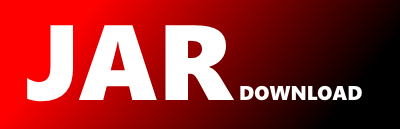
edu.vt.middleware.ldap.handler.EntryDnSearchResultHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: EntryDnSearchResultHandler.java 1330 2010-05-23 22:10:53Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1330 $
Updated: $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
package edu.vt.middleware.ldap.handler;
import javax.naming.NamingException;
import javax.naming.directory.Attributes;
import javax.naming.directory.SearchResult;
/**
* EntryDnSearchResultHandler
adds the search result DN as an
* attribute to the result set. Provides a client side implementation of RFC
* 5020.
*
* @author Middleware Services
* @version $Revision: 1330 $ $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
public class EntryDnSearchResultHandler extends CopySearchResultHandler
{
/**
* Attribute name for the entry dn. The value of this constant is {@value}.
*/
private String dnAttributeName = "entryDN";
/**
* Whether to add the entry dn if an attribute of the same name exists. The
* value of this constant is {@value}.
*/
private boolean addIfExists;
/**
* Returns the DN attribute name.
*
* @return String
*/
public String getDnAttributeName()
{
return this.dnAttributeName;
}
/**
* Sets the DN attribute name.
*
* @param s String
*/
public void setDnAttributeName(final String s)
{
this.dnAttributeName = s;
}
/**
* Returns whether to add the entryDN if an attribute of the same name exists.
*
* @return boolean
*/
public boolean isAddIfExists()
{
return this.addIfExists;
}
/**
* Sets whether to add the entryDN if an attribute of the same name exists.
*
* @param b boolean
*/
public void setAddIfExists(final boolean b)
{
this.addIfExists = b;
}
/** {@inheritDoc} */
protected Attributes processAttributes(
final SearchCriteria sc,
final SearchResult sr)
throws NamingException
{
final Attributes newAttrs = sr.getAttributes();
if (newAttrs.get(this.dnAttributeName) == null) {
newAttrs.put(this.dnAttributeName, sr.getName());
} else if (this.addIfExists) {
newAttrs.get(this.dnAttributeName).add(sr.getName());
}
return newAttrs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy