void setAlgorithm(final String s)
{
this.algorithm = s;
}
/**
* This sets the mode used for decryption.
*
* @param s String
*/
public void setMode(final String s)
{
this.mode = s;
}
/**
* This sets the padding used for decryption.
*
* @param s String
*/
public void setPadding(final String s)
{
this.padding = s;
}
/**
* This sets the initialization vector used for encryption/decryption.
*
* @param s String
*/
public void setIv(final String s)
{
this.iv = s;
}
/**
* This sets the private key used for decryption.
*
* @param f File
*/
public void setPrivateKey(final File f)
{
this.privateKey = f;
}
/** See @link{org.apache.tools.ant.Task}.
*/
public abstract void execute();
/**
* Creates a new symmetric algorithm cipher and initializes it using task
* properties.
*
* @return New instance ready for use.
*
* @throws CryptException On cryptographic errors.
* @throws IOException On IO errors reading symmetric key.
*/
protected SymmetricAlgorithm createAlgorithm()
throws CryptException, IOException
{
final SymmetricAlgorithm crypt = SymmetricAlgorithm.newInstance(
this.algorithm,
this.mode,
this.padding);
if (this.iv != null) {
crypt.setIV(new HexConverter().toBytes(this.iv));
}
crypt.setKey(CryptReader.readSecretKey(this.privateKey, this.algorithm));
return crypt;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy