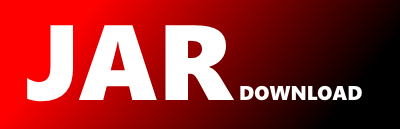
edu.vt.middleware.ldap.dsml.Dsmlv2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: Dsmlv2.java 1330 2010-05-23 22:10:53Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1330 $
Updated: $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
package edu.vt.middleware.ldap.dsml;
import java.util.Iterator;
import javax.naming.NamingException;
import javax.naming.directory.SearchResult;
import edu.vt.middleware.ldap.bean.LdapEntry;
import edu.vt.middleware.ldap.bean.LdapResult;
import org.dom4j.Document;
import org.dom4j.DocumentHelper;
import org.dom4j.Element;
import org.dom4j.Namespace;
import org.dom4j.QName;
/**
* Dsmlv2
contains functions for converting LDAP search result sets
* into DSML version 2.
*
* @author Middleware Services
* @version $Revision: 1330 $ $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
public final class Dsmlv2 extends AbstractDsml
{
/** serial version uid. */
private static final long serialVersionUID = -1503268164295032020L;
/** Default constructor. */
public Dsmlv2() {}
/**
* This will take the results of a prior LDAP query and convert it to a DSML
* Document
.
*
* @param results Iterator
of LDAP search results
*
* @return Document
*/
public Document createDsml(final Iterator results)
{
Document dsml = null;
try {
final LdapResult lr = this.beanFactory.newLdapResult();
lr.addEntries(results);
dsml = this.createDsml(lr);
} catch (NamingException e) {
if (this.logger.isErrorEnabled()) {
this.logger.error("Error creating Element from SearchResult", e);
}
}
return dsml;
}
/**
* This will take the results of a prior LDAP query and convert it to a DSML
* Document
.
*
* @param result LdapResult
*
* @return Document
*/
public Document createDsml(final LdapResult result)
{
final Namespace ns = new Namespace("", "urn:oasis:names:tc:DSML:2:0:core");
final Document doc = DocumentHelper.createDocument();
final Element dsmlElement = doc.addElement(new QName("batchResponse", ns));
final Element entriesElement = dsmlElement.addElement(
new QName("searchResponse", ns));
// build document object from results
if (result != null) {
for (LdapEntry le : result.getEntries()) {
final Element entryElement = this.createDsmlEntry(
new QName("searchResultEntry", ns),
le,
ns);
entriesElement.add(entryElement);
}
}
final Element doneElement = entriesElement.addElement(
new QName("searchResultDone", ns));
final Element codeElement = doneElement.addElement(
new QName("resultCode", ns));
codeElement.addAttribute("code", "0");
return doc;
}
/**
* This will take a DSML Document
and convert it to an Iterator
* of LDAP search results.
*
* @param doc Document
of DSML
*
* @return Iterator
- of LDAP search results
*/
public Iterator createSearchResults(final Document doc)
{
return this.createLdapResult(doc).toSearchResults().iterator();
}
/**
* This will take a DSML Document
and convert it to a
* LdapResult
.
*
* @param doc Document
of DSML
*
* @return LdapResult
*/
public LdapResult createLdapResult(final Document doc)
{
final LdapResult result = this.beanFactory.newLdapResult();
if (doc != null && doc.hasContent()) {
final Iterator> entryIterator = doc.selectNodes(
"/*[name()='batchResponse']" +
"/*[name()='searchResponse']" +
"/*[name()='searchResultEntry']").iterator();
while (entryIterator.hasNext()) {
final LdapEntry le = this.createLdapEntry(
(Element) entryIterator.next());
if (le != null) {
result.addEntry(le);
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy