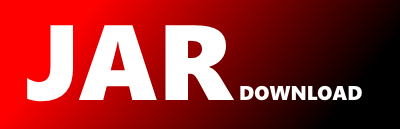
edu.vt.middleware.ldap.dsml.AbstractDsml Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: AbstractDsml.java 1330 2010-05-23 22:10:53Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1330 $
Updated: $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
package edu.vt.middleware.ldap.dsml;
import java.io.IOException;
import java.io.Reader;
import java.io.Serializable;
import java.io.Writer;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
import javax.naming.directory.SearchResult;
import edu.vt.middleware.ldap.LdapUtil;
import edu.vt.middleware.ldap.bean.LdapAttribute;
import edu.vt.middleware.ldap.bean.LdapAttributes;
import edu.vt.middleware.ldap.bean.LdapBeanFactory;
import edu.vt.middleware.ldap.bean.LdapBeanProvider;
import edu.vt.middleware.ldap.bean.LdapEntry;
import edu.vt.middleware.ldap.bean.LdapResult;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.DocumentHelper;
import org.dom4j.Element;
import org.dom4j.Namespace;
import org.dom4j.QName;
import org.dom4j.io.OutputFormat;
import org.dom4j.io.SAXReader;
import org.dom4j.io.XMLWriter;
/**
* AbstractDsml
contains functions for converting LDAP search
* result sets into DSML.
*
* @author Middleware Services
* @version $Revision: 1330 $ $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
public abstract class AbstractDsml implements Serializable
{
/** serial version uid. */
private static final long serialVersionUID = -5626050181955100494L;
/** Log for this class. */
protected final Log logger = LogFactory.getLog(this.getClass());
/** Ldap bean factory. */
protected LdapBeanFactory beanFactory = LdapBeanProvider.getLdapBeanFactory();
/**
* Returns the factory for creating ldap beans.
*
* @return LdapBeanFactory
*/
public LdapBeanFactory getLdapBeanFactory()
{
return this.beanFactory;
}
/**
* Sets the factory for creating ldap beans.
*
* @param lbf LdapBeanFactory
*/
public void setLdapBeanFactory(final LdapBeanFactory lbf)
{
if (lbf != null) {
this.beanFactory = lbf;
}
}
/**
* This will take the results of a prior LDAP query and convert it to a DSML
* Document
.
*
* @param results Iterator
of LDAP search results
*
* @return Document
*/
public abstract Document createDsml(final Iterator results);
/**
* This will take the results of a prior LDAP query and convert it to a DSML
* Document
.
*
* @param result LdapResult
*
* @return Document
*/
public abstract Document createDsml(final LdapResult result);
/**
* This will take an LDAP search result and convert it to a DSML entry
* element.
*
* @param entryName QName
name of element to create
* @param ldapEntry LdapEntry
to convert
* @param ns Namespace
of DSML
*
* @return Document
*/
protected Element createDsmlEntry(
final QName entryName,
final LdapEntry ldapEntry,
final Namespace ns)
{
// create Element to hold result content
final Element entryElement = DocumentHelper.createElement(entryName);
if (ldapEntry != null) {
final String dn = ldapEntry.getDn();
if (dn != null) {
entryElement.addAttribute("dn", dn);
}
for (Element e :
createDsmlAttributes(ldapEntry.getLdapAttributes(), ns)) {
entryElement.add(e);
}
}
return entryElement;
}
/**
* This will return a list of DSML attribute elements from the supplied
* LdapAttributes
.
*
* @param ldapAttributes LdapAttributes
* @param ns Namespace
of DSML
*
* @return List
of elements
*/
protected List createDsmlAttributes(
final LdapAttributes ldapAttributes,
final Namespace ns)
{
final List attrElements = new ArrayList();
for (LdapAttribute attr : ldapAttributes.getAttributes()) {
final String attrName = attr.getName();
final Set> attrValues = attr.getValues();
final Element attrElement = createDsmlAttribute(
attrName,
attrValues,
ns,
"attr",
"name",
"value");
if (attrElement.hasContent()) {
attrElements.add(attrElement);
}
}
return attrElements;
}
/**
* This will take an attribute name and it's values and return a DSML
* attribute element.
*
* @param attrName String
* @param attrValues Set
* @param ns Namespace
of DSML
* @param elementName String
of the attribute element
* @param elementAttrName String
of the attribute element
* @param elementValueName String
of the value element
*
* @return Element
*/
protected Element createDsmlAttribute(
final String attrName,
final Set> attrValues,
final Namespace ns,
final String elementName,
final String elementAttrName,
final String elementValueName)
{
final Element attrElement = DocumentHelper.createElement("");
if (attrName != null) {
attrElement.setQName(new QName(elementName, ns));
if (elementAttrName != null) {
attrElement.addAttribute(elementAttrName, attrName);
}
if (attrValues != null) {
final Iterator> i = attrValues.iterator();
while (i.hasNext()) {
final Object rawValue = i.next();
String value = null;
boolean isBase64 = false;
if (rawValue instanceof String) {
value = (String) rawValue;
} else if (rawValue instanceof byte[]) {
value = LdapUtil.base64Encode((byte[]) rawValue);
isBase64 = true;
} else {
if (this.logger.isWarnEnabled()) {
this.logger.warn(
"Could not cast attribute value as a byte[]" +
" or a String");
}
}
if (value != null) {
final Element valueElement = attrElement.addElement(
new QName(elementValueName, ns));
valueElement.addText(value);
if (isBase64) {
valueElement.addAttribute("encoding", "base64");
}
}
}
}
}
return attrElement;
}
/**
* This will write the supplied LDAP search results to the supplied writer in
* the form of DSML.
*
* @param results Iterator
of LDAP search results
* @param writer Writer
to write to
*
* @throws IOException if an error occurs while writing
*/
public void outputDsml(
final Iterator results,
final Writer writer)
throws IOException
{
final XMLWriter xmlWriter = new XMLWriter(
writer,
OutputFormat.createPrettyPrint());
xmlWriter.write(createDsml(results));
writer.flush();
}
/**
* This will write the supplied LDAP result to the supplied writer in the form
* of DSML.
*
* @param result LdapResult
* @param writer Writer
to write to
*
* @throws IOException if an error occurs while writing
*/
public void outputDsml(final LdapResult result, final Writer writer)
throws IOException
{
final XMLWriter xmlWriter = new XMLWriter(
writer,
OutputFormat.createPrettyPrint());
xmlWriter.write(createDsml(result));
writer.flush();
}
/**
* This will take a Reader containing a DSML Document
and convert
* it to an Iterator of LDAP search results.
*
* @param reader Reader
containing DSML content
*
* @return Iterator
- of LDAP search results
*
* @throws DocumentException if an error occurs building a document from the
* reader
* @throws IOException if an I/O error occurs
*/
public Iterator importDsml(final Reader reader)
throws DocumentException, IOException
{
final Document dsml = new SAXReader().read(reader);
return createLdapResult(dsml).toSearchResults().iterator();
}
/**
* This will take a Reader containing a DSML Document
and convert
* it to an LdapResult
.
*
* @param reader Reader
containing DSML content
*
* @return LdapResult
*
* @throws DocumentException if an error occurs building a document from the
* reader
* @throws IOException if an I/O error occurs
*/
public LdapResult importDsmlToLdapResult(final Reader reader)
throws DocumentException, IOException
{
final Document dsml = new SAXReader().read(reader);
return createLdapResult(dsml);
}
/**
* This will take a DSML Document
and convert it to an Iterator
* of LDAP search results.
*
* @param doc Document
of DSML
*
* @return Iterator
- of LDAP search results
*/
protected abstract LdapResult createLdapResult(final Document doc);
/**
* This will take a DSML Element
containing an entry of type
* and convert it to an LDAP entry.
*
* @param entryElement Element
of DSML content
*
* @return LdapEntry
*/
protected LdapEntry createLdapEntry(final Element entryElement)
{
final LdapEntry ldapEntry = this.beanFactory.newLdapEntry();
ldapEntry.setDn("");
if (entryElement != null) {
final String name = entryElement.attributeValue("dn");
if (name != null) {
ldapEntry.setDn(name);
}
if (entryElement.hasContent()) {
// load the attribute elements
final Iterator> attrIterator = entryElement.elementIterator("attr");
while (attrIterator.hasNext()) {
final Element attrElement = (Element) attrIterator.next();
final String attrName = attrElement.attributeValue("name");
if (attrName != null && attrElement.hasContent()) {
final LdapAttribute ldapAttribute = this.beanFactory
.newLdapAttribute();
ldapAttribute.setName(attrName);
final Iterator> valueIterator = attrElement.elementIterator(
"value");
while (valueIterator.hasNext()) {
final Element valueElement = (Element) valueIterator.next();
final String value = valueElement.getText();
if (value != null) {
final String encoding = valueElement.attributeValue("encoding");
if (encoding != null && "base64".equals(encoding)) {
ldapAttribute.getValues().add(LdapUtil.base64Decode(value));
} else {
ldapAttribute.getValues().add(value);
}
}
}
ldapEntry.getLdapAttributes().addAttribute(ldapAttribute);
}
}
}
}
return ldapEntry;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy