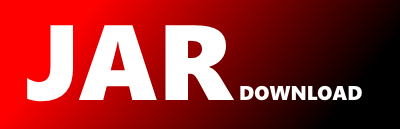
edu.vt.middleware.ldap.handler.DefaultConnectionHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: DefaultConnectionHandler.java 1442 2010-07-01 18:05:58Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1442 $
Updated: $Date: 2010-07-01 14:05:58 -0400 (Thu, 01 Jul 2010) $
*/
package edu.vt.middleware.ldap.handler;
import java.util.Hashtable;
import javax.naming.NamingException;
import javax.naming.ldap.InitialLdapContext;
import edu.vt.middleware.ldap.LdapConfig;
import edu.vt.middleware.ldap.LdapConstants;
/**
* DefaultConnectionHandler
creates a new LdapContext
* using environment properties obtained from {@link
* LdapConfig#getEnvironment()}.
*
* @author Middleware Services
* @version $Revision: 1442 $
*/
public class DefaultConnectionHandler extends AbstractConnectionHandler
{
/** Default constructor. */
public DefaultConnectionHandler() {}
/**
* Creates a new DefaultConnectionHandler
with the supplied ldap
* config.
*
* @param lc ldap config
*/
public DefaultConnectionHandler(final LdapConfig lc)
{
this.setLdapConfig(lc);
}
/**
* Copy constructor for DefaultConnectionHandler
.
*
* @param ch to copy properties from
*/
public DefaultConnectionHandler(final DefaultConnectionHandler ch)
{
this.setLdapConfig(ch.getLdapConfig());
this.setConnectionStrategy(ch.getConnectionStrategy());
this.setConnectionRetryExceptions(ch.getConnectionRetryExceptions());
this.setConnectionCount(ch.getConnectionCount());
}
/** {@inheritDoc} */
protected void connectInternal(
final String authtype,
final String dn,
final Object credential,
final Hashtable env)
throws NamingException
{
if (this.logger.isDebugEnabled()) {
this.logger.debug("Bind with the following parameters:");
this.logger.debug(" authtype = " + authtype);
this.logger.debug(" dn = " + dn);
if (this.config.getLogCredentials()) {
if (this.logger.isDebugEnabled()) {
this.logger.debug(" credential = " + credential);
}
} else {
if (this.logger.isDebugEnabled()) {
this.logger.debug(" credential = ");
}
}
if (this.logger.isTraceEnabled()) {
this.logger.trace(" env = " + env);
}
}
// note that when using simple authentication (the default),
// if the credential is null the provider will automatically revert the
// authentication to none
env.put(LdapConstants.AUTHENTICATION, authtype);
if (dn != null) {
env.put(LdapConstants.PRINCIPAL, dn);
if (credential != null) {
env.put(LdapConstants.CREDENTIALS, credential);
}
}
try {
this.context = new InitialLdapContext(env, null);
} catch (NamingException e) {
if (this.context != null) {
try {
this.context.close();
} finally {
this.context = null;
}
}
throw e;
}
}
/** {@inheritDoc} */
public DefaultConnectionHandler newInstance()
{
return new DefaultConnectionHandler(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy