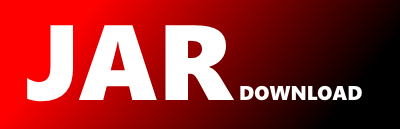
edu.vt.middleware.ldap.handler.CopySearchResultHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: CopySearchResultHandler.java 1786 2011-01-05 14:45:07Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1786 $
Updated: $Date: 2011-01-05 09:45:07 -0500 (Wed, 05 Jan 2011) $
*/
package edu.vt.middleware.ldap.handler;
import javax.naming.NamingException;
import javax.naming.directory.Attributes;
import javax.naming.directory.SearchResult;
/**
* CopySearchResultHandler
converts a NamingEnumeration of search
* results into a List of search results.
*
* @author Middleware Services
* @version $Revision: 1786 $ $Date: 2011-01-05 09:45:07 -0500 (Wed, 05 Jan 2011) $
*/
public class CopySearchResultHandler extends CopyResultHandler
implements SearchResultHandler
{
/** Attribute handler. */
private AttributeHandler[] attributeHandler;
/** {@inheritDoc} */
public AttributeHandler[] getAttributeHandler()
{
return this.attributeHandler;
}
/** {@inheritDoc} */
public void setAttributeHandler(final AttributeHandler[] ah)
{
this.attributeHandler = ah;
}
/**
* This will return a deep copy of the supplied SearchResult
.
*
* @param sc SearchCriteria
used to find enumeration
* @param sr SearchResult
to copy
*
* @return SearchResult
*
* @throws NamingException if the result cannot be read
*/
protected SearchResult processResult(
final SearchCriteria sc,
final SearchResult sr)
throws NamingException
{
return
new SearchResult(
this.processDn(sc, sr),
sr.getClassName(),
sr.getObject(),
this.processAttributes(sc, sr),
sr.isRelative());
}
/**
* Process the dn of an ldap search result.
*
* @param sc SearchCriteria
used to find search result
* @param sr SearchResult
to extract the dn from
*
* @return String
processed dn
*/
protected String processDn(final SearchCriteria sc, final SearchResult sr)
{
return sr.getName();
}
/**
* Process the attributes of an ldap search.
*
* @param sc SearchCriteria
used to find search result
* @param sr SearchResult
to extract the attributes from
*
* @return Attributes
processed attributes
*
* @throws NamingException if the LDAP returns an error
*/
protected Attributes processAttributes(
final SearchCriteria sc,
final SearchResult sr)
throws NamingException
{
Attributes newAttrs = sr.getAttributes();
if (this.attributeHandler != null && this.attributeHandler.length > 0) {
for (AttributeHandler ah : this.attributeHandler) {
newAttrs = AttributesProcessor.executeHandler(sc, newAttrs, ah);
}
}
return newAttrs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy