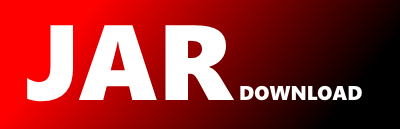
edu.vt.middleware.ldap.auth.Authenticator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: Authenticator.java 1330 2010-05-23 22:10:53Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1330 $
Updated: $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
package edu.vt.middleware.ldap.auth;
import java.io.InputStream;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import javax.naming.NamingException;
import javax.naming.directory.Attributes;
import edu.vt.middleware.ldap.SearchFilter;
import edu.vt.middleware.ldap.auth.handler.AuthenticationResultHandler;
import edu.vt.middleware.ldap.auth.handler.AuthorizationHandler;
import edu.vt.middleware.ldap.auth.handler.CompareAuthorizationHandler;
/**
* Authenticator
contains functions for authenticating a user
* against an LDAP.
*
* @author Middleware Services
* @version $Revision: 1330 $ $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
public class Authenticator extends AbstractAuthenticator
implements Serializable
{
/** serial version uid. */
private static final long serialVersionUID = -444519681288987247L;
/** Default constructor. */
public Authenticator() {}
/**
* This will create a new Authenticator
with the supplied
* AuthenticatorConfig
.
*
* @param authConfig AuthenticatorConfig
*/
public Authenticator(final AuthenticatorConfig authConfig)
{
this.setAuthenticatorConfig(authConfig);
}
/**
* This returns the AuthenticatorConfig
of the
* Authenticator
.
*
* @return AuthenticatorConfig
*/
public AuthenticatorConfig getAuthenticatorConfig()
{
return this.config;
}
/**
* This will set the config parameters of this Authenticator
* using the default properties file, which must be located in your classpath.
*/
public void loadFromProperties()
{
this.setAuthenticatorConfig(AuthenticatorConfig.createFromProperties(null));
}
/**
* This will set the config parameters of this Authenticator
* using the supplied input stream.
*
* @param is InputStream
*/
public void loadFromProperties(final InputStream is)
{
this.setAuthenticatorConfig(AuthenticatorConfig.createFromProperties(is));
}
/**
* This will attempt to find the LDAP DN for the supplied user. {@link
* AuthenticatorConfig#dnResolver} is invoked to perform this operation.
*
* @param user String
to find dn for
*
* @return String
- user's dn
*
* @throws NamingException an LDAP error occurs
*/
public String getDn(final String user)
throws NamingException
{
return this.config.getDnResolver().resolve(user);
}
/**
* This will authenticate by binding to the LDAP using parameters given by
* {@link AuthenticatorConfig#setUser} and {@link
* AuthenticatorConfig#setCredential}. See {@link #authenticate(String,
* Object)}.
*
* @return boolean
- whether the bind succeeded
*
* @throws NamingException if the authentication fails for any other reason
* than invalid credentials
*/
public boolean authenticate()
throws NamingException
{
return
this.authenticate(this.config.getUser(), this.config.getCredential());
}
/**
* This will authenticate by binding to the LDAP with the supplied user and
* credential. If {@link AuthenticatorConfig#setAuthorizationFilter} has been
* called, then it will be used to authorize the user by performing an ldap
* compare. See {@link #authenticate(String, Object, SearchFilter)}.
*
* @param user String
username for bind
* @param credential Object
credential for bind
*
* @return boolean
- whether the bind succeeded
*
* @throws NamingException if the authentication fails for any other reason
* than invalid credentials
*/
public boolean authenticate(final String user, final Object credential)
throws NamingException
{
return
this.authenticate(
user,
credential,
new SearchFilter(
this.config.getAuthorizationFilter(),
this.config.getAuthorizationFilterArgs()));
}
/**
* This will authenticate by binding to the LDAP with the supplied user and
* credential. If the supplied filter is not null it will be injected into a
* new instance of CompareAuthorizationHandler and set as the first
* AuthorizationHandler to execute. If {@link
* AuthenticatorConfig#setAuthenticationResultHandlers(
* AuthenticationResultHandler[])} has been called, then it will be used to
* post process authentication results. See {@link #authenticate(String,
* Object, AuthenticationResultHandler[], AuthorizationHandler[])}.
*
* @param user String
username for bind
* @param credential Object
credential for bind
* @param filter SearchFilter
to authorize user
*
* @return boolean
- whether the bind succeeded
*
* @throws NamingException if the authentication fails for any other reason
* than invalid credentials
*/
public boolean authenticate(
final String user,
final Object credential,
final SearchFilter filter)
throws NamingException
{
final List authzHandler =
new ArrayList();
if (filter != null && filter.getFilter() != null) {
authzHandler.add(new CompareAuthorizationHandler(filter));
}
if (this.config.getAuthorizationHandlers() != null) {
authzHandler.addAll(
Arrays.asList(this.config.getAuthorizationHandlers()));
}
return
this.authenticate(
user,
credential,
this.config.getAuthenticationResultHandlers(),
authzHandler.toArray(new AuthorizationHandler[0]));
}
/**
* This will authenticate by binding to the LDAP with the supplied user and
* credential. The user's DN will be looked up before performing the bind by
* calling {@link DnResolver#resolve(String)}. See {@link
* #authenticateAndAuthorize(String, Object, AuthenticationResultHandler[],
* AuthorizationHandler[])}.
*
* @param user String
username for bind
* @param credential Object
credential for bind
* @param authHandler AuthenticationResultHandler[]
to post
* process authentication results
* @param authzHandler AuthorizationHandler[]
to process
* authorization after authentication
*
* @return boolean
- whether the bind succeeded
*
* @throws NamingException if the authentication fails for any other reason
* than invalid credentials
*/
public boolean authenticate(
final String user,
final Object credential,
final AuthenticationResultHandler[] authHandler,
final AuthorizationHandler[] authzHandler)
throws NamingException
{
return
super.authenticateAndAuthorize(
this.getDn(user),
credential,
authHandler,
authzHandler);
}
/**
* This will authenticate by binding to the LDAP using parameters given by
* {@link AuthenticatorConfig#setUser} and {@link
* AuthenticatorConfig#setCredential}. See {@link
* #authenticate(String,Object,String[])}
*
* @param retAttrs String[]
attributes to return
*
* @return Attributes
- of authenticated user
*
* @throws NamingException if any of the ldap operations fail
*/
public Attributes authenticate(final String[] retAttrs)
throws NamingException
{
return
this.authenticate(
this.config.getUser(),
this.config.getCredential(),
retAttrs);
}
/**
* This will authenticate by binding to the LDAP with the supplied user and
* credential. If {@link AuthenticatorConfig#setAuthorizationFilter} has been
* called, then it will be used to authorize the user by performing an ldap
* compare. See {@link #authenticate(String, Object, SearchFilter, String[])}
*
* @param user String
username for bind
* @param credential Object
credential for bind
* @param retAttrs String[]
to return
*
* @return Attributes
- of authenticated user
*
* @throws NamingException if any of the ldap operations fail
*/
public Attributes authenticate(
final String user,
final Object credential,
final String[] retAttrs)
throws NamingException
{
return
this.authenticate(
user,
credential,
new SearchFilter(
this.config.getAuthorizationFilter(),
this.config.getAuthorizationFilterArgs()),
retAttrs);
}
/**
* This will authenticate by binding to the LDAP with the supplied user and
* credential. If the supplied filter is not null it will be injected into a
* new instance of CompareAuthorizationHandler and set as the first
* AuthorizationHandler to execute. See {@link #authenticate(String, Object,
* String[], AuthenticationResultHandler[], AuthorizationHandler[])}.
*
* @param user String
username for bind
* @param credential Object
credential for bind
* @param filter SearchFilter
to authorize user
* @param retAttrs String[]
to return
*
* @return Attributes
- of authenticated user
*
* @throws NamingException if any of the ldap operations fail
*/
public Attributes authenticate(
final String user,
final Object credential,
final SearchFilter filter,
final String[] retAttrs)
throws NamingException
{
final List authzHandler =
new ArrayList();
if (filter != null && filter.getFilter() != null) {
authzHandler.add(new CompareAuthorizationHandler(filter));
}
if (this.config.getAuthorizationHandlers() != null) {
authzHandler.addAll(
Arrays.asList(this.config.getAuthorizationHandlers()));
}
return
this.authenticate(
user,
credential,
retAttrs,
this.config.getAuthenticationResultHandlers(),
authzHandler.toArray(new AuthorizationHandler[0]));
}
/**
* This will authenticate by binding to the LDAP with the supplied user and
* credential. The user's DN will be looked up before performing the bind by
* calling {@link DnResolver#resolve(String)}. See {@link
* #authenticateAndAuthorize(String, Object, boolean, String[],
* AuthenticationResultHandler[], AuthorizationHandler[])}.
*
* @param user String
username for bind
* @param credential Object
credential for bind
* @param retAttrs String[]
to return
* @param authHandler AuthenticationResultHandler[]
to post
* process authentication results
* @param authzHandler AuthorizationHandler[]
to process
* authorization after authentication
*
* @return Attributes
- of authenticated user
*
* @throws NamingException if any of the ldap operations fail
*/
public Attributes authenticate(
final String user,
final Object credential,
final String[] retAttrs,
final AuthenticationResultHandler[] authHandler,
final AuthorizationHandler[] authzHandler)
throws NamingException
{
return
this.authenticateAndAuthorize(
this.getDn(user),
credential,
true,
retAttrs,
authHandler,
authzHandler);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy